Optimizing Cache Performance with Caffeine in Spring Boot
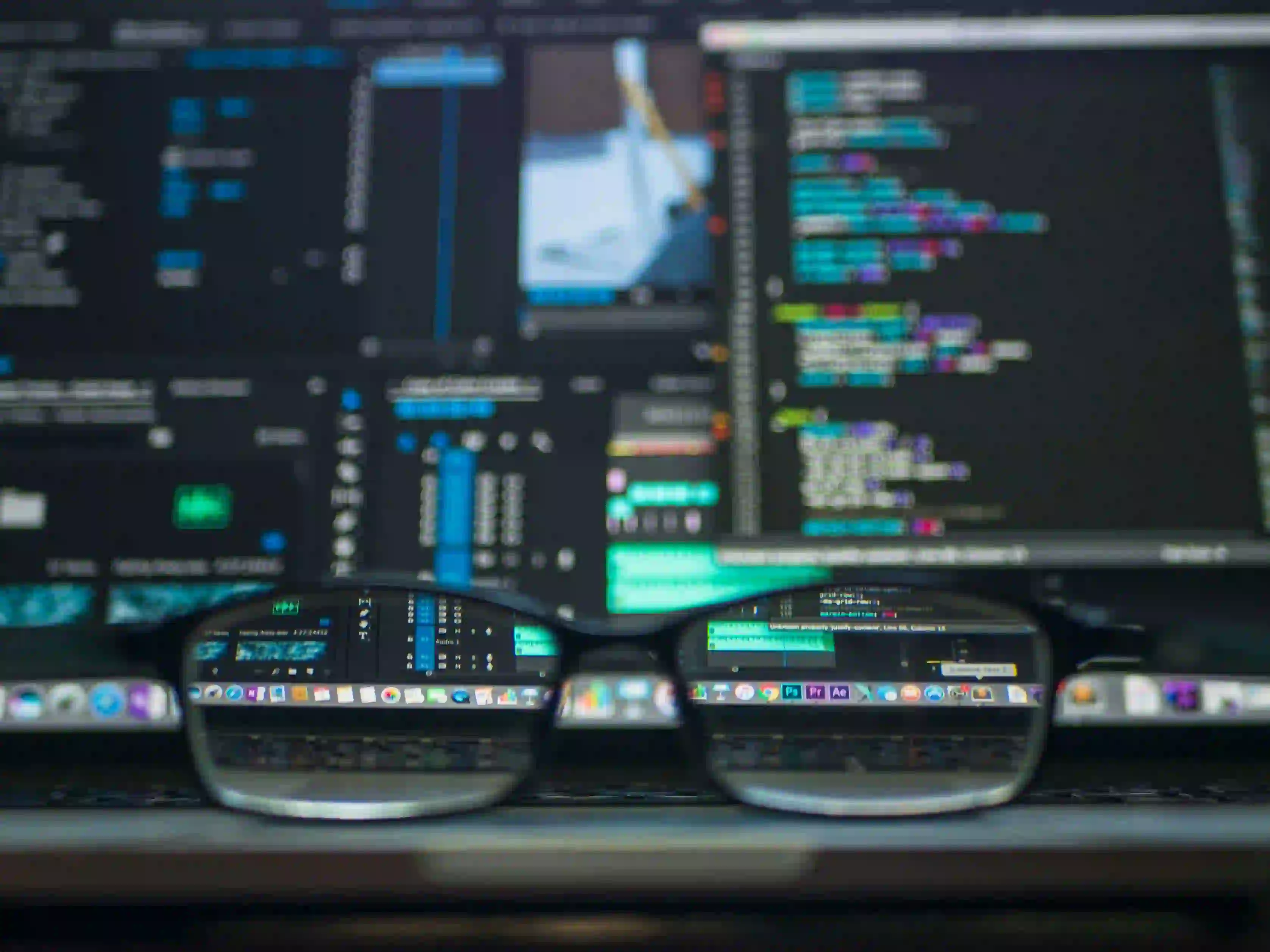
Optimizing Cache Performance with Caffeine in Spring Boot
Caching is a powerful technique used in software development to enhance performance and efficiency. When data is requested frequently, it can be time-consuming to fetch this data repeatedly from the underlying data source. Caffeine, a high-performance caching library for Java, shines in this domain by offering automatic loading, eviction policies, and outstanding throughput. In this blog post, we will explore how to integrate Caffeine caching into your Spring Boot application for optimum performance.
