Common Pitfalls When Using JavaParser in Your Projects
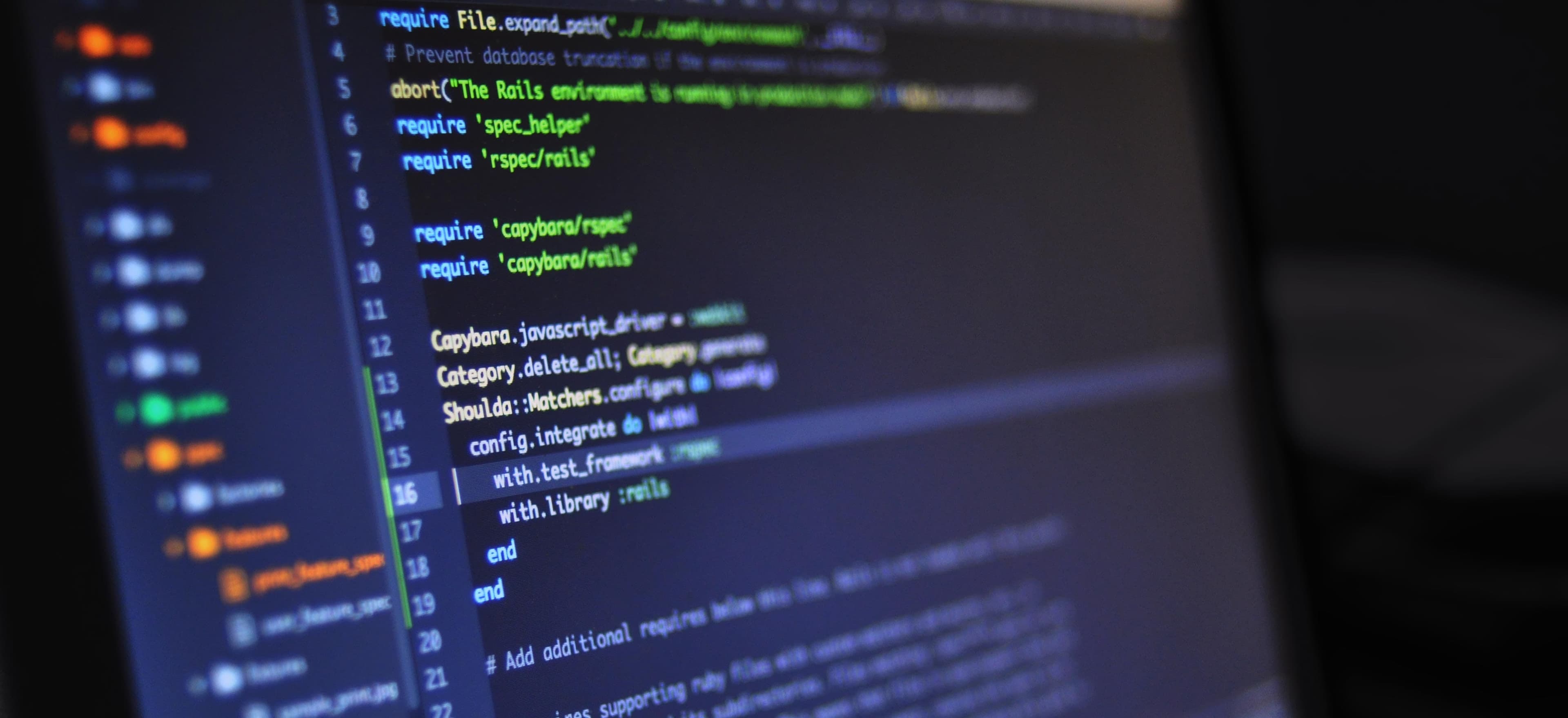
- Published on
Common Pitfalls When Using JavaParser in Your Projects
JavaParser is a popular library that provides a rich API for parsing, analyzing, and transforming Java source code into an Abstract Syntax Tree (AST). Its capabilities are invaluable for projects involving code analysis, automated refactoring, or even simple syntax checking. However, as with any powerful tool, users may encounter pitfalls that can lead to frustration or inefficient code. In this blog post, we will discuss some common issues developers face while using JavaParser and how to avoid them.
The Roadmap to JavaParser
Before we delve into the specifics, let’s briefly discuss what JavaParser is and its main functionalities. JavaParser allows you to:
- Parse Java source files into an AST.
- Navigate the tree to analyze or modify the code.
- Generate new source code from the manipulated AST.
This library can be particularly useful in projects that require understanding or transforming code dynamically. For an introduction and documentation, you can check out the JavaParser GitHub repository.
