Overcoming Common Pitfalls in Continuous Delivery
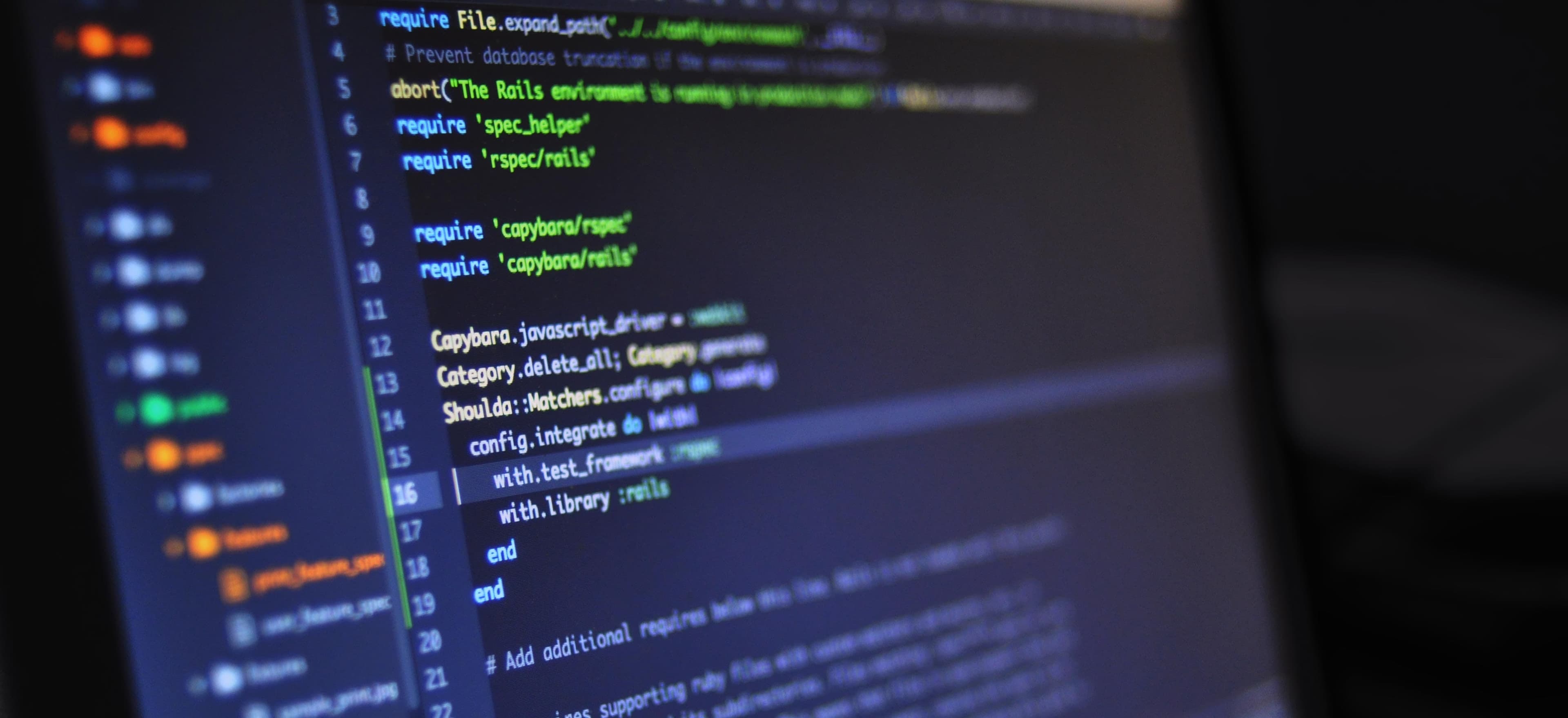
- Published on
Overcoming Common Pitfalls in Continuous Delivery
Continuous Delivery (CD) has revolutionized how software is developed and deployed. It emphasizes speed, efficiency, and the ability to reliably deliver software to users. However, many organizations struggle with common pitfalls associated with implementing Continuous Delivery. This blog post will explore these pitfalls and provide strategies to overcome them.
Understanding Continuous Delivery
Before diving into the pitfalls, let’s briefly define Continuous Delivery. It is a software development practice where code changes are automatically prepared for a release to production. CD requires a set of best practices in development, testing, and deployment to ensure that new features can be reliably released at any time.
Benefits of Continuous Delivery
- Faster Time to Market: Shortened release cycles enable organizations to respond to market demands quickly.
