Common Design Pattern Pitfalls in Spring Boot Development
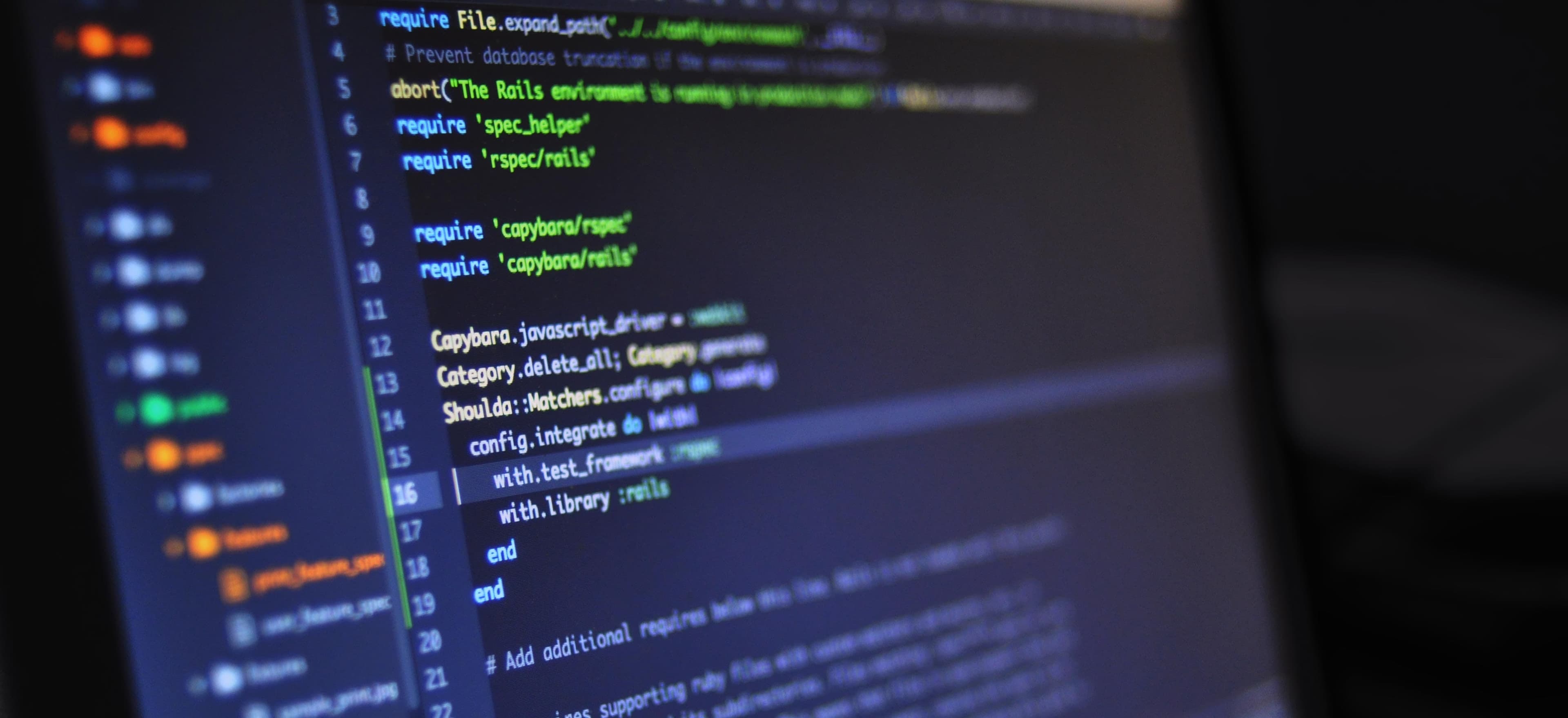
- Published on
Common Design Pattern Pitfalls in Spring Boot Development
Spring Boot has become one of the go-to frameworks for building enterprise-ready applications due to its simplicity and power. However, like any framework, it is important to maintain good design practices to avoid common pitfalls. Design patterns, when implemented correctly, can enhance the structure and maintainability of your Spring Boot application. This post delves into common pitfalls that developers might encounter when applying these patterns within Spring Boot.
Understanding Design Patterns
Before we explore common pitfalls, it's important to first understand what design patterns are. According to the Gang of Four, design patterns provide a standardized solution to common software challenges. They help improve code readability and reduce the complexities involved in developing software applications.
Common Design Patterns in Spring Boot
Spring Boot applications frequently leverage design patterns such as:
- Singleton
- Factory
- Strategy
- Observer
- Template Method
While these patterns can be powerful, improper implementation can lead to issues in maintainability, performance, scalability, and understanding of your codebase.
