Mastering PreparedStatement Placeholders in Oracle JDBC
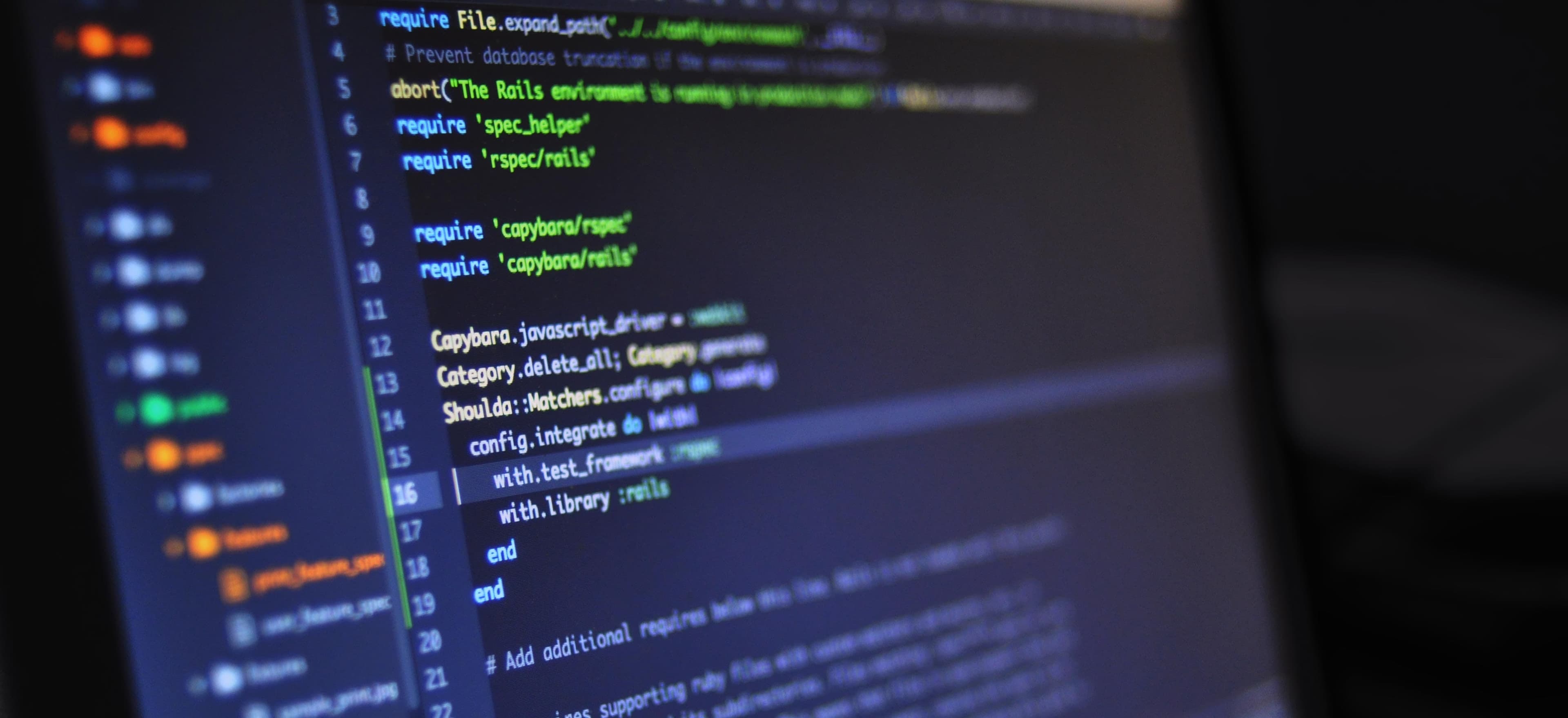
- Published on
Mastering PreparedStatement Placeholders in Oracle JDBC
When dealing with databases in Java, efficient data manipulation is key. In this realm, the PreparedStatement
class in Java Database Connectivity (JDBC) comes into play as an essential tool for managing SQL statements. One of the most powerful features of PreparedStatement
is its ability to use placeholders. This blog post will explore how to effectively use PreparedStatement placeholders in Oracle JDBC, offering practical examples along the way.
