Streamlining Data-Driven Testing with JUnit and EasyTest
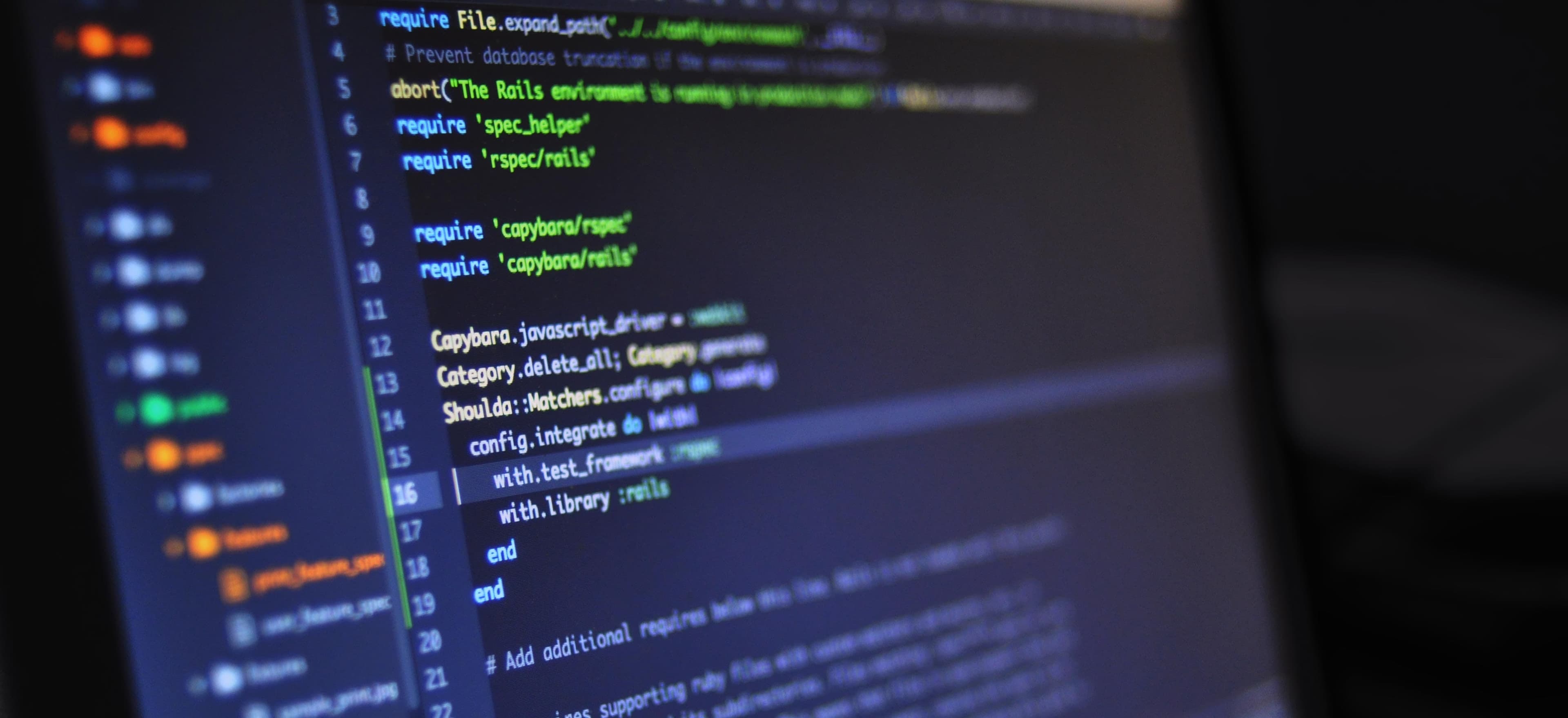
- Published on
Streamlining Data-Driven Testing with JUnit and EasyTest
In software development, ensuring code quality and correctness is paramount. Among various testing approaches, data-driven testing has gained considerable attention as it allows developers to run the same tests against multiple sets of data. In this blog post, we'll explore how to streamline data-driven testing using two powerful tools: JUnit and EasyTest.
What is Data-Driven Testing?
Before diving into implementation, let's define data-driven testing. This methodology involves running the same test with different inputs, which helps verify that your code behaves correctly across a range of scenarios. This approach not only improves test coverage, but it also minimizes code duplication and makes tests easier to maintain.
