Overcoming Common Pitfalls in Domain-Specific Language Design
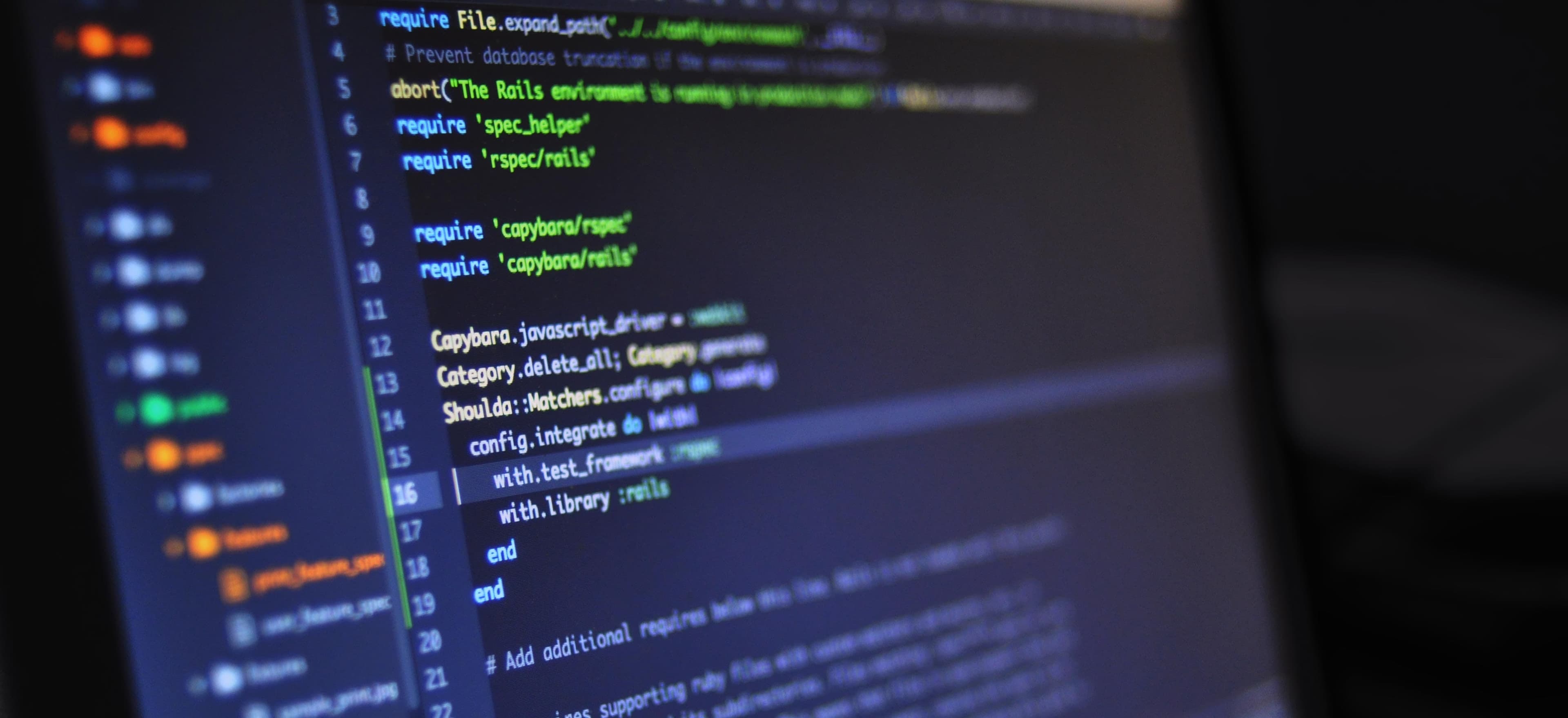
- Published on
Overcoming Common Pitfalls in Domain-Specific Language Design
Designing a Domain-Specific Language (DSL) is a challenging yet rewarding endeavor. It allows developers to create focused solutions tailored to specific problems. However, like any software design process, creating a DSL comes with its own set of pitfalls that can sabotage the effectiveness and adoption of the language. This blog post explores common pitfalls in DSL design, offering insights and strategies to overcome them.
What is a Domain-Specific Language?
A Domain-Specific Language is a programming language designed to address problems specific to a particular domain. Unlike general-purpose languages like Java or Python, DSLs are optimized for particular tasks and provide abstractions that enhance productivity and expressiveness.
