Mastering Gradle Integration Testing: Common Pitfalls to Avoid
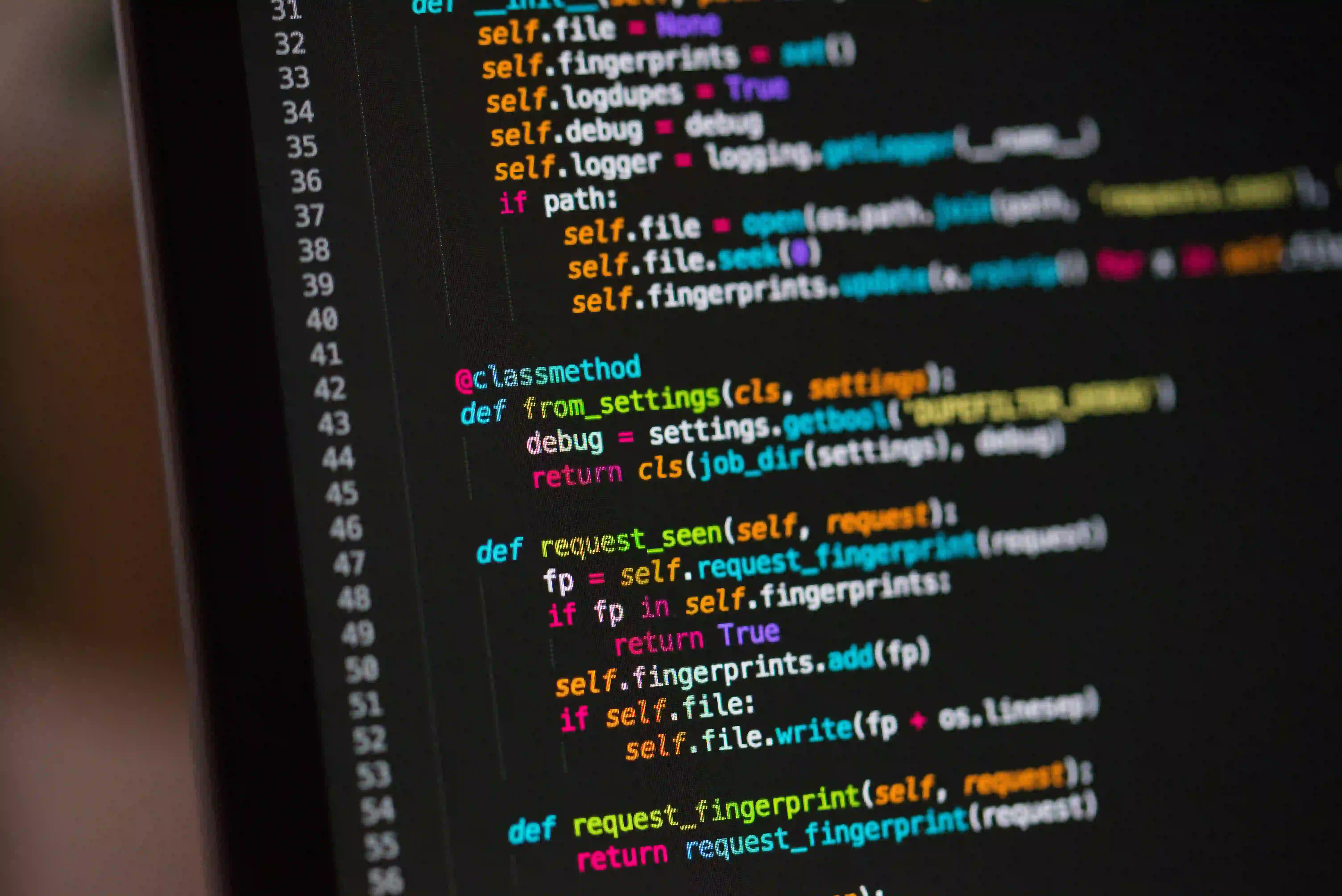
Mastering Gradle Integration Testing: Common Pitfalls to Avoid
Integration testing is a crucial phase in software development. It ensures that individual modules work together as expected, protecting against regressions and ensuring overall system functionality. When using Gradle, a powerful build automation tool, to manage your projects, understanding the integration testing lifecycle becomes essential. However, there are common pitfalls that developers often encounter.
This blog post will explore those pitfalls, offering solutions and best practices to avoid them. By the end of this article, you'll have a better sense of how to effectively implement integration testing in Gradle-based projects.
What is Gradle?
Gradle is an open-source build automation tool designed for multi-language software development. It provides a flexible model for managing builds, including support for Java, Groovy, Kotlin, and Scala. With Gradle, you can automate the compilation, testing, and deployment processes.
Integration tests in Gradle can be seamlessly integrated into the workflow, ensuring that your code is constantly verified against interoperability issues.
Why Integration Testing?
Integration testing helps in validating the interactions between different components of your application. It answers critical questions such as:
- Are two modules gracefully working together?
- Do the interfaces between components behave as expected?
- Is data properly transferred across different modules?
