Common Java 9 Module Errors and How to Fix Them
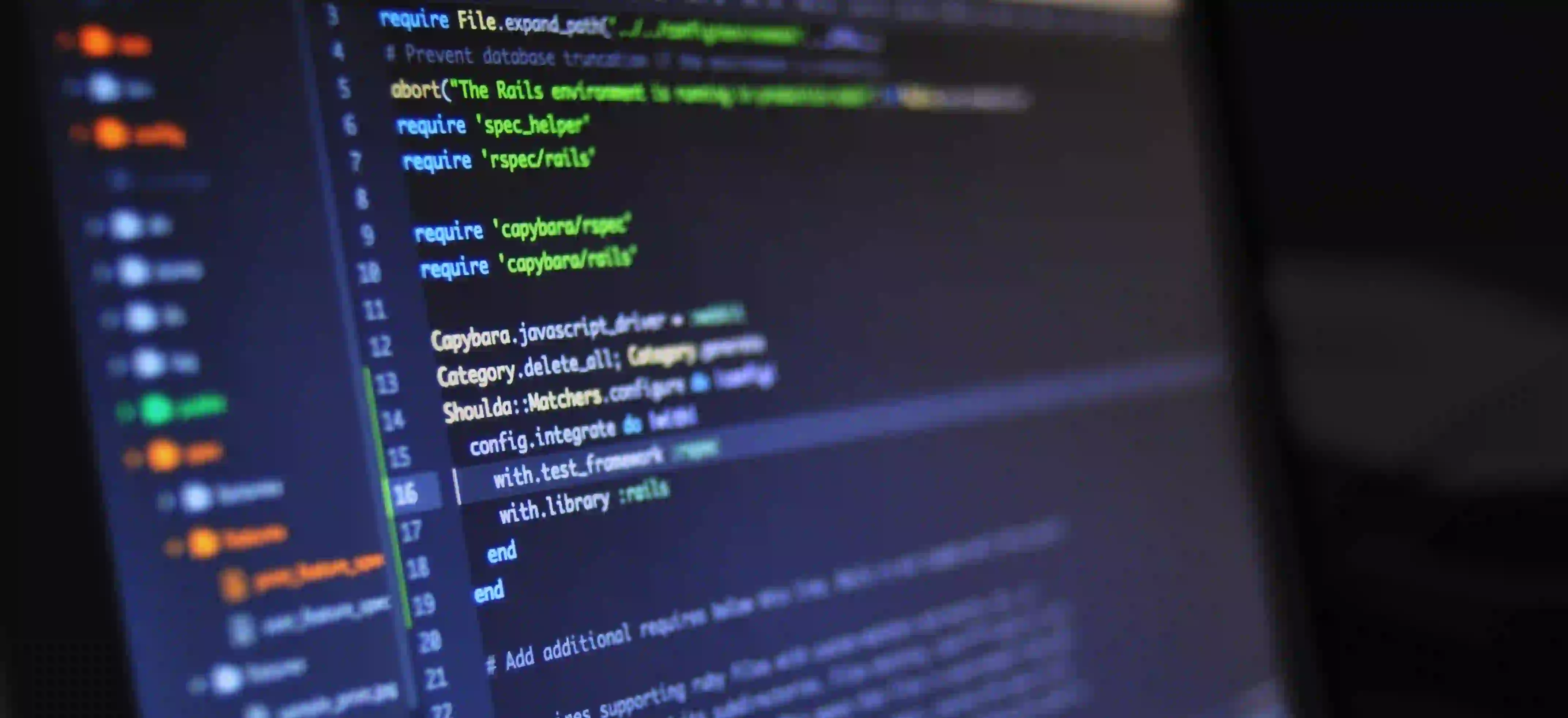
Common Java 9 Module Errors and How to Fix Them
Java 9 introduced the Java Platform Module System (JPMS), also known as Project Jigsaw. This revolutionary change enhances the Java ecosystem, allowing for better modularization of code. However, it also comes with its own set of challenges. Developers transitioning from earlier versions of Java to Java 9 may encounter various module-related errors. In this article, we will explore some common Java 9 module errors and how to effectively fix them.
