Solving Complex Communication with Java's Mediator Pattern
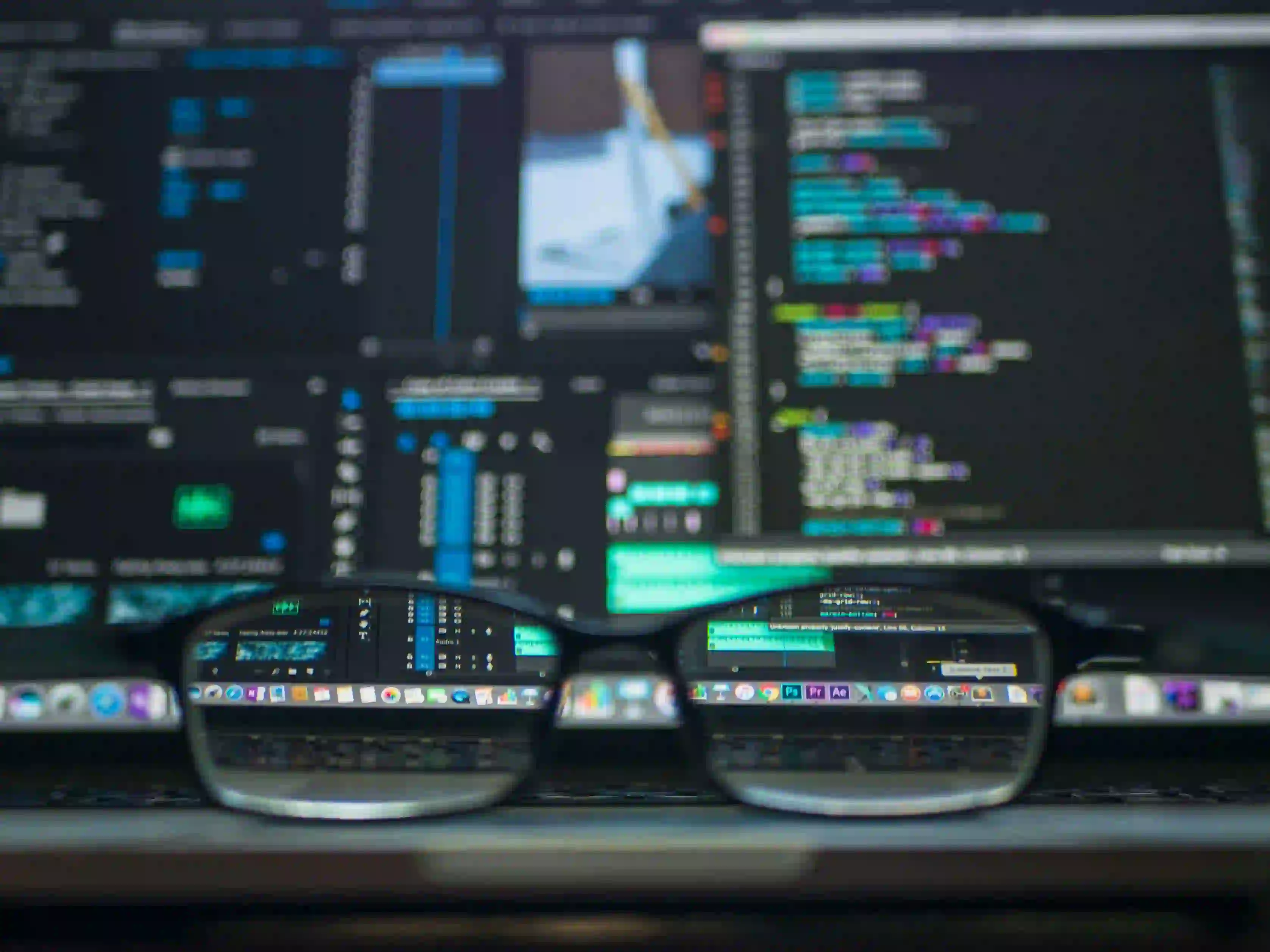
Solving Complex Communication with Java's Mediator Pattern
In software design, the communication between various components can often become complex and tangled, leading to what is commonly referred to as the spaghetti code problem. A particularly effective way to manage such complexity is by utilizing design patterns. One such pattern is the Mediator Pattern. In this blog post, we will dive deep into the Mediator Pattern, how it can solve intricate communication challenges in Java applications, and provide practical examples, including illustrative code snippets.
What is the Mediator Pattern?
