Mastering EJB 3.0: Troubleshooting Dependency Injection Issues
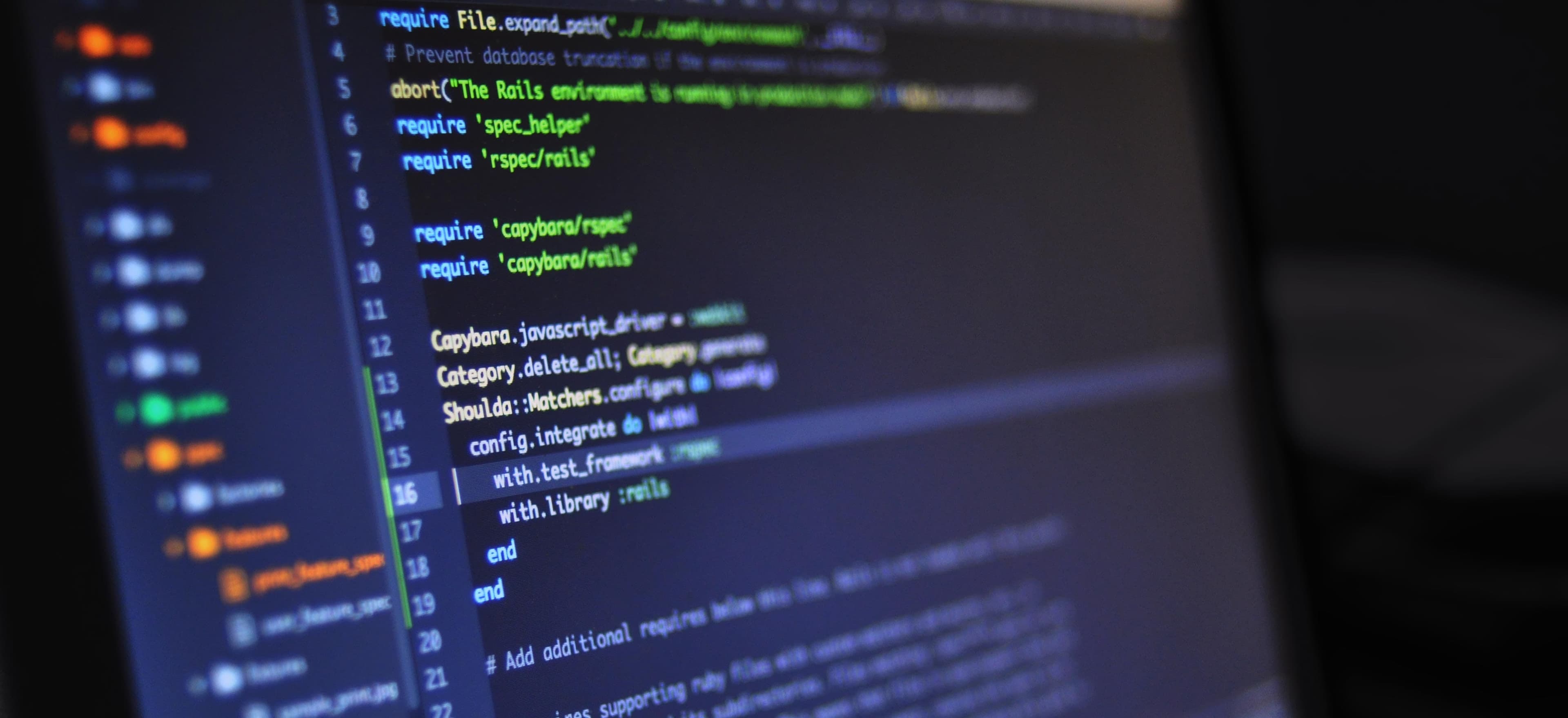
- Published on
Mastering EJB 3.0: Troubleshooting Dependency Injection Issues
Enterprise JavaBeans (EJB) 3.0 revolutionized Java EE programming by simplifying the development of enterprise-level applications. One of the key features contributing to this simplicity is Dependency Injection (DI). However, like any technology, EJB can present challenges. This blog post will guide you through troubleshooting common DI issues in EJB 3.0 applications while equipping you with the knowledge to master your Java EJB implementations.
Understanding Dependency Injection in EJB
Dependency Injection is a design pattern that allows you to remove hard-coded dependencies, making your applications more modular and easier to manage. In the context of EJB, DI enables you to bind components without direct instantiation in your code. Here's a brief overview of how EJB handles DI:
- Annotations: EJB uses annotations such as
@EJB
,@Inject
, and@Resource
to denote dependencies that will be injected at runtime. - Container Management: The EJB container manages the lifecycle of components and their dependencies, ensuring that they are available when needed.
