Configuration Mistakes That Sabotage Your Test Cleanliness
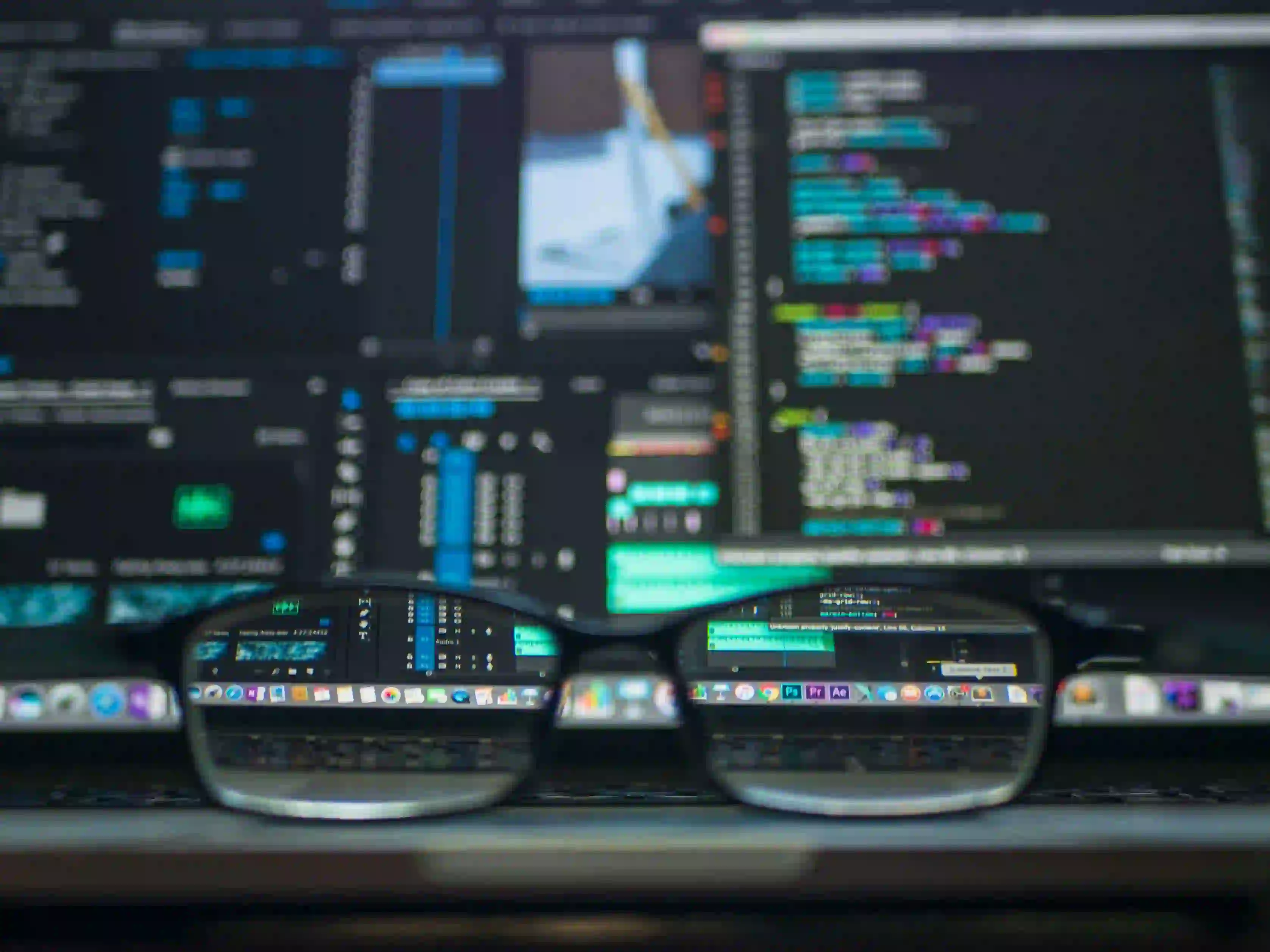
Configuration Mistakes That Sabotage Your Test Cleanliness in Java
Testing is an integral part of software development, ensuring that applications function as expected and remain resilient to changes. The cleanliness of these tests is vital; any configuration mistakes can lead to inconsistent results, wasted time, and ultimately, flawed software. In this blog post, we will explore common configuration mistakes in Java testing that can undermine the quality and reliability of your tests.
Understanding Test Cleanliness
Before delving into mistakes, let's define what we mean by "test cleanliness." Clean tests are:
- Isolated: They do not rely on external systems that can introduce variability.
