Resolving JAX-RS 2.1 Jersey Client Dependency Issues
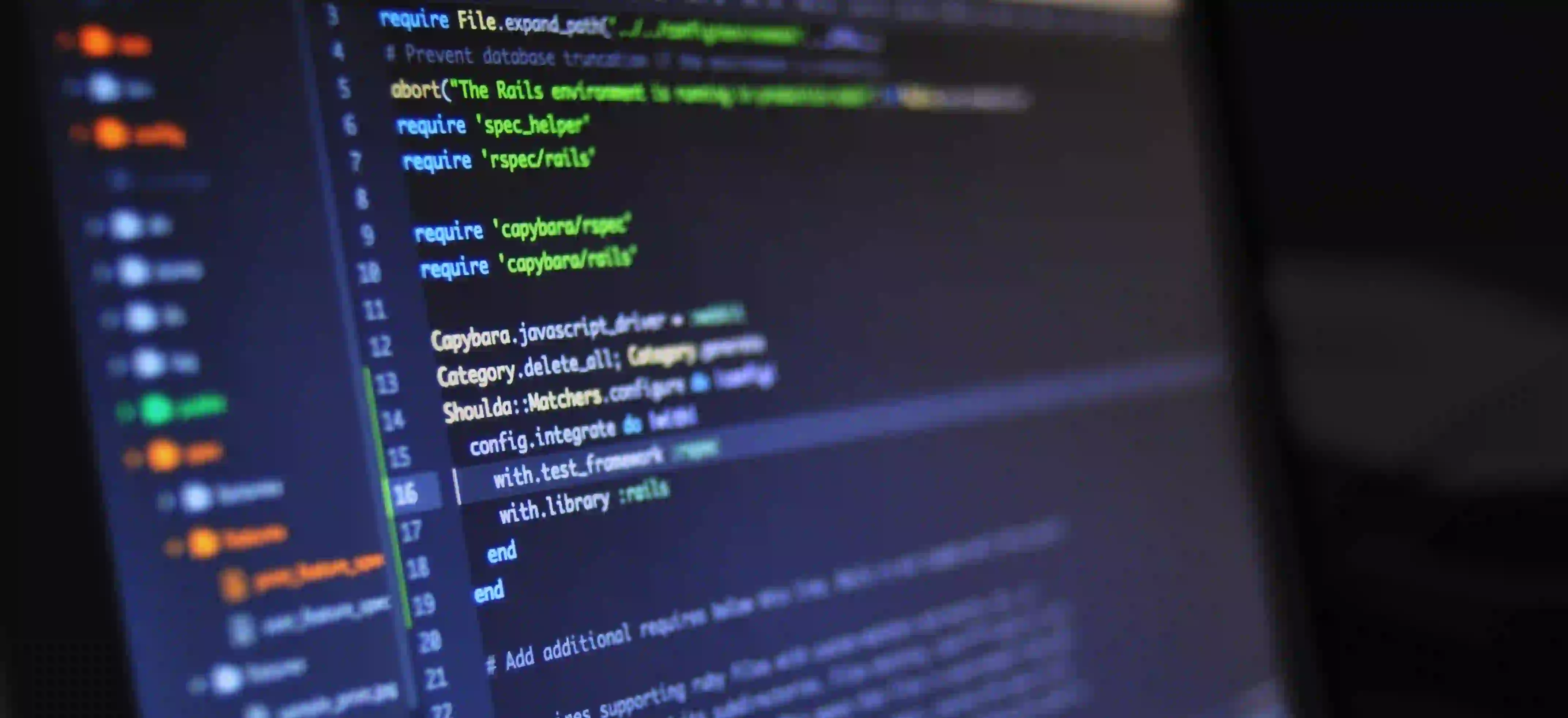
Resolving JAX-RS 2.1 Jersey Client Dependency Issues
In today's microservices architecture, RESTful APIs have become increasingly prominent. The JAX-RS (Java API for RESTful Web Services) specification simplifies the development of RESTful services for Java applications. Among the various implementations of JAX-RS, Jersey stands out as one of the most used. This blog post addresses common dependency issues developers encounter when working with the JAX-RS 2.1 Jersey Client. We will guide you through the necessary configurations, offer solutions to typical problems, and provide code snippets for clarity.
Understanding Jersey and JAX-RS
Jersey is the reference implementation of JAX-RS, making it an ideal choice for building RESTful APIs in Java. JAX-RS 2.1 introduces several enhancements over its predecessors, including client-side request processing and a higher level of abstraction.
Before diving deeper, let's briefly discuss how to include Jersey in your project. This section will provide an example Maven dependency configuration.
Example Maven Dependency Configuration
If you are using Maven, you can start by including the necessary dependencies in your pom.xml
:
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>2.35</version>
</dependency>
Note: Always check for the latest version on Maven Repository.
Common Dependency Issues
1. Version Compatibility
One of the most common issues developers face relates to conflicting or incompatible versions of libraries required by Jersey. For example, if you have other dependencies in your project that rely on an older version of Jersey, issues can arise.
Solution:
- Dependency Tree Analysis: Use the following Maven command to visualize your dependencies and their versions:
mvn dependency:tree
- Exclusions: If you detect a conflict, consider excluding the old version. Here’s how to exclude a transitive dependency:
<dependency>
<groupId>some.group</groupId>
<artifactId>some-artifact</artifactId>
<version>1.0</version>
<exclusions>
<exclusion>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-common</artifactId>
</exclusion>
</exclusions>
</dependency>
This provides a clear way to manage which versions are brought into your project.
2. Class Not Found Issues
Developers often encounter ClassNotFoundException
or NoClassDefFoundError
. These errors indicate that certain classes that Jersey depends on are missing from the classpath.
Solution:
Verify that all required Jersey libraries are included in your classpath. For example, ensure that jersey-server
, jersey-client
, and jersey-media-json-jackson
are included if your application sends or receives JSON data.
3. Dependency Scope
Another aspect that might create confusion is the scope
of your dependencies. If any Jersey library is set to test
or another less accessible scope, it won't be available at runtime.
Solution:
Make sure that the Jersey dependencies have the appropriate scope. By default, the scope should be set to compile
, like so:
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>2.35</version>
<scope>compile</scope>
</dependency>
Building a Simple Jersey Client
Now that we have covered some of the common dependency issues, let's build a simple Jersey Client to demonstrate how to use it effectively.
Client Initialization
To create a client, you can use the ClientBuilder
class. Here’s a basic example:
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
public class JerseyClientExample {
public static void main(String[] args) {
// Build the client
Client client = ClientBuilder.newClient();
// Make a GET request to a sample API
Response response = client.target("https://jsonplaceholder.typicode.com/posts/1")
.request(MediaType.APPLICATION_JSON)
.get();
// Print the response
String jsonResponse = response.readEntity(String.class);
System.out.println("Response: " + jsonResponse);
// Close the client
client.close();
}
}
Why This Code Works:
- Client Initialization: Using
ClientBuilder.newClient()
creates a new instance of the client. - Targeting the API: The
target
method specifies the URL endpoint. - Making Requests: The
request
method specifies the accepted media type, followed by the HTTP method (in this case,get()
). - Reading Response: The response is read as a string, allowing you to output it or manipulate it further.
Enhancing the Client with Error Handling
It's crucial to include error handling in production applications. Here's an enhanced version:
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.Entity;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
public class EnhancedJerseyClient {
public static void main(String[] args) {
Client client = ClientBuilder.newClient();
try {
Response response = client.target("https://jsonplaceholder.typicode.com/posts")
.request(MediaType.APPLICATION_JSON)
.post(Entity.entity("{\"title\":\"foo\",\"body\":\"bar\",\"userId\":1}", MediaType.APPLICATION_JSON));
if (response.statusInfo().getFamily() == Response.Status.Family.SUCCESSFUL) {
String jsonResponse = response.readEntity(String.class);
System.out.println("Response: " + jsonResponse);
} else {
System.err.println("Error: " + response.getStatus() + ": " + response.readEntity(String.class));
}
} finally {
client.close();
}
}
}
Why This Code Works:
- Error Handling: The structure checks the response status before trying to read the response entity.
- POST Request Example: The updated code demonstrates how to perform a POST request while sending JSON data, showing the client's capability to handle various HTTP methods.
My Closing Thoughts on the Matter
Dependency management in Java, especially with Jersey and JAX-RS, can be cumbersome if not properly handled. By ensuring version compatibility, managing scopes, and including all necessary libraries, you can mitigate these common issues. In this post, we explored the structure of a Jersey Client and enhanced its usability through proper error handling.
For more in-depth coverage of JAX-RS, you can refer to the official Jersey Documentation or the JAX-RS Specification. These resources can provide further insights and advanced capabilities in RESTful services.
By remaining vigilant and systematic in your dependency management, you can focus on developing robust and scalable RESTful services without running into roadblocks. Happy coding!