Common Pitfalls of Inner Class Field Access in Java
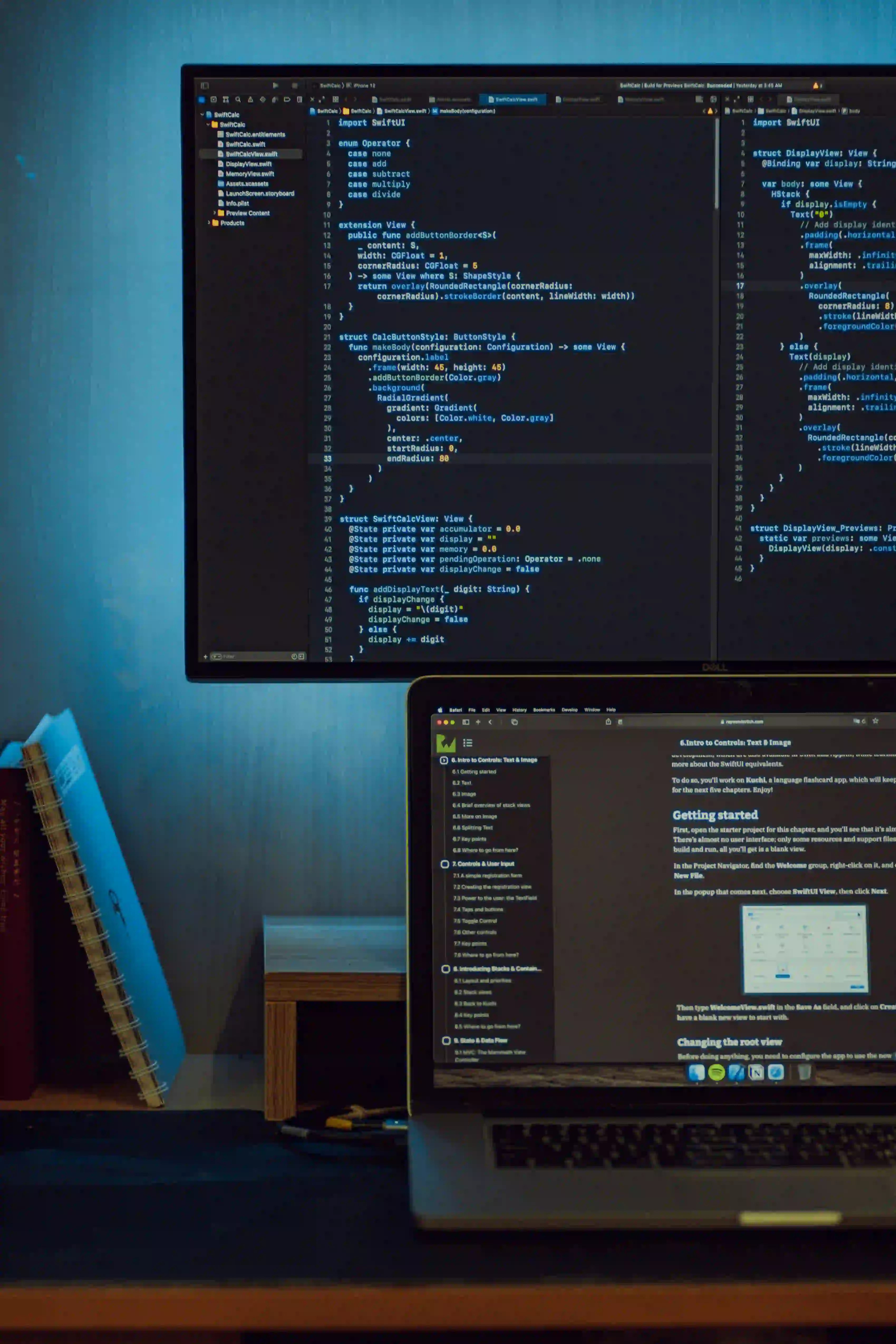
Common Pitfalls of Inner Class Field Access in Java
Java is a powerful, object-oriented programming language that promotes clean, maintainable code. However, it's not without its intricacies. One notable aspect of Java is its support for inner classes, which can lead to certain pitfalls, especially concerning field access. Understanding these pitfalls is crucial for Java developers looking to write robust and error-free code.
In this blog post, we will explore common mistakes made with inner class field access, discuss how they can lead to bugs, and illustrate best practices through code snippets.
What are Inner Classes?
Before diving into potential pitfalls, let's first clarify what inner classes are. An inner class is defined within the scope of another class and has access to the enclosing class's members, including private ones. Here's a simple example:
class Outer {
private int outerField = 10;
class Inner {
void display() {
System.out.println("Outer field: " + outerField);
}
}
}
Why Use Inner Classes?
