Common Hibernate 4 Schema Creation Issues and Fixes
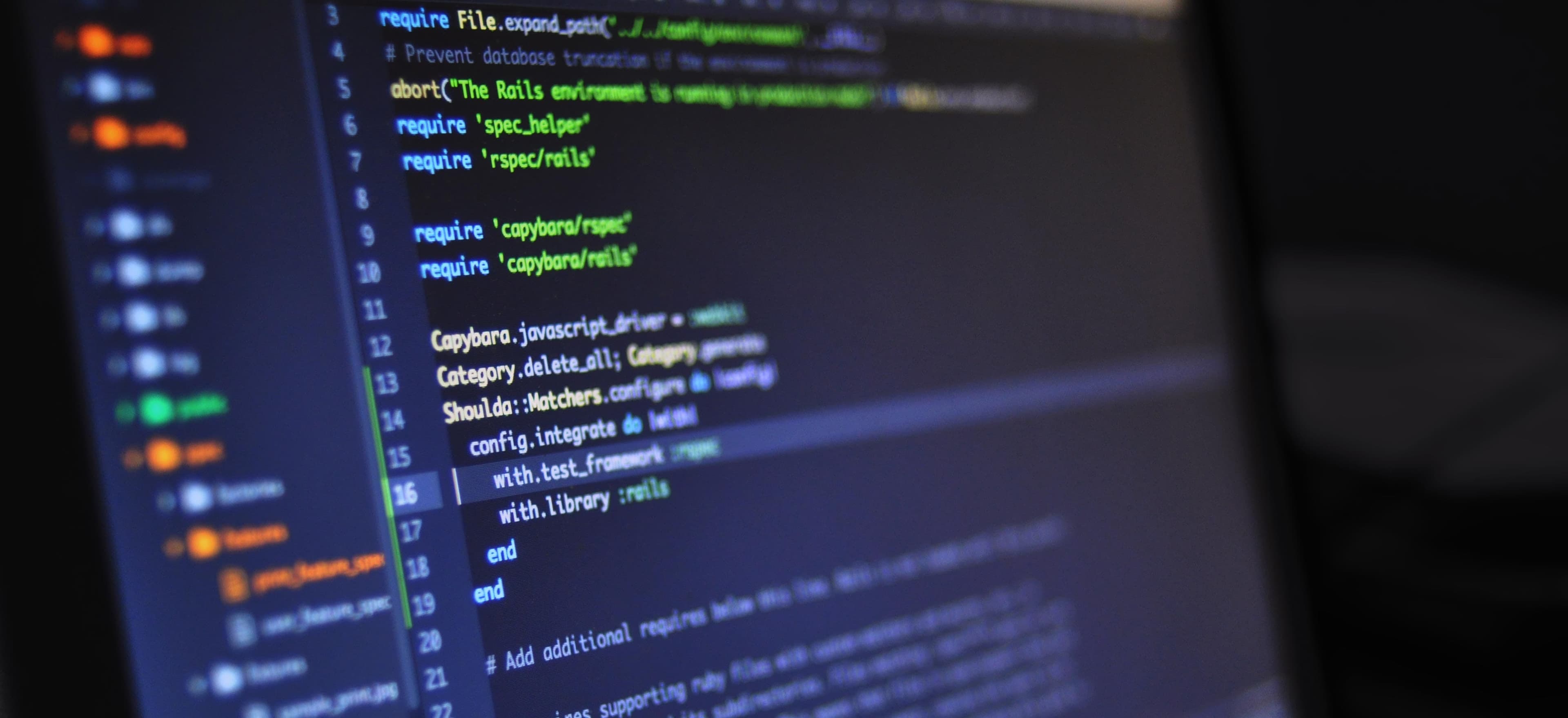
- Published on
Common Hibernate 4 Schema Creation Issues and Fixes
Hibernate is a powerful Object-Relational Mapping (ORM) tool for Java applications. However, while using Hibernate for schema creation, developers often encounter various issues that can hinder productivity and lead to frustration. This post will cover some of the most common Hibernate 4 schema creation issues and their associated fixes, providing you with practical insights to enhance your development experience.
Understanding Hibernate Schema Creation
Hibernate can automatically generate database schemas from your entity classes. This feature is particularly useful during development, as it allows for rapid iteration and testing of database structures. To leverage this feature, you typically set the hbm2ddl.auto
property in your configuration file. Here are its common settings:
create
: Drops the existing schema and creates a new one each time the application starts.update
: Updates the schema based on the current state of the entity classes.validate
: Validates the schema without making any changes.none
: Does nothing regarding the schema generation.