Understanding Java Modules
Before diving into the errors, it's crucial to understand the concept of modules. A module is essentially a grouping of related packages and resources. Java modules can improve encapsulation, reduce the size of applications, and enhance performance. Here's a simple example of a module declaration in Java:
module com.example.calculator {
exports com.example.calculator.operations;
exports com.example.calculator.utils;
}
In this example, we’ve declared a module named com.example.calculator
that exports two packages. Only the specified packages are accessible by other modules, improving encapsulation.
Common Java 9 Module Errors
While working with Java modules, developers may encounter several common errors. Let's discuss these errors, their causes, and how to fix them.
1. module not found
Error
One of the most frequent errors developers encounter is the "module not found" error. This happens when the Java compiler cannot locate the specified module.
Causes:
- Incorrect module name in the command-line arguments.
- The module does not exist in the classpath.
Solution:
Ensure that the module is correctly named and exists in your module path. To compile and run your module properly, use the --module-path
option in your javac
and java
commands, like so:
javac --module-path mods -d out $(find src -name "*.java")
java --module-path mods -m com.example.calculator/com.example.calculator.Main
This example assumes your compiled modules are in the mods
directory, and the module's main class is Main
.
2. IllegalAccessError
This error arises when you attempt to access a class or member that is not accessible due to the visibility constraints imposed by the module system.
Causes:
- Attempting to access a class from another module that has not been exported.
Solution:
To resolve this error, make sure to export the necessary packages in the module-info.java
file. For example:
module com.example.calculator {
exports com.example.calculator.operations;
}
If you're accessing a class from a third-party module, ensure that the library is designed to be modular and that you have the required export statements. You can refer to the official documentation for collaborating modules here.
3. module does not name a main class
If you are trying to execute a module but encounter this error, it likely indicates that the specified module does not contain a valid main class.
Causes:
- The
module-info.java
file does not specify any main class. - The main class is incorrectly specified in the execution command.
Solution:
Make sure your module-info.java
file includes the correct main class. You can specify the main class while running the module using the -m
option. Here’s a practical example:
java --module-path mods -m com.example.calculator/com.example.calculator.Main
In this command, we execute the main class located in the com.example.calculator
module. Make sure the path and name are correct.
4. NoClassDefFoundError
This common error occurs when the Java runtime cannot find a class that was present during the compile time.
Causes:
- The class might have been in a different module, and that module is not on the runtime module path.
Solution: To fix this issue, verify that all necessary modules are included in the module path when executing your application. You can add multiple modules using:
java --module-path mods -m com.example.calculator/com.example.calculator.Main
Make sure to include all dependent modules in the mods
directory to resolve any NoClassDefFoundError
.
5. UnsupportedOperationException
You may encounter this error if you try to use classes or members that the module system restricts.
Causes:
- Attempting to manipulate collections, such as trying to modify a list that was returned as an unmodifiable list.
Solution: To avoid this, always check the documentation for the classes and methods you are using. If a method implies immutability, you'll need to find a way to create a modifiable version:
List<String> modifiableList = new ArrayList<>(Collections.unmodifiableList(originalList));
6. Cannot locate the Module
If you receive this error, it suggests your Java program cannot find modules on the module path.
Causes:
- Similar to the module not found error, either your command line is incorrect or the module is not compiled and not present in the specified location.
Solution: Double-check the module path in your execution command:
java --module-path out -m com.example.calculator/com.example.calculator.Main
Always ensure your modules are compiled successfully and that you're pointing to the correct directory.
Closing Remarks
The introduction of the Java Platform Module System in Java 9 offers a wealth of benefits but also requires a paradigm shift in how we think about application structure. Understanding and resolving common module errors can greatly enhance your development experience.
For further reading on Java modules and their benefits, check out resources like Oracle's official guide or further delve into modular programming best practices.
By familiarizing yourself with these common issues and their resolutions, you can improve your code quality and harness the full power of Java's modular capabilities effectively. Happy coding!