Singleton Pattern Pitfalls
Pitfall: Overuse of Singleton
Singleton is a common design pattern where a class has only one instance throughout the application lifecycle. However, overusing Singleton can lead to hidden dependencies and make unit testing more complicated.
Example
@Component
public class SingletonService {
private static SingletonService instance;
private SingletonService() {}
public static SingletonService getInstance() {
if (instance == null) {
instance = new SingletonService();
}
return instance;
}
}
Why This Is a Pitfall:
- The above implementation creates hidden dependencies. If you need to mock
SingletonService
, it becomes challenging to do so. - The Spring framework offers dependency injection, so a Singleton should be a managed bean instead.
Correct Approach
@Service
public class SingletonService {
// Business logic here
}
This allows Spring to manage the instance, making testing straightforward by leveraging Spring's test framework.
Factory Pattern Pitfalls
Pitfall: Incorrect Granularity
The Factory pattern provides an interface for creating objects. However, if the factory method creates objects that are too granular, it can lead to rigid and unusable designs.
Example
@Component
public class ProductFactory {
public Product createProduct(String type) {
if (type.equals("A")) {
return new ProductA();
} else if (type.equals("B")) {
return new ProductB();
}
throw new IllegalArgumentException("Unknown product type");
}
}
Why This Is a Pitfall:
- Adding another product type requires changing existing code, violating the Open/Closed Principle.
Correct Approach
public interface Product {
void create();
}
@Component
public class ProductFactory {
@Autowired
private ApplicationContext context;
public Product createProduct(String type) {
return (Product) context.getBean(type);
}
}
This way, you can add new product types dynamically without modifying existing code. All you need to do is annotate new classes with @Component
and give them the right identifier name.
Strategy Pattern Pitfalls
Pitfall: Overhead of Context Switching
The Strategy pattern lets you select an algorithm at runtime. Yet, the context switching between strategies can introduce overhead if not managed properly.
Example
public interface SortingStrategy {
void sort(int[] array);
}
public class QuickSort implements SortingStrategy {
public void sort(int[] array) {
// Logic for quicksort
}
}
public class SortManager {
private SortingStrategy strategy;
public void setStrategy(SortingStrategy strategy) {
this.strategy = strategy;
}
public void sortArray(int[] array) {
strategy.sort(array);
}
}
Why This Is a Pitfall:
- Constantly switching strategies may result in unnecessary performance overhead.
Correct Approach
Use caching or pre-evaluate needs before switching strategies. Analyze usage patterns and favor the most-used strategy to minimize context switching.
Observer Pattern Pitfalls
Pitfall: Memory Leaks Due to Improper Handling
The Observer pattern is useful for handling events, but if observers are not deregistered properly, it can result in memory leaks.
Example
public class EventManager {
private List<Observer> observers = new ArrayList<>();
public void addObserver(Observer observer) {
observers.add(observer);
}
public void notifyObservers(Event event) {
for (Observer observer : observers) {
observer.update(event);
}
}
}
Why This Is a Pitfall:
- If observers are long-lived while the event manager is not, callbacks can keep them alive longer than intended.
Correct Approach
Ensure observers deregister themselves or implement mechanisms to clean up observers when they are no longer needed.
public void removeObserver(Observer observer) {
observers.remove(observer);
}
By adding a removal mechanism, you can help mitigate potential memory leaks.
Template Method Pitfalls
Pitfall: Bloated Template Methods
The Template Method pattern provides a skeleton of an operation, allowing subclasses to define certain steps. However, if your template method gets too complex, it can lose its simplicity.
Example
public abstract class DataProcessor {
public final void processData() {
validate();
readData();
process();
writeData();
}
protected abstract void process();
private void validate() {
// some validation logic
}
private void readData() {
// logic to read data
}
private void writeData() {
// logic to write data
}
}
Why This Is a Pitfall:
- If the process becomes convoluted, it negates the purpose of the Template Method pattern.
Correct Approach
Break down complex steps into smaller, focused methods that maintain readability.
protected void readData() {
// Clear and focused data reading logic
}
The Bottom Line
Design patterns are powerful allies in software development but can quickly become pitfalls if misused. Recognizing and addressing these pitfalls will lead to more maintainable, scalable, and robust Spring Boot applications. Constantly strive for cleaner code, efficient patterns, and effective testing approaches.
Understanding both the advantages and disadvantages of design patterns is key. Make use of Spring's powerful dependency management and testing capabilities to avoid common pitfalls while leveraging design patterns wisely. Adapting your approach based on your application's needs will ensure your application not only works effectively but remains a pleasure to maintain.
For more insights on effective Spring Boot development practices, consider checking the following resources:
- Spring Framework Documentation
- Effective Java by Joshua Bloch
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides.