Essential Setup for Effective Hibernate Testing
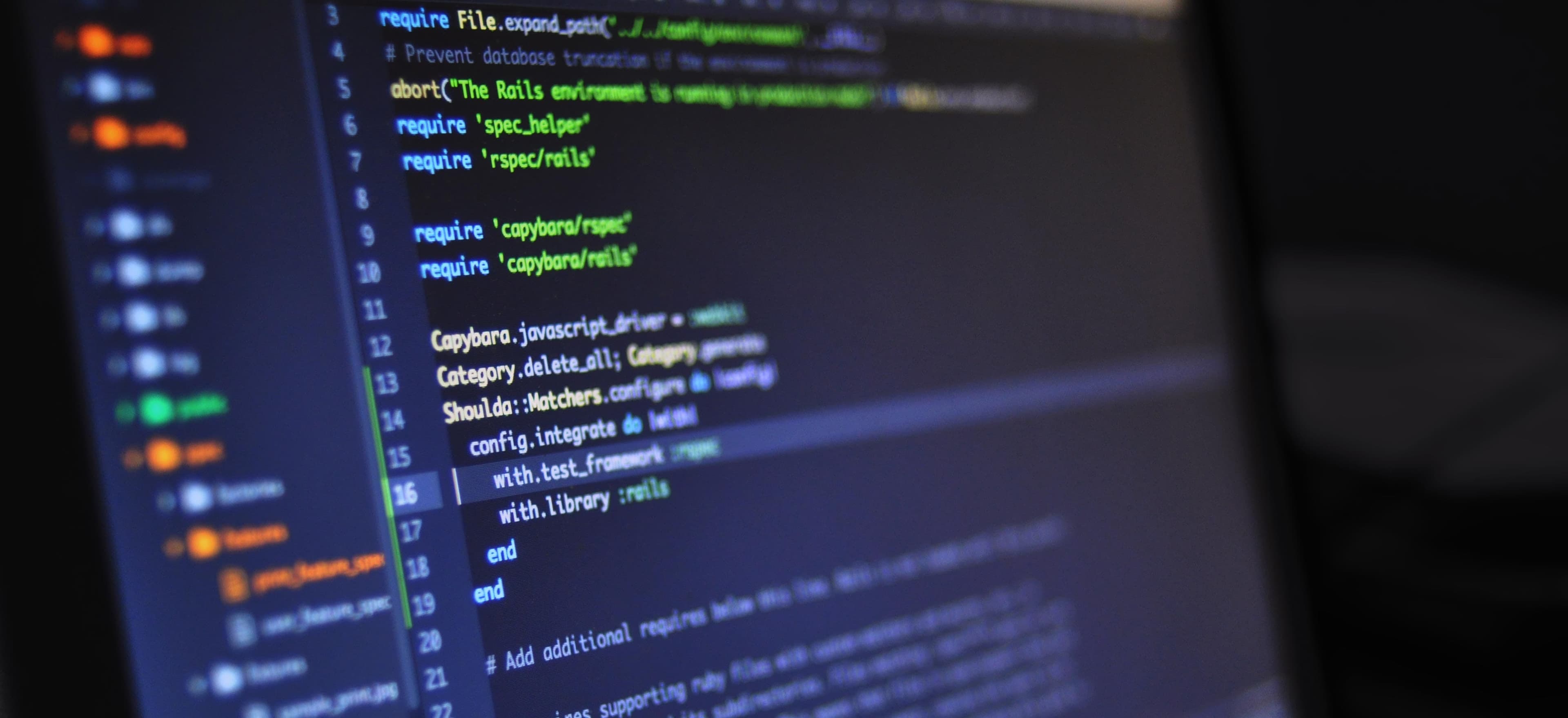
- Published on
Essential Setup for Effective Hibernate Testing
When working with Hibernate in Java applications, effective testing is vital to ensure that your data access layer functions as expected. In this blog post, we will explore essential setup strategies for testing Hibernate applications. From unit tests to integration tests, each has unique requirements and testing approaches. Let’s dive in!
What is Hibernate?
Hibernate is a powerful ORM (Object-Relational Mapping) framework for Java that simplifies database interactions. It allows developers to map Java classes to database tables and vice versa, enabling easier data manipulation. Understanding Hibernate is crucial for building robust applications, as it abstracts complexities and enhances productivity.
For those unfamiliar with ORM, you might want to start with an overview of Hibernate to fully grasp its functions and features.
Importance of Testing with Hibernate
Testing is a fundamental practice that helps maintain code quality. Specific advantages of testing Hibernate applications include:
- Ensuring Data Integrity: Verifying that the application correctly reads, saves, and updates data is crucial for any application.
- Identifying Bugs Early: Testing can catch errors early in the development cycle before they escalate into production issues.
- Performance Assurance: Hibernate can lead to performance bottlenecks if not configured properly. Testing can help identify such issues and ensure optimal configurations.
