Mastering Code Efficiency: Android Developer's Biggest Challenge
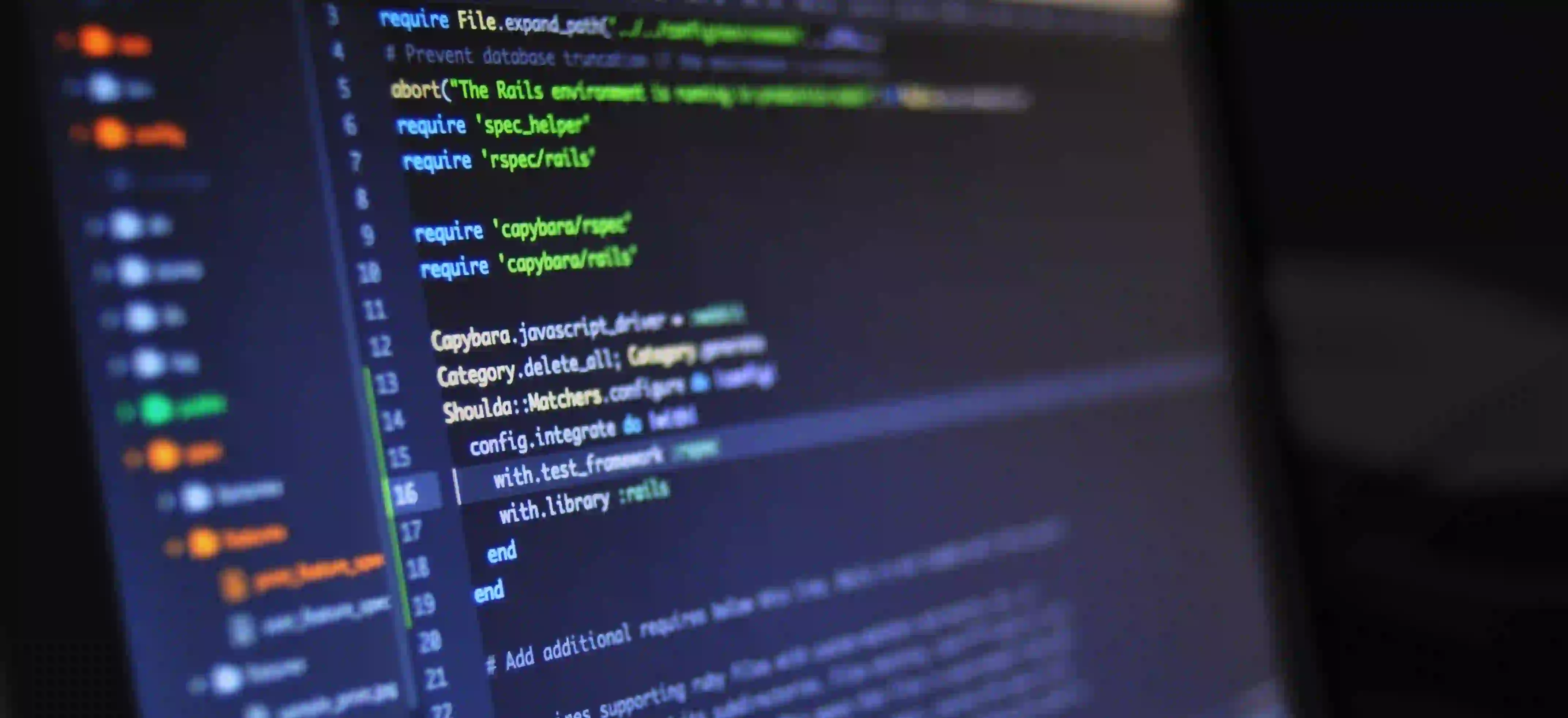
Mastering Code Efficiency: Android Developer's Biggest Challenge
In the world of Android development, creating functional and aesthetically pleasing applications is only part of the job. The true challenge lies in delivering a seamless user experience, which relies heavily on code efficiency. In this blog post, we will explore the importance of code efficiency, dive into strategies for mastering it, and provide practical examples to improve your Android apps.
