Mastering Async State Testing in Java: Key Strategies
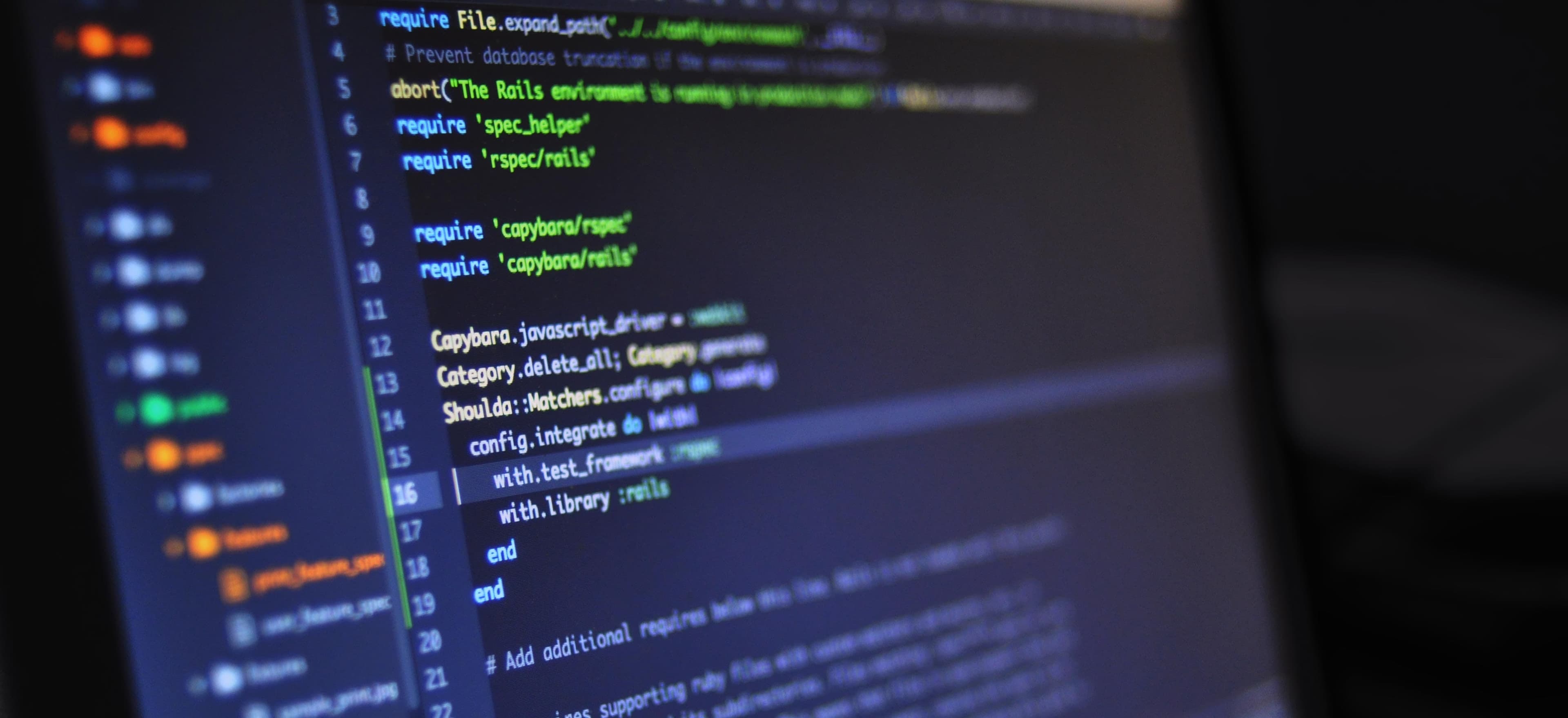
- Published on
Mastering Async State Testing in Java: Key Strategies
When it comes to developing modern applications, asynchronous programming is essential. It allows your programs to manage multiple tasks simultaneously, making them more responsive and efficient. However, testing asynchronous state changes presents unique challenges. If you ever struggled with testing async state changes, you might find yourself in the same boat as those who are “Struggling with Testing Async State Changes in Svelte?” A comprehensive understanding of async testing can significantly elevate your Java development skills.
In this article, we will cover essential strategies to navigate the complexities of asynchronous state testing in Java, including best practices, tools, and code examples.
Understanding Asynchronous Programming in Java
Asynchronous programming allows your application to perform operations without blocking the execution of the main thread. This is often achieved through the use of features such as:
- Threads
- Futures
- CompletableFutures
- Reactive Programming with RxJava and Project Reactor
Using these constructs effectively can enhance the performance of your applications, but they also introduce complications when it comes to testing.
