Mastering Oracle Functions: Common Pitfalls to Avoid
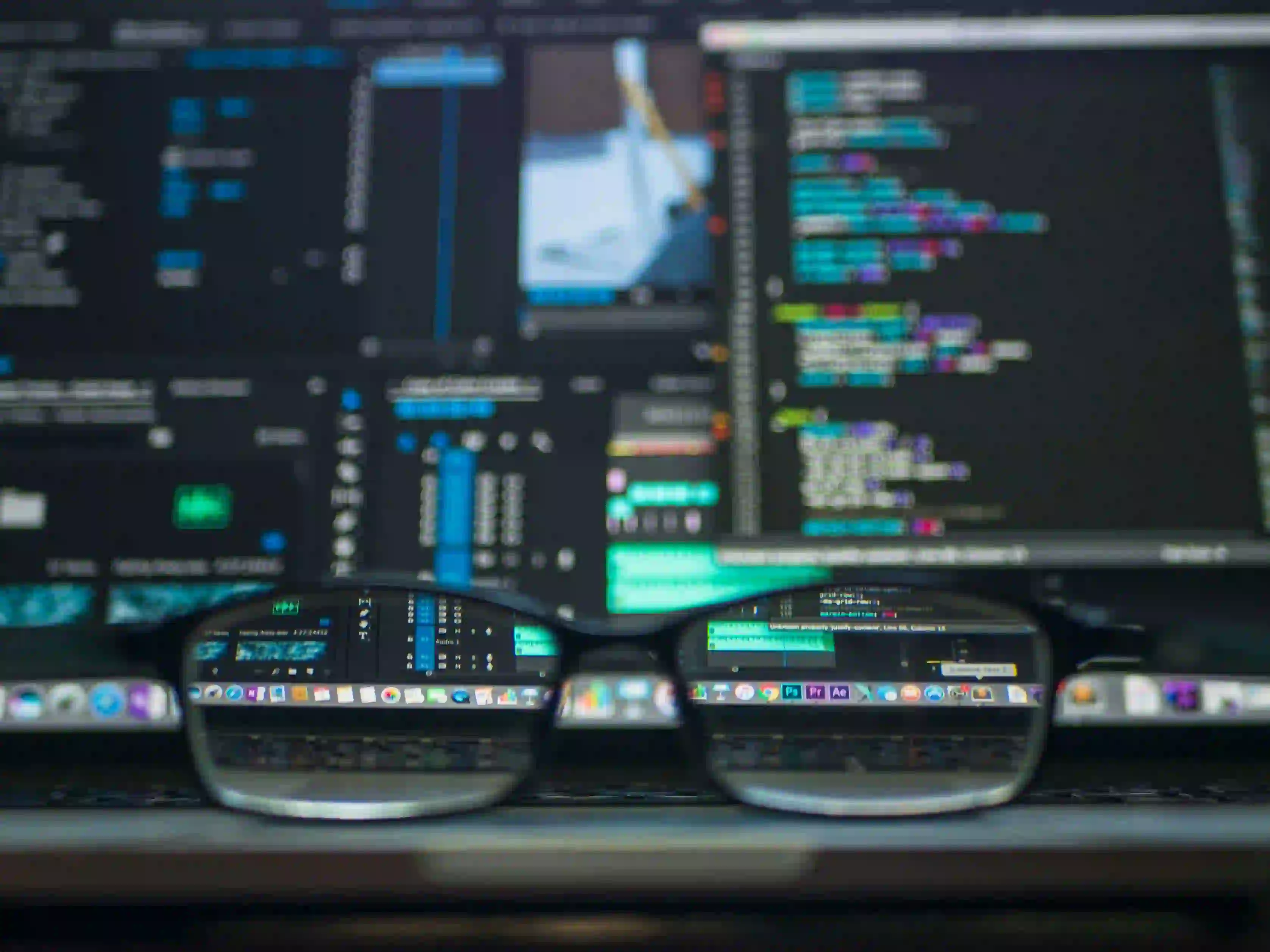
Mastering Oracle Functions: Common Pitfalls to Avoid
When working with Oracle databases, utilizing functions can greatly enhance your ability to manipulate, summarize, and analyze data effectively. However, as with any powerful tool, there are common pitfalls that developers can encounter. Understanding these pitfalls is crucial not only for troubleshooting but for creating efficient, secure, and maintainable SQL code.
In this article, we will delve into Oracle functions, their significance, and the common mistakes developers make. By learning to avoid these pitfalls, you can master Oracle functions and improve your database interactions.
Understanding Oracle Functions
Oracle functions are built-in routines that can be used to perform operations on the data stored within the database. They take parameters, execute operations, and return values. This capability allows for data manipulation and enhanced query efficiency.
Types of Oracle Functions
- Single-row functions: Operate on single rows of data and return a single value.
- Aggregate functions: Operate on multiple rows and return a single summary value (e.g., COUNT, MAX, AVG).
- Analytic functions: Perform calculations across a set of rows that are somehow related to the current row.
- User-defined functions: Custom functions created by developers to suit specific needs.
Importance of Oracle Functions
Oracle functions encapsulate complex logic, promote code reuse, and optimize performance. They allow developers to create modular code through abstraction, simplifying code maintenance and enhancing readability.