Understanding PreparedStatement and Its Benefits
PreparedStatement
is a sub-interface of Statement
that allows you to execute precompiled SQL statements. The main advantages of using PreparedStatement
include:
- Performance: SQL statements are precompiled, which can boost performance, particularly for repeated execution.
- Security: PreparedStatements help prevent SQL injection attacks by separating SQL logic from data.
- Clarity: Placeholders make the code cleaner and clearer, enhancing readability.
Did You Know? PreparedStatements are only slightly less efficient in Oracle than native PL/SQL because the database can caché them during execution.
Syntax Overview
A common pattern when using PreparedStatement
is to define SQL statements with placeholders represented by ?
for parameters:
String sql = "SELECT * FROM employees WHERE department_id = ? AND salary > ?";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
In this example, the ?
will be replaced with actual parameter values at runtime.
Setting Values for Placeholders
Once the PreparedStatement
has been created, values can be assigned to its placeholders using the setXXX()
methods, where XXX
indicates the data type being set. Here’s how you can set the parameters safely:
preparedStatement.setInt(1, 5); // Setting integer value for department_id
preparedStatement.setDouble(2, 50000.00); // Setting double value for salary
The first argument corresponds to the position of the placeholder in the SQL statement.
Example: Using PreparedStatement Placeholders with Oracle JDBC
Let’s construct a full example to demonstrate how we can use PreparedStatement
in Java to fetch employees from the database based on their department and salary.
Step 1: Setting Up Connection
Before we dive into the SQL execution, we need to set up our database connection:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class EmployeeFetcher {
private static final String DB_URL = "jdbc:oracle:thin:@localhost:1521:xe";
private static final String USER = "username";
private static final String PASS = "password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(DB_URL, USER, PASS);
}
}
Why: It is crucial to isolate the connection logic to maintain a clean separation of concerns.
Step 2: Fetching Employees
Here's a method that utilizes the PreparedStatement
to retrieve employees based on department and salary:
public void fetchEmployees(int departmentId, double salaryThreshold) {
String sql = "SELECT employee_id, employee_name FROM employees WHERE department_id = ? AND salary > ?";
try (Connection connection = getConnection();
PreparedStatement preparedStatement = connection.prepareStatement(sql)) {
preparedStatement.setInt(1, departmentId);
preparedStatement.setDouble(2, salaryThreshold);
try (ResultSet resultSet = preparedStatement.executeQuery()) {
while (resultSet.next()) {
int employeeId = resultSet.getInt("employee_id");
String employeeName = resultSet.getString("employee_name");
System.out.println("Employee ID: " + employeeId + ", Name: " + employeeName);
}
}
} catch (SQLException e) {
e.printStackTrace();
}
}
Explanation:
- Connection Management: We use a try-with-resources statement to ensure that our database resources are closed automatically.
- Parameters Setting: Parameters are set in the correct order and types using
setInt
andsetDouble
. - Executing Query: The execution of the query is straightforward with
executeQuery()
on thePreparedStatement
.
Step 3: Running and Testing the Code
To execute this method and see it in action, you can create a main method:
public static void main(String[] args) {
EmployeeFetcher fetcher = new EmployeeFetcher();
fetcher.fetchEmployees(5, 50000.00);
}
This execution will print out the employees who belong to department 5 and have a salary greater than 50,000.
Best Practices for Using PreparedStatement
- Always Use Placeholders: Avoid concatenating SQL statements with user input. Always use placeholders to safeguard against SQL injection.
- Mind the Data Types: Ensure you are using the correct data types for
setXXX()
methods for the parameters. - Reuse PreparedStatements: If possible, reuse
PreparedStatement
instances, especially in repeated executions, as it can reduce database load.
Final Thoughts
Mastering PreparedStatement
placeholders in Oracle JDBC can significantly streamline your database interactions in Java applications. Not only does it provide performance improvements, but it also enhances security and clarity in your code.
Using these best practices and understanding the principles behind PreparedStatement
will enable you to build robust, secure, and efficient data-driven applications. For more detailed JDBC information, check the Oracle JDBC Developer’s Guide.
Happy coding!
Checkout our other articles