- Loose Coupling: This pattern promotes loose coupling between classes, enhancing testability and maintainability.
Common Issues with Dependency Injection
Despite its advantages, EJB developers may encounter issues when using Dependency Injection. Here are some common problems and their solutions.
1. NullPointerException
When Accessing Injected Beans
When you attempt to access an injected bean, you might encounter a NullPointerException
. This often occurs due to:
- Incorrect injection point.
- Activation issues with the EJB container.
How to Fix:
-
Ensure that you're using the correct annotations. For example, if you're using
@Inject
, verify that the target bean is properly annotated and available in the context.@Stateless public class MyStatelessBean { @Inject private MyDependency myDependency; public void doSomething() { myDependency.execute(); // Ensure myDependency is not null } }
-
Verify that the bean definition exists in the same context where it’s being injected:
@EJB MyOtherStatelessBean myOtherBean; // Proper context resolution
2. CDI Scopes and Context Issues
Context and Dependency Injection (CDI) scopes can also lead to issues. Scope annotations like @RequestScoped
, @SessionScoped
, and @ApplicationScoped
determine the longevity of a bean and its availability.
How to Fix:
-
Ensure that you’re not trying to inject a bean with a shorter lifecycle into a longer-lived bean:
@SessionScoped public class MySessionBean { @Inject private MyRequestScopedBean myRequestBean; // Problematic, as myRequestBean can be null here. }
-
If you absolutely need a request-scoped bean in a session-scoped one, consider alternative approaches, such as utilizing producer methods.
3. Proxies and AOP Issues
EJB leverages proxies for various purposes including transaction management. When the injected instance is of an interface type, it could lead to unexpected behavior if business methods are not visible on the real implementation.
How to Fix:
-
Ensure interfaces are correctly defined and adhered to in your EJB implementation. Use interfaces to expose public methods that are business-related.
@Stateless public class MyStatelessBean implements MyStatelessBeanRemote { @Override public void performAction() { // Business logic here } }
4. Class Loading Problems
Another common issue arises due to class loading conflicts, especially when using multiple modules or libraries. This often results in NoClassDefFoundError
or ClassNotFoundException
.
How to Fix:
-
Verify your server's classpath configuration. Make sure that necessary dependencies are included in the correct locations.
-
Watch out for conflicting versions of libraries. Using Maven or Gradle can help manage these dependencies effectively.
Best Practices to Avoid Dependency Injection Pitfalls
-
Leverage Annotations Wisely: Make full use of annotations like
@Singleton
,@Stateless
,@Stateful
, and@ApplicationScoped
to define your components clearly. Contextual clarity aids deployment and reduces runtime issues. -
Consistent Naming Conventions: Use clear and consistent naming for your beans and resources. A well-structured naming convention helps in identifying and resolving issues quickly.
-
Utilize Unit Tests: Include unit tests for components to validate that the DI works as expected. Tools like JUnit and Mockito can simulate the EJB container, allowing you to verify the behavior of your beans.
-
Document Dependencies: Maintain clear documentation of your beans and their dependencies. This practice aids new developers in understanding the system and helps in troubleshooting.
Example Project Structure for EJB 3.0
To better illustrate how DI works and to provide a structure you can refer to, consider the following simple EJB project structure:
my-ejb-project/
|-- src/
| |-- main/
| | |-- java/
| | | |-- com/
| | | | |-- myapp/
| | | | | |-- beans/
| | | | | | |-- MyStatelessBean.java
| | | | | | |-- MyServiceBean.java
| |-- resources/
| | |-- META-INF/
| | | |-- persistence.xml
|-- pom.xml
Example Code Snippet: EJB with DI
Here's a sample code block showcasing how to use DI in an EJB:
package com.myapp.beans;
import javax.ejb.Stateless;
import javax.inject.Inject;
@Stateless
public class MyServiceBean {
@Inject
private MyStatelessBean myStatelessBean;
public void performService() {
// Use the injected bean
myStatelessBean.serviceMethod();
}
}
The Closing Argument
Mastering Dependency Injection in EJB 3.0 takes practice and diligence. By understanding common pitfalls and implementing best practices, you can avoid many of the common issues that arise in real-world scenarios. Remember, clear and modular code is your ally.
For further reading on EJB and Dependency Injection, consider visiting Oracle’s EJB documentation or exploring specific frameworks that complement EJB, such as Spring Framework.
Crafting robust enterprise applications with EJB is an attainable skill. With these insights into troubleshooting DI issues, you're well on your way to mastering EJB 3.0 and building effective, scalable Java applications. Happy coding!