Common Issues When Creating Executable JARs with Maven
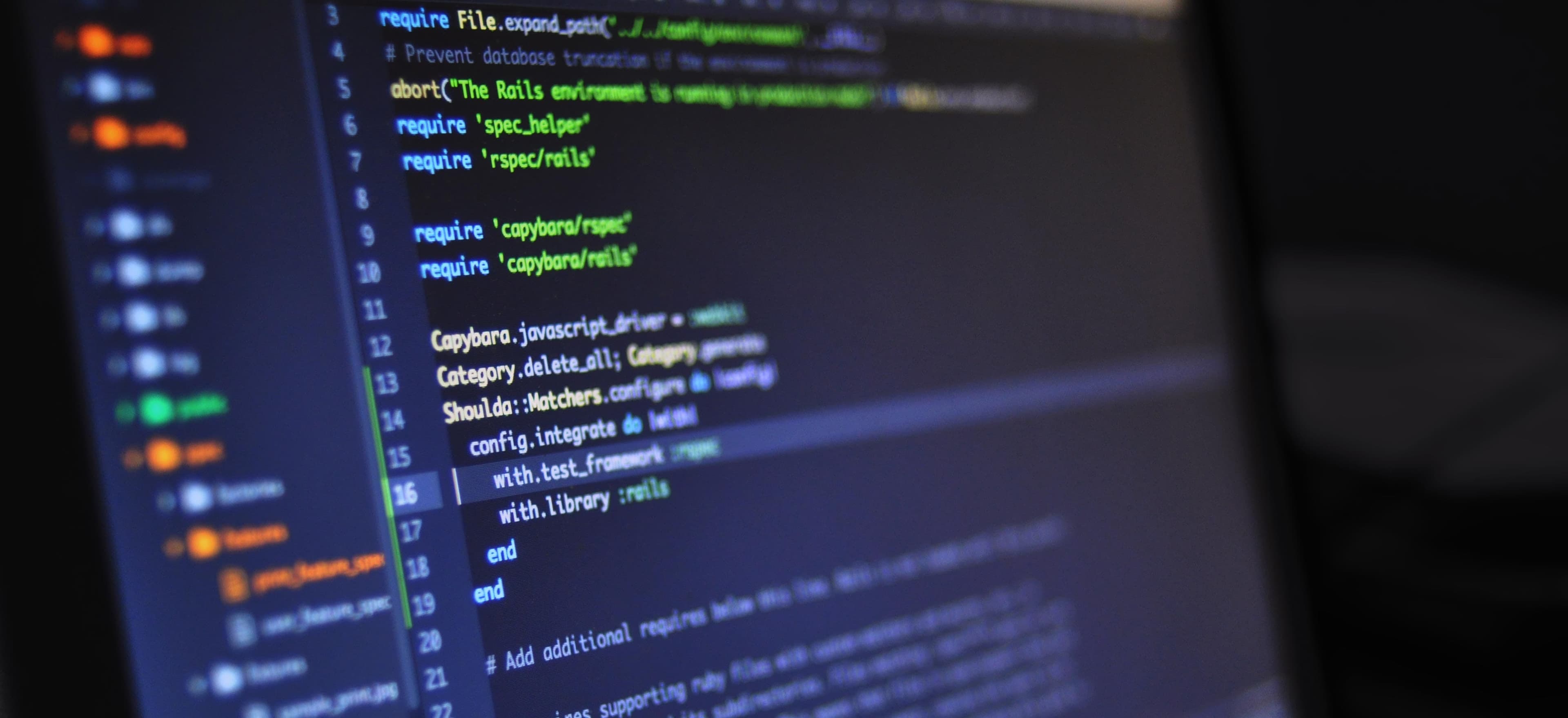
- Published on
Common Issues When Creating Executable JARs with Maven
Creating executable JAR files using Maven can be a straightforward task, but there are numerous pitfalls that developers may encounter during the process. This blog post aims to highlight some of the common issues associated with generating executable JARs, while providing solutions and best practices to solve these problems.
Understanding Executable JARs
An executable JAR file is a packaged Java application that can be run directly using the Java Runtime Environment (JRE). To create an executable JAR in Maven, you typically utilize the "maven-jar-plugin" to specify the main class and configure the manifest. However, there are common stumbling blocks that can trip up developers.
