Common API Design Pitfalls with Eclipse Collections
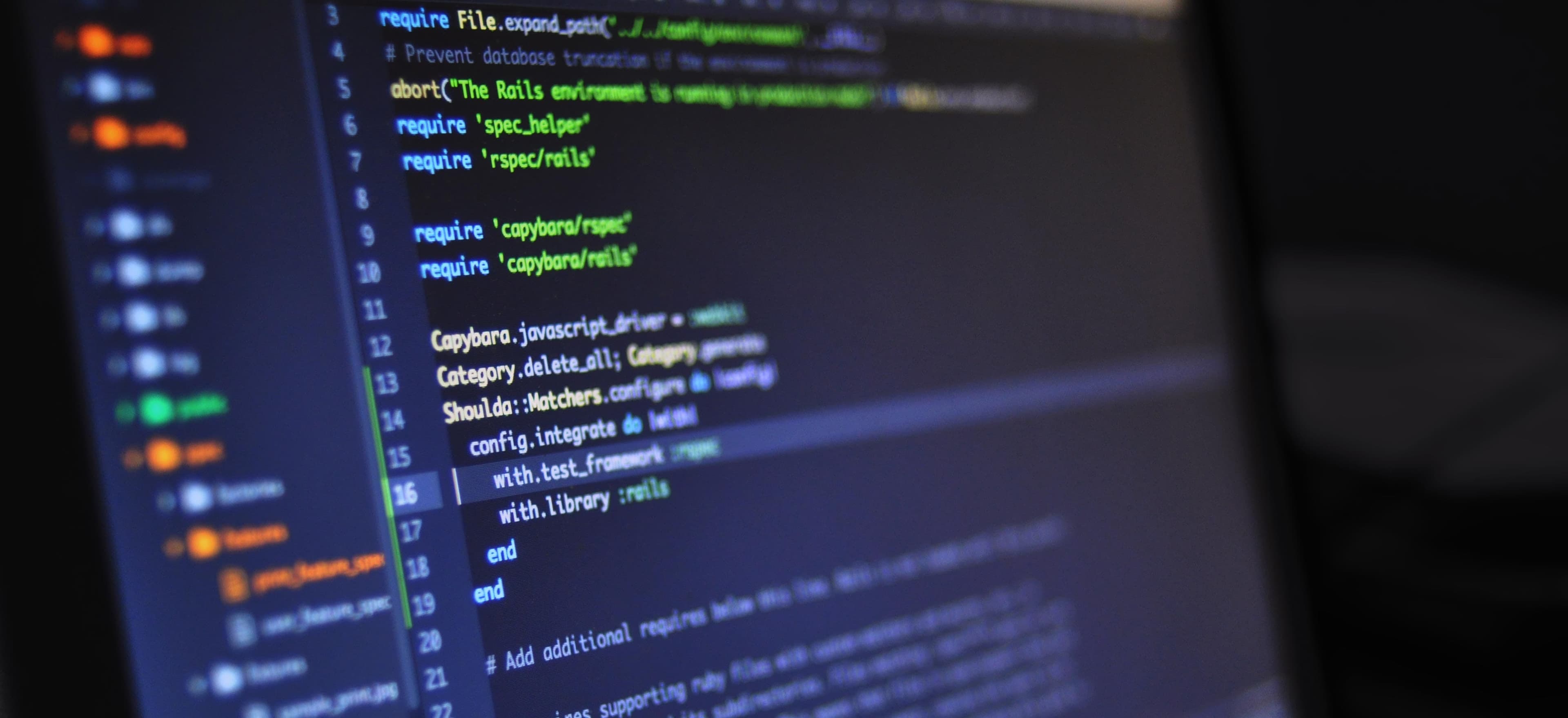
- Published on
Common API Design Pitfalls with Eclipse Collections
When designing APIs, particularly in the context of collections, developers often stumble into common pitfalls that can lead to ill-structured and hard-to-use interfaces. Eclipse Collections is a powerful Java collections framework that enhances the standard Java collections APIs. However, even with its advantages, there are mistakes that can arise when designing APIs with it.
In this blog post, we will explore these pitfalls, their implications, and strategies to avoid them. We will also provide code snippets using Eclipse Collections to illustrate best practices.
1. Inconsistent Naming Conventions
Consistency is key in API design. When you mix different naming conventions, it can confuse users. Naming should clearly reflect the intent of the method or variable.
