Choosing Between CompletableFuture and Parallel Stream in Java 8
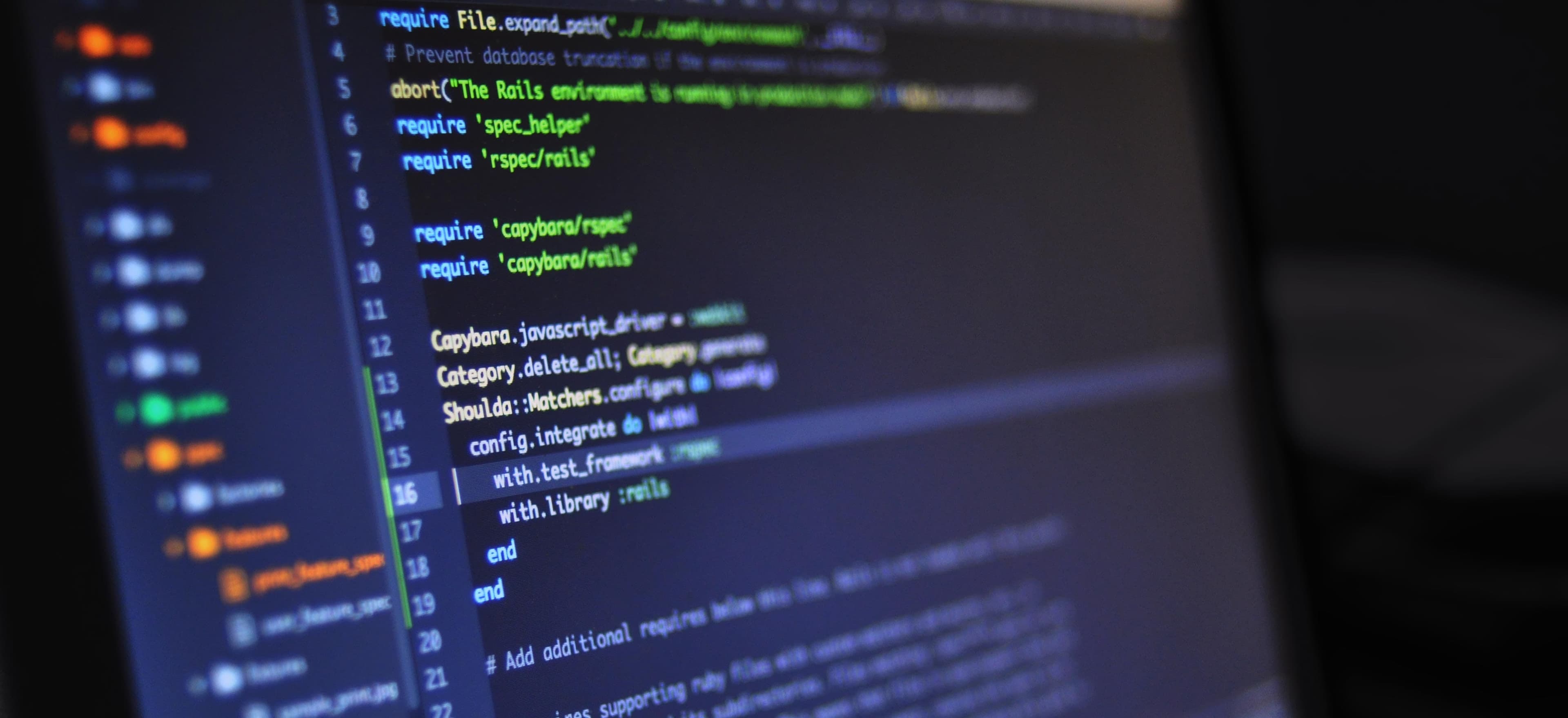
- Published on
Choosing Between CompletableFuture and Parallel Stream in Java 8
Java 8 brought a significant paradigm shift in how we approach concurrency and parallelism with the introduction of the CompletableFuture
and parallel streams. While both options have their unique advantages, deciding when to use one over the other can be cumbersome. In this post, we will dive deep into the characteristics of both, helping you make an informed choice.
