Common Pitfalls in Spock Mocking You Should Avoid
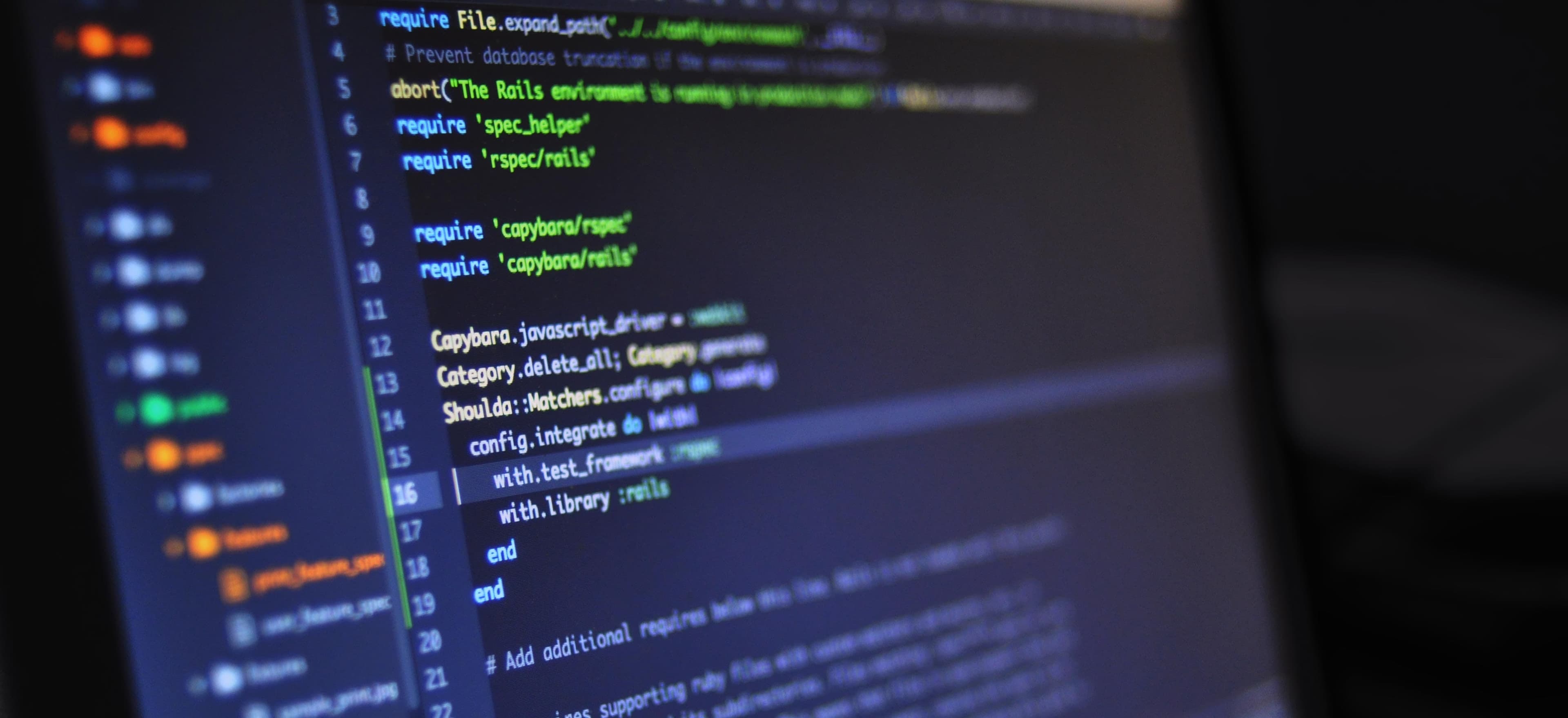
- Published on
Common Pitfalls in Spock Mocking You Should Avoid
Spock is a powerful testing framework for Java and Groovy applications that aims to simplify the testing process through its expressive language and concise structure. One of the key features of Spock is its ability to handle mocking and stubbing efficiently. However, like any powerful tool, improper use can lead to common pitfalls that can obscure and destabilize your tests. In this blog post, we will explore some typical mistakes developers make when using mocking in Spock and offer guidance on how to avoid them.
Understanding Mocking and Stubbing
Before delving into the pitfalls, let's clarify what we mean by mocking and stubbing.
- Mocking is the process of creating a simulated object that mimics the behavior of a real object. This is useful for isolating the unit under test and ensuring that tests are not dependent on external systems.
- Stubbing provides predefined responses to method calls without verifying the interactions with the method or the way it was called.
