Streamline Entity to DTO Mapping with Java 8 Lambdas
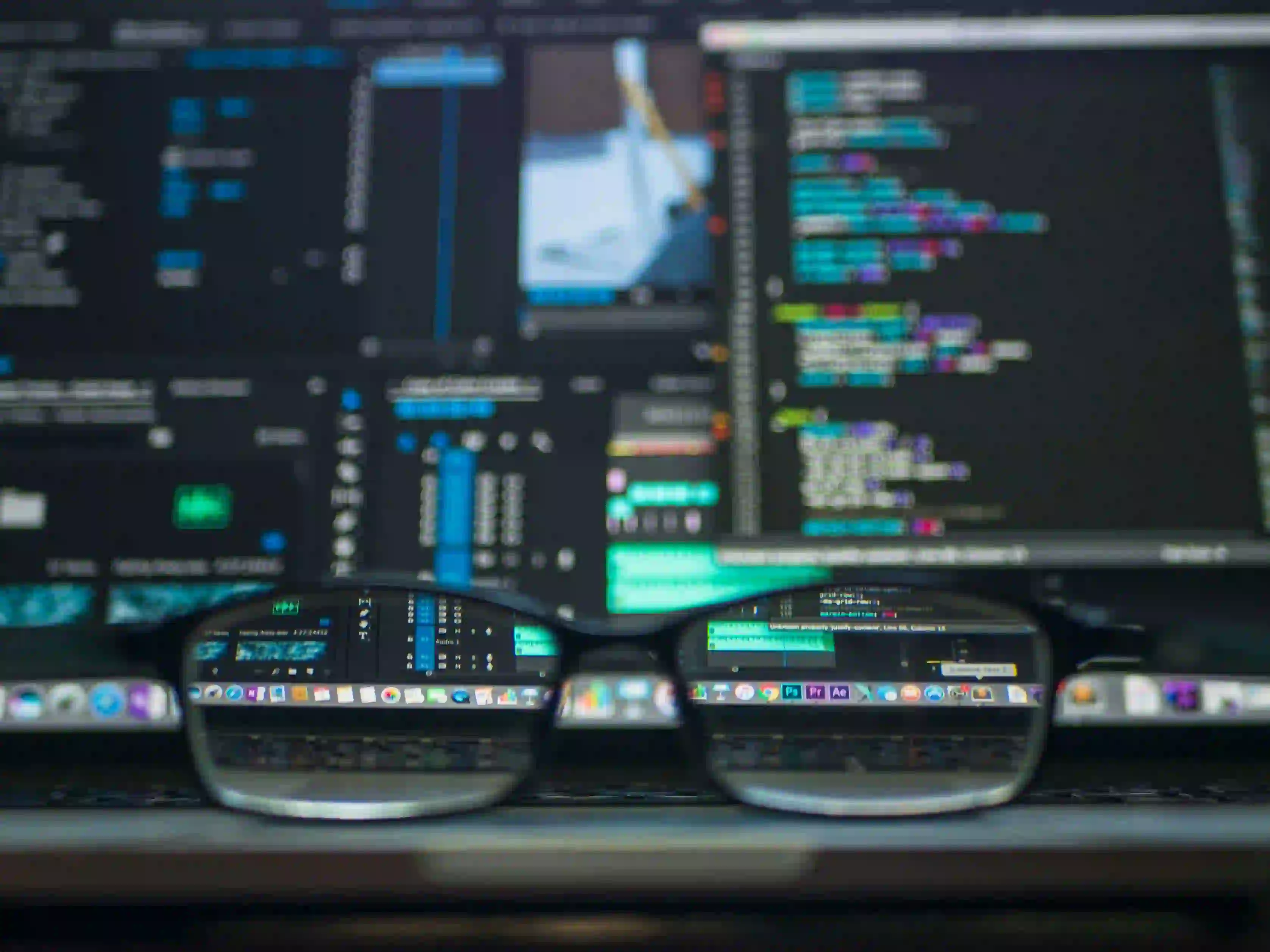
Streamline Entity to DTO Mapping with Java 8 Lambdas
In modern software development, efficiently mapping between different layers of an application – such as the Entity layer and the Data Transfer Object (DTO) layer – is pivotal. Entities represent the domain model, while DTOs are optimized for data transfer. Traditionally, the mapping process can become verbose and repetitive, posing challenges in maintainability and readability. Fortunately, Java 8 introduced lambda expressions, stream APIs, and other enhancements that can simplify this tedious chore.
In this blog post, we will explore techniques to streamline entity-to-DTO mapping using Java 8's functional programming capabilities. We will cover real-world examples, best practices, and the advantages offered by this approach. By the end, you will be equipped with tools and examples that showcase how to create cleaner, more maintainable code.
