Simplifying Java-Based UI: Efficient Div Toggling Strategies
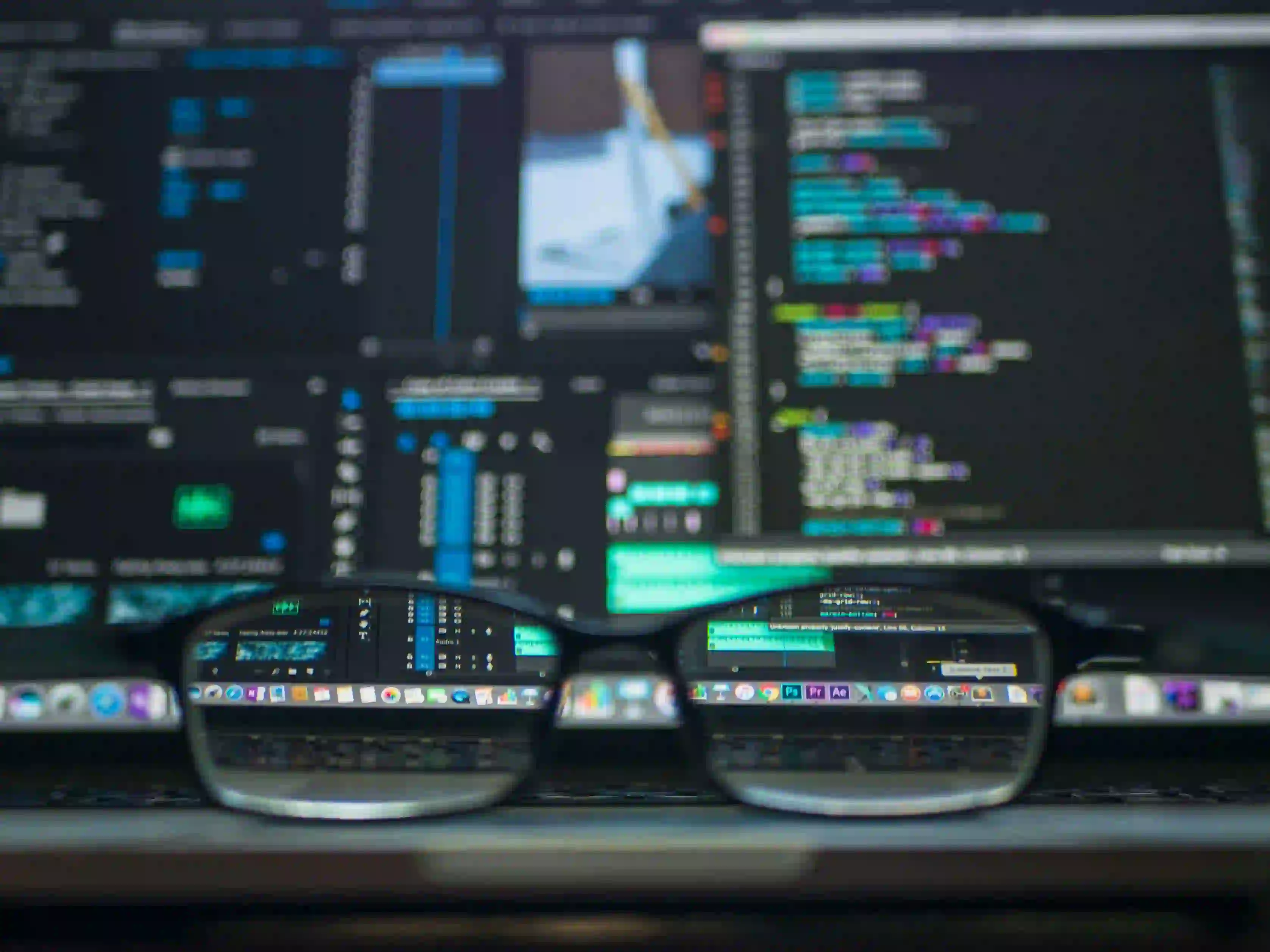
Simplifying Java-Based UI: Efficient Div Toggling Strategies
In the evolving landscape of UI development, having a dynamic and responsive interface is essential. Java, with its robust capabilities, empowers developers to create interactive applications. One common challenge that developers face is efficiently toggling div elements in user interfaces. In this blog post, we will explore the strategies for effective div toggling in Java-based applications, drawing on insights from existing practices, including concepts covered in "Mastering UI: Seamless Div Toggling Techniques" (infinitejs.com/posts/mastering-ui-seamless-div-toggling-techniques).
Understanding Div Toggling in Java
Div toggling refers to the functionality that allows developers to show or hide elements (divs) in a web interface. This practice is crucial in creating a clean and user-friendly experience. In Java-based applications, especially those using frameworks like JavaFX or Swing, the approach to achieving seamless transitions can vary significantly.