Inner classes come in handy when you want to logically group classes that are only used in one place. They also enable more readable and maintainable code, particularly when the inner class interacts closely with the outer class.
Common Pitfalls with Inner Class Field Access
1. Implicit Reference to the Enclosing Instance
One common pitfall when working with inner classes is forgetting that they maintain an implicit reference to the enclosing instance. This can lead to unintended memory retention.
Example:
class Outer {
private String outerField = "Outer field";
class Inner {
void display() {
System.out.println(outerField);
}
}
}
// Usage
Outer outer = new Outer();
Outer.Inner inner = outer.new Inner();
inner.display();
In this situation, an instance of Inner
keeps a reference to the Outer
instance, which could potentially lead to memory leaks if not managed properly. If you create many inner class instances without releasing their outer instances, you may inadvertently retain more memory than necessary.
2. Accessing Outer Class Variables Using this
When accessing outer class fields from an inner class, Java provides the this
keyword as a reference to the inner class instance, not the outer class. A common mistake is confusing these references.
Example:
class Outer {
private String outerField = "Outer field";
class Inner {
void display() {
System.out.println("Inner this: " + this.outerField); // Compilation Error
}
}
}
This code will produce a compilation error because this.outerField
does not refer to the outerField
of the enclosing class but rather expects outerField
to exist in the Inner
class. To access the outer field, you should explicitly refer to it as:
System.out.println("Outer field: " + Outer.this.outerField);
3. Modifying Outer Class Fields
Modifying outer class fields from an inner class is straightforward, but practitioners should be cautious of potential side effects on encapsulation and predictability.
Example:
class Outer {
private int count = 0;
class Inner {
void increment() {
count++;
System.out.println("Count: " + count);
}
}
}
When the increment
method is called, you might expect that count
is only modified through this inner class. However, if count
is accessed or modified elsewhere in the Outer
class or from another class, it could lead to unpredictable behavior. Always ensure that state changes are well documented and understood.
4. Static Nested Classes and Memory Consumption
Static nested classes are a specialized form of inner classes that do not automatically hold a reference to their outer class. This can be beneficial for memory management. However, developers often misapply them, confusing their behavior with ordinary inner classes.
Example:
class Outer {
private static String staticOuterField = "Static Outer field";
static class StaticInner {
void display() {
System.out.println(staticOuterField);
}
}
}
// Usage
Outer.StaticInner staticInner = new Outer.StaticInner();
staticInner.display(); // This works because StaticInner is a static nested class.
Static nested classes can be used without the need for an instance of the outer class. However, they cannot access non-static fields or methods of the outer class.
5. Thread Safety Issues
When inner classes are accessed by multiple threads, particularly when they modify shared fields of the outer class, this can lead to concurrency problems.
Example:
class Outer {
private int sharedCounter = 0;
class Inner implements Runnable {
public void run() {
for (int i = 0; i < 1000; i++) {
sharedCounter++; // Not thread-safe
}
}
}
}
// Usage
Outer outer = new Outer();
Thread thread1 = new Thread(outer.new Inner());
Thread thread2 = new Thread(outer.new Inner());
thread1.start();
thread2.start();
In this case, sharedCounter
is not thread-safe, which means that the final value can vary depending on the timing of the threads' execution. To protect shared resources, consider using synchronization mechanisms:
synchronized (Outer.this) {
sharedCounter++;
}
Best Practices for Inner Class Field Access
To avoid pitfalls, here are some best practices when working with inner classes in Java:
-
Be Aware of Implicit References: Understand that inner classes hold a reference to the outer class and plan memory management accordingly.
-
Use the
OuterClassName.this
Syntax: Always useOuterClassName.this.fieldName
when you need to access outer class members. -
Limit State Changes: Be prudent about modifying outer class fields from an inner class. Make sure such changes are necessary and are well-communicated.
-
Use Static Nested Classes When Appropriate: If you don’t need access to the outer class’s instance members, consider using static nested classes.
-
Implement Thread Safety: When accessing shared resources, use appropriate synchronization mechanisms to avoid concurrency issues.
Final Considerations
Inner classes can be a boon for Java developers if used correctly, but they come with their own set of challenges. By understanding these common pitfalls with inner class field access and adhering to best practices, developers can write more efficient and error-free Java code.
If you want to learn more about inner classes, be sure to check out the Java Tutorials on the official Oracle website for comprehensive insights.
By remaining aware and cautious, you can take full advantage of inner classes while avoiding the common traps that can hinder your Java programming experience. Happy coding!