Common Pitfalls in Java XML String Parsing
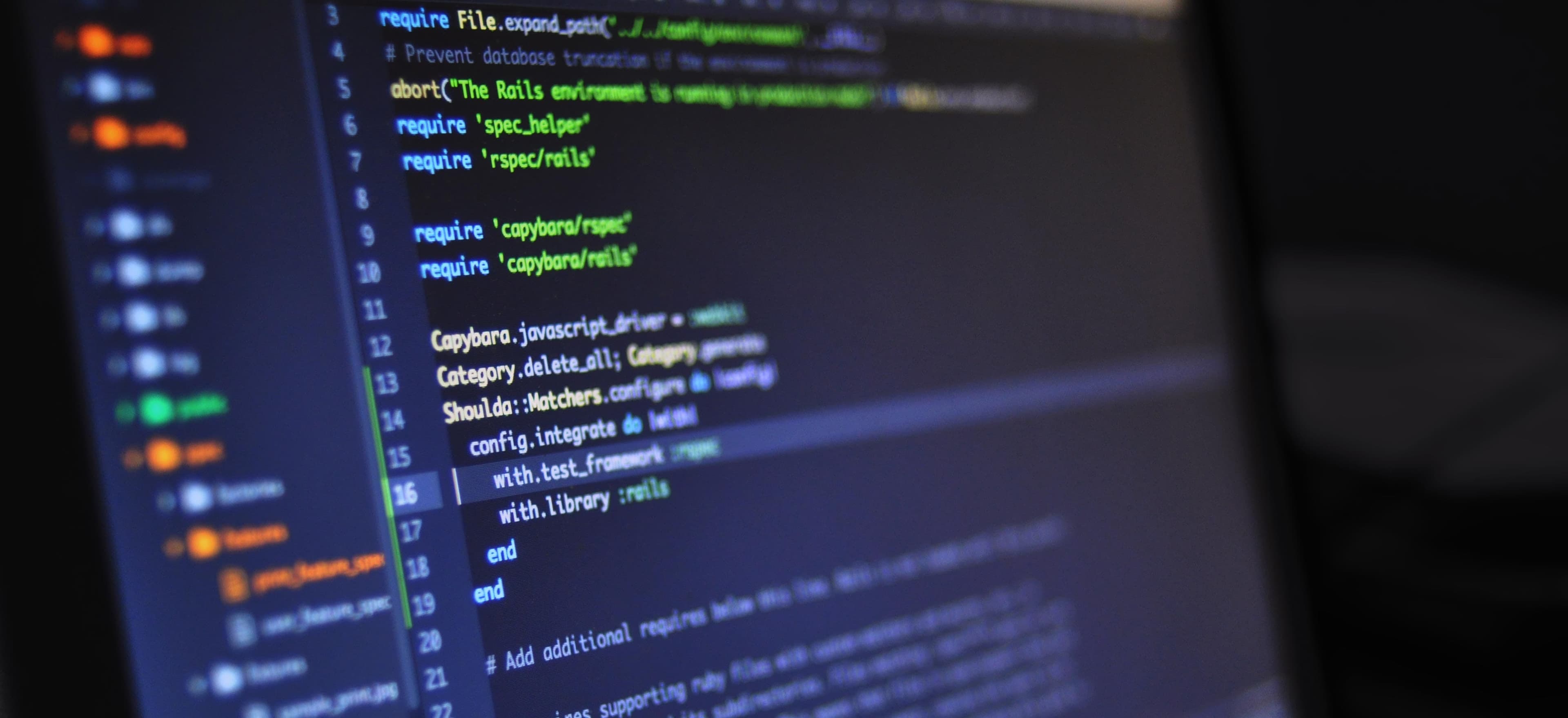
- Published on
Common Pitfalls in Java XML String Parsing
Parsing XML in Java can be straightforward if you know what to watch out for. However, common pitfalls may lead developers into frustrating debugging sessions and unexpected behavior. In this blog post, we will discuss these pitfalls, offer solutions, and provide code snippets to illustrate each point.
Overview of XML Parsing in Java
Java provides several libraries for parsing XML, including the Document Object Model (DOM) and Simple API for XML (SAX). Additionally, Java has libraries like JAXB (Java Architecture for XML Binding) that simplify working with XML by mapping it to a Java object model.