- Repeatable: They yield the same results every time, under the same conditions.
- Maintained: They are easy to modify and adapt as the application evolves.
Achieving test cleanliness allows for more effective automated testing, thus improving the overall development process.
Common Configuration Mistakes
1. Poor Dependency Management
Java projects often use dependency management tools like Maven or Gradle. Misconfigurations in your pom.xml
(for Maven) or build.gradle
(for Gradle) files can lead to inconsistent test environments.
Example: Maven Dependency Scope
Consider the following snippet from a pom.xml
file:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
It's crucial to set the correct scope for dependencies. Using the wrong scope can lead to missing dependencies during testing, resulting in runtime errors that obscure the actual problem areas.
Why this matters: The test scope means that JUnit will only be available in the test lifecycle. Misconfiguring it can lead to using a non-test version causing failures in your tests.
2. Shared Resources
One of the quickest ways to jeopardize test cleanliness is by sharing resources across tests. This can lead to flaky tests—those that pass or fail inconsistently.
Example: Using Static Resources
Suppose you have a static variable in a test class:
public class DatabaseConnectionTest {
private static DatabaseConnection connection;
@BeforeClass
public static void setup() {
connection = new DatabaseConnection("test_database");
}
@Test
public void testQuery() {
String result = connection.query("SELECT * FROM users");
assertNotNull(result);
}
}
In this example, the static connection
could retain state across multiple tests, leading to false positives or negatives.
Why this matters: Isolating tests ensures that each one runs independently. Utilizing shared state can introduce hidden dependencies, leading to unreliable results.
3. Not Using a Test Profile
Failing to create a distinct test profile often results in the code using production settings during testing.
Example: Spring Profile Configuration
For Spring applications, you can define a specific profile for tests:
# application-test.yaml
spring:
datasource:
url: jdbc:h2:mem:testdb
driver-class-name: org.h2.Driver
jpa:
hibernate:
ddl-auto: update
show-sql: true
When you fail to specify the profile during testing, you might inadvertently connect to a real database instead of a mock or in-memory database.
Why this matters: Production database changes can affect testing outcomes. A dedicated profile allows you to use a controlled environment, ensuring that tests do not impact production data and vice-versa.
4. Ignoring Configuration Files
Overlooking or improperly configuring properties files can cause tests to fail without an apparent reason.
Example: property file loading in Java
A property file might look like this:
# config.properties
database.url=jdbc:h2:mem:testdb
database.username=test
database.password=password
Misconfiguration in loading this properties file in tests can lead to the application not being able to connect to the data source.
Why this matters: Consistently loading the correct configuration files ensures that tests can execute operations as expected.
5. Lack of Test Data Management
In many cases, tests need to have a predictable state or predefined data to work with. Neglecting to set up or tear down data properly can lead to inconsistencies.
Example: Setting Up Test Data
You might want to reset a database before running each test:
@Before
public void setUp() {
database.clearTables();
database.insert("test_user");
}
By ensuring that the database is in a known state before each test, you can avoid carryover from previous tests.
Why this matters: Test data setup and teardown keep tests isolated and consistent, helping achieve maximal test cleanliness.
Best Practices for Test Cleanliness
Use Mocking Frameworks
Frameworks like Mockito can help isolate tests by allowing you to mock dependencies that interact with external systems, such as databases or REST APIs.
public class UserServiceTest {
@Mock
private UserRepository userRepository;
private UserService userService;
@Before
public void setUp() {
MockitoAnnotations.openMocks(this);
userService = new UserService(userRepository);
}
@Test
public void testRetrieveUser() {
when(userRepository.findById(1)).thenReturn(new User(1, "John Doe"));
User user = userService.retrieveUser(1);
assertEquals("John Doe", user.getName());
}
}
Why this matters: Mocking makes it easier to focus on the class under test without worrying about external state or behavior.
Regularly Review Configuration Files
Config files should not be set and forgotten. Regular reviews help avoid unintentional errors.
Continuous Integration (CI)
Integrate your tests into CI pipelines to ensure that they execute reliably whenever there's a change in the codebase.
To Wrap Things Up
Maintaining test cleanliness is essential for achieving stable and reliable software. Configuration mistakes can easily sabotage your testing efforts, leading to false results and increased time spent debugging. By avoiding these common pitfalls—poor dependency management, shared resources, inadequate profile usage, ignoring configuration files, and mismanaging test data—you can enhance the quality of your tests.
For more on effective Java testing practices, consider checking out JUnit Testing Basics and Best Practices in Mocking.
By leveraging automation and best practices, you can maximize your testing efficiency and maintain clean, reliable tests in your Java applications.