Fixing Checkstyle Issues in IntelliJ with Maven Integration
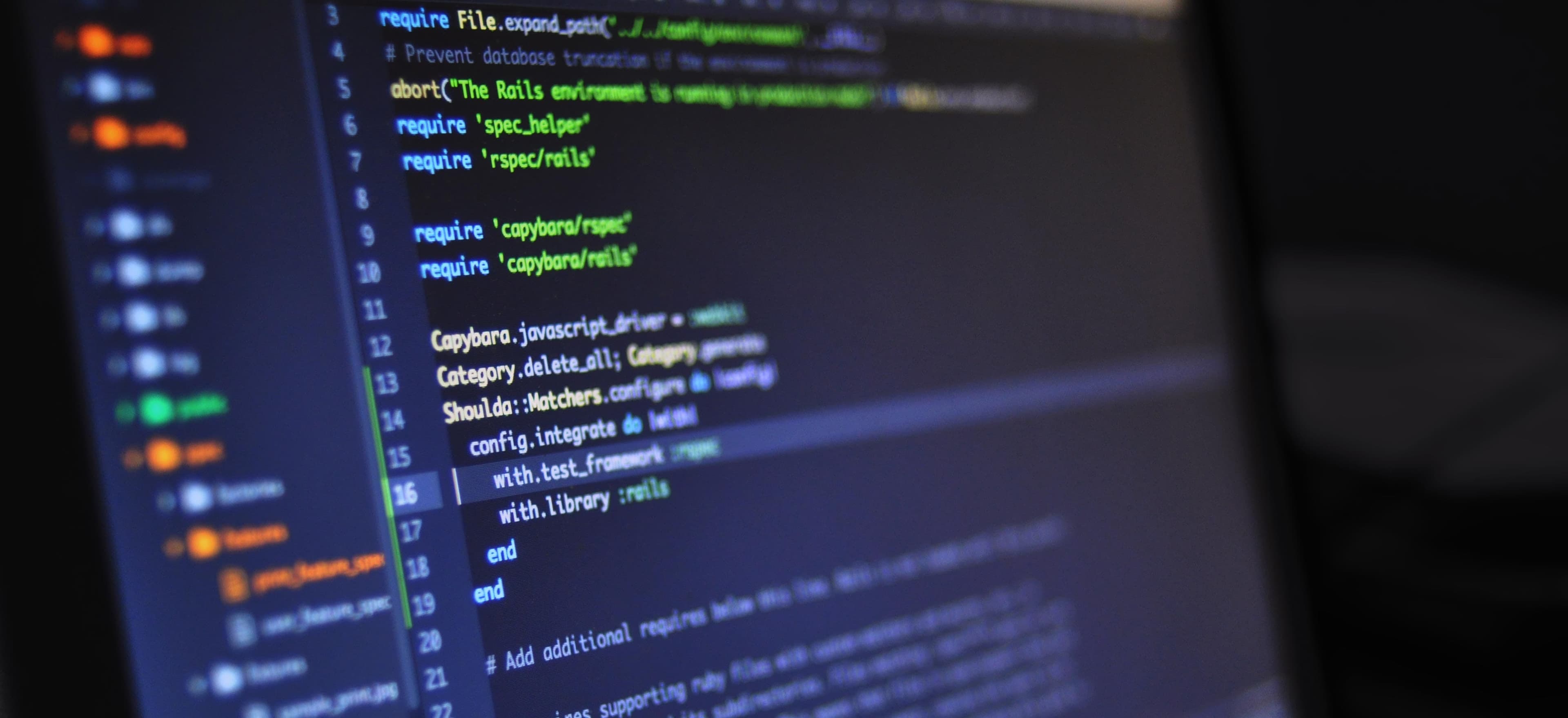
- Published on
Fixing Checkstyle Issues in IntelliJ with Maven Integration
Checkstyle is a powerful code analysis tool that helps developers adhere to coding standards in Java projects. When you're using IntelliJ IDEA in combination with Maven, it can streamline the process of maintaining code quality. In this blog post, we will discuss how to integrate Checkstyle into your Maven projects within IntelliJ and troubleshoot common issues.
What is Checkstyle?
Checkstyle is an open-source tool that checks Java code for adherence to a defined set of coding rules. It helps developers catch potential bugs, enforces best practices, and enhances the overall readability of the code. You can create your own rules or use built-in configurations.
Setting Up Checkstyle with Maven in IntelliJ
Before diving into issues that may arise, let’s start with setting up Checkstyle in your Maven project in IntelliJ.
