Maximize Your Number: Mastering K-Digit Removals
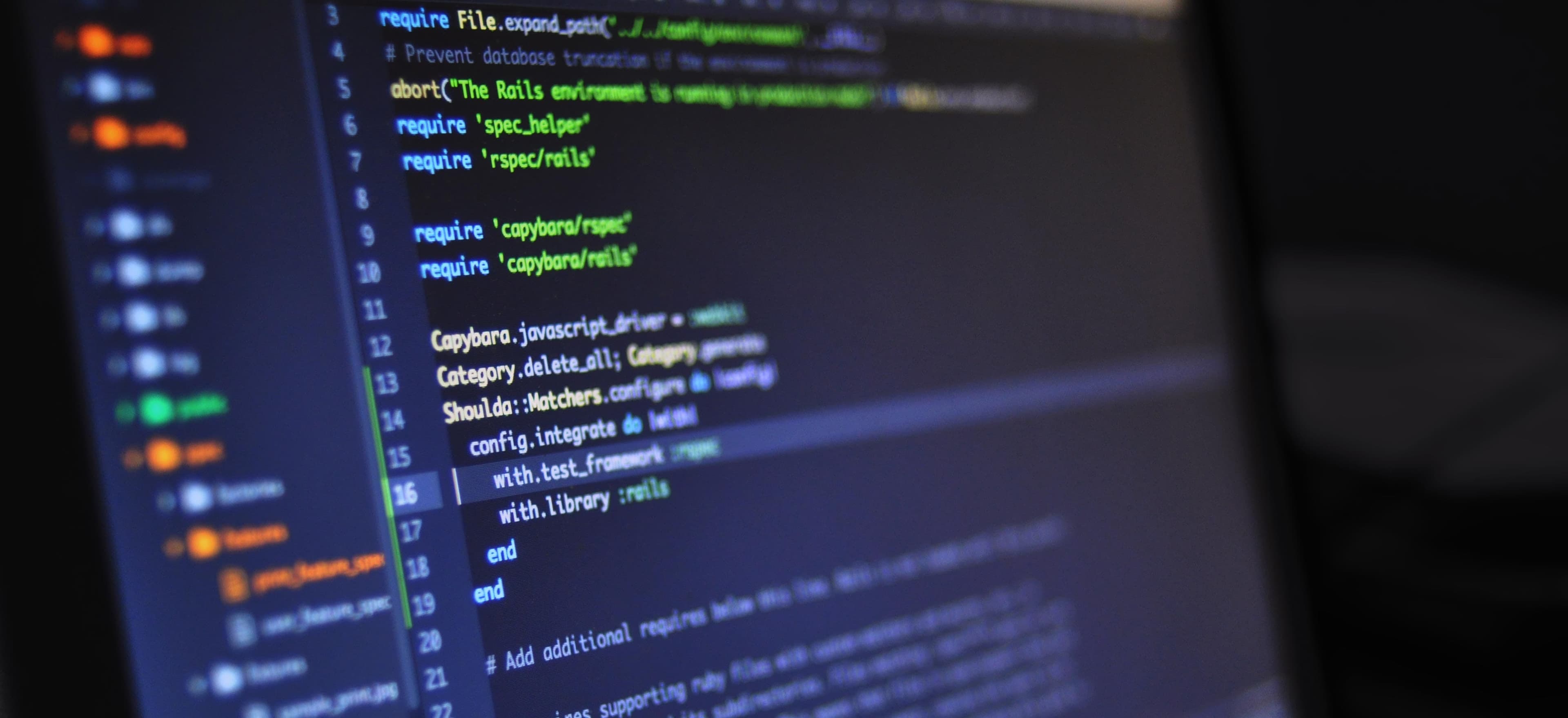
- Published on
Maximize Your Number: Mastering K-Digit Removals
In the world of coding interviews and competitive programming, you often face problems that require a combination of logic, strategy, and efficiency. One such problem revolves around maximizing a number by removing k digits from a larger number. This intriguing challenge not only tests your understanding of data structures but also your ability to think critically about digit manipulation.
In this blog post, we will explore the problem comprehensively, break down the solution using Java, and provide you with the tools to tackle such problems confidently. Let’s dive into our topic!
Understanding the Problem
We are tasked with taking a non-negative integer represented as a string and removing k digits from it to form the largest possible integer. The number should not contain any leading zeros unless it is '0'. This problem has numerous applications in algorithm design and optimization.
Example Walkthrough
Let's consider an example to clarify:
Input:
num = "1432219"
k = 3
Output:
"943"
Explanation:
By removing the digits '1', '4', and '2', we can arrange the remaining digits to form the highest number possible, which is '943'.
Core Idea Behind the Solution
To effectively remove the digits and ensure we obtain the highest possible number, we will leverage a data structure called a stack. The key to this approach is:
- Traverse each digit in the number.
- Use a stack to maintain the digits of the resultant number.
- Compare the current digit with the top of the stack. If the current digit is greater, we can remove the smaller digit from the stack (if we haven't yet removed k digits).
- Continue this process until we've examined all digits.
- If we still haven't removed all k digits after traversing, we can simply truncate the stack.
Complexity Analysis
The time complexity of this approach is O(n) because we are iterating through the number only once, where n is the number of digits. The space complexity is also O(n) for the stack.
Now, let's put this into action with some code!
Java Implementation
Here is a simple yet effective Java implementation for the K-Digit Removals problem:
import java.util.Stack;
public class MaxNumber {
public String removeKdigits(String num, int k) {
// Use a stack to keep our digits
Stack<Character> stack = new Stack<>();
// Iterate through each digit in the string
for (char digit : num.toCharArray()) {
// While we can remove digits and the last digit in stack is less than the current digit
while (k > 0 && !stack.isEmpty() && stack.peek() < digit) {
stack.pop(); // remove the smallest digit
k--;
}
stack.push(digit); // add the current digit
}
// If we have more digits to remove, remove from the end
while (k-- > 0 && !stack.isEmpty()) {
stack.pop();
}
// Build the result into a String
StringBuilder result = new StringBuilder();
while (!stack.isEmpty()) {
result.append(stack.pop());
}
// Since we popped from the stack, reverse the order
result.reverse();
// Remove leading zeros
while (result.length() > 1 && result.charAt(0) == '0') {
result.deleteCharAt(0);
}
return result.length() == 0 ? "0" : result.toString();
}
public static void main(String[] args) {
MaxNumber maxNumber = new MaxNumber();
String result = maxNumber.removeKdigits("1432219", 3);
System.out.println(result); // Output: "943"
}
}
Code Explanation
-
Stack Initialization: We begin by initializing a stack to hold our digits.
-
Digit Traversal: For each digit, we check if it forms a larger number by comparing it to the stack's top.
while (k > 0 && !stack.isEmpty() && stack.peek() < digit) { stack.pop(); k--; }
Here, we pop the stack whenever the current digit is more significant than the top, allowing us to maximize the number gradually.
-
Handling Remaining Digits: After processing all digits from the original number, if we still need to remove any digits, we truncate the tail of our stack.
-
Constructing the Result: We build the final number using a
StringBuilder
from the stack, ensuring to reverse it afterward since stacks are LIFO (Last In First Out). -
Leading Zeros Management: We remove potential leading zeros by checking the first character in the result.
The Closing Argument
The K-Digit Removals problem is a fascinating exercise in algorithmic thinking, requiring a clear understanding of stacks and greedy techniques. Through the described approach, we are able to formulate an efficient solution, as demonstrated in our Java code example.
If you found value in this post, consider further exploring Greedy Algorithms or Data Structures in Java to expand your programming repertoire!
Keep coding hard, and remember – every problem has a solution just waiting to be uncovered!