What is Caffeine?
Caffeine is an in-memory cache library that is designed for speed and efficiency. It provides several features, including:
- Automatic Loading: Caffeine can fetch data automatically when it's not present in the cache.
- Eviction Policies: You can configure policies based on size, time, and weight.
- Concurrency: Designed for multi-threaded environments, ensuring high throughput even under load.
For more information, visit the Caffeine GitHub repository.
Benefits of Using Caffeine in Spring Boot
- Speed: Caffeine is built for low-latency access and high concurrency.
- Ease of Use: With Spring Boot, you can integrate Caffeine quickly and efficiently.
- Flexibility: Caffeine provides different cache configurations that can be customized based on your application's needs.
Setting Up Caffeine with Spring Boot
Let’s go through the steps to integrate Caffeine into a Spring Boot application.
Step 1: Add Dependencies
To use Caffeine in your Spring Boot application, add the following dependency to your pom.xml
file if you are using Maven:
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>3.0.5</version> <!-- Check for the latest version -->
</dependency>
If you are using Gradle, add the following line to your build.gradle
:
implementation 'com.github.ben-manes.caffeine:caffeine:3.0.5' // Check for the latest version
Step 2: Configure Caffeine Cache
Next, you should configure the Caffeine cache in your Spring Boot application. Create a configuration class as follows:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import com.github.benmanes.caffeine.cache.Caffeine;
import org.springframework.cache.CacheManager;
import org.springframework.cache.caffeine.CaffeineCacheManager;
import java.util.concurrent.TimeUnit;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager();
cacheManager.setCaffeine(Caffeine.newBuilder()
.initialCapacity(100) // Initial capacity of the cache
.maximumSize(500) // Max size of the cache, can be set as per requirement
.expireAfterWrite(10, TimeUnit.MINUTES)); // Expire entries after a given duration
return cacheManager;
}
}
Explanation of the Cache Configuration
- initialCapacity: The initial amount of space allocated for the cache. This helps in optimizing memory usage at startup.
- maximumSize: The maximum number of entries the cache can hold. Once this limit is reached, the least-recently-used entries will be evicted.
- expireAfterWrite: After a specified duration, entries will be automatically removed from the cache. This is useful for ensuring that stale data doesn’t linger.
Step 3: Using Caffeine Cache in Your Services
Once you've set up the cache configuration, you can use it in your application services. Here is an example service that utilizes caching to optimize performance:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
// Simulates a database call
private User getUserFromDatabase(String userId) {
// Simulate delay
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return new User(userId, "John Doe");
}
@Cacheable("users") // Cache with the name 'users'
public User getUser(String userId) {
return getUserFromDatabase(userId);
}
}
How Caching Works Here
- The @Cacheable annotation indicates that the result of calling
getUser
should be cached. When the method is called with the sameuserId
, the cached result is returned instead of making a time-consuming database call. - For the first call to
getUser("123")
, the method will execute, taking about two seconds. However, subsequent calls togetUser("123")
will return almost instantly, significantly improving performance.
Step 4: Testing the Caching Behavior
To observe caching in action, you can create a simple REST controller that utilizes the UserService
.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/user/{userId}")
public User getUser(@PathVariable String userId) {
return userService.getUser(userId);
}
}
Testing with Postman or cURL
- Make a GET request to
/user/123
to test the caching functionality. You should experience a delay of about 2 seconds. - Make the same request again, and you should see that the response time is significantly quicker, indicating that the result was fetched from the cache.
Step 5: Customizing the Caffeine Cache
Caffeine also allows for more complex configurations, such as using custom eviction policies or loading data asynchronously. Here’s an example of how to create a cache that loads data on-demand:
@Cacheable(value = "users", key = "#userId", cacheManager = "cacheManager")
public User getUser(String userId) {
return getUserFromDatabase(userId);
}
For asynchronous loading, consider using CacheLoader
to load data in a more complex scenario. For detailed customizations, refer to the Caffeine documentation.
To Wrap Things Up
By integrating Caffeine caching into your Spring Boot application, you can significantly improve response times and reduce the load on your data sources. We explored the benefits of caching, how to set it up, and practical examples to implement it effectively.
For further exploration, consider looking into:
- Other cache eviction strategies
- Monitoring cache performance
- Cypress testing for cache behavior
Following the outlined steps will give you a robust caching mechanism in your Spring Boot applications that enhances performance and scales effectively. Happy coding!