Optimizing Time Measurement Between Java and Kernel
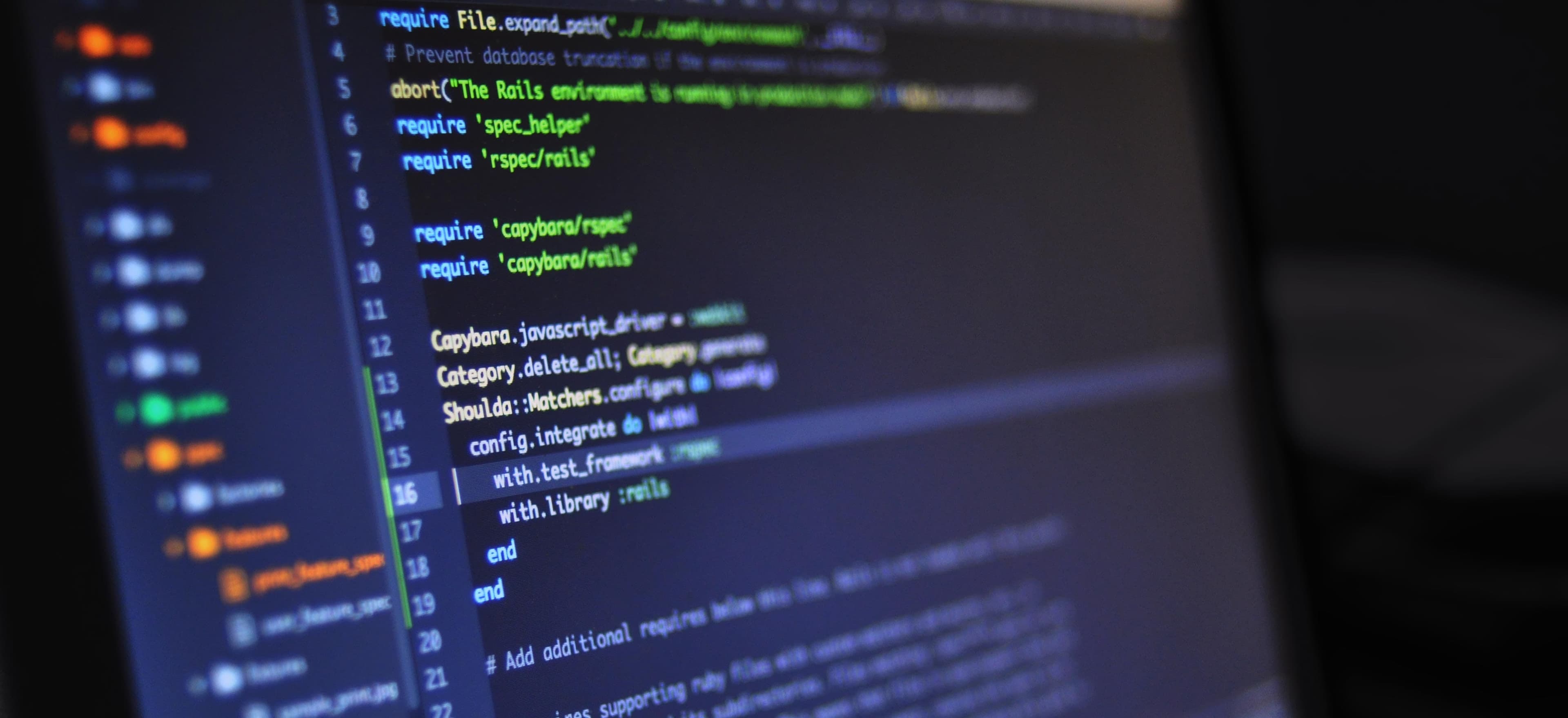
- Published on
Optimizing Time Measurement Between Java and Kernel
Performance measurement is a critical aspect of software development. In Java, accurate measurement of execution time can significantly impact performance tuning and the identification of bottlenecks. However, Java typically operates in a high-level virtual environment, meaning measurements can be affected by the Java Virtual Machine (JVM) and other abstractions. Therefore, understanding how to optimize time measurement between Java and the operating system kernel can help developers get more reliable performance metrics.