Types of Testing in Hibernate
1. Unit Testing
Typically, unit tests focus on individual components, such as repositories, in isolation. These tests verify if a specific method behaves as expected. With Hibernate, unit tests might require mocking the session and transaction management.
2. Integration Testing
Integration tests gauge how different components work together. In terms of Hibernate, this includes testing the interaction between your application and the database. Often, using an actual database (or an in-memory database like H2) for integration tests yields better results.
Key Dependencies
To effectively test Hibernate applications, you should include essential dependencies in your pom.xml
if you are using Maven. Here's a sample setup:
<dependencies>
<!-- Hibernate core dependency -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.21.Final</version>
</dependency>
<!-- JUnit for unit testing -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<!-- Mockito for mocking -->
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.6.28</version>
<scope>test</scope>
</dependency>
<!-- H2 Database for integration testing -->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<version>1.4.200</version>
<scope>test</scope>
</dependency>
</dependencies>
Sample Setup for Hibernate Testing
Let’s create a simple Hibernate model and showcase how to set up effective unit and integration tests.
Step 1: Create a Simple Hibernate Entity
We will define a User
entity:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class User {
@Id
private Long id;
private String name;
private String email;
// Constructors, Getters, and Setters
public User(Long id, String name, String email) {
this.id = id;
this.name = name;
this.email = email;
}
// Getters and Setters omitted for brevity
}
Step 2: Create a Repository for User
This repository provides access to the User
entity:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
public class UserRepository {
private SessionFactory sessionFactory;
public UserRepository(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public User findUserById(Long id) {
Session session = sessionFactory.openSession();
Transaction transaction = null;
User user = null;
try {
transaction = session.beginTransaction();
user = session.get(User.class, id);
transaction.commit();
} catch (Exception e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
return user;
}
}
Step 3: Unit Testing the Repository
Now, let’s write a unit test for the findUserById
method using Mockito to mock the Hibernate session:
import static org.mockito.Mockito.*;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.junit.Before;
import org.junit.Test;
public class UserRepositoryTest {
private SessionFactory sessionFactory;
private Session session;
@Before
public void setUp() {
sessionFactory = mock(SessionFactory.class);
session = mock(Session.class);
when(sessionFactory.openSession()).thenReturn(session);
}
@Test
public void testFindUserById() {
User expectedUser = new User(1L, "John Doe", "johndoe@example.com");
when(session.get(User.class, 1L)).thenReturn(expectedUser);
UserRepository repository = new UserRepository(sessionFactory);
User actualUser = repository.findUserById(1L);
assertEquals(expectedUser.getName(), actualUser.getName());
verify(session).get(User.class, 1L);
}
}
Explanation of Mocking
By mocking the SessionFactory
and Session
, we isolate the unit under test (the UserRepository
class). This way, we can test its logic without requiring a real database connection.
Step 4: Integration Testing
For integration testing, let’s configure Hibernate with an H2 in-memory database to test the repository's interactions with the database. For this, we can use JUnit:
import static org.junit.Assert.*;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class UserRepositoryIntegrationTest {
private SessionFactory sessionFactory;
private UserRepository userRepository;
@Before
public void setUp() {
sessionFactory = new Configuration().configure("hibernate.cfg.xml").buildSessionFactory();
userRepository = new UserRepository(sessionFactory);
// Set up an initial user in the database
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
User user = new User(1L, "John Doe", "johndoe@example.com");
session.save(user);
transaction.commit();
session.close();
}
@After
public void tearDown() {
sessionFactory.close();
}
@Test
public void testFindUserById() {
User user = userRepository.findUserById(1L);
assertNotNull(user);
assertEquals("John Doe", user.getName());
}
}
Explanation of Integration Testing
In this integration test, we set up an H2 in-memory database. By configuring Hibernate, we simulate a real database environment. The test checks whether the findUserById
method retrieves the correct data corresponding to our set-up user.
Best Practices for Hibernate Testing
-
Utilize In-memory Databases: H2 or similar databases provide fast results without needing to set up a full database server.
-
Keep Tests Independent: Ensure that each test can run independently without depending on the outcome of others.
-
Use Transaction Management: Utilize transactions to roll back changes after tests run to preserve data.
-
Create Test-Specific Configuration Files: Separate test configurations to avoid affecting production settings.
-
Automate Tests: Integrate testing into your CI/CD pipeline to ensure code quality throughout the development lifecycle.
In Conclusion, Here is What Matters
Setting up effective Hibernate testing involves a strategic approach that emphasizes both unit and integration testing. By utilizing mocks for isolating components and an in-memory database for integration tests, you can ensure your application remains robust and reliable. Good testing practices not only foster confidence in your code but also lead to maintainable and scalable software.
For more in-depth knowledge, check out the official Hibernate Testing Documentation. By implementing the strategies discussed here, you can enhance your understanding of Hibernate while ensuring the integrity of your applications. Happy coding!