Example of Inconsistent Naming
MutableList<String> items = Lists.mutable.empty();
items.add("Apple");
items.add("Banana");
// Poor naming convention
MutableList<String> fruitList = Lists.mutable.empty(); // Inconsistent in terms of purpose
fruitList.add("Cherry");
In the above example, the developer uses items
and fruitList
for lists that hold similar content types. A better approach is to maintain a uniform naming convention:
Improved Consistency
MutableList<String> fruits = Lists.mutable.empty();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
Why Consistency Matters
Consistency in naming helps maintain clarity in your codebase. When users see a consistent naming scheme, they can quickly understand the purpose of each variable or method, speeding up the learning curve.
2. Lack of Documentation
Another significant pitfall is the absence of documentation. Even the best API can become a chore to use if users are left to guess how to implement it. Documentation is essential for clear explanations.
Example of Poor Documentation
public MutableList<Integer> calculateValues() {
// This method calculates values based on some logic
MutableList<Integer> results = Lists.mutable.empty();
// Logic...
return results;
}
Improved Documentation
/**
* Calculates the values based on predefined logic.
*
* @return A mutable list of calculated integer values.
*/
public MutableList<Integer> calculateValues() {
MutableList<Integer> results = Lists.mutable.empty();
// Logic...
return results;
}
Why Documentation is Crucial
Well-documented methods and classes aid in maintainability. They allow both current and future developers to understand and use the API correctly, reducing the time spent on deciphering the code.
3. Not Utilizing Fluent Interfaces
Eclipse Collections provides a set of methods enabling fluent programming, which allows method chaining. When APIs do not leverage this capability, they can become cumbersome for users.
Example Without Fluent Interface
MutableList<String> list = Lists.mutable.empty();
list.add("One");
list.add("Two");
list.add("Three");
for (String s : list) {
System.out.println(s);
}
Enhanced Example Using Fluent Interface
Lists.mutable.empty()
.add("One")
.add("Two")
.add("Three")
.each(System.out::println);
Why Fluent Interfaces are Beneficial
Fluent interfaces enhance readability and reduce boilerplate code, making the code cleaner and more expressive. Developers can quickly understand the flow of data and operations, leading to better maintainability.
4. Failing to Handle Null Values
Null value handling is often overlooked, which can lead to NullPointerExceptions. Java and Eclipse Collections provide ways to manage optional data effectively.
Example With Potential NullPointerException
public MutableList<String> getStrings(MutableList<String> input) {
MutableList<String> output = Lists.mutable.empty();
for (String s : input) {
output.add(s.toUpperCase()); // Potential NPE if `s` is null
}
return output;
}
Improved Handling of Null Values
public MutableList<String> getStrings(MutableList<String> input) {
MutableList<String> output = Lists.mutable.empty();
for (String s : input) {
if (s != null) {
output.add(s.toUpperCase());
}
}
return output;
}
Why Null Handling is Important
Proper handling of null values not only prevents runtime exceptions but also ensures that the API behaves predictably. Adopting null-safe practices leads to more robust and reliable code.
5. Overcomplicating the API
Complex APIs can deter users. Striving for simplicity should guide your design decisions. An API with too many options can overwhelm users and lead to misuse.
Example of an Overcomplicated API
public MutableList<String> findAndTransform(MutableList<String> input, boolean toUpperCase, boolean trimSpaces) {
MutableList<String> output = Lists.mutable.empty();
for (String s : input) {
if (s != null) {
String transformed = s;
if (toUpperCase) {
transformed = transformed.toUpperCase();
}
if (trimSpaces) {
transformed = transformed.trim();
}
output.add(transformed);
}
}
return output;
}
Simplified API Design
public MutableList<String> transformStrings(MutableList<String> input, Function<String, String> transformation) {
MutableList<String> output = Lists.mutable.empty();
for (String s : input) {
if (s != null) {
output.add(transformation.apply(s));
}
}
return output;
}
Why Simplicity is Key
A simpler API reduces the learning curve for new users. By providing fewer parameters and allowing transformations via functional interfaces, developers can adopt the API with ease.
Closing the Chapter
Designing an effective API, especially one that utilizes frameworks like Eclipse Collections, requires careful consideration of various factors. By avoiding pitfalls like inconsistent naming, lack of documentation, neglecting fluent interfaces, mishandling nulls, and overcomplicating the design, you can create a robust and user-friendly API.
For more in-depth guidance, consider checking Eclipse Collections' official documentation and review best practices regularly. Embrace these principles to enhance your API design and provide a better experience for your users.
Additional Resources
- API Design Guidelines
- Best Practices for Designing Java APIs
By following these strategies, you can avoid common pitfalls and create a clean, efficient, and user-friendly API that leverages the full power of Eclipse Collections.