Overcoming Common ZK Framework Performance Issues
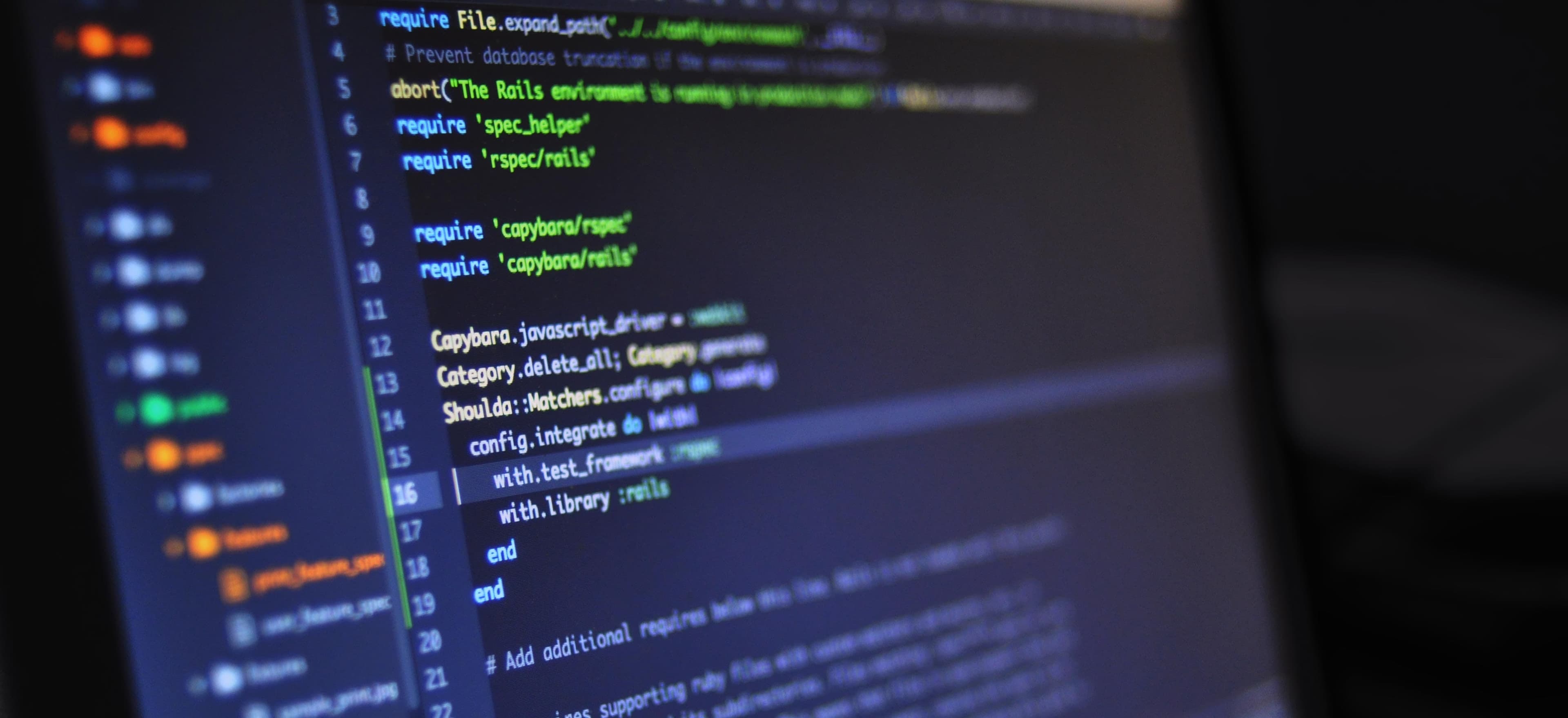
- Published on
Overcoming Common ZK Framework Performance Issues
The ZK Framework is a popular choice for building rich web applications in Java. Known for its simplicity and powerful features, it allows developers to create dynamic and interactive user interfaces with minimal coding. Despite these advantages, ZK applications can encounter performance issues if not optimized properly. In this blog post, we will explore common performance pitfalls in the ZK Framework and how to mitigate them effectively.
Understanding ZK Framework Architecture
To better appreciate how to improve performance, let's briefly understand the architecture of the ZK Framework. ZK follows a server-centric architecture. This means that most of the processing happens on the server side, which then sends the relevant data back to the client. This model allows for rich user experiences without the need for complex JavaScript, but it can lead to bottlenecks if not managed correctly.