The Role of JUnit
JUnit is a widely-used testing framework in the Java ecosystem. It provides annotations, assertions, and runners that simplify the process of writing and running tests. JUnit has built-in support for parameterized tests, which is the basis of data-driven testing in Java.
Introducing EasyTest
EasyTest is a minimalist testing framework that complements JUnit by providing an easy way to run parameterized tests using external data sources like CSV files or Excel sheets. This can significantly ease the setup and execution of data-driven tests by abstracting some of the complexities involved in handling data input.
Setting Up Your Environment
To get started, you need the following prerequisites installed on your machine:
- Java Development Kit (JDK): Ensure you have a JDK installed. You can download it from Oracle's official site.
- JUnit: Java's popular testing framework. You can add it to your Maven project with the following dependency:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
- EasyTest: You can include EasyTest in your project with the following Maven dependency:
<dependency>
<groupId>com.github.davidm</groupId>
<artifactId>easytest</artifactId>
<version>4.0.1</version>
</dependency>
Once you have these dependencies set, you're all set to dive into data-driven testing.
Writing Data-Driven Tests with JUnit
Using Parameterized Tests
JUnit provides an annotation named @RunWith(Parameterized.class)
that allows you to create parameterized tests easily. Here is a simple example:
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Arrays;
import java.util.Collection;
import static org.junit.Assert.assertEquals;
@RunWith(Parameterized.class)
public class CalculatorTest {
private int input1;
private int input2;
private int expectedResult;
private Calculator calculator;
public CalculatorTest(int input1, int input2, int expectedResult) {
this.input1 = input1;
this.input2 = input2;
this.expectedResult = expectedResult;
}
@Before
public void setUp() {
calculator = new Calculator();
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{1, 1, 2},
{2, 2, 4},
{3, 3, 6},
{4, 5, 9}
});
}
@Test
public void testAdd() {
assertEquals(expectedResult, calculator.add(input1, input2));
}
}
Explanation of the Code
- Parameterized: The
@RunWith(Parameterized.class)
annotation tells JUnit to run the tests using a parameterized runner. - Constructor: We define a constructor that takes the input values and expected result.
- Data Method: The static
data
method provides input parameters for the test cases. - Assertions: Inside the test, we use assertions to check if the addition is performed correctly.
This structure makes it easy to add more test cases simply by including more data in the data()
method, enhancing both clarity and maintainability.
Incorporating EasyTest for Complex Scenarios
While JUnit’s native ability to handle parameterized tests is beneficial, EasyTest takes it a step further by enabling the use of external files. Let’s see how that looks with EasyTest:
Example using EasyTest
Assume you have a CSV file named data.csv
that contains the following data:
input1,input2,expectedResult
1,1,2
2,2,4
3,3,6
4,5,9
Now, you can easily create a data-driven test using EasyTest:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.easytest.DataProvider;
import org.easytest.EasyTest;
import org.easytest.TestConfig;
@RunWith(EasyTest.class)
public class EasyTestExample {
private Calculator calculator = new Calculator();
@Test
@DataProvider(file = "data.csv")
public void testAdd(int input1, int input2, int expectedResult) {
assertEquals(expectedResult, calculator.add(input1, input2));
}
}
Explanation of EasyTest Code
- @DataProvider: The annotation allows the test method to accept parameters from the external CSV file.
- File Path: Ensure the file is in the correct location relative to your project so that EasyTest can access it.
This implementation makes it exceptionally easy to manage large sets of test data without cluttering your code. By utilizing external data sources, you bring your test cases into a more manageable and scalable format.
Benefits of Data-Driven Testing
Taking a step back, there are significant advantages to incorporating data-driven testing with JUnit and EasyTest:
- Improved Coverage: You can easily test edge cases and various scenarios without writing repetitive code.
- Easier Maintenance: Changes to test data can be made externally, saving time and effort, as you do not need to touch the test code itself.
- Clarity and Readability: Both JUnit and EasyTest offer clear structures that enhance readability and make test logic apparent.
- Scalability: As your application grows, the modular approach allows you to easily add more test cases.
Final Thoughts
Data-driven testing is an invaluable technique that enhances your application's robustness and reliability. By leveraging JUnit’s parameterized capabilities alongside the flexibility of EasyTest, you can create scalable, easy-to-maintain tests that cover various scenarios. For more in-depth exploration of JUnit, consider checking out JUnit 5 User Guide or visit the EasyTest GitHub page for further resources.
Start implementing data-driven tests in your projects today, and see how it simplifies your testing strategy!
Checkout our other articles