Common Issues in Hibernate 4 Schema Creation
1. NoSchemaFoundException
Issue:
One of the most common issues developers face is the NoSchemaFoundException
, which indicates that Hibernate cannot find the specified schema for the database. This usually happens when the schema is not created before running the application.
Fix:
Make sure the specified schema exists in the database before performing any operations. You can also set the hibernate.hbm2ddl.auto
property to create
during development. Here's how you can configure it in your hibernate.cfg.xml
:
<property name="hibernate.hbm2ddl.auto">create</property>
2. Misconfigured Dialect
Issue:
Another frequent issue arises from a misconfigured dialect. The dialect informs Hibernate of the SQL dialect specific to your database. If the dialect is incorrect, schema generation will fail.
Fix:
Make sure to use the correct dialect for your database. For example, if you're using MySQL, your configuration should look like this:
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
Refer to the official Hibernate documentation for a list of supported dialects.
3. Entity Class Annotations
Issue:
Improperly annotated entity classes can also cause schema creation issues. If the mappings between your entities and database tables are not set up correctly, Hibernate will fail to generate a suitable schema.
Fix:
Ensure that your entity classes are correctly annotated. Below is an example of a properly annotated entity class:
import javax.persistence.*;
@Entity
@Table(name = "User")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username", nullable = false, unique = true)
private String username;
@Column(name = "password", nullable = false)
private String password;
// Getters and Setters
}
In this code:
- The
@Entity
annotation marks the class as a Hibernate entity. - The
@Table
annotation specifies the name of the corresponding database table. - The
@Id
annotation identifies the primary key, and@GeneratedValue
indicates how the ID will be generated.
4. Inconsistent Class and Database Naming
Issue:
Another common problem stems from inconsistent naming conventions between your Java classes and your database.
Fix:
Stick to consistent naming conventions across your entity classes and your database. By default, Hibernate converts camel case to snake case. For example, the entity UserProfile
would be mapped to the table user_profile
.
You can control the naming strategy if you are using custom naming conventions or need to match legacy databases. For instance:
<property name="hibernate.physical_naming_strategy">org.hibernate.boot.model.naming.PhysicalNamingStrategyStandardImpl</property>
5. Missing Dependencies
Issue:
Sometimes the Hibernate schema creation will fail due to missing dependencies or incorrect library versions in your project configuration (e.g., Maven or Gradle).
Fix:
Ensure you have all the necessary dependencies and the correct version of Hibernate. An example Maven dependency would look like this:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>4.3.11.Final</version>
</dependency>
Additionally, check for other dependencies required for the database you are using.
6. Enabling SQL Logging
Issue:
During the schema generation process, you may not have enough feedback about what's happening. The absence of logging makes it challenging to pinpoint issues.
Fix:
Enable SQL logging in your Hibernate configuration. This helps debug errors more effectively:
<property name="hibernate.show_sql">true</property>
<property name="hibernate.format_sql">true</property>
With these properties enabled, you will see SQL statements printed in the console, providing insight into the schema creation happening behind the scenes.
Best Practices for Schema Creation with Hibernate
-
Use a Separate Profile for Development: Always use a dedicated profile for development with settings to drop and recreate schemas. Production should use
update
orvalidate
. -
Keep Migrations in Mind: For production environments, consider using a database migration tool such as Liquibase or Flyway to manage schema updates rather than relying solely on Hibernate.
-
Review Exceptions Carefully: Always review exceptions in the logs to get clear insights on schema issues. A tiny misconfiguration can cause substantial problems.
-
Regularly Update Dependencies: Regularly check and update your Hibernate dependencies to leverage the latest features and bug fixes.
-
Refer to Documentation: Always refer to the Hibernate ORM documentation for up-to-date information on features, configurations, and best practices.
Closing Remarks
Hibernate is a powerful tool, yet it's not without its pitfalls during schema creation. By understanding the common issues and their solutions outlined in this post, you can quickly troubleshoot problems and improve your development workflow.
Stay organized, follow best practices, and make the most of Hibernate's capabilities. As your application grows and evolves, a sound understanding of schema creation will be pivotal in maintaining an efficient workflow.
Happy Coding!
Checkout our other articles