Common Pitfalls When Using Oracle Functions
While functions are powerful, mishandling them can lead to errors, performance issues, and unexpected outcomes. Let's explore some of the most common pitfalls and how to avoid them.
1. Not Understanding NULL Handling
One common mistake is not properly handling NULL
values. Functions in Oracle can behave unexpectedly when they encounter NULL
data.
Example Snippet
SELECT SUM(salary) FROM employees;
Commentary: The above query will return NULL
if there are no employees, potentially leading to confusion. To handle this, you can utilize the NVL
function.
SELECT NVL(SUM(salary), 0) FROM employees;
This ensures that you get zero rather than NULL
. It's crucial always to anticipate NULL
values and implement proper handling strategies.
2. Overusing PL/SQL in SQL Queries
Integrating PL/SQL functions into SQL statements can lead to performance degradation. This often happens when developers don't leverage SQL's inherent strengths, opting for PL/SQL where simple SQL would suffice.
Example Snippet
-- Using PL/SQL function within a SQL statement
CREATE OR REPLACE FUNCTION calculate_bonus(emp_id NUMBER) RETURN NUMBER IS
BEGIN
RETURN (SELECT salary * 0.10 FROM employees WHERE employee_id = emp_id);
END;
Commentary: Instead of using the PL/SQL function, adopting SQL directly within queries is often more efficient.
SELECT employee_id, salary * 0.10 AS bonus FROM employees;
This direct approach is not only simpler but also allows the Oracle optimizer to do its job more efficiently.
3. Ignoring the Performance Impact of Functions
Another major pitfall is not considering the performance implications of using functions in WHERE clauses. Functions can cause performance issues by preventing database optimizations.
Example Snippet
SELECT * FROM employees WHERE NVL(department_id, 0) = 10;
Commentary: The above query might inhibit Oracle from using indexes effectively. Instead, try to rewrite your logic:
SELECT * FROM employees WHERE department_id = 10 OR department_id IS NULL;
This approach can utilize indexes properly and improve query performance.
4. Neglecting to Use Appropriate Functions
Oracle has a robust library of built-in functions, but developers often overlook these, resulting in unnecessarily complicated code. Always evaluate if an existing function can simplify your code.
Example Snippet
-- Finding the longest name in the employees table
SELECT MAX(LENGTH(first_name || ' ' || last_name)) FROM employees;
Commentary: Instead of manually constructing the longest name, consider using the built-in CONCAT
function for better readability and performance.
SELECT MAX(LENGTH(CONCAT(first_name, last_name))) FROM employees;
Embrace Oracle functions designed for specific tasks to simplify and enhance your code.
5. Not Testing User-defined Functions
User-defined functions introduce custom logic but can easily incorporate bugs. Failing to adequately test these functions can result in missed errors and unexpected behaviors in production.
Example Snippet
CREATE OR REPLACE FUNCTION calculate_age(birth_date DATE) RETURN NUMBER IS
BEGIN
RETURN TRUNC(MONTHS_BETWEEN(SYSDATE, birth_date) / 12);
END;
Commentary: This function requires comprehensive testing, especially for boundary conditions like leap years, months with varying days, and future dates. Always validate your logic rigorously before deployment.
6. Ignoring Security Risks with User-Defined Functions
When creating user-defined functions, developers sometimes overlook security implications, exposing the database to SQL injection vulnerabilities. Always validate input parameters thoroughly.
Example Snippet
CREATE OR REPLACE FUNCTION get_employee_details(emp_id NUMBER) RETURN VARCHAR2 IS
emp_name VARCHAR2(100);
BEGIN
SELECT name INTO emp_name FROM employees WHERE employee_id = emp_id;
RETURN emp_name;
END;
Commentary: If emp_id
is not validated, an attacker could exploit this functionality. Always implement input validation measures, such as:
CREATE OR REPLACE FUNCTION get_employee_details(emp_id NUMBER) RETURN VARCHAR2 IS
emp_name VARCHAR2(100);
BEGIN
-- Validate emp_id
IF emp_id <= 0 THEN
RETURN 'Invalid Employee ID';
END IF;
...
7. Not Leveraging Analytical Functions
Analytic functions can simplify complex calculations, but developers often resort to cumbersome joins and subqueries instead of taking advantage of these robust tools.
Example Snippet
-- Using a subquery to find the average salary by department
SELECT e1.department_id, AVG(e1.salary)
FROM employees e1
JOIN employees e2 ON e1.department_id = e2.department_id
GROUP BY e1.department_id;
Commentary: Instead of this approach, analytic functions can yield cleaner, more efficient results:
SELECT department_id, salary, AVG(salary) OVER (PARTITION BY department_id) AS avg_salary
FROM employees;
This method enhances readability and performance.
Key Takeaways
Mastering Oracle functions requires understanding their strengths, weaknesses, and common pitfalls. By avoiding the discussed mistakes, you can significantly enhance the performance, reliability, and security of your Oracle database solutions.
To further solidify your knowledge, explore the official Oracle documentation on SQL functions, and consider diving into Oracle's Analytic Functions for advanced data manipulations.
By continuously learning and refining your understanding of Oracle functions, you position yourself for greater success in database development and management. Happy coding!