Understanding DTOs and Entities
Before diving into mapping, let's clarify the difference between DTOs and Entities.
-
Entity: An entity represents a table in a database. It contains attributes that correspond to the columns and typically includes behavior apart from basic data manipulation.
-
DTO (Data Transfer Object): A DTO is a plain object that carries data between processes. DTOs are often used to aggregate data and transfer it efficiently, minimizing the number of calls to the backend.
Example:
Consider the following simple User
entity:
@Entity
public class User {
private Long id;
private String name;
private String email;
// Constructors, getters, and setters
}
And its corresponding UserDTO
:
public class UserDTO {
private Long userId;
private String fullName;
private String emailAddress;
// Constructors, getters, and setters
}
The Traditional Mapping Approach
In a traditional mapping setup, converting an entity to a DTO usually involves a manual process for each field:
public UserDTO mapToDTO(User user) {
if (user == null) return null;
UserDTO userDTO = new UserDTO();
userDTO.setUserId(user.getId());
userDTO.setFullName(user.getName());
userDTO.setEmailAddress(user.getEmail());
return userDTO;
}
While this works, it becomes cumbersome when dealing with many fields or entities. This is where Java 8's streams and lambdas come into play.
Utilizing Java 8 Streams and Lambdas
Simplified Mapping with Streams
Java 8's stream API brings a functional approach that can help streamline our mapping code. Here’s how to perform the mapping using streams:
public List<UserDTO> mapToDTOs(List<User> users) {
return users.stream()
.map(user -> new UserDTO(user.getId(), user.getName(), user.getEmail()))
.collect(Collectors.toList());
}
Explanation:
- Stream Creation:
users.stream()
creates a stream from the user list. - Map Function: The
map
method applies a function to each element in the stream, transformingUser
entities intoUserDTOs
. - Collection:
collect(Collectors.toList())
gathers the resulting DTOs back into a list.
This approach is concise and scales easily, allowing for simple changes should your DTO structure evolve.
Mapping with Custom Transformation Logic
Sometimes, simple property copying isn't enough. You may need custom transformation logic. Here’s how to implement it in a clear and effective way:
public List<UserDTO> mapToDTOs(List<User> users) {
return users.stream()
.map(user -> {
UserDTO userDTO = new UserDTO();
userDTO.setUserId(user.getId());
userDTO.setFullName(user.getName().toUpperCase()); // Custom transformation
userDTO.setEmailAddress(user.getEmail());
return userDTO;
})
.collect(Collectors.toList());
}
Why Use Custom Logic?
Using custom transformations delivers more than just meeting DTO requirements. It can unify the format and ensure consistency across your application.
Handling Null Values
In a robust application, you should account for potential null values. Establishing safety during mapping is critical. Here's how you can implement that:
public List<UserDTO> mapToDTOs(List<User> users) {
return users.stream()
.filter(Objects::nonNull) // Filter out any null entities
.map(user -> new UserDTO(user.getId(), user.getName(), user.getEmail()))
.collect(Collectors.toList());
}
Upsides of This Approach:
- Safety: Prevents null pointer exceptions.
- Clarity: Clearly communicates the intent of filtering out nulls.
Performance Considerations
When dealing with large datasets, performance could become an issue. Streams provide an efficient approach with lazily evaluated computations. However, consider how often you filter or map to ensure optimal data handling.
Example of Parallel Streams
For highly concurrent data processing, leveraging parallelism can significantly enhance performance:
public List<UserDTO> mapToDTOs(List<User> users) {
return users.parallelStream() // Utilize multiple cores
.filter(Objects::nonNull)
.map(user -> new UserDTO(user.getId(), user.getName(), user.getEmail()))
.collect(Collectors.toList());
}
When to Use Parallel Streams
Always evaluate if the overhead of managing multiple threads is worth the performance benefits. Parallel processing suits situations with large datasets and heavy computation.
Final Thoughts
Mapping entities to DTOs doesn’t have to be a tedious task filled with repetitive code. By leveraging the capabilities of Java 8 streams and lambdas, you can create clearer, more maintainable code that enhances productivity.
Key Takeaways
- Clarity and Conciseness: Streamlined mapping reduces boilerplate code.
- Custom Transformations: Customize DTO creation easily.
- Safety: Ensure robust handling of null values.
- Performance: Optimize processing with parallel streams when necessary.
For additional reading on Java 8 features, visit the official Java documentation. By integrating these practices into your application, you'll streamline the mapping process while enhancing the overall maintainability of your codebase.