Mocking and stubbing are essential for unit testing, and Spock makes these tasks easier. But remember, with great power comes great responsibility.
Pitfall 1: Over-Mocking
Explanation
One of the most common mistakes in testing is over-mocking. While it might seem like a good idea to mock every dependency, it can lead to tests that are difficult to understand and maintain. It can also lead to brittle tests that break for reasons unrelated to the component being tested.
Example
class UserService {
UserRepository userRepository
NotificationService notificationService
User createUser(String username) {
def user = new User(username: username)
userRepository.save(user)
notificationService.sendNotification(user)
return user
}
}
In the code above, if we mock both userRepository
and notificationService
, the test might not provide much value since we're checking the interactions rather than the actual logic of createUser
.
Recommendation
Mock only the dependencies that are necessary for isolating your unit under test. If a method primarily interacts with one dependency, consider leaving other dependencies as real instances or stubbing them minimally.
def "should save user and send notification"() {
given:
def userRepository = Mock(UserRepository)
def notificationService = Mock(NotificationService)
UserService userService = new UserService(userRepository: userRepository,
notificationService: notificationService)
when:
def user = userService.createUser("john_doe")
then:
1 * userRepository.save(user)
1 * notificationService.sendNotification(user)
}
Pitfall 2: Ignoring Behavior Verification
Explanation
In Spock, you can both mock and verify interactions with mocked objects. A frequent error is to use mocks extensively while neglecting to verify that the correct methods were called with the expected parameters.
Example
def "user should be saved"() {
given:
def userRepository = Mock(UserRepository)
UserService userService = new UserService(userRepository: userRepository)
when:
userService.createUser("john_doe")
then:
// A common oversight is not verifying interactions
}
Recommendation
Always verify that the expected methods are called on your mock objects. This not only ensures that your code interacts correctly with its dependencies but also improves the clarity of your tests.
then:
1 * userRepository.save(_)
Using the _
wildcard allows you to verify that save
is called without checking the specifics of the user object.
Pitfall 3: Not Using Spock's Built-In Features
Explanation
Spock offers several features that enhance test maintainability, such as data-driven testing and interaction-based testing. Failing to leverage these features can lead to duplication and convoluted test scenarios.
Example
Instead of writing separate tests for each scenario of user creation, you can use Spock's data-driven testing feature to handle multiple cases in one test.
Recommendation
Use Spock's where
block for data-driven testing. It can significantly reduce code duplication.
def "should create user with different usernames"() {
given:
def userRepository = Mock(UserRepository)
UserService userService = new UserService(userRepository: userRepository)
when:
def user = userService.createUser(username)
then:
1 * userRepository.save(user)
where:
username << ["john_doe", "jane_doe", "user_123"]
}
Pitfall 4: Misunderstanding Mocking Levels
Explanation
Spock provides different levels of mocking, including mocks, stubs, and spies. A frequent pitfall is misunderstanding when to use each type, which can lead to unnecessary complexity.
Recommendation
- Use mocks for behavior verification, where you want to ensure that certain methods are called.
- Use stubs when you only need to provide specific results for method calls without caring about the interactions.
- Use spies when you want to partially mock a real object to verify behavior while still leveraging its actual implementation.
Example of Creating a Stub
def "user repository should return user"() {
given:
def userRepository = Stub(UserRepository) {
findByUsername("john_doe") >> new User("john_doe")
}
UserService userService = new UserService(userRepository: userRepository)
when:
def user = userService.getUserByUsername("john_doe")
then:
user.username == "john_doe"
}
Pitfall 5: Using Incorrect Argument Matchers
Explanation
Another common pitfall is using argument matchers incorrectly. This can lead to tests failing unexpectedly or passing when they shouldn’t.
Example
def "should save user"() {
given:
def userRepository = Mock(UserRepository)
UserService userService = new UserService(userRepository: userRepository)
when:
userService.createUser("john_doe")
then:
// Using wrong argument matcher can lead to misleading assertions
1 * userRepository.save("john_doe")
}
Recommendation
Use the appropriate argument matchers, like _
, any()
, etc., based on the context of your tests.
then:
1 * userRepository.save({ it.username == "john_doe" })
Final Thoughts
Mocking in Spock can streamline your testing process and clarify interactions, but it is crucial to avoid these common pitfalls. By practicing mindful mocking, verifying behaviors that matter, and leveraging Spock's features, you can write clear, maintainable, and effective tests.
For more insights, check out the official Spock documentation and enhance your testing prowess!
Happy testing!