Handling Java Application Exceptions in Massive Event Loads
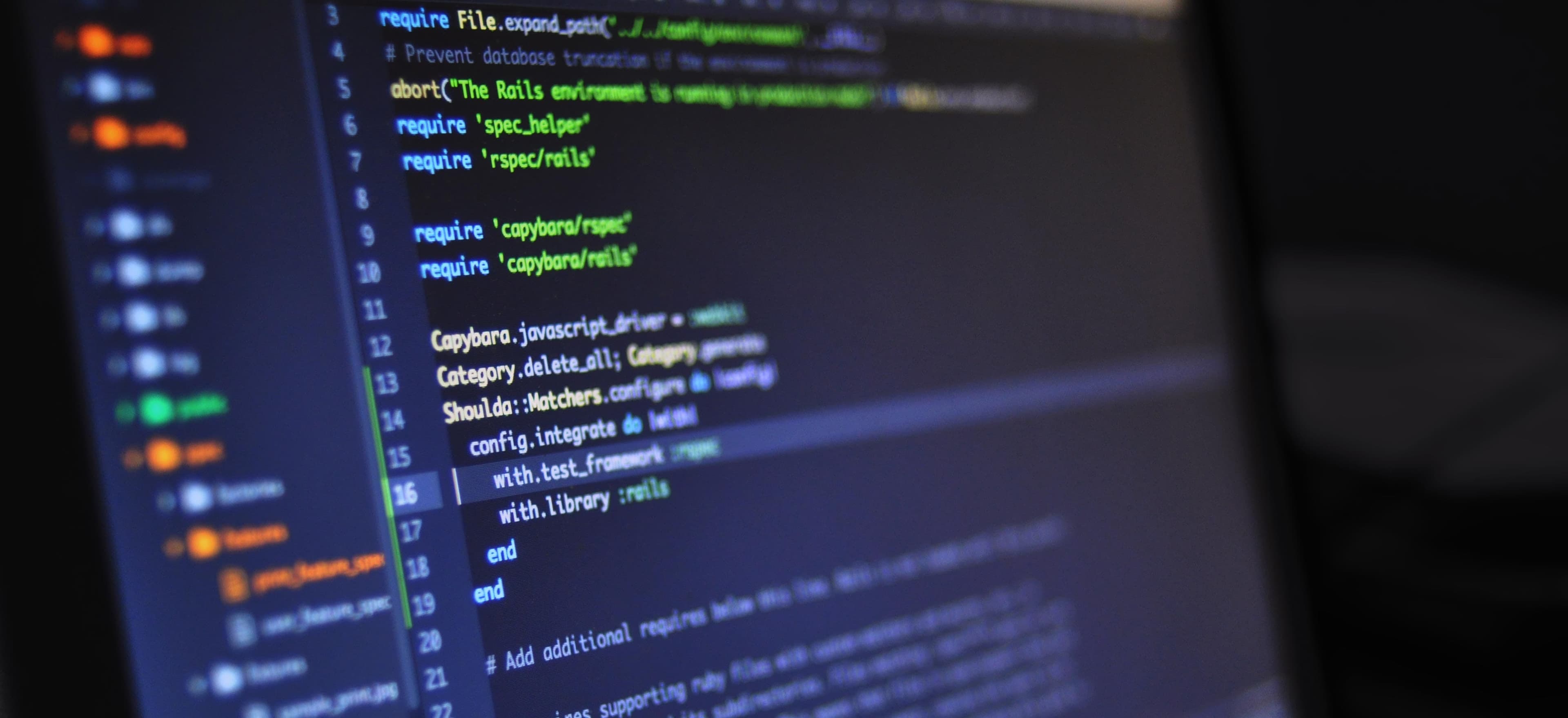
- Published on
Handling Java Application Exceptions in Massive Event Loads
Managing a Java application under massive event loads is a significant challenge for software developers. When systems are bombarded with an overwhelming number of requests, exceptions can arise that disrupt the normal flow of the application, leading to poor performance or complete breakdowns. In this blog post, we will explore effective strategies for handling exceptions in Java applications under high-pressure scenarios.
Understanding Exceptions in Java Applications
In Java, an exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. In the context of massive event loads, exceptions can arise from various sources, including:
- Input/output failures
- Network connectivity issues
- Out-of-memory errors
- Database access problems
Properly handling these exceptions is essential for maintaining the stability and reliability of your application.
Types of Exceptions in Java
There are two main categories of exceptions in Java:
-
Checked Exceptions: These are exceptions that are checked at compile-time. If your code might throw a checked exception, you must either handle it with a try-catch block or declare it in the method signature. Examples include
IOException
andSQLException
. -
Unchecked Exceptions: These are not checked at compile-time and derive from
RuntimeException
. You are not mandated to catch them, but it is a good practice to handle them. Examples includeNullPointerException
,ArrayIndexOutOfBoundsException
, andConcurrentModificationException
.
