Common Pitfalls in Securing REST APIs with Spring Security
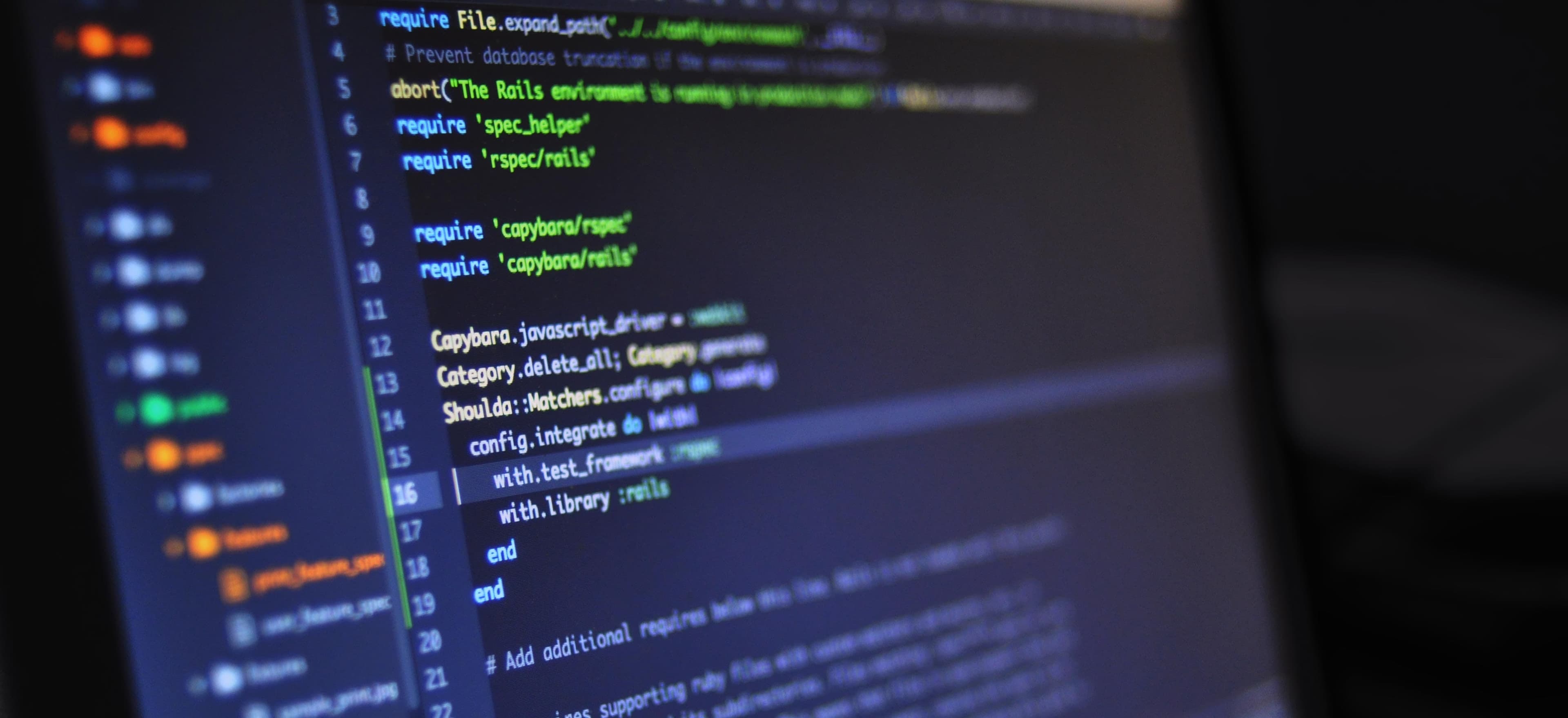
- Published on
Common Pitfalls in Securing REST APIs with Spring Security
In today’s world, securing your REST APIs is more important than ever. With the rise of data breaches and cyber threats, obtaining a robust security posture is critical. Spring Security is a popular framework for securing applications, but it comes with its own set of challenges and pitfalls. In this blog post, we will explore common mistakes developers make when securing REST APIs with Spring Security, and how to avoid them.
Understanding REST API Security
Before we dive into the pitfalls, it’s essential to understand what REST API security entails. At its core, securing a REST API involves authentication (verifying user identity) and authorization (granting access based on permissions).
Key Terms
- Authentication: Confirming the identity of users.