Understanding Java's Concurrency Features
Java has long supported multi-threading, but Java 8 introduced a modern approach to dealing with asynchronous programming through functional interfaces. This has made concurrency easier to manage and much more readable.
What is CompletableFuture?
CompletableFuture
is part of the java.util.concurrent
package, designed to facilitate the creation of asynchronous tasks. This class allows you to write non-blocking code, meaning you can issue commands without waiting for them to complete.
Key Features:
- Enables handling asynchronous computations.
- Provides methods to compose multiple futures.
- Supports combining results from multiple asynchronous tasks using methods like
thenCombine()
,thenCompose()
, etc.
Here's a simple example illustrating how to use CompletableFuture
.
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> {
// Simulating long computation
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return 1;
});
CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> {
// Simulating another long computation
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return 2;
});
// Combine results
CompletableFuture<Integer> combinedFuture = future1.thenCombine(future2, Integer::sum);
// Block and get the result
System.out.println(combinedFuture.join()); // Output: 3
}
}
Why Use CompletableFuture?
-
Non-Blocking Behavior:
CompletableFuture
is non-blocking. When you create a future, you can submit other tasks without waiting for the first to complete. -
Flexible Composition: You can chain multiple operations, which can lead to more readable code in complex workflows.
-
Error Handling: It provides methods like
exceptionally()
to handle exceptions that may occur within asynchronous computations.
What Are Parallel Streams?
Parallel streams are a feature of Java 8 that allows you to process collections in parallel. By utilizing multiple cores, parallel streams can improve performance for large datasets by splitting work across processors.
Key Features:
- Uses the Fork/Join framework to process collections efficiently.
- Processes data in a more declarative way compared to traditional loops.
Here's a sample code to show how to use parallel streams.
import java.util.Arrays;
import java.util.List;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.parallelStream()
.mapToInt(Integer::intValue)
.sum();
// Output: 15
System.out.println(sum);
}
}
Why Use Parallel Streams?
-
Simplicity: You can compute results quickly by focusing only on operations without managing threads.
-
Automatic Partitioning: The Fork/Join framework splits the collection under-the-hood, allowing for optimal CPU resource utilization.
-
Performance: For computations involving large data sets, using parallel streams can lead to significant performance improvements.
When Should You Use Which?
CompletableFuture: Use Cases
-
Asynchronous Tasks: When your computations involve I/O-bound operations such as web requests or database calls.
-
Complex Workflows: When tasks depend on multiple asynchronous operations,
CompletableFuture
shines. You can easily chain operations and manage dependencies. -
Improved Error Handling: When you require robust error handling strategies through future compositions.
Parallel Streams: Use Cases
-
CPU-bound Operations: When you want to perform calculations that can benefit from multi-core processors.
-
Aggregation Tasks: When the task involves aggregating collections or working with large data sets, parallel streams can offer a better performance.
-
Simplicity Over Control: When your task is straightforward and doesn't need the complexity of thread management, parallel streams offer a succinct and simple way to achieve parallelism.
Performance Considerations
While both CompletableFuture
and parallel streams are efficient in their usage, their performance can diverge based on the nature of the tasks.
-
For CPU-intensive tasks involving heavy computation, parallel streams generally yield better results because they leverage all your CPU cores.
-
For tasks that rely heavily on waiting, such as I/O-bound tasks,
CompletableFuture
can prove more effective and practical.
Wrapping Up
In summary, both CompletableFuture
and parallel streams are invaluable tools introduced in Java 8 for handling concurrency effectively. Understanding their capabilities and use cases will help you make more informed decisions in your programming tasks.
- Use CompletableFuture when:
- You need non-blocking I/O operations.
- Your tasks involve complex workflows or dependencies.
- Choose parallel streams when:
- You're processing large collections or datasets in a relatively simple manner.
- You can effectively leverage multiple CPU cores.
No matter which you choose, mastering these tools will undoubtedly enhance your programming prowess in Java. For additional insights into asynchronous programming in Java, check out Java Documentation on CompletableFuture and Java Documentation on Streams.
Happy coding!