Boost Your Java Project with AOP Method Logging Magic
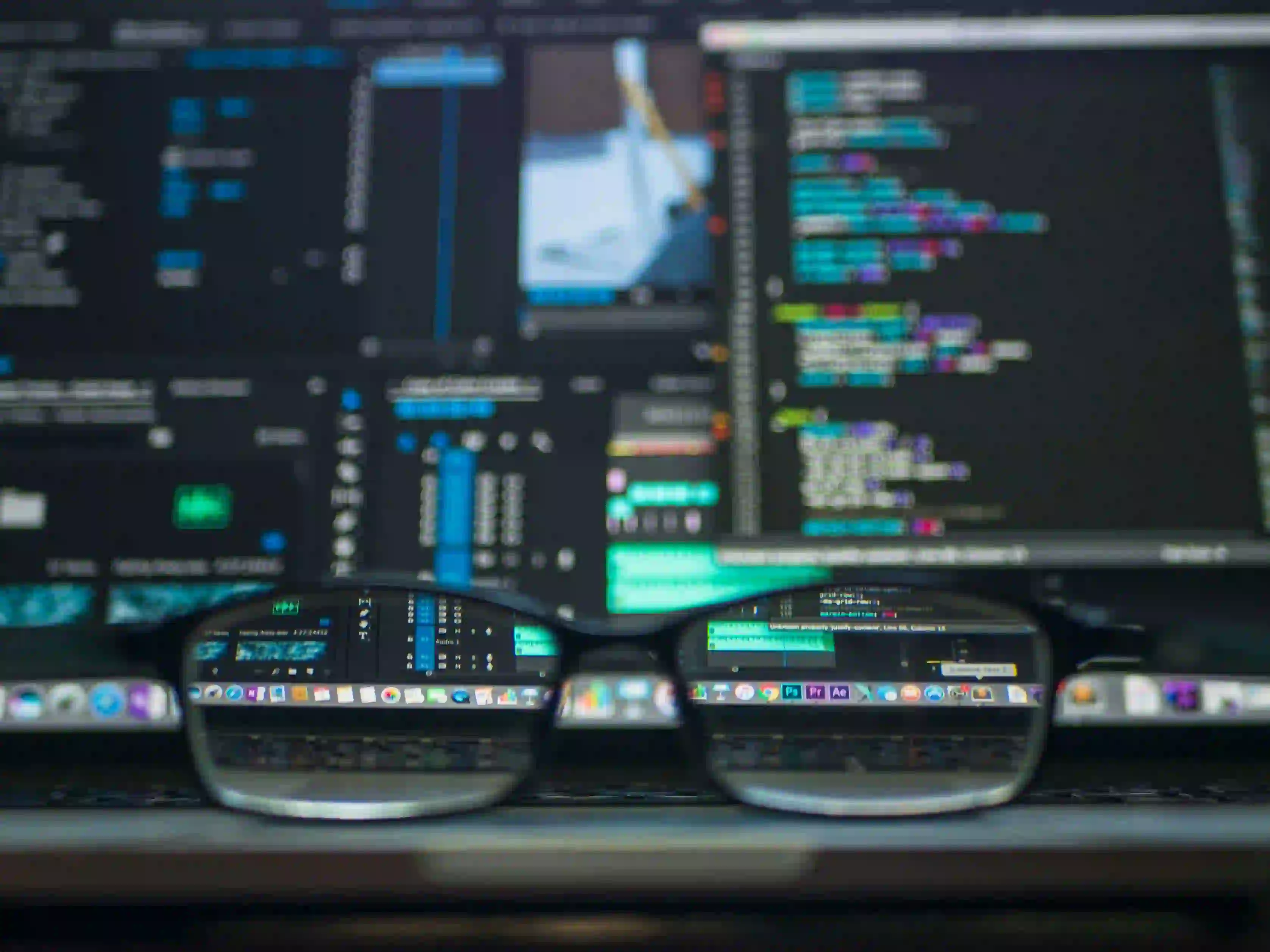
Boost Your Java Project with AOP Method Logging Magic
In today's fast-paced development environment, maintaining code quality and ensuring smooth operation can feel like an overwhelming task. However, robust logging can significantly enhance your ability to monitor and troubleshoot applications. One of the best practices in this arena is Aspect-Oriented Programming (AOP) for method logging. This blog post will explore how to implement AOP method logging in your Java projects, improving your coding efficiency and debugging capabilities.
