Why Testing Private Methods Can Lead to Fragile Code
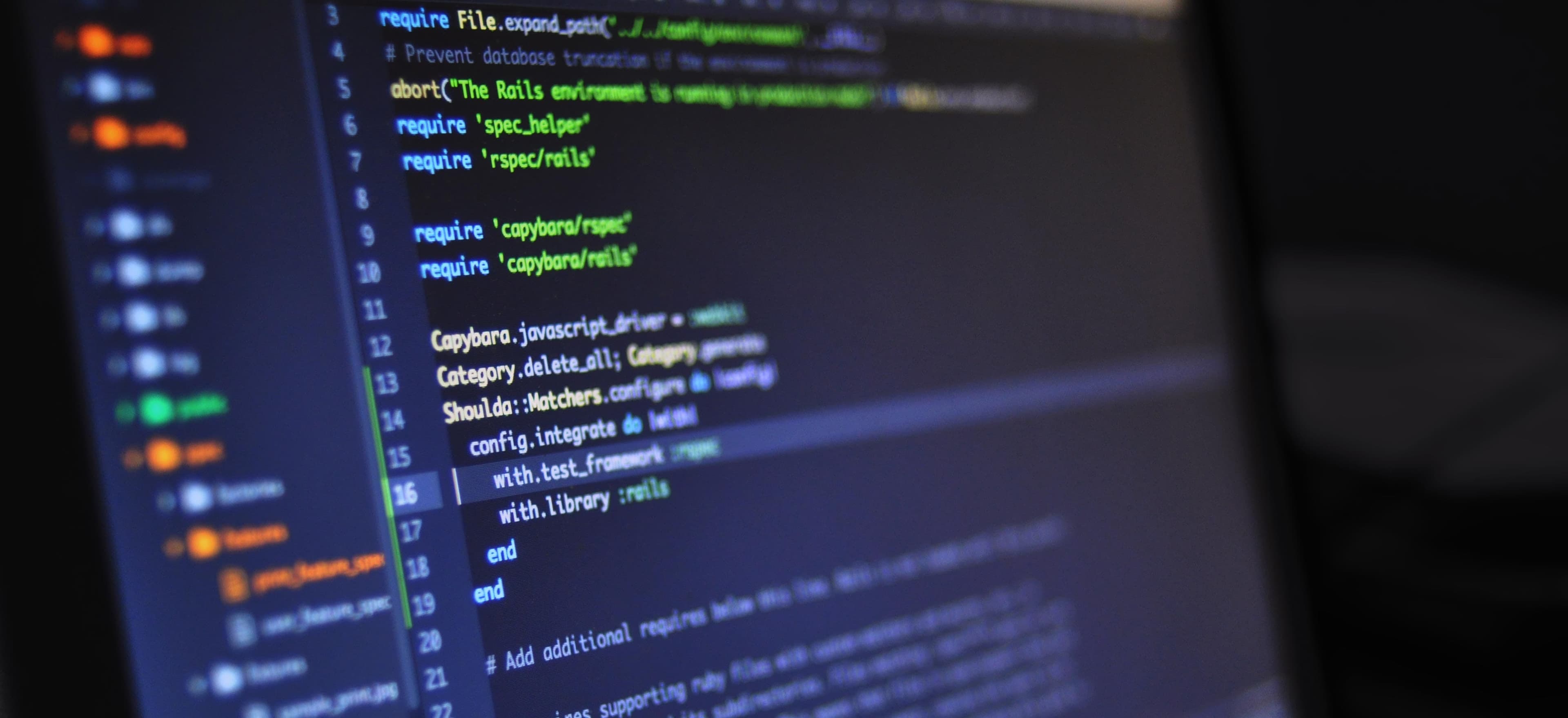
- Published on
Why Testing Private Methods Can Lead to Fragile Code
The Starting Line
In the world of software development, testing is often heralded as an essential practice. Developers employ various strategies to ensure their code is robust, reliable, and maintainable. However, one contentious area revolves around the testing of private methods. While it may seem beneficial to ensure every nook and cranny of the code is verified, doing so can lead to fragile codebases. In this blog post, we will explore the risks associated with testing private methods, with an emphasis on design principles and best practices in unit testing.
