Mastering Java Error Handling: Beyond Standard Exceptions
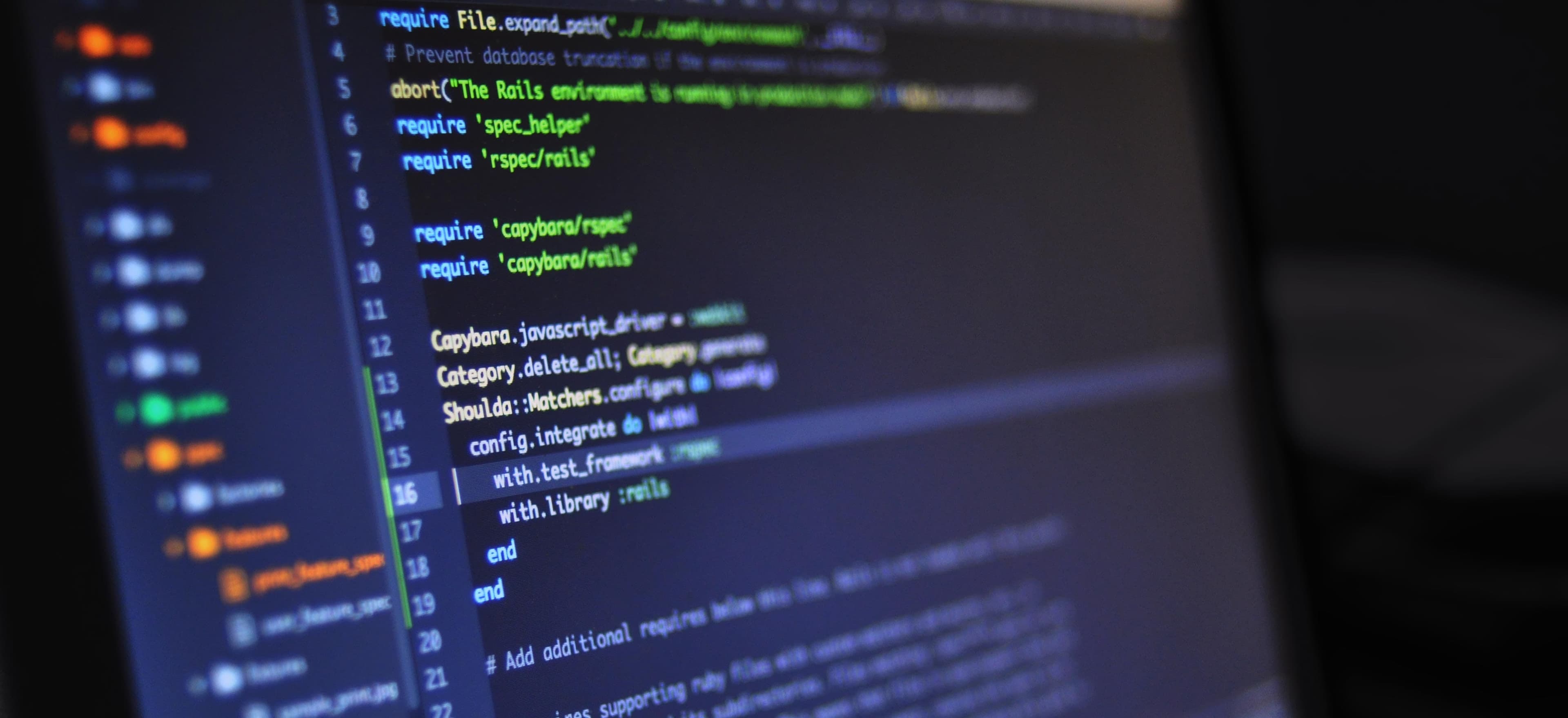
- Published on
Mastering Java Error Handling: Beyond Standard Exceptions
Error handling is a crucial aspect of software development. Particularly in Java, where robust exception handling is integrated into the design of the language, understanding how to navigate errors can significantly impact your application's performance and reliability.
While Java provides built-in exceptions that help you manage errors, there exists a rich ecosystem of custom exceptions and error-handling techniques that can elevate your coding skills. This blog post dives deep into advanced error-handling strategies, equipping you with the knowledge to master Java error handling.
Understanding Standard Exceptions
Before we explore advanced techniques, it's important to understand the standard exceptions Java offers. Java's built-in exception handling framework consists of Throwable
, Error
, and Exception
.