Why is Div Toggling Important?
- User Experience: Properly implemented toggling can greatly improve user interaction with the application.
- Space Management: Keeping interfaces neat by hiding non-essential elements until they are needed enhances the overall aesthetics of the UI.
- Performance: Efficient toggling reduces the unnecessary rendering of off-screen elements, making applications faster and more responsive.
Basic Structure of a Java Application with Div Toggling
Whether you are using JavaFX or Swing, the core logic remains similar. Below, we examine a simple JavaFX application that demonstrates div toggling through button actions.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class DivTogglingExample extends Application {
private boolean isVisible = false; // Toggle state
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
VBox layout = new VBox(); // Main layout
Button toggleButton = new Button("Toggle Div");
Button contentDiv = new Button("Content Div "); // Represents a div
// Set initial visibility
contentDiv.setVisible(isVisible);
toggleButton.setOnAction(e -> {
isVisible = !isVisible; // Toggle the state
contentDiv.setVisible(isVisible); // Change visibility
});
layout.getChildren().addAll(toggleButton, contentDiv); // Add buttons to layout
Scene scene = new Scene(layout, 300, 200); // Define scene size
primaryStage.setScene(scene);
primaryStage.setTitle("Div Toggling Example");
primaryStage.show();
}
}
Code Explanation
-
Boolean Variable: Here,
isVisible
keeps track of whether the content div should be displayed. This toggling logic is straightforward and provides a clear basis for managing the visibility state. -
Button Actions: When the button is clicked, the visibility of the content div changes based on the current state. This encapsulates the basic behavior of toggling.
-
Flexible Layout: The use of
VBox
allows for easy vertical stacking of UI elements, making the code cleaner and easier to read.
Enhancing User Feedback with Animation
While the above example demonstrates basic functionality, adding user experience (UX) features like animations can significantly enhance the effectiveness of div toggling. Animations can help users understand changes happening in the UI.
Here is an extended version of the previous example, adding fade-in and fade-out animations:
import javafx.animation.FadeTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.util.Duration;
public class AnimatedDivTogglingExample extends Application {
private boolean isVisible = false;
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
VBox layout = new VBox();
Button toggleButton = new Button("Toggle Div");
Button contentDiv = new Button("Content Div");
contentDiv.setVisible(isVisible); // Set initial visibility
toggleButton.setOnAction(e -> {
FadeTransition fade = new FadeTransition(Duration.millis(300), contentDiv);
if (isVisible) {
fade.setFromValue(1.0); // Fully visible
fade.setToValue(0.0); // Fading out to invisible
} else {
fade.setFromValue(0.0); // Fully invisible
fade.setToValue(1.0); // Fading in to visible
contentDiv.setVisible(true);
}
fade.play();
fade.setOnFinished(event -> isVisible = !isVisible); // Update toggle state
});
layout.getChildren().addAll(toggleButton, contentDiv);
Scene scene = new Scene(layout, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Animated Div Toggling Example");
primaryStage.show();
}
}
Code Explanation
-
FadeTransition: This class creates a smooth transition effect. The
setFromValue
andsetToValue
methods control the visibility dynamically, allowing for a seamless user experience. -
Animation Duration: Specifying a duration makes the interaction feel polished. A fade-in takes place for 300 milliseconds, which is often considered optimal for user feedback; it's neither too slow nor too fast.
Best Practices for Div Toggling in Java
-
State Management: Always track the state of the div's visibility. Employ Boolean flags or enumerations where appropriate to maintain clarity in your code.
-
User-Centric Design: Integrate visual cues (like animations or color changes) that signal to users when actions are taken, improving engagement and response.
-
Accessibility: Ensure that toggling actions are accessible through keyboard shortcuts and are screen-reader friendly. This is especially crucial for application adoption across diverse user demographics.
-
Performance Testing: As your UI grows complex, continuously test for performance issues related to toggling. Use profiling tools to identify and resolve any slowdowns.
-
Reusability: Consider abstracting common toggle functions into utility classes or methods to keep your code DRY (Don't Repeat Yourself). For example, you might develop a reusable
toggleVisibility(Node node)
method.
Wrapping Up
Div toggling plays a crucial role in crafting interactive Java-based user interfaces. By implementing strategies like state management and animations, developers can enhance user experience significantly. The examples provided illustrate basic toggling functionalities and how to enrich them with animations to elevate user engagement.
For more insights on seamless techniques for div toggling, visit the informative article, "Mastering UI: Seamless Div Toggling Techniques," at infinitejs.com/posts/mastering-ui-seamless-div-toggling-techniques.
By applying the strategies discussed, developers can ensure that their Java applications are not only functional but also engaging and user-friendly, paving the way for successful UI design outcomes.
Happy coding!