Why Code Efficiency Matters
In software development, code efficiency refers to how well your code uses system resources. Efficient code not only enhances performance but also improves maintainability and scalability. Here are a few reasons why you should prioritize code efficiency:
- Performance: Users expect apps to run smoothly. Efficient code ensures that applications execute faster and use less battery power.
- User Experience: An app that responds to user interactions quickly and smoothly creates a positive experience, which can lead to better reviews and increased usage.
- Maintainability: Clean and efficient code is easier to read and modify, allowing for faster bug fixes and feature additions.
- Scalability: As an application adds features and scales up, efficient code will handle increased loads better than bloated or poorly structured code.
A Deep Dive into Efficiency Strategies
Now that we've established the importance of code efficiency, let’s go over some strategies that Android developers can implement to optimize their code.
1. Optimize Layouts
Using layouts efficiently is crucial in Android development. Nested layouts can significantly decrease performance, particularly during rendering. Use ConstraintLayout instead of nested LinearLayouts or RelativeLayouts.
Example:
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, World!"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintLeft_toLeftOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Why?
Using ConstraintLayout
reduces the number of view groups, thus improving the layout rendering performance.
2. Load Resources Wisely
Android loads resources in memory to display them. It is crucial to load only the resources you need when you need them. Use Lazy Loading
to defer the loading of resources until they are actually required.
Example:
private void loadImage(String url) {
Glide.with(this)
.load(url)
.into(imageView);
}
Why?
By employing libraries like Glide, you are leveraging efficient image loading. Glide handles caching and memory efficiently, improving both performance and user experience.
3. Use Background Tasks
Never perform long-running operations on the main thread. Use AsyncTask or, better yet, WorkManager to handle background tasks. This allows for code execution that does not interrupt the UI thread.
Example:
new AsyncTask<Void, Void, String>() {
@Override
protected String doInBackground(Void... voids) {
// Simulate long-running task
return fetchDataFromNetwork();
}
@Override
protected void onPostExecute(String result) {
textView.setText(result);
}
}.execute();
Why?
This ensures that the application remains responsive while performing lengthy operations. It enhances performance and user experience by keeping the UI fluid.
4. Avoid Memory Leaks
Memory leaks can severely affect an application's performance and lead to crashes. Always unregister your listeners and keep track of contexts.
Example:
@Override
protected void onDestroy() {
super.onDestroy();
myService.stopSelf();
myReceiver.unregisterReceiver();
}
Why?
This ensures that when your activity is destroyed, any resources allocated will be released. This reduces unnecessary memory consumption and ensures efficient app performance.
5. Efficient Data Handling
When dealing with large datasets, use RecyclerView instead of ListView. RecyclerView is a more efficient and flexible way to handle a large set of data.
Example:
RecyclerView recyclerView = findViewById(R.id.recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
MyAdapter adapter = new MyAdapter(dataList);
recyclerView.setAdapter(adapter);
Why?
RecyclerView optimizes the memory footprint and rendering by recycling views, thereby reducing the overhead when dealing with extensive datasets.
Tools for Measuring Code Efficiency
To master code efficiency, it is essential to measure and analyze your app's performance. Here are a few tools that Android developers can utilize:
- Android Profiler: Built into Android Studio, this tool helps you monitor CPU, memory, and network performance in real time. You can find detailed information about memory allocation and CPU usage.
- Lint: A static code analysis tool that detects inefficiencies in your code. It can warn you about performance issues and enforce best practices.
- StrictMode: A developer tool that detects potentially long-running operations on the main thread. Using StrictMode allows for identifying inefficiencies early in the development process.
To Wrap Things Up
Mastering code efficiency in Android development is not merely a coding task; it is a holistic approach to delivering high-quality, responsive applications. By optimizing layouts, managing resources wisely, utilizing background tasks, avoiding memory leaks, and handling data efficiently, developers can create applications that are not only functional but exhibit exceptional performance.
As you implement these strategies, remember that continuous learning and iteration are key to improving your coding skills and application efficiency. Experiment with different techniques, and always strive for a well-optimized codebase. Check out the official Android documentation for more details on best practices (Android Developer).
By prioritizing code efficiency, you’ll set yourself up for success in Android development and create applications that stand out in a crowded marketplace.
Now go ahead and refine your coding skills, and share your thoughts in the comments below! Happy coding!