- Authorization: Determining what an authenticated user can access.
- Token-Based Authentication: Leveraging tokens (like JWT) for stateless authentication.
The benefits of securing REST APIs with Spring Security include ease of integration, a plethora of features, and community support. However, improper implementation can lead to vulnerabilities.
Common Pitfalls
1. Not Using HTTPS
Why It’s Problematic: One of the simplest yet most catastrophic mistakes is not using HTTPS. Data sent over HTTP is unencrypted and can be intercepted.
Solution: Always serve your APIs over HTTPS. This encrypts the data in transit, making it difficult for malicious actors to exploit.
// Spring Security configuration for enforcing HTTPS
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.requiresChannel()
.anyRequest()
.requiresSecure();
}
2. Insecure Password Storage
Why It’s Problematic: Using insecure methods to store passwords (like plain text) can lead to data breaches.
Solution: Always hash passwords using strong algorithms. BCrypt is a common choice in Spring Security.
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
// Hashing a password before storage
String rawPassword = "user_password";
BCryptPasswordEncoder encoder = new BCryptPasswordEncoder();
String hashedPassword = encoder.encode(rawPassword);
3. Weak Authentication Mechanisms
Why It’s Problematic: Relying solely on basic authentication or simple token mechanisms can leave your application vulnerable.
Solution: Opt for modern and robust authentication mechanisms such as OAuth 2.0, which is well-integrated with Spring Security.
4. Missing CSRF Protection
Why It’s Problematic: CSRF (Cross-Site Request Forgery) attacks can occur if your API is vulnerable.
Solution: While Spring Security enforces CSRF protections by default, REST APIs often use stateless authentication (like JWT). In that case, you may want to disable CSRF protection for certain endpoints.
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.ignoringAntMatchers("/api/**"); // Disable CSRF for REST APIs
}
5. Insufficient Role Management
Why It’s Problematic: Simply allowing access based on user roles without fine-grained controls can lead to over-privileged accounts.
Solution: Implement fine-grained permissions using @PreAuthorize
or @Secured
annotations to safeguard endpoints.
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/admin/data")
public List<Data> getAdminData() {
return dataService.getAdminData();
}
6. Overlooking Logging and Monitoring
Why It’s Problematic: Without proper logging and monitoring, detecting and responding to security incidents becomes difficult.
Solution: Implement logging for sensitive actions, and monitor logs for unusual access patterns.
7. Not Validating Input Data
Why It’s Problematic: Failing to validate input data can lead to injection attacks or buffer overflows.
Solution: Always validate user input against strict schemas to ensure only expected data types and formats reach your business logic.
@RequestMapping(value = "/user/{id}", method = RequestMethod.GET)
public ResponseEntity<User> getUser(@PathVariable("id") @Min(1) Long id) {
return ResponseEntity.ok(userService.getUserById(id));
}
8. Lack of Rate Limiting
Why It’s Problematic: APIs that do not implement rate limiting are prone to abuse from malicious users trying to overwhelm the service.
Solution: Use libraries like Bucket4j or Spring’s built-in functionalities to control the rate of incoming requests.
@Bean
public Filter rateLimitFilter() {
return new RateLimitFilter(); // Custom implementation to enforce rate limits
}
9. Misconfiguring CORS (Cross-Origin Resource Sharing)
Why It’s Problematic: Improperly configuring CORS can expose your API to unwanted requests.
Solution: Specify allowed origins in your Spring Security configuration.
@Override
protected void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**").allowedOrigins("https://yourfrontend.com");
}
10. Failing to Disable Default Security Settings
Why It’s Problematic: Default security settings may provide unnecessary access, leading to potential vulnerabilities.
Solution: Thoroughly review and customize default settings in your Spring Security configuration as per your application’s requirements.
Final Thoughts
Securing your REST APIs is not an option; it’s a necessity. Properly leveraging Spring Security alongside an understanding of common pitfalls can significantly enhance your application's security posture. By addressing these pitfalls, you ensure your API remains robust against emerging threats and vulnerabilities.
For additional reading on Spring Security, check out the Spring Security documentation. Remember, staying informed and proactive is your best defense.
Useful Resources
- Spring Security – Getting Started
- OWASP API Security Top 10
- Secure Your RESTful API with Spring Security
By avoiding these common pitfalls, you can create a REST API that is not only functional but also secure. Keeping security as a priority during the entire development lifecycle will save you time and resources in the long run.