Balancing Type Safety and Expressiveness: A Developer's Dilemma
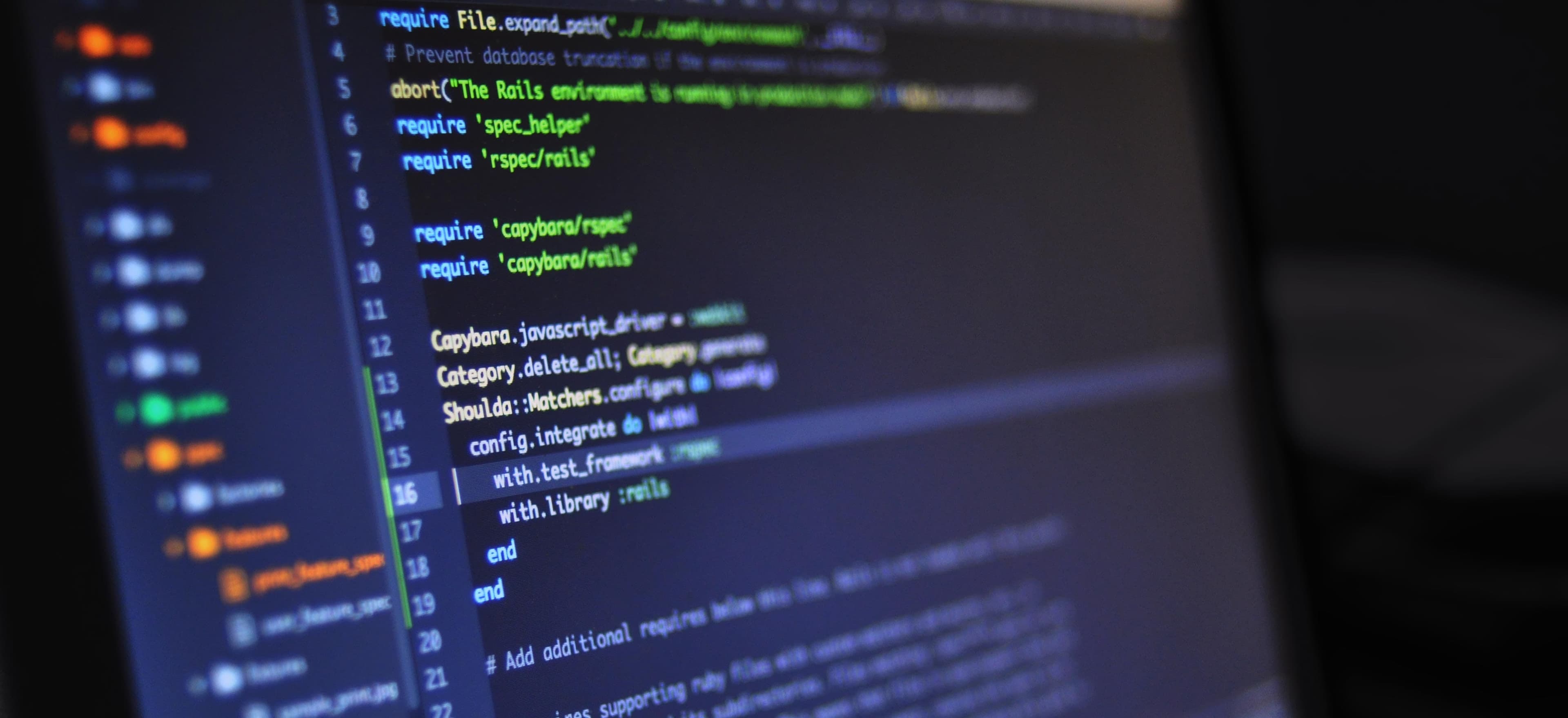
- Published on
Balancing Type Safety and Expressiveness: A Developer's Dilemma in Java
Java has long been celebrated for its strong type system. This system provides developers with several advantages: it prevents many runtime errors, enhances code readability, and enables better tooling and refactoring. However, this strong type system can sometimes clash with the desire for expressiveness in code — the ability to write clear and concise code that effectively communicates intent. This post delves into the delicate balance between type safety and expressiveness in Java, highlighting why this is a critical consideration for developers.
The Importance of Type Safety
Type safety in Java ensures that variables are bound to a specific data type. This checks for type-related errors at compile-time rather than at runtime. For instance, consider the following Java code that defines a method for dividing two numbers:
public double divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator cannot be zero.");
}
return (double) numerator / denominator;
}
Why This Matters
- Error Prevention: Type safety reduces the likelihood of errors. For instance, without a strong type system, a developer might accidentally pass a string to the
divide
method, resulting in a runtime exception. - Clarity: The method signature clearly indicates the expected types, making it easier for others to understand how to correctly use the method.
The downside? This rigidity can stifle creativity or lead to verbose code.
The Quest for Expressiveness
Expressiveness allows developers to write code that communicates its intent more efficiently. Let's take an example using Java's optional type to express the potential absence of a value:
import java.util.Optional;
public Optional<Double> safeDivide(int numerator, int denominator) {
if (denominator == 0) {
return Optional.empty(); // No result
}
return Optional.of((double) numerator / denominator);
}
In the safeDivide
method, we've opted for Optional<Double>
instead of throwing an exception.
Why This Matters
- Conciseness: The code succinctly conveys that the method might not return a valid number rather than cluttering it with error-handling logic upfront.
- Improved Readability: It indicates that the absence of a value is an acceptable and expected outcome, encouraging users of the method to think about potential nulls right away.
However, to achieve this expressiveness, we’ve shifted slightly away from strict type safety.
Striking the Balance: Generics
Java's generics offer an excellent compromise between type safety and expressiveness. Generics enable developers to create classes, interfaces, and methods that can operate on one or more types while still maintaining type safety. Consider a simple generic class as follows:
public class Box<T> {
private T content;
public void setContent(T content) {
this.content = content;
}
public T getContent() {
return content;
}
}
Benefits of Generics
- Flexibility: Developers can use the
Box
class with different types without losing type safety. - Clear API: Type parameters make it clear what kind of object is being dealt with. This adds expressiveness to the API.
By allowing certain parts of our code to be type-agnostic, we achieve more concise code without sacrificing safety.
Patterns in Practice: Using Interfaces and Abstract Classes
Another way to balance type safety with expressiveness is through the use of interfaces and abstract classes. These constructs allow developers to define contracts for behavior while retaining the ability to implement diverse classes. For instance:
public interface Shape {
double area();
}
public class Circle implements Shape {
private final double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
Why This Matters
- Type Safety: The
Shape
interface ensures that any implementing class provides anarea()
method, enforcing a consistent API. - Flexibility: Developers can work with any class that implements
Shape
, leading to a powerful polymorphic behavior.
This pattern enhances expressiveness, allowing for cleaner designs, such as the Strategy or Factory patterns.
Limitations of Type Safety and Expressiveness
While balancing type safety and expressiveness is crucial, it's important to recognize the limitations. Some scenarios may lead to complex code, losing clarity in favor of type constraints. For example, the following code uses type parameters to produce a utility method, but leads to complex syntax:
public static <T extends Number> double sumList(List<T> list) {
double sum = 0;
for (T number : list) {
sum += number.doubleValue();
}
return sum;
}
Why This Matters
- Complexity: While generics provide safety, they can lead to complications in usage — especially when dealing with multiple constraints or nested generics.
- Readability: Developers unfamiliar with generics may find such methods challenging to read and implement effectively.
This underscores the importance of considering your team's familiarity with constructs while striving for balance.
Real-World Considerations
When making decisions regarding the balance between type safety and expressiveness, consider these key factors:
- Team Knowledge and Experience: Ensure that your team is comfortable with generics and complex type systems.
- Use Case: Assess whether the added complexity is justified by the benefits in safety and clarity.
Start small. Implement type-safe methods with clear error handling, then gradually introduce generics and interfaces as the team acclimates to the pattern.
Bringing It All Together
Balancing type safety and expressiveness in Java is a nuanced journey. Type safety provides robust error prevention and code clarity, while expressiveness fosters concise and intention-revealing code.
Java offers a wealth of tools to strike this balance, from generics to interface design. As developers, the key lies in leveraging these features thoughtfully, remaining aware of the costs they might impose, and evaluating them in the context of real-world applications.
For more depth on the implications of type systems in programming, you might find this article on programming languages illuminating. Additionally, exploring Java Generics can provide further insights into how to harness the power of generics for your development needs.
By mastering this delicate balance, developers can craft applications that are not only robust and safe but also elegant and expressive. Happy coding!
Checkout our other articles