Strategies to Handle Exceptions Under Massive Event Loads
1. Centralized Exception Handling
Implementing a centralized exception handling mechanism can significantly simplify error management. Instead of scattering try-catch blocks throughout your application, you can use an exception handler to capture and process exceptions in a unified way.
Example: Centralized Exception Handler
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.http.HttpStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public String handleGlobalException(Exception ex) {
// Log exception details
System.out.println("Global Exception Caught: " + ex.getMessage());
return "An error occurred while processing the request.";
}
}
In this code snippet, we use @ControllerAdvice
to define a global exception handler. This allows us to intercept all exceptions raised by controller methods, enabling consistent logging and user feedback.
2. Implementing Resilient Architectures
When dealing with massive event loads, implementing design patterns such as Circuit Breaker and Retry can help manage exceptions effectively. These patterns allow your application to "fail fast" and avoid overwhelming backend systems during peak loads.
Example: Circuit Breaker Pattern
import java.util.concurrent.TimeUnit;
import com.netflix.hystrix.contrib.javanica.annotation.HystrixCommand;
public class EventService {
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String processEvent(String event) {
// Simulating an event processing delay
if (Math.random() > 0.5) {
throw new RuntimeException("Unable to process event");
}
return "Event processed successfully";
}
public String fallbackMethod(String event) {
return "Service is currently unavailable. Please try again later.";
}
}
In this example, we use the Hystrix library, which implements the Circuit Breaker pattern. If the processEvent
method fails, the fallbackMethod
is invoked, providing the user with a relevant message instead of allowing the application to crash.
3. Logging and Monitoring
Effective logging and monitoring are crucial for detecting and understanding exceptions in real-time. Use a logging framework like Log4j or SLF4J to capture detailed log messages.
Example: Using SLF4J for Logging
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void findUserById(String userId) {
try {
// Simulating a database call
throw new IllegalArgumentException("User ID cannot be null");
} catch (Exception e) {
logger.error("Exception occurred while finding user with ID {}: {}", userId, e.getMessage());
// Handle exception as needed
}
}
}
By utilizing SLF4J, we can log a message that includes both context and the exception details. This logging will aid in diagnosing problems later and enhances transparency within the application.
4. Rate Limiting
When faced with massive loads, it may be necessary to implement a rate limiting mechanism to control the flow of requests reaching your application. This can help reduce the occurrence of exceptions and maintain functionality at scale.
Example: Simple Rate Limiter
import java.util.concurrent.atomic.AtomicInteger;
public class SimpleRateLimiter {
private final int maxRequests;
private final long timeframeMillis;
private final AtomicInteger requestCount = new AtomicInteger(0);
private long startTime;
public SimpleRateLimiter(int maxRequests, long timeframeMillis) {
this.maxRequests = maxRequests;
this.timeframeMillis = timeframeMillis;
this.startTime = System.currentTimeMillis();
}
public boolean allowRequest() {
long currentTime = System.currentTimeMillis();
if (currentTime - startTime > timeframeMillis) {
requestCount.set(0);
startTime = currentTime;
}
if (requestCount.incrementAndGet() > maxRequests) {
return false; // Reject the request
}
return true; // Allow the request
}
}
In this code snippet, a simple rate limiter tracks the number of requests made in a specified timeframe. This mechanism can help prevent excessive load on the application and reduce the likelihood of exceptions, ensuring smoother performance during peak times.
5. Graceful Degradation
While we strive for absolute application availability, unexpected errors can occur. In such cases, implementing a graceful degradation strategy can enhance user experience. This involves providing limited functionality rather than complete failure.
Example: Graceful Degradation
public String fetchUserProfile(String userId) {
try {
// Attempt to fetch user profile from remote service
return remoteService.getUserProfile(userId);
} catch (Exception e) {
System.out.println("Error fetching user profile. Returning default profile.");
return getDefaultUserProfile();
}
}
private String getDefaultUserProfile() {
return "{ \"name\": \"Guest\", \"email\": \"guest@example.com\" }";
}
In this method, if the user profile cannot be fetched, a default profile is returned instead. This maintains user engagement and functionality even when there are backend issues.
Lessons Learned
Handling exceptions in Java applications under massive event loads requires a combination of robust design patterns, precise coding practices, and monitoring mechanisms. By centralizing exception handling, implementing resilience patterns, logging comprehensively, applying rate limits, and embracing graceful degradation, you can significantly reduce the risk of catastrophic failures while maintaining a smooth user experience.
Further Reading
By following the best practices outlined in this post, you will be well on your way to building fault-tolerant Java applications that efficiently handle massive event loads. Remember, the key to success in this area lies in preparation and proactive management of exceptions.
Checkout our other articles