Common Pitfalls
1. Incomplete Parsing of Java Code
One of the familiar issues with JavaParser is incomplete parsing, especially with complex Java code that includes generics or annotations.
Example Issue: When parsing Java code that includes nested generics, JavaParser might throw exceptions or leave your AST incomplete.
Why This Happens: JavaParser has its limitations, and some Java syntax constructs may not be fully supported, particularly in edge cases.
Solution: Always ensure that the version of JavaParser you are using supports the Java features you plan to analyze. Regularly check for updates or consult the JavaParser documentation for the latest features.
import com.github.javaparser.JavaParser;
import com.github.javaparser.ast.CompilationUnit;
public class ParseExample {
public static void main(String[] args) {
String code = "class Test<T> { T value; }";
CompilationUnit cu = JavaParser.parse(code); // Parses the code
System.out.println(cu.toString());
}
}
2. Modifying AST Without Understanding Its Structure
Many developers make the mistake of modifying the Abstract Syntax Tree without having a solid understanding of how the tree is structured. Misunderstanding the relationships within the tree can lead to logical errors.
Solution: Before making modifications, consider traversing the tree and printing out its structure. This will give you clarity on how different nodes relate.
import com.github.javaparser.ast.visitor.VoidVisitorAdapter;
public class ASTTraversal {
public static void main(String[] args) {
String code = "class Sample { void method() {} }";
CompilationUnit cu = JavaParser.parse(code);
cu.accept(new VoidVisitorAdapter<Void>() {
@Override
public void visit(ClassOrInterfaceDeclaration n, Void arg) {
System.out.println("Class Name: " + n.getName());
super.visit(n, arg);
}
}, null);
}
}
3. Neglecting Error Handling
Failing to implement proper error handling when parsing and analyzing code can lead to crashes or undefined behaviors in your application. This is often overlooked.
Example: You might ignore checking if the code is well-formed which can lead to runtime exceptions.
Solution: Incorporate try-catch blocks around your parsing logic to gracefully handle errors.
public static void main(String[] args) {
String malformedCode = "class Sample { void method( { }"; // Missing parentheses
try {
CompilationUnit cu = JavaParser.parse(malformedCode);
} catch (ParseProblemException e) {
System.err.println("Parsing failed: " + e.getMessage());
}
}
4. Assuming AST Will Be Static
Once the AST is generated, assuming that it will remain consistent across manipulations can lead to incorrect assumptions. JavaParser's AST is mutable, meaning changes will affect the structure.
Example Issue: Adding or removing nodes can alter the positions of other nodes, leading to unexpected results during subsequent analysis.
Solution: Consider cloning your AST if you plan to perform multiple transformations.
CompilationUnit original = JavaParser.parse("class Test { }");
CompilationUnit clone = original.clone(); // Clone original AST
// Perform mutations on the clone without affecting the original
5. Overusing Visitor Pattern
While the Visitor pattern offered by JavaParser allows excellent separation of concerns, over-reliance on it can lead to complex and ramified code structures. A simple walk-through can often suffice for straightforward problems.
Solution: Use the Visitor pattern where necessary but consider simpler alternatives like Lambda expressions for minor traversals.
cu.findAll(MethodDeclaration.class).forEach(method -> {
System.out.println("Method: " + method.getName());
});
6. Not Taking Advantage of Existing Features
JavaParser provides a wealth of features that facilitate common tasks, such as AST modifications, pretty-printing, and even code generation. Ignoring these built-in utilities often results in redundant code.
Example: Instead of manually creating nodes for a new method, take advantage of the provided fluent API.
Code Snippet:
MethodDeclaration method = new MethodDeclaration()
.setName("newMethod")
.setBody(new BlockStmt().addStatement("System.out.println(\"Hello, World!\");"));
cu.getClassByName("Sample").ifPresent(c -> c.addMember(method));
7. Inadequate Testing of Generated Code
After manipulating AST and generating new code, inadequate testing may lead to undetected issues in the resulting Java source code. It is essential to verify if the modified code compiles and functions as expected.
Solution: Use unit tests that compile and run the generated code while checking for logical consistency.
A Final Look
JavaParser is an exceptionally powerful tool that can greatly enhance your Java projects. However, being aware of common pitfalls can save you time and frustration. To seamlessly integrate JavaParser into your projects, maintain a clear understanding of its structure, handle errors gracefully, and take advantage of the library's full capabilities.
Remember, with great power comes great responsibility. Harness it wisely and always test your changes thoroughly.
For further reading on JavaParser, explore its documentation and feel free to reach out with any questions or comments you might have.
References
By adhering to the tips and tricks discussed in this article, you'll be well on your way to mastering JavaParser and utilizing it effectively in your projects. Happy coding!
Checkout our other articles