Is ActiveJ the Solution for Java's Performance Bottlenecks?
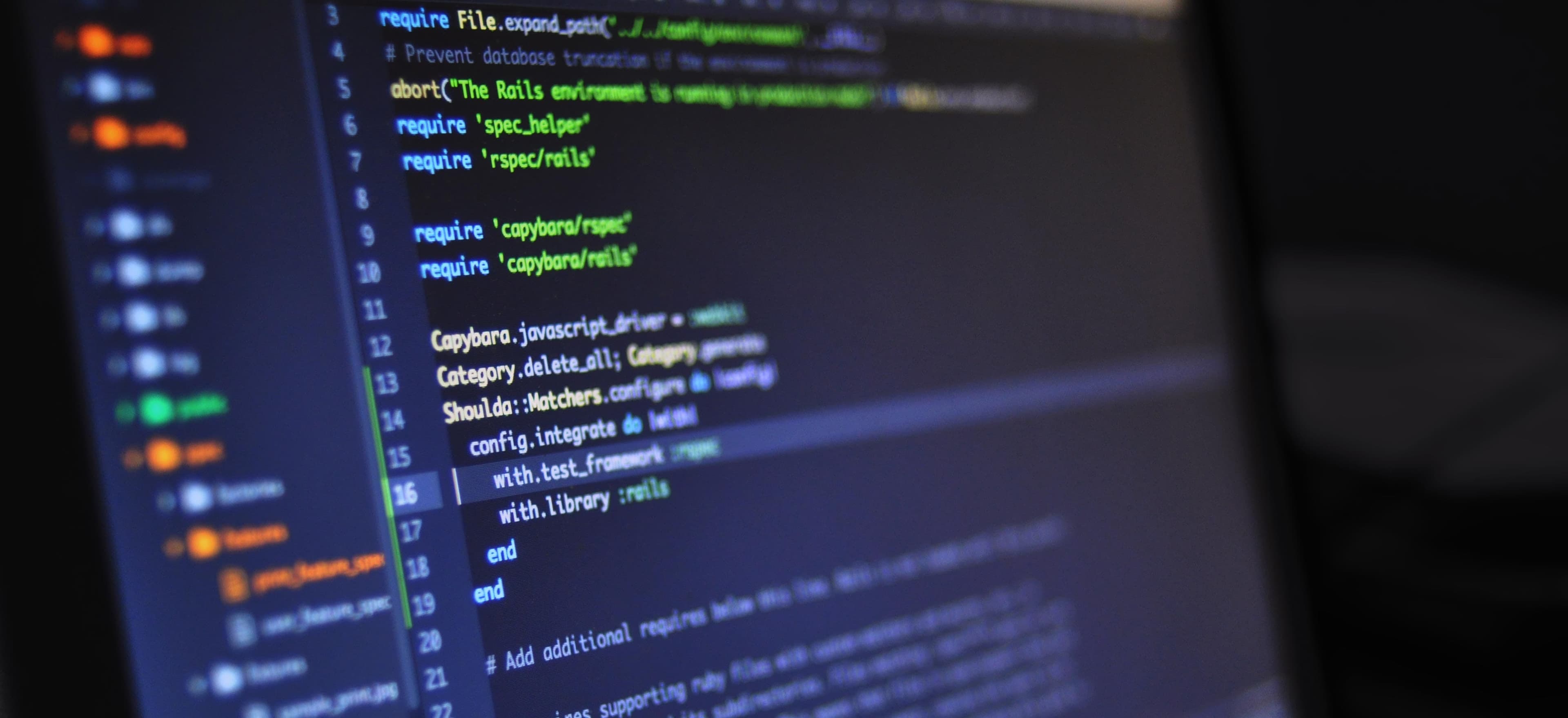
- Published on
Is ActiveJ the Solution for Java's Performance Bottlenecks?
In the realm of modern application development, few things are as critical as performance. Java, known for its portability, robustness, and vast ecosystem, often faces performance bottlenecks, particularly when scaling web applications and processing high-throughput data sets. Enter ActiveJ: an ambitious, innovative framework designed to break the barriers of traditional Java performance.
In this blog post, we'll explore what ActiveJ is, how it addresses common performance issues in Java, and provide practical examples to illustrate its power and ease of use.
What is ActiveJ?
ActiveJ is a Java framework that facilitates asynchronous, high-performance programming. It's built on the foundation of lightweight, reactive programming principles, providing a robust environment for building scalable applications without the overhead typical of traditional Java frameworks like Spring or Java EE. Key features include:
- Asynchronous I/O for non-blocking operations
- High throughput and low latency
- Modular architecture with a focus on microservices
- Built-in tools for serialization and HTTP handling
To facilitate understanding, we will break down these elements further, showcasing how they potentially alleviate performance bottlenecks.
Addressing Java's Performance Issues
1. Asynchronous I/O
Java's traditional synchronous I/O can lead to significant performance bottlenecks in applications that handle numerous concurrent requests. Each request can block a thread, leading to thread starvation and increased latency.
ActiveJ takes a different approach by providing asynchronous I/O capabilities. This non-blocking paradigm allows the application to free up threads during I/O operations, maintaining a pool of active threads that can handle more tasks efficiently.
Code Example: Asynchronous Server
Here’s a simple implementation of an asynchronous HTTP server using ActiveJ:
import static io.activej.http.HttpServer.*;
import io.activej.http.HttpServer;
import io.activej.http.HttpRequest;
import io.activej.http.HttpResponse;
import io.activej.promise.Promise;
public class AsyncHttpServer {
public static void main(String[] args) {
HttpServer server = HttpServer.create(8080);
server
.serve("/", request -> handleRequest(request))
.listen()
.thenAccept(httpServer -> System.out.println("Server started on port 8080"));
}
private static Promise<HttpResponse> handleRequest(HttpRequest request) {
// Simulate some asynchronous processing, e.g. fetching data from a database
return Promise.of(() -> {
String responseMessage = "Hello, ActiveJ!";
return HttpResponse.ok200()
.withPlainText(responseMessage);
});
}
}
Why use Async I/O?
In the above code, the server’s handleRequest
method processes requests asynchronously. This non-blocking nature means that while waiting for operations like database calls or file access, the server can continue to accept other requests, vastly improving throughput.
2. High Throughput and Low Latency
ActiveJ is designed to maximize throughput while maintaining low latency. The framework's architecture allows it to scale horizontally, where multiple instances of the application can handle more requests concurrently without significant performance degradation.
The built-in optimization techniques, such as connection pooling and buffering, help achieve better performance.
Conceptual Insight
Throughput metrics often dictate an application’s efficiency in high-demand environments. ActiveJ encompasses several optimizations out of the box, ensuring better resource management and reduced bottlenecks, which is crucial for applications like microservices that must operate seamlessly under load.
3. Modular Architecture
One of the most commendable features of ActiveJ is its modular architecture. Developers can opt for specific modules instead of loading a monolithic framework with unnecessary components, ensuring that the applications remain lightweight.
With features like:
- Serialization
- HTTP handling
- Voids and futures for non-blocking task execution
You can craft only the necessary components, allowing your applications to remain fast and agile.
Code Snippet: Using Modules
import io.activej.inject.module.Module;
import io.activej.inject.module.ModuleBuilder;
public class ActiveJModule {
public static void main(String[] args) {
Module module = ModuleBuilder.create()
.bind(HttpServer.class)
.bind(Serializer.class)
.build();
// Here we can wire up our modules as needed
}
}
Why does Modular Architecture Matter? By incorporating only the modules you need, you reduce the overall footprint and resource demand. In a cloud-native environment, these savings can translate into significant cost reductions when scaling applications.
4. Competitive Edge with Serialization
Serialization in Java has always been a topic of discussion, especially regarding performance. Standard Java serialization is often slow and can lead to significant memory overhead.
ActiveJ offers a specialized serializer that drastically improves the speed of object serialization and deserialization, utilizing techniques like zero-copy and direct memory access.
Code Example: Custom Serialization
import io.activej.serializer.Serializer;
import io.activej.serializer.SerializerRegistry;
public class User {
public String name;
public int age;
// Custom serializer for User class
public static final Serializer<User> SERIALIZER = Serializer.of(User.class)
.addField("name", User::getName, User::setName)
.addField("age", User::getAge, User::setAge);
}
Why is Advanced Serialization Important? With efficient serialization, you can cut down on time spent during data transfer between services or while persisting data, ultimately leading to snappier application responses.
Wrapping Up
In summary, ActiveJ presents a compelling alternative for Java developers seeking to overcome performance bottlenecks. Its asynchronous nature, high throughput design, modular architecture, and efficient serialization techniques mark it as an evolution in the Java programming landscape.
As web applications grow increasingly complex and demand higher performance at scale, frameworks like ActiveJ are essential to maintain responsiveness and reliability.
You can learn more about ActiveJ and its capabilities directly from the official documentation or check out its GitHub repository for source code and additional examples.
So, if you are grappling with performance issues in your Java applications, it might be time to give ActiveJ a try. Your future users will thank you for the improved experience.
Checkout our other articles