Benefits of Using DSLs
-
Improved Readability: A well-designed DSL can make code easier to read and understand, which is essential for domain experts who may not be skilled programmers.
-
Enhanced Productivity: By providing specialized constructs, DSLs can reduce the amount of code necessary to implement solutions, leading to faster development cycles.
-
Better Domain Mapping: DSLs can offer constructs that map closely to domain-specific concepts, making it easier for developers to express their ideas.
Common Pitfalls in DSL Design
To ensure successful DSL implementation, it's crucial to recognize and overcome potential pitfalls.
1. Overcomplicating the Language
When designing a DSL, it's tempting to add numerous features to meet all potential requirements. However, overcomplication can lead to:
- Steep Learning Curves: Excess features can confuse users.
- Inefficiencies: More complex parsing and evaluation mechanisms may slow down execution.
Solution: Focus on the core problems the DSL aims to solve. The YAGNI principle (You Aren't Gonna Need It) applies here; avoid adding functionalities that are not immediately required.
2. Lack of User Involvement
A common mistake is the failure to involve end-users in the design process. Without user feedback, a DSL may not align with their needs.
Solution: Engage domain experts throughout the design process. Regular feedback will help align the language features with user requirements.
Code Example: Simple DSL in Java
Let’s illustrate a basic DSL design for a configuration language that specifies server settings in a clean format.
public class ServerConfig {
private String host;
private int port;
public ServerConfig host(String host) {
this.host = host;
return this;
}
public ServerConfig port(int port) {
this.port = port;
return this;
}
public void apply() {
System.out.println("Server is set to " + host + ":" + port);
}
public static void main(String[] args) {
new ServerConfig()
.host("localhost") // Specifying host
.port(8080) // Specifying port
.apply(); // Applying configuration
}
}
Why This Works: This simple DSL allows users to specify server configuration in a fluent style. The builder pattern enhances readability and encourages a natural language flow. Each method call on ServerConfig
modifies the state of the object, leading to cleaner code.
3. Ignoring Error Handling
Poor error handling can frustrate users and lead to challenging debugging scenarios.
Solution: Implement robust error-checking mechanisms. For DSLs, compile-time or runtime checks should inform users about incorrect syntax or misuse distinctly.
public class ServerConfig {
private String host;
private int port;
public ServerConfig host(String host) {
if(host == null || host.trim().isEmpty()) {
throw new IllegalArgumentException("Host cannot be null or empty");
}
this.host = host;
return this;
}
public ServerConfig port(int port) {
if(port < 1024 || port > 65535) {
throw new IllegalArgumentException("Port must be between 1024 and 65535");
}
this.port = port;
return this;
}
//... Rest of the class as before
}
Why This Matters: This implementation promotes user confidence by informing them immediately about mistakes in their configurations—whether checking for null values or ensuring the port number is within an acceptable range.
4. Not Considering Performance
Performance issues often surface unexpectedly with DSLs. If the underlying engine for evaluating the DSL is inefficient, it can dramatically affect usability.
Solution: Profile your DSL's execution. Identify bottlenecks and optimize them. Consider caching strategies or alternative parsing mechanisms to enhance performance.
5. Inadequate Documentation
Documentation often takes a backseat during DSL development. However, without comprehensive documentation, users may struggle to understand how to use the language effectively.
Solution: Provide clear and concise documentation that includes:
- Getting Started Guides: Birch the initial steps for new users.
- Code Examples: Use cases illustrating effective use of the DSL.
- FAQs: Address common issues and questions.
For further reading on DSL documentation practices, refer to A Guide to DSL Documentation.
6. Failing to Maintain the DSL
After the initial release, a DSL may require ongoing maintenance and updates based on user needs. Without proper maintenance, the DSL can quickly become outdated.
Solution: Establish a feedback loop with users and be prepared to make enhancements and corrective measures as needed.
In Conclusion, Here is What Matters
Designing an effective Domain-Specific Language is a balancing act between simplicity, usability, and robustness. By avoiding common pitfalls such as overcomplication, lack of user involvement, and inadequate error handling, you can create a DSL that effectively serves its intended purpose. Engage with your users, document thoroughly, and maintain your DSL actively to ensure its longevity and effectiveness.
For a deeper dive into DSL patterns and best practices, consider exploring DSL Design Patterns.
By keeping these strategies in mind, you can navigate the complex landscape of DSL design more successfully and ultimately deliver a valuable tool to your users.
Checkout our other articles