- Throwable: The superclass of all errors and exceptions in the Java language.
- Error: Represents serious problems that a typical application should not catch.
- Exception: Represents conditions that a typical application might want to catch.
Java exceptions can be further categorized into checked and unchecked exceptions:
- Checked Exceptions: These are exceptions that need to be declared in the method signature (e.g.,
IOException
,SQLException
). - Unchecked Exceptions: These exceptions can occur at any point during execution and do not need to be declared (e.g.,
NullPointerException
,ArrayIndexOutOfBoundsException
).
Understanding these predefined exceptions lays the groundwork for advanced error handling.
Custom Exceptions: Why and How to Create Them
Creating your custom exception classes can help you manage specific error situations effectively. Custom exceptions allow for better error categorization and improved readability.
Why Create Custom Exceptions?
- Specificity: Provide clear indication of what went wrong.
- Maintainability: Makes code more understandable at a glance.
- Control: Enables you to handle exceptions in a more controlled manner.
How to Create Custom Exceptions
Here's how you can create a custom exception in Java:
public class InvalidUserInputException extends Exception {
public InvalidUserInputException(String message) {
super(message);
}
}
In this snippet, we define InvalidUserInputException
, a custom exception that extends Exception
. It accepts a message that provides insight into the error.
Using Custom Exceptions
You can use the custom exception within your application as follows:
public void processInput(String userInput) throws InvalidUserInputException {
if (userInput == null || userInput.isEmpty()) {
throw new InvalidUserInputException("User input cannot be null or empty.");
}
// Process the input
}
In the method processInput
, we check for invalid user input. If the condition is not met, we throw our custom InvalidUserInputException
. This practice allows for better clarity and control over error management in your application.
Best Practices in Error Handling
To ensure that error handling remains effective, it is crucial to follow certain best practices:
1. Use Specific Exceptions
Avoid using generic exceptions like Exception
or RuntimeException
. Specific exceptions provide more context for debugging.
2. Don't Swallow Exceptions
While it might be tempting to catch exceptions and do nothing, this obscures errors. Always log caught exceptions.
try {
riskyOperation();
} catch (SomeSpecificException e) {
logger.error("Something went wrong", e);
}
3. Always Clean Up
In cases of resources that need to be closed (like files or network connections), ensure you clean up using finally
blocks or Java's try-with-resources statement.
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
// Read from the file
} catch (IOException e) {
logger.error("File not found", e);
}
4. Document Your Exceptions
When writing methods that throw exceptions, document them using Javadoc.
/**
* Processes the user input.
*
* @param userInput the input to process
* @throws InvalidUserInputException if the input is null or empty
*/
public void processInput(String userInput) throws InvalidUserInputException {
// Implementation
}
Advanced Error Handling Techniques
Beyond the basics lies a multitude of advanced techniques you can leverage.
1. Exception Wrapping
Sometimes, you might have an exception that is too generic or that originates from a deeply nested method. Wrapping exceptions can help by providing more context.
public void process() {
try {
doRiskyOperation();
} catch (LowLevelException e) {
throw new HighLevelException("High level error occurred", e);
}
}
In this example, LowLevelException
is re-thrown as HighLevelException
to provide more context at a higher level.
2. Global Exception Handling
For applications like web applications, consider implementing a global exception handler. This allows you to maintain a centralized place for error handling.
Using Spring Boot for example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(InvalidUserInputException.class)
public ResponseEntity<String> handleInvalidInput(InvalidUserInputException e) {
return ResponseEntity.badRequest().body(e.getMessage());
}
}
This approach not only simplifies error handling but also keeps your controllers clean and focused on their intended functionality.
3. Use of Optional
In scenarios where a method may not return a value, consider using the Optional
class introduced in Java 8.
public Optional<User> findUserById(String userId) {
if (userId == null) {
return Optional.empty();
}
// Logic to retrieve user
}
With Optional
, you can avoid unnecessary checks for null and provide a cleaner interface.
The Bottom Line
Mastering error handling in Java goes beyond catching and throwing exceptions. By utilizing custom exceptions, adhering to best practices, and implementing advanced techniques, you can write code that is robust, understandable, and maintainable.
As you venture further into Java, don't forget to reference the official Java documentation for additional insights on exceptions and error handling.
Remember, the way you handle errors can significantly impact your application's users, making it a vital skill for every Java developer. Embrace the challenge and continue growing your skills in this critical area of software development. Happy coding!