Step 1: Add Checkstyle Plugin to Your Maven Project
Open your pom.xml
file and add the Checkstyle plugin under the <plugins>
section. Here is how your pom.xml
might look:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<!-- other configurations -->
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.2</version> <!-- Check for the latest version -->
<configuration>
<configLocation>checkstyle.xml</configLocation> <!-- Checkstyle configuration file -->
<outputDirectory>target/checkstyle</outputDirectory>
</configuration>
</plugin>
</plugins>
</build>
</project>
Why This Configuration Matters
- Version: It's essential to specify the latest version of the Checkstyle plugin to utilize recent features and fixes.
- Config Location: This points to your Checkstyle configuration file. You can create a custom
checkstyle.xml
or use a predefined one. - Output Directory: This defines where the Checkstyle reports will be stored.
Step 2: Create or Obtain a Checkstyle Configuration File
You can find predefined configuration files in Checkstyle's official repository. For instance, you might want to use the Sun Checks or Google Checks configuration file.
You can add a custom checkstyle.xml
file to the root of your project:
<!DOCTYPE module PUBLIC
"-//Checkstyle//DTD Checkstyle Configuration 1.3//EN"
"https://checkstyle.sourceforge.io/dtds/configuration_1_3.dtd">
<module name="Checker">
<module name="TreeWalker">
<module name="WhitespaceAround"/>
<module name="EmptyLineSeparator"/>
<module name="FileTabCharacter"/>
<!-- Add more rules as necessary -->
</module>
</module>
Why Use a Custom Configuration?
By customizing your Checkstyle configuration, you can enforce your team's coding standards while ignoring rules that may not be relevant for your specific project. This flexibility makes Checkstyle a vital tool in any development pipeline.
Step 3: Run Checkstyle in IntelliJ
To run Checkstyle in IntelliJ, you can do the following:
- Open your IntelliJ IDEA.
- Navigate to the Maven tool window (usually on the right side).
- Find your project under "Lifecycle" and look for "checkstyle:check".
- Click on "checkstyle:check" to execute the Checkstyle analysis.
Common Issues and How to Fix Them
Now that we have integrated Checkstyle into our Maven project, let's discuss some common issues you might encounter and how to resolve them.
Issue 1: Plugin Not Found
One of the common issues developers face is the "Plugin not found" error. This typically indicates that the Maven repository cannot find the specified plugin.
Solution:
Ensure you have the correct Maven repository settings in settings.xml
. If you are behind a corporate firewall, you may need to add a proxy configuration to your settings.xml
.
Issue 2: Configuration File Not Found
Another frequent problem is the inability to find the specified checkstyle.xml
configuration.
Solution:
- Ensure that the file path in the
configLocation
tag is accurate. - If your configuration file is not in the root directory, you need to adjust the path accordingly, such as
src/main/resources/checkstyle.xml
.
Issue 3: Checkstyle Violations Not Showing in IntelliJ
If you've run Checkstyle but do not see any results or feedback on code violations within IntelliJ, you might be missing some configurations.
Solution:
Check if the "Checkstyle" plugin is installed in IntelliJ:
- Go to File > Settings > Plugins.
- Search for "Checkstyle" and ensure it's enabled. If not, install and restart IntelliJ IDEA.
Comprehensive Checkstyle Reports
After running the Checkstyle analysis, results are generated in the specified output directory. You can check the HTML report (if you have set up HTML output) for a more user-friendly view.
Here's how to enable the HTML report in your configuration:
<reporting>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.2</version>
<configuration>
<outputFormat>xml</outputFormat>
<outputDirectory>${project.build.directory}/checkstyle-reports</outputDirectory>
</configuration>
<executions>
<execution>
<id>checkstyle-report</id>
<phase>prepare-package</phase>
<goals>
<goal>checkstyle</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</reporting>
Why HTML Reports?
HTML reports provide an easy-to-read format for developers and project stakeholders, allowing them to quickly assess code quality without diving into raw data.
Closing the Chapter
By integrating Checkstyle into your IntelliJ environment with Maven, you can streamline your Java coding practices and ensure adherence to predefined coding standards. Throughout this blog post, we've examined the setup process, identified potential issues, and provided solutions.
Maintaining code quality is important for team collaboration and software sustainability. As your project grows, continually adjusting and refining the Checkstyle configuration can lead to better coding practices across the board.
For further reading and resources, be sure to check out Checkstyle's Official Documentation and Maven Checkstyle Plugin Documentation. Integrating tools like Checkstyle not only enhances code quality but also fosters a culture of excellence in software development.
Happy coding!
Checkout our other articles