Why Your Java Code Needs an Upgrade to Version 11
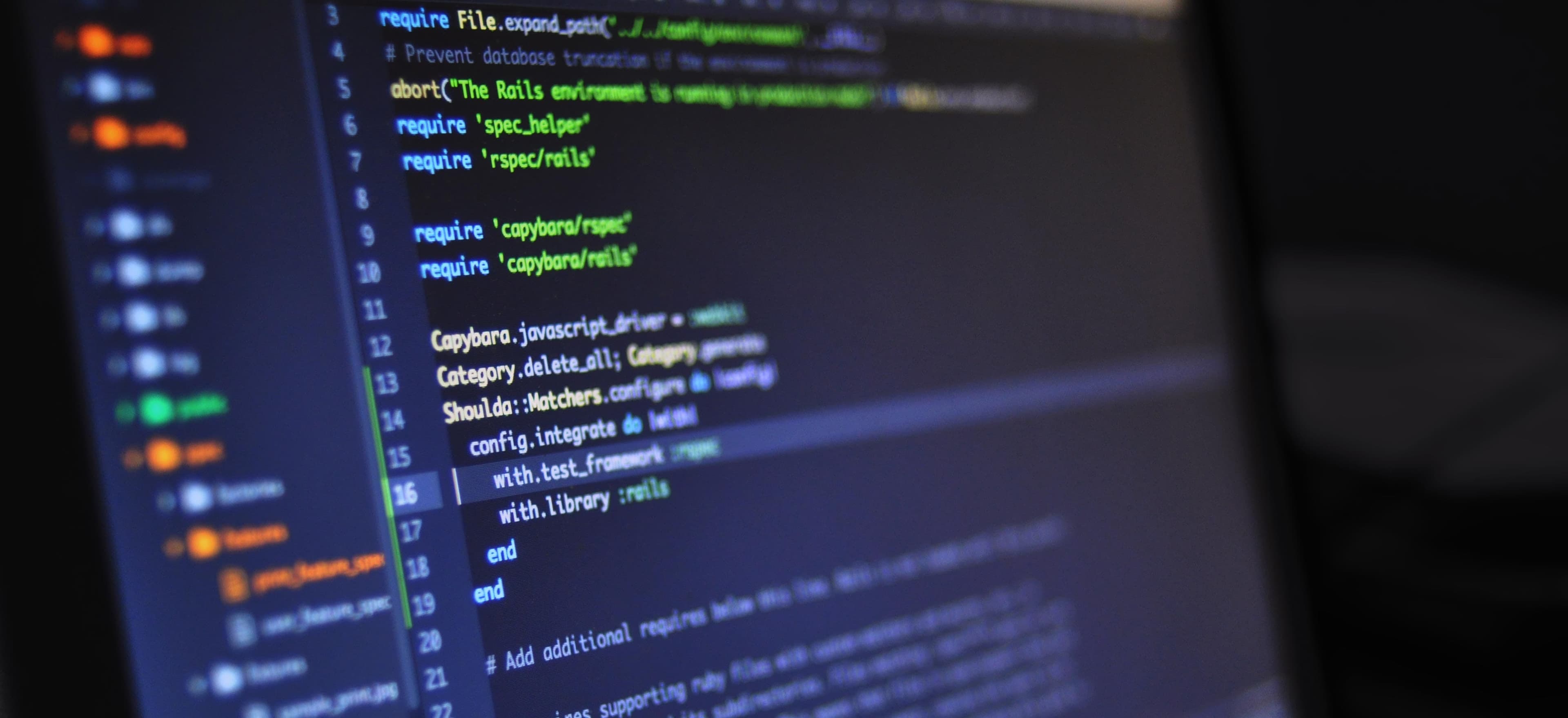
- Published on
Why Your Java Code Needs an Upgrade to Version 11
Java has been a stalwart in the software development universe since its birth in the mid-'90s. Through its various iterations, it has continuously embraced change, striving for improvements in performance, security, and ease of use. Enter Java 11, the long-term support (LTS) version released on September 25, 2018. If you're still running an earlier version of Java, this blog post will explore the compelling reasons for upgrading your code to Java 11.