Here's an example of DOM parsing:
import org.w3c.dom.Document;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
public class XMLParserExample {
public static void main(String[] args) {
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse("example.xml");
// Further processing goes here
} catch (Exception e) {
e.printStackTrace();
}
}
}
In the code above, we first set up a DocumentBuilderFactory
, create a DocumentBuilder
, and then parse an XML file. Simple, right? Yet, the simplicity can mask deeper issues.
Common Pitfalls in XML Parsing
1. Not Handling Exceptions Properly
While we catch generic exceptions in our example, XML parsing can throw several specific exceptions. For example, SAXParseException
arises from errors in the XML format itself.
try {
// your XML parsing logic
} catch (SAXParseException e) {
System.out.println("Error on line: " + e.getLineNumber());
System.out.println("Error message: " + e.getMessage());
} catch (Exception e) {
e.printStackTrace();
}
Why handle specific exceptions? This helps you identify errors more efficiently and understand where the issues lie in the XML structure.
2. Ignoring XML Validation
Failing to validate your XML can lead to unexpected runtime errors. Using an XML Schema Definition (XSD) file can significantly enhance the robustness of your XML processing.
SchemaFactory factory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = factory.newSchema(new File("schema.xsd"));
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
unmarshaller.setSchema(schema);
This code snippet shows how to set up XML validation against an XSD.
Why validate? It ensures that the XML adheres to a predefined structure, thus reducing the number of runtime errors.
3. Not Considering Namespace Handling
XML namespaces can be confusing, and neglecting them can cause your queries to fail. Always be aware of the namespaces when parsing XML.
NodeList nodes = doc.getElementsByTagNameNS("http://example.com/schema", "item");
Why use namespaces? They prevent element name conflicts and help maintain data integrity in large XML documents.
4. Overloading Memory with Huge XML Files
When parsing large XML files, you may run into memory issues. For this reason, consider using a streaming parser like SAX or StAX (Streaming API for XML).
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
DefaultHandler handler = new DefaultHandler() {
public void startElement(String uri, String localName, String qName, Attributes attributes) {
// Process start of an element
}
public void endElement(String uri, String localName, String qName) {
// Process end of an element
}
public void characters(char[] ch, int start, int length) {
// Process character data
}
};
saxParser.parse(new File("large.xml"), handler);
Why stream? Streaming parsers load only a part of the XML document into memory at a time, thus saving memory and allowing you to process large XML files seamlessly.
5. Misunderstanding Character Encoding
Different XML documents may be in various character encodings. Failing to recognize and handle encoded characters can lead to corrupted data.
InputStreamReader reader = new InputStreamReader(new FileInputStream("example.xml"), "UTF-8");
Why pay attention to character encoding? It ensures that you read and process characters as intended, preventing data loss or misinterpretation.
6. Using XPath Inefficiently
XPath can be powerful for extracting data from XML. However, improperly constructed XPath queries may degrade performance.
XPathFactory xpathFactory = XPathFactory.newInstance();
XPath xpath = xpathFactory.newXPath();
String expression = "//item[condition]";
NodeList result = (NodeList) xpath.evaluate(expression, doc, XPathConstants.NODESET);
Why optimize XPath? Performance can significantly drop with complex or poorly structured queries. Always aim for simplicity and clarity.
7. Forgetting Whitespace Handling
Whitespace issues, especially within text nodes, can lead to unexpected behavior. Always ensure you trim string data after parsing.
String trimmedData = node.getTextContent().trim();
Why manage whitespace? It helps avoid errors in comparisons and data processing later.
In Conclusion, Here is What Matters
In summary, parsing XML strings in Java can be robust and easy if you sidestep common pitfalls. Always handle exceptions specific to your context, validate XML structures, respect namespaces, use streaming for large files, manage character encoding, optimize XPath queries, and handle whitespace properly.
By recognizing these issues and implementing the recommendations discussed in this post, you can avoid a lot of headaches and create more efficient, reliable applications. For you to deepen your understanding, consider checking Oracle's Official XML Documentation and practice parsing XML with simple examples.
Further Reading
- Understanding XML Parsing
- JAXB Tutorial
- XPath Functions
By implementing the strategies discussed, you'll improve your XML parsing skills, making your Java applications more robust and easier to maintain. Happy coding!
Checkout our other articles