Why Testing Async State Changes is Challenging
Testing asynchronous code is harder than synchronous code due to:
- Race Conditions: The order of execution is non-deterministic, making it tricky to ensure your tests execute correctly each time.
- Delayed Execution: Async operations may not complete in the test-run time, leading to flaky tests.
- State Management: Changes to the application's state may occur out of sync with expectations, complicating the testing process.
Understanding these challenges is the first step in mastering async state testing.
Best Practices for Async State Testing
To effectively test asynchronous state changes in Java, consider implementing the following best practices:
1. Use CompletableFuture
CompletableFuture
enables non-blocking asynchronous programming. It provides methods to control task execution and manage results seamlessly.
Example: Basic CompletableFuture Usage
import java.util.concurrent.CompletableFuture;
public class AsyncDemo {
public static CompletableFuture<String> fetchData() {
return CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(1000); // Simulate long-running task
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Data fetched";
});
}
public static void main(String[] args) {
CompletableFuture<String> future = fetchData();
future.thenAccept(System.out::println);
}
}
Why This Matters: This code demonstrates how to execute a background task and handle the result when it's ready. Tests can be designed to assert the final result effectively.
2. Apply Awaitility for Asynchronous Testing
Awaitility is an excellent library for testing asynchronous behavior in Java. It allows you to write concise and readable tests that wait for a condition to be true, subscribing to a more functional style.
Example: Testing Asynchronous Results with Awaitility
import static org.awaitility.Awaitility.await;
import static java.util.concurrent.TimeUnit.SECONDS;
import java.util.concurrent.CompletableFuture;
public class AsyncTest {
private CompletableFuture<String> future = new CompletableFuture<>();
public void simulateAsyncTask() {
// Simulate task completion after 1 second
CompletableFuture.runAsync(() -> {
try {
Thread.sleep(1000);
future.complete("Completed");
} catch (InterruptedException e) {
future.completeExceptionally(e);
}
});
}
@org.junit.Test
public void testAsync() {
simulateAsyncTask();
await().atMost(3, SECONDS)
.until(future::isDone);
assert future.isDone();
assert "Completed".equals(future.join());
}
}
Why This Matters: This test uses Awaitility to wait up to three seconds for the CompletableFuture
to complete. This eliminates flakiness and helps create reliable tests for async operations.
3. Mocking Async Dependencies
When dealing with asynchronous code, you may need to mock services that provide the asynchronous response. Libraries like Mockito allow you to easily create mocks to control the execution flow.
Example: Mocking an Asynchronous Service
import static org.mockito.Mockito.*;
import java.util.concurrent.CompletableFuture;
public class AsyncServiceTest {
interface AsyncService {
CompletableFuture<String> fetchFromService();
}
public void testMockAsyncService() {
AsyncService asyncService = mock(AsyncService.class);
when(asyncService.fetchFromService()).thenReturn(CompletableFuture.completedFuture("Mocked Data"));
CompletableFuture<String> result = asyncService.fetchFromService();
assert "Mocked Data".equals(result.join());
}
}
Why This Matters: By mocking asynchronous services, you can isolate the code under test, leading to more focused and effective tests.
4. Utilizing Project Reactor for Advanced Asynchronous Testing
For more complex use cases, especially in reactive programming, consider Project Reactor. This library allows developers to compose and process streams of data in a non-blocking way.
Example: Testing a Reactive Stream
import reactor.core.publisher.Mono;
import reactor.test.StepVerifier;
public class ReactiveServiceTest {
public Mono<String> getData() {
return Mono.just("Reactive Data");
}
@org.junit.Test
public void testReactiveService() {
Mono<String> data = getData();
StepVerifier.create(data)
.expectNext("Reactive Data")
.verifyComplete();
}
}
Why This Matters: StepVerifier allows you to create tests that adhere to the reactive principles, ensuring that your streams behave as expected.
In Conclusion, Here is What Matters
Testing asynchronous state changes can be a daunting task, but understanding the right strategies can make the process much easier. By utilizing features like CompletableFuture
, Awaitility, Mockito, and components from Project Reactor, you can create effective and reliable tests for your asynchronous Java applications.
For developers tackling challenges similar to those noted in the article “Struggling with Testing Async State Changes in Svelte?”, acknowledging how these principles apply in other languages and frameworks can provide a broader understanding of async testing methodologies.
Make sure to explore further and expand your testing toolkit. For a detailed guide on testing async state changes in Svelte, check out the article here.
Now that you’ve equipped yourself with these strategies, go forth and master async testing in your Java applications! Happy coding!