The Mediator Pattern is behavioral design pattern that defines an object that coordinates communication between different objects. This pattern promotes loose coupling by keeping objects from referring to each other explicitly, thereby reducing the dependencies between them.
Key Benefits:
- Reduced Complexity: The Mediator handles communication, simplifying each participant's role.
- Decoupling: Components interact indirectly through the Mediator, making them easier to modify and maintain.
- Single Responsibility Principle: Classes can focus on their primary role without worrying about the communication mechanisms.
When to Use the Mediator Pattern
Consider using the Mediator Pattern in scenarios like:
- Complex Event Handling: When multiple components need to react to events.
- User Interface Dynamics: Interactions between UI elements can be managed centrally.
- Networked Applications: Managing communication between nodes without tightly coupling them.
Implementing the Mediator Pattern in Java
Let's explore a concrete implementation of the Mediator Pattern in Java. Suppose we want to simulate a chat room where multiple users can communicate without being directly aware of each other. We will define a Mediator interface, a ConcreteMediator, and the Colleague classes representing users.
Step 1: Define the Mediator Interface
The Mediator interface declares a method for communication and keeps a record of its participants:
import java.util.ArrayList;
import java.util.List;
interface Mediator {
void send(String message, Colleague colleague);
void registerColleague(Colleague colleague);
}
Step 2: Create the Colleague Abstract Class
This class represents participants in the chat room. It will hold a reference to the Mediator.
abstract class Colleague {
protected Mediator mediator;
public Colleague(Mediator mediator) {
this.mediator = mediator;
}
public abstract void receive(String message);
}
Step 3: Implement the Concrete Colleague Classes
Here's how we can implement user classes that will interact within the chat room.
class User extends Colleague {
private String name;
public User(Mediator mediator, String name) {
super(mediator);
this.name = name;
mediator.registerColleague(this);
}
@Override
public void receive(String message) {
System.out.println(name + " received: " + message);
}
public void send(String message) {
System.out.println(name + " sends: " + message);
mediator.send(message, this);
}
}
Step 4: Implement the Concrete Mediator
The ConcreteMediator manages communication between the users:
class ChatRoom implements Mediator {
private List<Colleague> colleagues;
public ChatRoom() {
colleagues = new ArrayList<>();
}
@Override
public void send(String message, Colleague colleague) {
for (Colleague c : colleagues) {
// Do not send the message back to the sender
if (c != colleague) {
c.receive(message);
}
}
}
@Override
public void registerColleague(Colleague colleague) {
colleagues.add(colleague);
}
}
Step 5: Putting it All Together
Now we can simulate a chat room where users can interact:
public class MediatorPatternDemo {
public static void main(String[] args) {
Mediator chatRoom = new ChatRoom();
User alice = new User(chatRoom, "Alice");
User bob = new User(chatRoom, "Bob");
User charlie = new User(chatRoom, "Charlie");
alice.send("Hello everyone!");
bob.send("Hi Alice!");
charlie.send("Good morning!");
}
}
Explanation of the Code
- Mediator Interface: Defines how messages are sent and manages the participants.
- Colleague Class: Represents users, allowing them to send and receive messages.
- ConcreteMediator (ChatRoom): Holds references to the users and routes messages appropriately.
- User Interaction: When a user sends a message, the Mediator ensures that it gets delivered to all other users without directly involving them.
Advantages of Using the Mediator Pattern
- It significantly reduces the dependencies between components.
- The addition of new components or changing existing ones becomes trivial, as they only need to interact with the Mediator.
- The codebase remains clean and adheres to the Single Responsibility Principle.
When Not to Use the Mediator Pattern
While the Mediator Pattern offers numerous benefits, it is not a one-size-fits-all solution. It may introduce unnecessary complexity if:
- The communication is simple enough that explicit links between objects are clearer.
- You have a small number of participants where direct communication is less cumbersome.
To Wrap Things Up
The Mediator Pattern is an invaluable tool for simplifying complex communication between various components in Java applications. By promoting loose coupling and enhancing code maintainability, it aligns well with modern software design principles.
In this blog post, we covered the Mediator Pattern comprehensively and provided a practical code example to illustrate its usage. As software systems continue to grow in complexity, design patterns like the Mediator will be crucial in helping developers manage and maintain their applications effectively.
For further reading, consider checking out resources on design patterns or Java programming.
Feel free to explore, implement, and adapt the Mediator Pattern in your projects to experience the benefits firsthand! Happy coding!