Common Issues
1. Manifest Configuration
One of the primary causes of failure when creating an executable JAR is incorrect manifest configuration. The manifest file must specify the entry point of your application - that is, the main class that contains the public static void main(String[] args)
method.
Common Mistake: Leaving Out the Main-Class Attribute
A frequent mistake is forget to specify the Main-Class
attribute in the manifest file.
Example Code Snippet:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>3.2.0</version>
<configuration>
<archive>
<manifest>
<addDefaultSpecificationEntries>true</addDefaultSpecificationEntries>
<addDefaultImplementationEntries>true</addDefaultImplementationEntries>
<mainClass>com.example.MyMainClass</mainClass>
</manifest>
</archive>
</configuration>
</plugin>
</plugins>
</build>
Why This Matters:
If you don't specify the main class, Java won’t know where to start your application, resulting in an error like no main manifest attribute
. Always ensure your mainClass
is correctly set.
2. Dependency Issues
Another common issue is related to dependencies. When creating an executable JAR, it is important to include all required dependencies, particularly when libraries are needed at runtime. Using just a simple JAR will not bundle dependencies.
Solution: Using the Maven Shade Plugin
To create a "fat" executable JAR that includes all dependencies, you can configure the Maven Shade Plugin.
Example Code Snippet:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
<configuration>
<transformers>
<transformer implementation="org.apache.maven.plugins.shade.resource.ManifestResourceTransformer">
<mainClass>com.example.MyMainClass</mainClass>
</transformer>
</transformers>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
Why This Matters:
Including dependencies allows your JAR to run independently of the Maven environment, thus avoiding ClassNotFoundException
errors when running the JAR.
3. Conflicts in Dependencies
Sometimes your application may fail due to conflicts in dependencies, particularly if two or more libraries require different versions of the same dependency.
Solution: Dependency Management
To manage dependencies effectively, declare dependency versions explicitly and rely on the dependency tree. This can be visualized using the mvn dependency:tree
command.
Example Code Snippet:
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>example-lib</artifactId>
<version>1.0.1</version>
</dependency>
<!-- other dependencies -->
</dependencies>
Why This Matters:
By managing versions properly, you can avoid runtime exceptions caused by version mismatch.
4. Incorrect Packaging Type
An issue that can easily go unnoticed is setting the wrong packaging type in your pom.xml
, such as using jar
but intending to use war
.
Solution: Specify the Correct Packaging Type
Ensure that you've specified the correct packaging type as follows:
<packaging>jar</packaging>
Why This Matters:
If you specify war
, Maven will attempt to create a web application which is unnecessary for a standalone application.
5. Path Issues
Sometimes, even if everything else is set correctly, you might encounter problems due to incorrect path references. This often happens when resources required by the application (like properties files) aren't properly included in the JAR.
Solution: Ensure Resource Inclusion
You can explicitly configure resources within your pom.xml
to ensure they are included in the JAR.
Example Code Snippet:
<build>
<resources>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.properties</include>
</includes>
</resource>
</resources>
</build>
Why This Matters:
Including resources ensures your application runs without missing configuration files or other necessary data.
Testing the Executable JAR
After addressing the aforementioned issues, it’s crucial to perform a test run to ensure that the JAR behaves as expected. You can test your JAR file from the command line with the following command:
java -jar target/my-application.jar
Best Practices for Creating Executable JARs
- Always specify the main class: This avoids the common error of missing the entry point.
- Use the Shade Plugin: Bundling dependencies helps ensure your application can run independently.
- Regularly monitor dependency versions: Address conflicts early in development to avoid issues during packaging.
- Include necessary resources: Ensure application dependencies and resources are captured in your final JAR.
- Test extensively: Always run your JAR post-package to catch runtime issues.
My Closing Thoughts on the Matter
Creating executable JARs with Maven should not be a daunting task. By understanding and navigating these common issues, you can ensure smoother development and deployment processes. Properly managing your build lifecycle with Maven will allow you to focus on building awesome applications instead of being bogged down by errors.
If you want to delve deeper into Maven and its various functionalities, consider checking out the official Maven Documentation. Happy coding!