Resolving IllegalStateException: Common Blocking Issues
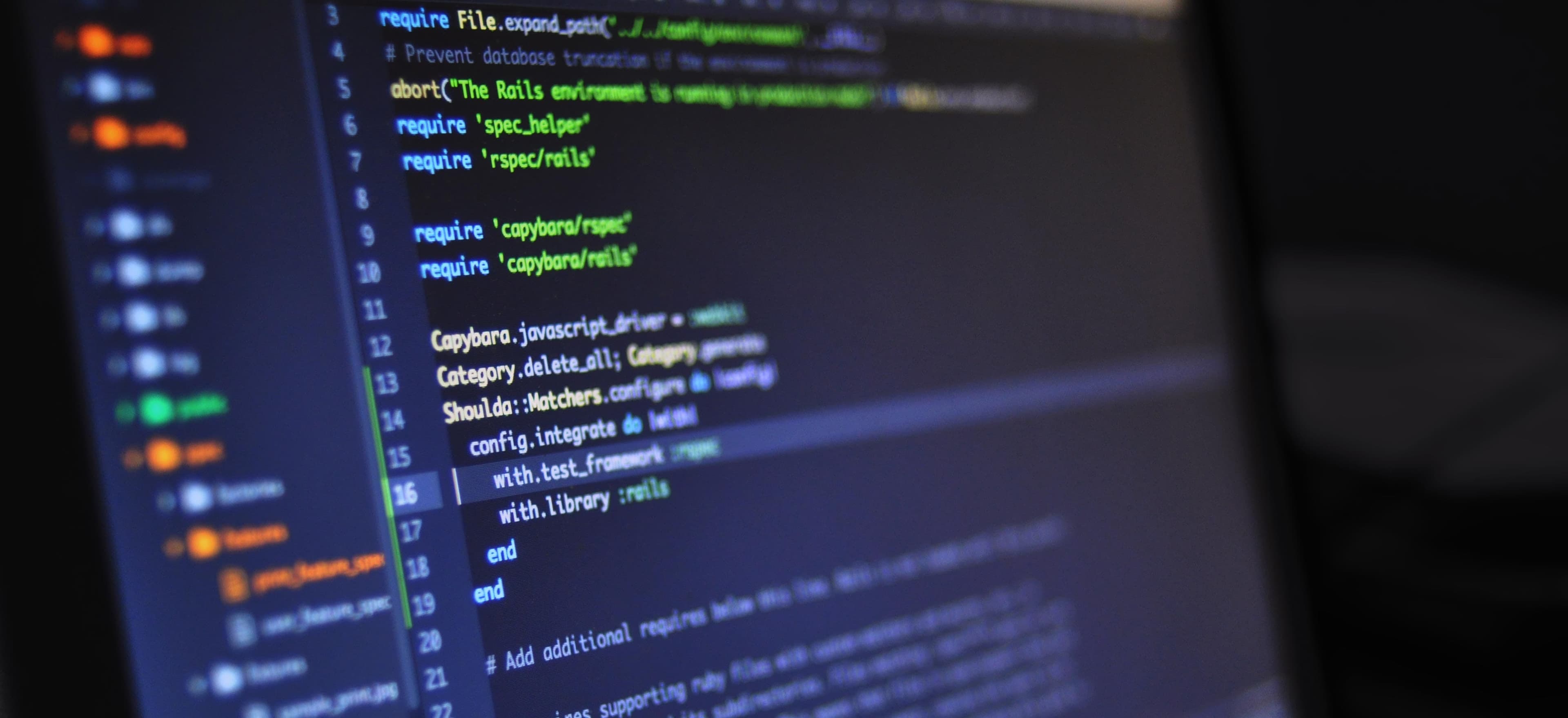
- Published on
Resolving IllegalStateException: Common Blocking Issues in Java
IllegalStateException is a common runtime exception that Java developers encounter while working with different parts of Java's API. Understanding this exception is crucial, as it can obstruct your application flow and lead to unexpected behaviors.
In this post, we will:
- Understand IllegalStateException.
- Identify common scenarios that lead to this exception.
- Provide code snippets illustrating these issues.
- Discuss best practices for prevention.
What is IllegalStateException?
