Solving Data Consistency Issues in Microservice Communication
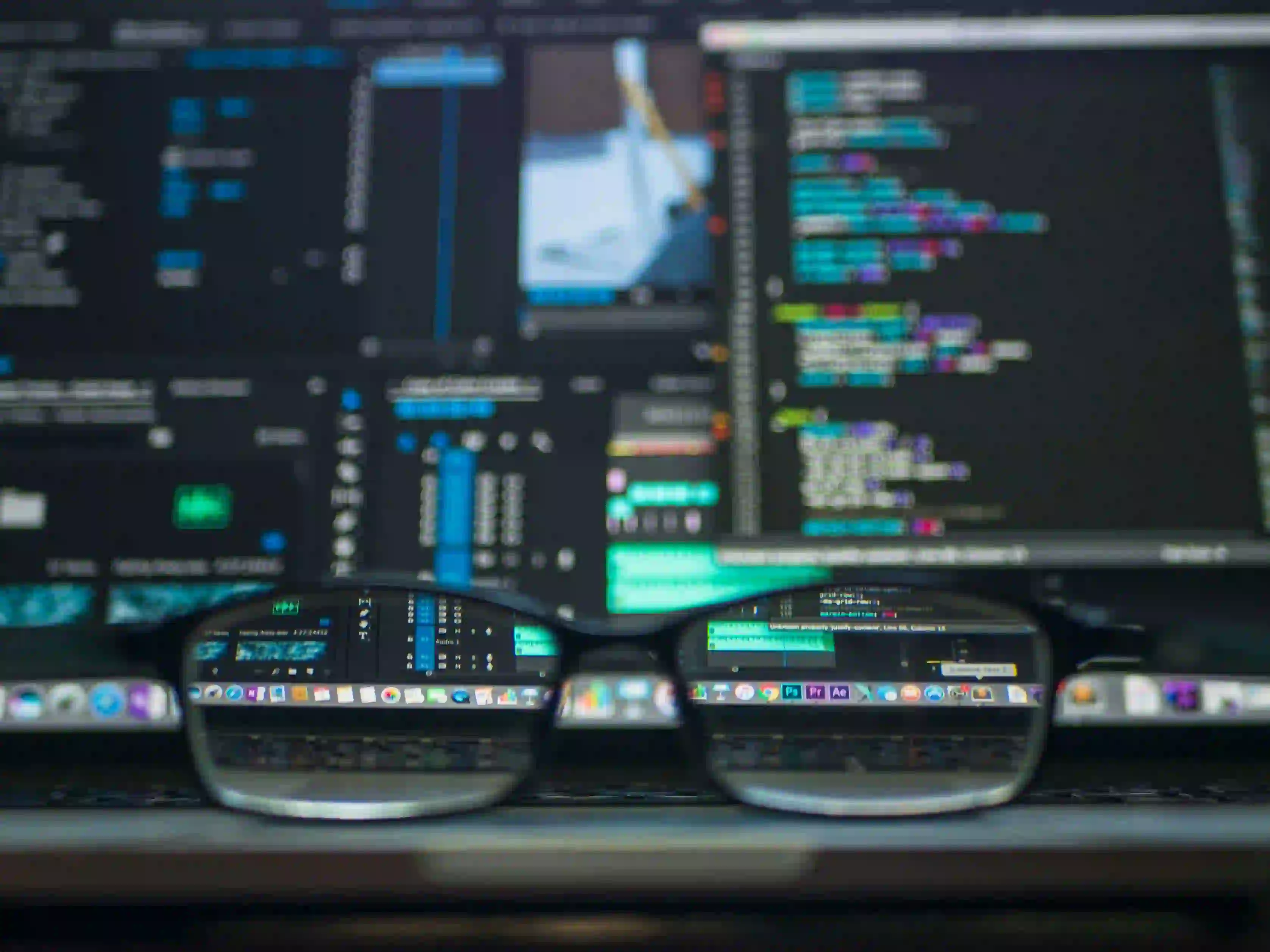
Solving Data Consistency Issues in Microservice Communication
In today's software architecture landscape, microservices are becoming increasingly popular for their scalability, flexibility, and ability to handle complex applications in a modular way. However, with great power comes great responsibility, particularly when it comes to ensuring data consistency across these services. In this blog post, we will explore common data consistency issues faced in microservice communication and how to address them effectively.
Understanding Data Consistency in Microservices
Data consistency refers to the accuracy and reliability of data across different components in a system. In a microservices architecture, different services own different data models, and these services often communicate over a network. This can lead to several data consistency issues, particularly when dealing with complex data transactions that span multiple services.