Key Components
- ZUL Files: ZK uses XML-like files (ZUL) to define the user interface components.
- Java Backing Beans: These are the server-side components that handle business logic and interact with the UI.
- Request Lifecycle: Each interaction with the UI sends a request to the server, which processes the request and updates the UI accordingly.
By understanding these components, we can identify areas where performance can be enhanced.
Common Performance Issues
1. Overloading the Event Queue
Issue
One of the most common performance issues in ZK applications is an overloaded event queue. This happens when multiple client-side events trigger frequent interactions with the server, leading to a backlog of requests.
Solution
To address this, consider the following best practices:
- Debounce Events: Reduce the frequency of event triggers. For example, when handling input events in a text box, add a delay before sending data back to the server.
@Listen("onChanging = #myTextBox")
public void onTextChanged(Event event) {
String inputValue = ((Textbox) event.getTarget()).getValue();
// Process change with some delay
Executors.newSingleThreadScheduledExecutor()
.schedule(() -> processInput(inputValue), 500, TimeUnit.MILLISECONDS);
}
- Batch Requests: Group multiple requests into a single call to reduce the server load.
2. Unoptimized Data Loading
Issue
Loading large datasets directly can slow down your application. This is common when displaying data in components like grids and lists.
Solution
- Pagination: Always implement pagination to load data in smaller chunks. This prevents overwhelming the UI and makes it more responsive.
@GlobalCommand("loadData")
@NotifyChange("dataList")
public void loadData() {
int pageSize = 20;
int currentPage = pagination.getCurrentPage();
dataList = dataService.getData(currentPage * pageSize, pageSize);
}
- Lazy Loading: This technique allows data to be loaded on-demand. For example, load items as the user scrolls through a list.
3. Inefficient UI Components
Issue
Using too many UI components or complex components can significantly impact performance. Each component involves rendering overhead, and complex hierarchies can slow response times.
Solution
-
Simplify the UI: Minimize the use of unnecessary components. Consolidate similar components into a single component when possible.
-
Optimize Component Lifecycle: Limit the number of stateful components and utilize stateless components when possible.
@Init
public void init() {
// Using a simpler component instead of multiple states
Listbox listBox = new Listbox();
listBox.setModel(new ListModelList<>(getSimpleData()));
}
4. Heavy Use of Bindings
Issue
While data binding is a powerful feature in ZK, overusing it can lead to performance degradation. Each binding requires an update and change notification, which can be resource-intensive.
Solution
- Manual Updates: Consider manual updates for components that don’t require frequent changes, reducing unnecessary binding overhead.
@Listen("onClick = #updateButton")
public void updateDisplay() {
MyModel myModel = getModel();
myComponent.setValue(myModel.getDisplayValue());
}
Optimizing Server Resources
5. Resource Management
Issue
ZK applications can consume substantial server resources if not monitored properly. Memory leaks and excessive CPU usage can result from poorly managed resources.
Solution
-
Profile Resource Usage: Use profiling tools to monitor CPU and memory usage, helping identify bottlenecks.
-
Manage Sessions: Keep track of user sessions and properly terminate idle sessions to free up resources.
@Listen("onTimeout = someTimer")
public void handleTimeout() {
UserSession session = SessionManager.getCurrentSession();
if (session.isIdle()) {
session.invalidate();
}
}
6. Caching Strategies
Issue
Frequent database queries are a major performance bottleneck. Retrieving the same data repeatedly can slow down your application.
Solution
- Implement Caching: Use caching frameworks like EHCache or Hazelcast to store frequently accessed data.
@Cacheable("myCache")
public List<MyModel> getCachedData() {
return databaseQueryService.getData();
}
- Cache UI Components: Components that do not change frequently can be cached to reduce loading times.
The Closing Argument
Overcoming performance issues in ZK Framework applications requires proactive measures and optimization strategies. By understanding the architecture and components, you can effectively address common problems such as event overload, data loading, UI inefficiencies, and server resource management.
Furthermore, consistently profiling your application and adapting these strategies will contribute to a smoother, more responsive user experience. As you implement these practices, remember that performance optimization is an ongoing process that adapts with the growth of your application.
Additional Resources
For further reading on optimizing ZK applications, consider these resources:
- ZK Developer Documentation
- Java Performance Tuning
- Building Scalable Applications in Java
- Improving Application Performance
Incorporating these strategies in your ZK application development process will not only enhance performance but also create a more reliable and user-friendly experience. Happy coding!
Checkout our other articles