What is Java 11?
Java 11 is a significant release in the Java ecosystem and is part of the Java SE (Standard Edition) platform. As an LTS release, it means that it will receive updates and support for several years, making it a reliable choice for businesses and developers.
Benefits of Upgrading to Java 11
1. Improved Performance
Java 11 offers various performance improvements over its predecessors. For example, the introduction of the Epsilon Garbage Collector provides a low-overhead memory management option for applications that manage their memory themselves.
java -XX:+UseEpsilonGC -jar your_application.jar
Using the Epsilon GC allows developers to explore new paradigms in memory management without impacting performance. This lower latency can be particularly beneficial in cloud environments, where microservices often require scalable and efficient operations.
2. New Language Features
Java 11 introduces a number of new features that streamline the development process:
Var (Local Variable Type Inference)
You can use var
to let the compiler deduce the type of a local variable.
var map = new HashMap<String, List<String>>();
Using var
reduces verbosity but maintains clarity. Since the type is inferred, it becomes easier to read and maintain. However, use it judiciously; overuse could hinder readability.
String Methods
Java 11 comes with new methods in the String
class, enhancing functionality:
- isBlank(): Checks if a string is empty or contains only whitespace.
- lines(): Returns a stream of lines from a string.
- repeat(int count): Repeats a string a specified number of times.
Example usage of these methods:
String text = "Hello, World! \nWelcome to Java 11.\n";
text.lines().forEach(System.out::println);
These improvements together make string manipulation much more efficient.
3. Modernized APIs
Java 11 enhances several existing APIs, making them more functional and easier to use. A prime example is the new HTTP Client API, which standardizes the way HTTP requests are made and supports both synchronous and asynchronous requests.
Using the HTTP Client API:
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class HttpExample {
public static void main(String[] args) throws Exception {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/data"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
}
}
This code snippet illustrates how easy it is to make HTTP requests in Java 11, allowing for cleaner code and better readability.
4. Removal of Deprecated Features
Java 11 cleans house by removing several older, less-used features, such as:
- Java EE and CORBA modules.
- Applet API and associated classes.
While the removal of these features may seem detrimental at first, it reduces complexity and enhances security. By cleaning up the codebase, Java promotes better practices and encourages the use of modern libraries and frameworks.
5. Enhanced Security
With every new version, Oracle aims to enhance security, and Java 11 is no different. From improved TLS support to better strength for cryptographic algorithms, the upgrades are crucial for any application exposed to the internet.
Why You Should Upgrade Now
Active Community and Ecosystem
Java 11 receives updates and patches, ensuring you get the latest security enhancements and performance improvements. Staying on a previous version means a lack of support, which can expose your applications to vulnerabilities.
Compatibility with Libraries and Frameworks
Numerous popular libraries and frameworks like Spring Boot, Hibernate, and JUnit have already adopted Java 11, which may lead to compatibility issues if you remain on older versions.
Future-Proofing Your Applications
As you look to the future, upgrading your Java version means better alignment with new technologies, frameworks, and tools. The Java community quickly adapts to new versions, meaning that resources, libraries, and support for Java 11 will only grow.
Migration Ease
Upgrading to Java 11 might seem daunting, but in reality, it often requires fewer changes than expected. By carefully reviewing deprecations and adopting new APIs, many teams find they can complete the migration smoothly.
Bringing It All Together
Java 11 is not just a version — it's a focal point for current and future application development. Its performance enhancements, modern features, improved security, and robust community support emphasize why the upgrade is necessary and advantageous.
If your codebase is still relying on older Java versions, the time for action is now. The myriad of benefits Java 11 offers not only strengthens your applications but also enhances developer productivity and satisfaction.
For additional information about upgrading to Java 11 and exploring all its functionalities, consider visiting OpenJDK or the official Oracle documentation.
Start converting your codebase today, and usher in a new era of efficiency and maintainability in your Java applications!
Checkout our other articles