There are generally three types of consistency:
- Strong Consistency: This guarantees that a read will always return the most recently written value.
- Eventual Consistency: This ensures that, given enough time, all updates will propagate through the system. However, the system may temporarily return stale data.
- Causal Consistency: This ensures that operations that are causally related are seen by all nodes in the same order.
Microservices often operate under eventual consistency because it allows for better performance and availability. However, understanding the trade-offs is essential to ensure system reliability.
Common Data Consistency Issues
1. Synchronous Communication Problems
When microservices communicate synchronously—typically using HTTP requests or gRPC—the caller service waits for the callee to respond. This can lead to cascading failures and inconsistency when the callee is down or slow.
Solution: Use asynchronous communication strategies such as message queues (e.g., RabbitMQ, Apache Kafka). By decoupling services, you can handle high loads more effectively while maintaining eventual consistency.
Example Code:
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MessageSender {
private final RabbitTemplate rabbitTemplate;
@Autowired
public MessageSender(RabbitTemplate rabbitTemplate) {
this.rabbitTemplate = rabbitTemplate;
}
public void sendMessage(String message) {
// Sending message asynchronously to the queue
rabbitTemplate.convertAndSend("myQueue", message);
}
}
In this code, RabbitTemplate
is used to send messages to a queue without blocking the main service's processing. This allows the system to handle spikes in load without waiting on the response from other services.
2. Distributed Transactions
Microservices often need to perform operations that require coordination across multiple services. Traditional database transactions (ACID) are more challenging to implement across distributed systems.
Solution: Implement the Saga pattern or use a distributed transaction manager.
Saga Pattern Example:
public class PaymentSaga {
public void initiatePayment(String orderId) {
// Step 1: Reserve Inventory
boolean inventoryReserved = reserveInventory(orderId);
if (!inventoryReserved) {
// Rollback
cancelOrder(orderId);
return;
}
// Step 2: Process Payment
boolean paymentProcessed = processPayment(orderId);
if (!paymentProcessed) {
// Rollback
releaseInventory(orderId);
return;
}
// Step 3: Confirm Order
confirmOrder(orderId);
}
}
In the above saga, we perform a series of steps and maintain state at each step. If any step fails, we run compensating actions to revert any changes, ensuring that our services remain in a consistent state.
3. Data Ownership and Duplication
Each microservice ideally owns its data model. However, some services may need to access and manipulate data owned by others, leading to data duplication, which can complicate consistency.
Solution: Utilize API Gateways or Data Mesh architecture. An API Gateway can aggregate data from several microservices and provide read-optimized views without affecting individual data stores.
Example Aggregation Code:
@RestController
@RequestMapping("/api/orders")
public class OrderController {
@Autowired
private InventoryService inventoryService;
@Autowired
private PaymentService paymentService;
@GetMapping("/{id}")
public OrderDetails getOrderDetails(@PathVariable String id) {
// Fetch data from multiple services
InventoryData inventoryData = inventoryService.getInventory(id);
PaymentData paymentData = paymentService.getPayment(id);
return new OrderDetails(inventoryData, paymentData);
}
}
In this example, the OrderController
aggregates data from both InventoryService
and PaymentService
, providing a unified view without the need for duplicating data across services.
4. Eventual Consistency Challenges
With eventual consistency, there can be delays in how quickly data propagates through the system. This can lead to scenarios where one service holds stale data while another has updated information.
Solution: Use an event-driven architecture. By broadcasting events when data changes, services can listen for updates and refresh their own data accordingly.
Event Emission Example:
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.stereotype.Service;
@Service
public class OrderService {
private final ApplicationEventPublisher eventPublisher;
@Autowired
public OrderService(ApplicationEventPublisher eventPublisher) {
this.eventPublisher = eventPublisher;
}
public void completeOrder(String orderId) {
// Complete order logic...
// Publish event to notify other services
eventPublisher.publishEvent(new OrderCompletedEvent(orderId));
}
}
In this code snippet, when an order is completed, an event is published. Other microservices can subscribe to this event and update their data based on the received information, enhancing eventual consistency across the application.
A Final Look
In microservices, data consistency is a complex but manageable challenge. By understanding the types of consistency, recognizing common issues, and implementing practical solutions such as event-driven architectures, sagas, and asynchronous communication, you can build systems that remain reliable and consistent.
For more in-depth information on microservice patterns and practices, you can refer to Martin Fowler’s Microservices and the API Gateways documentation at AWS.
By acknowledging the nuances of microservices and implementing appropriate strategies, you can create a resilient, scalable, and consistent application architecture that thrives in a cloud-native environment.