What is AOP?
Aspect-Oriented Programming (AOP) is a programming paradigm that allows developers to modularize cross-cutting concerns, such as logging, security, or transaction management, separate from the business logic. This modularity improves code readability and maintainability.
For instance, instead of embedding logging code within business methods, you can define logging behavior in a separate aspect. The benefit? Cleaner code, easier maintenance, and better separation of concerns.
Why Use AOP for Method Logging?
-
Separation of Concerns: By isolating logging code from the actual business logic, your application becomes easier to understand and maintain.
-
Reduced Code Duplication: You can apply logging consistently across various methods without rewriting the same code.
-
Dynamic Behavior: You can change logging behavior without modifying the core business logic.
-
Centralized Configuration: Manage all logging aspects in one place, making it easier to update as the application evolves.
Getting Started with AOP in Java
To illustrate AOP method logging, we will use Spring AOP, a powerful and popular framework that supports AOP concepts in Java applications. Follow these steps to implement method logging.
Step 1: Set Up Your Project
If you are starting a new project, you can set up a Maven project easily. Here is a simple pom.xml
configuration:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>aop-logging-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
This configuration sets up a lightweight Spring Boot project with AOP support.
Step 2: Create a Service Class
Hereβs a simple service class where we will apply our logging aspect.
package com.example.service;
import org.springframework.stereotype.Service;
@Service
public class UserService {
public String getUserById(Long userId) {
return "User_" + userId;
}
public void createUser(String username) {
System.out.println("Creating user: " + username);
}
}
Step 3: Create an AOP Aspect for Logging
Now, let's create an aspect that will log method entries and exits. Here's how to do it using Spring AOP:
package com.example.aspect;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
private static final Logger logger = LoggerFactory.getLogger(LoggingAspect.class);
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
logger.info("Entering method: {}", joinPoint.getSignature());
Object[] args = joinPoint.getArgs();
for (Object arg : args) {
logger.info("Arg: {}", arg);
}
}
@After("execution(* com.example.service.*.*(..))")
public void logAfter(JoinPoint joinPoint) {
logger.info("Exiting method: {}", joinPoint.getSignature());
}
}
Code Analysis
-
@Aspect annotation: Marks the class as an AOP aspect.
-
@Before annotation: Indicates that the
logBefore
method runs before the method execution. -
Execution Pointcut: The expression
execution(* com.example.service.*.*(..))
points to any method within theservice
package. -
JoinPoint: Provides reflective access to both the state during the execution and the method being executed. Here, we used
joinPoint.getSignature()
to log method signatures. -
@After annotation: The
logAfter
method is invoked after the corresponding method execution.
Step 4: Configure Logging
To see the logs in action, you may need to configure logging in your application.properties
file:
logging.level.root=INFO
logging.level.com.example=DEBUG
Step 5: Testing the Implementation
You can now write a simple test to verify that logging is functioning correctly.
package com.example;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class AopLoggingExampleApplication {
public static void main(String[] args) {
SpringApplication.run(AopLoggingExampleApplication.class, args);
}
@Bean
CommandLineRunner run(UserService userService) {
return args -> {
userService.createUser("JohnDoe");
userService.getUserById(1L);
};
}
}
Final Thoughts
Implementing AOP logging in your Java application can streamline your debugging processes and improve the maintainability of your code base. This AOP setup with Spring allows you to monitor method execution without cluttering your core logic. By utilizing SLF4J and logging aspects, you gain invaluable insights into how your applications behave at runtime.
For more on AOP in Java using Spring, consider checking out the official Spring AOP documentation.
Whether you're a seasoned Java developer or just starting out, understanding and implementing AOP logging can significantly enhance your coding practices, contributing to a cleaner and more efficient development process.
Happy coding!