IllegalStateException is a part of the Java standard library, used to signal that a method has been invoked at an inappropriate time. It extends from RuntimeException, meaning it is unchecked and does not require explicit handling.
This exception usually indicates that the method invoked is not in a state that allows it to be executed. For example, trying to modify a collection that has already been locked or attempting an operation on a connection that has been closed.
Here’s the common structure of an IllegalStateException:
throw new IllegalStateException("Your message here");
When debugging such exceptions, keep an eye on the error messages, as they can guide you to the location or state that caused the problem.
Common Scenarios Leading to IllegalStateException
1. Misusing Collections
A common cause of IllegalStateException occurs when you interact with collections improperly. For instance, consider the following example with a Queue
.
import java.util.LinkedList;
import java.util.Queue;
public class QueueExample {
public static void main(String[] args) {
Queue<String> queue = new LinkedList<>();
// Removing an element from an empty queue
queue.remove(); // This will throw IllegalStateException
}
}
Why does this happen?
In the above example, remove()
is called on an empty queue. According to the Queue interface's contract, remove()
is not designed to handle empty states gracefully, leading to an IllegalStateException
.
How to Resolve?
To handle this appropriately, always check the state of the collection before performing operations:
if (!queue.isEmpty()) {
queue.remove();
} else {
System.out.println("Queue is empty!");
}
2. Threading Issues with Executors
When using Java's Executor framework, calling methods on a terminated executor leads to IllegalStateException. Here's a simple illustration:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(1);
executor.shutdown();
// Trying to submit a task after shutdown
executor.submit(() -> System.out.println("Task executed"));
}
}
Why does this happen?
Calling submit()
after shutdown()
on the executor is illegal because it is already in a terminated state.
How to Resolve?
Always check the state of your executor before submitting tasks:
if (!executor.isShutdown()) {
executor.submit(() -> System.out.println("Task executed"));
} else {
System.out.println("Cannot submit task, executor is shut down.");
}
3. Resource Management with Streams
Working with Java Streams can also lead to IllegalStateExceptions if the stream is already closed:
import java.util.stream.Stream;
public class StreamExample {
public static void main(String[] args) {
Stream<String> stream = Stream.of("A", "B", "C");
stream.forEach(System.out::println);
// Trying to reuse the closed stream
stream.forEach(System.out::println); // IllegalStateException
}
}
Why does this happen?
When you consume a stream, it becomes closed. Attempting to perform further operations on it results in IllegalStateException.
How to Resolve?
Always create a fresh stream for new operations, like so:
Stream<String> newStream = Stream.of("A", "B", "C");
newStream.forEach(System.out::println);
4. JDBC Connection Handling
IllegalStateException can also arise in JDBC connections. Closing a connection and trying to perform another operation can lead to this error, as shown below:
import java.sql.Connection;
import java.sql.DriverManager;
public class JdbcExample {
public static void main(String[] args) {
try {
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
connection.close();
// Performing operations on a closed connection
connection.createStatement(); // IllegalStateException
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why does this happen?
The connection is closed before the operation, rendering it invalid.
How to Resolve?
Use a try-with-resources statement to ensure that connections are properly managed.
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password")) {
connection.createStatement();
} catch (Exception e) {
e.printStackTrace();
}
Best Practices for Avoiding IllegalStateException
-
Check Object State: Before performing operations, always validate that the object is in the right state for the action.
-
Use Proper Synchronization: In multithreaded scenarios, ensure thread safety using synchronization mechanisms to prevent method invocations at the wrong time.
-
Avoid Reusing Closed Resources: Stream and database connections should be treated as disposable resources. Halting operations on them after closure is advisable.
-
Implement Defensive Programming: Consider employing defense mechanisms to catch and appropriately handle possible states rather than risking exceptions.
-
Consult Documentation: Familiarize yourself with the documentation of the libraries and frameworks you're using to understand the state contracts.
The Bottom Line
IllegalStateException can be a roadblock in your Java development journey. By understanding the common scenarios and strategies to mitigate these issues, you can write more robust applications. Always remember: validation, proper resource management, and understanding object states are key to developing a resilient Java application.
Feel free to dive deeper into topics like Java Collections Framework or Java Concurrency for enhanced understanding and better practices!
If you have any questions or need further clarification, don't hesitate to leave a comment below. Happy coding!
Checkout our other articles