Mastering XPath Matchers in Hoverfly for Java Testing
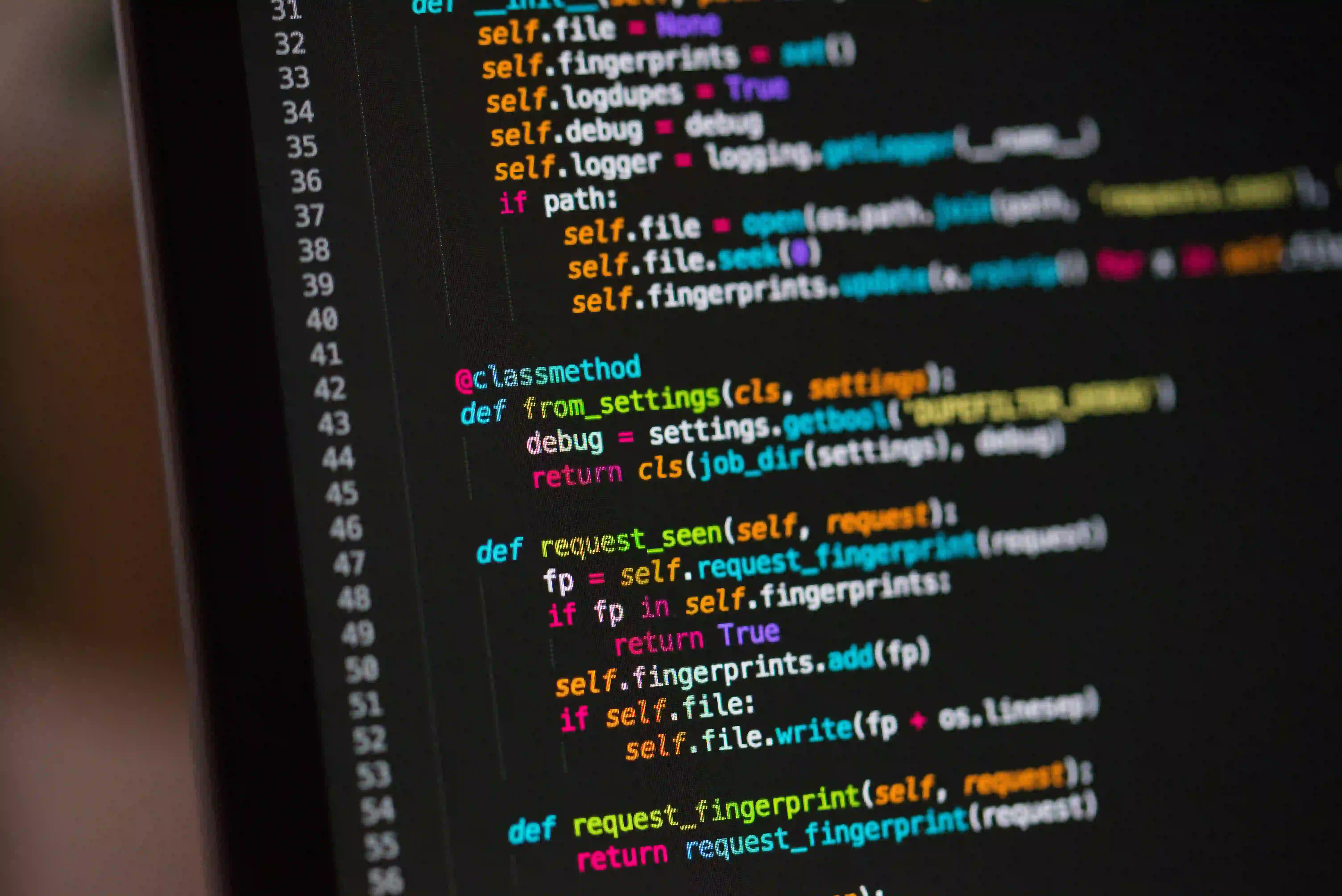
Mastering XPath Matchers in Hoverfly for Java Testing
Let us Begin
In the realm of Java development, ensuring robust and efficient testing is paramount. One of the tools that stand out in this domain is Hoverfly. Particularly, its use of XPath matchers can enhance your API testing process significantly. XPath, a powerful querying language for XML, allows you to navigate through elements and attributes in an XML document effectively.
In this blog post, we will dive deep into how to leverage XPath matchers in Hoverfly. We will explore their importance in testing and provide useful code snippets along the way to demystify their application. Let’s get started!
Understanding Hoverfly
For the uninitiated, Hoverfly is a lightweight, open-source API simulation tool that captures and simulates HTTP(S) traffic. It enables developers to test their applications without relying on external services, making it a game-changer during development.
Using Hoverfly simplifies the testing process by:
- Capturing HTTP requests: It can observe and record live traffic between a client and server.