- Higher Quality Code: Automatic testing ensures fault detection early in the development phase.
- Lower Risk of Failures: Frequent integrations reduce the chances of massive deployment failures.
Despite these advantages, many teams encounter hurdles on their journey to achieve effective Continuous Delivery. Let’s explore these common pitfalls and how to overcome them.
Common Pitfalls in Continuous Delivery
1. Lack of Automation
The Problem:
One of the most significant pitfalls in adopting Continuous Delivery is the lack of automation. Manual processes can slow down the deployment pipeline and introduce human error.
Solution:
Identify repetitive tasks in your development and deployment workflows and automate them. Use tools like Jenkins, GitLab CI, or CircleCI for CI/CD pipelines.
Example: Setting Up a Basic CI/CD Pipeline with Jenkins
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building..'
// Insert build commands here
sh 'mvn clean package'
}
}
stage('Test') {
steps {
echo 'Testing..'
// Insert test commands here
sh 'mvn test'
}
}
stage('Deploy') {
steps {
echo 'Deploying..'
// Insert deployment commands here
sh 'scp target/myapp.war user@server:/path/to/deploy'
}
}
}
}
Why Automation?
Automation minimizes errors, frees up developer time, and allows for continuous integration and deployment.
2. Poor Testing Practices
The Problem:
Many teams assume having automated tests is sufficient, but if those tests are not robust, they can lead to faulty releases.
Solution:
Implement a comprehensive testing strategy that includes unit tests, integration tests, and end-to-end tests. Continuous testing is vital!
Example: Writing a Simple JUnit Test
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
Why Comprehensive Testing?
Comprehensive testing ensures that every aspect of your application works as expected. Catching bugs early helps avoid reverting deployments and costly rollbacks later.
3. Inadequate Feedback Loop
The Problem:
Without a proper feedback mechanism, teams can overlook important issues that arise during the deployment process.
Solution:
Set up notifications and monitoring systems to provide real-time feedback on the deployment process. Utilize tools like Prometheus for monitoring and Slack for notifications.
Example Notification Setup:
# Prometheus alert rule
groups:
- name: example
rules:
- alert: HighErrorRate
expr: rate(http_requests_total{status="500"}[5m]) > 0.05
for: 2m
labels:
severity: page
annotations:
summary: "High error rate on {{ $labels.instance }}"
description: "The error rate is over 5% for the last 5 min."
Why Real-time Feedback?
Real-time feedback enables teams to react swiftly to issues, minimizing downtime and enhancing user satisfaction.
4. Resistance to Change
The Problem:
Cultural resistance within teams can hinder the best Continuous Delivery efforts. Change is often met with skepticism.
Solution:
Encourage a culture of Continuous Improvement. Support team training and create awareness about the benefits of Continuous Delivery.
Create Champions:
Identify team members who are enthusiastic about CD and empower them to lead training sessions.
Why Embrace Change?
Embracing change fosters an adaptive mindset. This not only improves the development process but also strengthens team collaboration.
5. Ignoring Security Practices
The Problem:
In the rush for speed, security is sometimes neglected, leading to vulnerabilities in the deployed application.
Solution:
Integrate security practices into your CI/CD pipeline, often referred to as DevSecOps. Regularly scan your code for vulnerabilities and employ tools like Snyk and OWASP ZAP.
Example: Implementing Snyk in Your Pipeline
stages:
- test
- deploy
test:
stage: test
script:
- npm install
- snyk test
deploy:
stage: deploy
script:
- echo "Deploying application..."
Why Secure Development?
Incorporating security measures into the pipeline ensures that vulnerabilities are detected before they reach production, safeguarding your applications and users.
To Wrap Things Up
Continuous Delivery is a powerful approach to software development, offering myriad benefits ranging from enhanced speed to reduced risks. However, several challenges can impede a smooth implementation. By addressing pitfalls like lack of automation, poor testing practices, inadequate feedback loops, resistance to change, and ignoring security measures, teams can create a robust Continuous Delivery environment.
Moving Forward
Establishing a successful Continuous Delivery strategy requires time, commitment, and continual learning. By overcoming these common pitfalls, organizations can deliver high-quality software faster, ultimately providing greater value to their users.
Additional Resources
For further reading on Continuous Delivery:
Embrace the journey of Continuous Delivery, learn from the pitfalls, and turn them into stepping stones towards success!