Understanding Time Measurement: A Brief Overview
Time measurement in Java involves evaluating how long a particular piece of code takes to execute. The primary methods you might use include:
- System.nanoTime()
- System.currentTimeMillis()
- Java 8's Instant
However, these functions retrieve time at different levels of granularity and accuracy.
System.nanoTime()
: This method is generally recommended for measuring performance due to its nanosecond precision.System.currentTimeMillis()
: While this provides millisecond precision, it's not ideal for fine-grained performance measuring, as it is subject to system clock updates.Instant
: Introduced in Java 8,Instant
offers a more modern approach to time management and is especially useful in conjunction with other time-related classes.
The Importance of Kernel-Level Timing
The kernel plays a crucial role in how Java and other applications are scheduled and executed. To optimize performance measurement, it’s essential to consider the interaction between Java applications and the operating system kernel.
Advantages of Kernel-Level Timing
- Precision: Kernel-level functions can provide more accurate time measurements than Java's specific methods due to less overhead.
- Context: System-level timings can help visualize the entire execution process, including context switches and scheduling delays.
- Cross-Language Benchmarks: You can bridge measurements across languages, making comparisons more straightforward.
Code Example: Using System.nanoTime()
To measure elapsed time in Java code, we can encapsulate our timing into a reusable method. Here’s an example:
public class PerformanceMeasurer {
public static long measureExecutionTime(Runnable task) {
long startTime = System.nanoTime(); // Start timing
task.run(); // Execute the task
return System.nanoTime() - startTime; // Calculate elapsed time
}
public static void main(String[] args) {
long timeTaken = measureExecutionTime(() -> {
// Simulate a time-consuming task
for (int i = 0; i < 1000000; i++) {
Math.sqrt(i);
}
});
System.out.println("Time taken: " + timeTaken + " nanoseconds");
}
}
Why This Code Works
- Precision with System.nanoTime(): The method offers high precision by measuring time in nanoseconds.
- Runnable Interface: It allows us to pass any code as a task, making the method versatile.
- Encapsulation: The timing logic is encapsulated within a reusable method, adhering to DRY (Don't Repeat Yourself) principles.
Integrating Kernel-Level Timing
To harness kernel-level timing, one could use native code via JNI (Java Native Interface). This might involve writing C or C++ to access high-resolution performance counters. Here’s a simplified C example of how high-resolution timing might look:
#include <time.h>
long long getHighResolutionTime() {
struct timespec ts;
clock_gettime(CLOCK_MONOTONIC, &ts);
return ts.tv_sec * 1e9 + ts.tv_nsec; // Convert to nanoseconds
}
Considerations for JNI Integration
- Complexity: JNI adds an additional layer of complexity, making it more susceptible to bugs if not handled carefully.
- Overhead: Invoking native methods can introduce overhead, potentially negating lower timing resolution gains.
If you are interested in more on JNI, check out the official documentation.
Best Practices for Time Measurement
1. Warm-Up the JVM
JVM optimizations can skew measurements. It's wise to execute your code several times before measurement to ensure that JIT compilation optimizations occur.
2. Avoid Measuring Small Code Segments
Measuring tiny code fragments may yield misleading results. Instead, aggregate the measurements for longer-running processes to average out inconsistencies.
3. Use Benchmarking Frameworks
Leveraging established benchmarking libraries like JMH (Java Microbenchmark Harness) provides a reliable way to measure performance. JMH handles many intricacies of micro-benchmarking, such as separate warm-up iterations and accurate measurement of execution time.
import org.openjdk.jmh.annotations.*;
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.MILLISECONDS)
public class MyBenchmark {
@Benchmark
public void testMethod() {
// Code to benchmark
for (int i = 0; i < 1000; i++) {
Math.random();
}
}
}
Conclusion
Optimizing time measurement between Java and the kernel can improve performance assessments and provide a clearer picture of code execution efficiency. Common Java methods like System.nanoTime()
offer a starting point, but developers should also consider kernel-level timing for critical evaluation.
Incorporating practices such as JVM warm-up, avoiding trivial measurements, and using established benchmarking frameworks like JMH can enhance the reliability and credibility of performance further.
By understanding both the Java and kernel sides of time measurement, developers can create more efficient applications, leading to an overall improvement in user experience and system performance.
For further reading on performance benchmarks and optimizations in Java, check out Java Performance: The Definitive Guide by Scott Oaks.
Happy coding!