Example Integration Test
Here’s a simple example of an integration test in a Gradle Java project. We'll create an integration test to validate a service that interacts with a repository.
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
class UserServiceIntegrationTest {
private UserService userService;
private UserRepository userRepository;
@BeforeEach
void setup() {
userRepository = new UserRepository(); // Simulating repository
userService = new UserService(userRepository);
}
@Test
void testCreateUser() {
User newUser = new User("john.doe", "John", "Doe");
userService.createUser(newUser);
assertEquals(newUser, userRepository.findUserByUsername("john.doe"), "User should be found in repository");
}
}
In this example:
- We set up the
UserService
andUserRepository
for testing. - We validate that when a user is created, it also ends up in the repository.
Next, let's look at common pitfalls to avoid during Gradle integration testing.
Common Pitfalls in Gradle Integration Testing
1. Incomplete Test Coverage
One of the primary pitfalls in integration testing is relying solely on unit tests. While unit tests verify the functionality of individual components, integration tests validate the interaction between components.
Solution: Adopt a testing pyramid that emphasizes a balanced approach. Aim for more unit tests, a moderate number of integration tests, and fewer end-to-end tests.
2. Ignoring Environment Consistency
Integration tests may pass in the local environment but fail in CI/CD pipelines. This can happen due to differences in configuration, dependencies, or even database instances.
Solution: Always ensure that you have a consistent testing environment. Use Docker or similar tools to containerize your application, maintaining environmental consistency across local and CI environments.
tasks.withType(Test) {
useJUnitPlatform()
testLogging {
events "passed", "skipped", "failed"
}
}
This Gradle snippet configures your testing tasks, ensuring proper logging occurs during test execution, which helps monitor environment inconsistencies.
3. Not Using Mocking Wisely
Overusing mocking in integration tests can lead to scenarios where your tests do not truly reflect realistic behavior. While mocking is essential for unit tests, in integration tests, you're interested in the actual state and behavior of components.
Solution: Use real implementations whenever possible in integration tests, reserving mocking for unit tests or where dependencies cannot be used.
4. Missing Dependencies
In gradual integration testing, proper dependency management is crucial. Missing dependencies can lead to tests that break without any informative errors, making debugging frustrating.
Solution: Leverage the Gradle Dependency Management System
Ensure all necessary libraries are included correctly in your build.gradle
file:
dependencies {
testImplementation 'org.junit.jupiter:junit-jupiter-engine:5.7.0'
implementation 'org.springframework.boot:spring-boot-starter'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
This Gradle setup allows for effective management of testing and runtime libraries.
5. Neglecting Test Isolation
Integration tests that share state might lead to unpredictable results due to non-isolated interactions. For instance, one test might alter data in a way that affects others.
Solution: Utilize transaction rollbacks or reset the environment between tests to maintain isolation.
You can also use framework features. For example, in Spring, you can use @Transactional
to roll back transactions after a test.
@SpringBootTest
@Transactional
class UserServiceIntegrationTest {
// Tests ensure transaction is rolled back after completion
}
6. Inadequate Logging and Reporting
A major pitfall for developers is neglecting to set up effective logging and reporting mechanisms. Without detailed logs, debugging failed integration tests becomes daunting.
Solution: Ensure that you properly configure logging in your build.gradle
:
test {
testLogging {
events " PASSED", " SKIPPED", "FAILED"
exceptionFormat 'full'
}
}
The above configuration helps capture more informative logs that can ease the troubleshooting process when a test fails.
7. Forgetting to Clean Up Resources
After running integration tests, failing to clean up resources, such as database connections or temporary files, can lead to performance degradation in the long run.
Solution: Implement teardown methods to ensure that resources are cleaned up post-tests.
@AfterEach
void tearDown() {
// Clear temporary data, close connections, etc.
}
8. Lack of Tooling
Using Gradle without any accompanying plugins may lead to cumbersome setups. It's important to leverage existing plugins that can optimize integration tests.
Solution: Utilize plugins like the Gradle TestKit for a comprehensive testing experience. It provides the ability to test Gradle plugins and builds.
plugins {
id 'java'
}
test {
useJUnitPlatform()
}
Wrapping Up
Mastering Gradle integration testing requires an understanding of both the fundamental practices of effective testing and the specific pitfalls that can hinder success. By recognizing and addressing these common pitfalls—such as incomplete coverage, environment inconsistency, and inadequate resource management—you can create robust integration tests that ensure your software systems function reliably.
Remember that integration testing is an ongoing process. Continually review and refine your approach to testing to keep your applications resilient to changes. With the right strategies, tooling, and methodologies in place, you'll be better equipped to handle the complexities of integration testing in your Gradle projects.
Further Reading
- Effective Java Testing by Dennis K. Birland
- Gradle User Guide
- Testing Java Microservices by Alex Soto, Jason Porter, and J. A. Johnson
Embrace these practices, and you'll not only enhance your integration testing but also elevate the overall quality of your software development!
Feel free to reach out for further questions, coding challenges, or insights into Gradle or integration testing! Happy coding!