The Role of Private Methods in Object-Oriented Design
Private methods serve a specific purpose in object-oriented programming (OOP). Their core role is to encapsulate behavior that isn't intended to be exposed or used outside of the class itself. By keeping these methods private, a developer can:
- Maintain Abstraction: Users of the class needn't be concerned about internal workings, focusing instead on the public interface.
- Reduce Complexity: The fewer external interactions there are, the easier it is to understand the class's behavior.
- Change Implementation Without Breaking Contracts: Locked away in a private scope, the implementation can be changed without affecting external consumers.
Example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
private int subtract(int a, int b) {
return a - b;
}
}
In this example, subtract
is a private method that calculates the difference between two numbers. It shouldn't concern users of the Calculator
class.
The Temptation of Testing Private Methods
When faced with failing tests, developers are often tempted to directly test private methods. This approach seems natural, given that the goal is to verify that every part of the code works. However, this can introduce several pitfalls.
1. Fragile Tests
Testing private methods can lead to brittle tests that are highly sensitive to changes in implementation. If a private method’s signature (name, parameters, etc.) is changed, it may break multiple tests, even if the public interface remains untouched.
Code Smell Example:
@Test
public void testSubtract() {
Calculator calculator = new Calculator();
int result = calculator.subtract(5, 3);
assertEquals(2, result);
}
In the above code, the test directly calls subtract
, which is a private method. If the subtract
method changes to optimize performance, all tests that reference this method will also require modification, leading to fragile tests.
2. Encouraging Poor Design
By focusing on testing private methods, developers may inadvertently prioritize implementation over behavior. A well-designed system should encapsulate its behavior through its public API, not expose inner workings. When tests evolve to focus on those private methods, they may reduce the incentive to rethink the design.
3. Misleading Confidence
When tests target private methods, there may be a false sense of security. A passing test does not guarantee that the overall system behaves correctly. Instead, tests should validate the outcomes produced by public methods, which rely on private methods as necessary.
Example of Better Testing:
Instead of testing the private method directly, we can ensure that its behavior is represented through public methods.
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(8, calculator.add(5, 3));
}
In this case, the test indirectly ensures that subtract
works because add
relies on expected behavior that includes subtraction as part of its logic.
Refactoring and Testing: Best Practices
Favor Public Interfaces
Testing should focus on public methods. This practice promotes a better design where each function's behavior is confirmed through its exposure to external entities, leading to less fragile tests.
Use Test-Driven Development (TDD)
Utilizing TDD encourages developers to think first about functionality from the user's perspective, influencing the design of both classes and their methods. TDD encourages specifying behavior and will lead to testing the public interface:
- Write a failing test.
- Write the minimum code necessary to pass the test.
- Refactor with testing in place.
Continuous Refactoring
Refactor your code to simplify and enhance clarity while ensuring that tests cover external behaviors rather than internal implementations. As your understanding grows, your design should evolve.
Handling Complex Logic
Sometimes, logic within a private method may feel too complex or critical not to test. In these cases, consider extracting the functionality into a separate class or utility that can be either public or package-private, thus enabling testing without exposing too much.
Example of Extraction:
If the subtract
function grows in complexity and importance, extract it:
public class MathUtils {
public static int subtract(int a, int b) {
return a - b;
}
}
Now, it can be tested independently:
@Test
public void testSubtract() {
assertEquals(2, MathUtils.subtract(5, 3));
}
Key Takeaways
While testing private methods may seem useful at first glance, it can ultimately lead to fragile systems susceptible to change. Prioritizing testing of public interfaces promotes more resilient designs, encourages abstraction, and reduces complexity.
By adhering to good practices such as Test-Driven Development and continuous refactoring, developers can foster a codebase that stands the test of time—not only ensuring functionality but also improving maintainability.
For a deeper dive into unit testing best practices and related topics, check out the following resources:
By focusing on public behaviors, your code can achieve both stability and clarity—qualities critical to scaling applications effectively in the ever-evolving landscape of software development.
Checkout our other articles