- Simulating APIs: Hoverfly can replay captured traffic to ensure consistent testing results.
- Flexibility: It allows testing with various matchers, including URL, JSON, and, importantly for this post, XPath.
Why XPath Matchers?
When dealing with XML data, XPath matchers become crucial due to their ability to precisely query XML content. This allows testers to validate specific data structures or values within XML responses—something that's often tedious with traditional string matchers.
Getting Started with XPath Matchers
To start using XPath matchers in Hoverfly, ensure you have your development environment set up:
- Hoverfly: Make sure you have Hoverfly installed. You can find installation instructions here.
- Java and Maven: Ensure your Java environment is ready, and you are familiar with Maven for dependency management.
Adding Hoverfly Dependencies
In your pom.xml
file, include the following dependencies for Hoverfly:
<dependencies>
<dependency>
<groupId>io.spectre</groupId>
<artifactId>hoverfly-java</artifactId>
<version>0.14.0</version>
<scope>test</scope>
</dependency>
</dependencies>
Crafting Your First Hoverfly Test with XPath
Let’s look at a simple example where we will use XPath matchers. Suppose we're testing an API that returns user data in XML format.
Sample XML Response:
<user>
<id>123</id>
<name>John Doe</name>
<email>john.doe@example.com</email>
</user>
The goal here is to assert that the name returned matches "John Doe".
Setting Up Hoverfly
First, we need to set up Hoverfly in our test class:
import io.spectre.hoverfly.junit.HoverflyRule;
import io.spectre.hoverfly.junit.HoverflyRuleConfig;
import org.junit.Rule;
import org.junit.Test;
public class UserApiTest {
@Rule
public HoverflyRule hoverflyRule = HoverflyRule.inCaptureOrSimulationMode(
HoverflyRuleConfig.diffConfig()
);
// Test methods will go here
}
Adding an XPath Matcher
Now, let's add an HTTP response simulation using XPath:
@Test
public void testUserNameByXPath() {
hoverflyRule.simulate(
ServiceConfig.service(
"http://api.example.com"
).body(
"<user><id>123</id><name>John Doe</name><email>john.doe@example.com</email></user>"
)
.matcher(
Matchers.xpath("//user/name[text()='John Doe']")
)
);
// Perform your API call here, e.g. using RestTemplate
// Assert the results
}
Explanation of the Code
-
HoverflyRule: We begin by establishing our
HoverflyRule
that captures or simulates requests and responses. -
ServiceConfig: This configures the service we are simulating. Ensure the URL matches the endpoint being tested.
-
Matchers.xpath: This method leverages the XPath expression. Here, we validate the
<name>
element directly to check if its content matches "John Doe".
Making API Calls
Typically, your testing will involve making the actual API calls after we set up the simulation:
public void performApiCall() {
RestTemplate restTemplate = new RestTemplate();
String response = restTemplate.getForObject("http://api.example.com/users/123", String.class);
// Here, you'd typically use an XML parser to convert the response to an object and check the name property.
}
XML Response Validation
You can validate the response using any XML parsing library like JAXB or DOM Parser. A sample code snippet with DOM might look like this:
// Convert XML response to Document
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(new InputSource(new StringReader(response)));
// Validate the user name
String userName = doc.getElementsByTagName("name").item(0).getTextContent();
assertEquals("John Doe", userName);
Advanced XPath Matchers
XPath offers extensive capabilities beyond simple matches. For instance, you can match based on attributes, conditions, or even nested elements.
Example: Complex XPath Query
Consider a scenario with user elements potentially containing additional data:
<users>
<user>
<id>123</id>
<name>John Doe</name>
<status>active</status>
</user>
<user>
<id>124</id>
<name>Jane Doe</name>
<status>inactive</status>
</user>
</users>
You might want to assert that the user is only returned if their status is "active":
hoverflyRule.simulate(
ServiceConfig.service("http://api.example.com/users")
.body("<users><user><id>123</id><name>John Doe</name><status>active</status></user></users>")
.matcher(Matchers.xpath("//user[name='John Doe' and status='active']"))
);
Here, the XPath expression matches the user only if they are both "John Doe" and "active".
My Closing Thoughts on the Matter
XPath matchers in Hoverfly provide a powerful mechanism for validating XML responses in your Java applications. By incorporating XPath expressions into your simulations, you streamline the testing process and increase the robustness of your software.
With the ability to validate not just values but complex conditions directly in XML responses, XPath matchers can significantly improve the efficiency and effectiveness of your API testing strategy.
For further reading on advanced XPath techniques, you may check out the W3Schools XPath Tutorial.
Harnessing tools like Hoverfly with XPath can lead to more reliable and maintainable code. Start implementing it in your testing suite and see the difference it makes!
Thank you for reading! For more articles on Java testing and automation techniques, refer to our Java Testing Insight Series. Happy coding!