Make Your Code Testable: Simple Tips for Better Quality
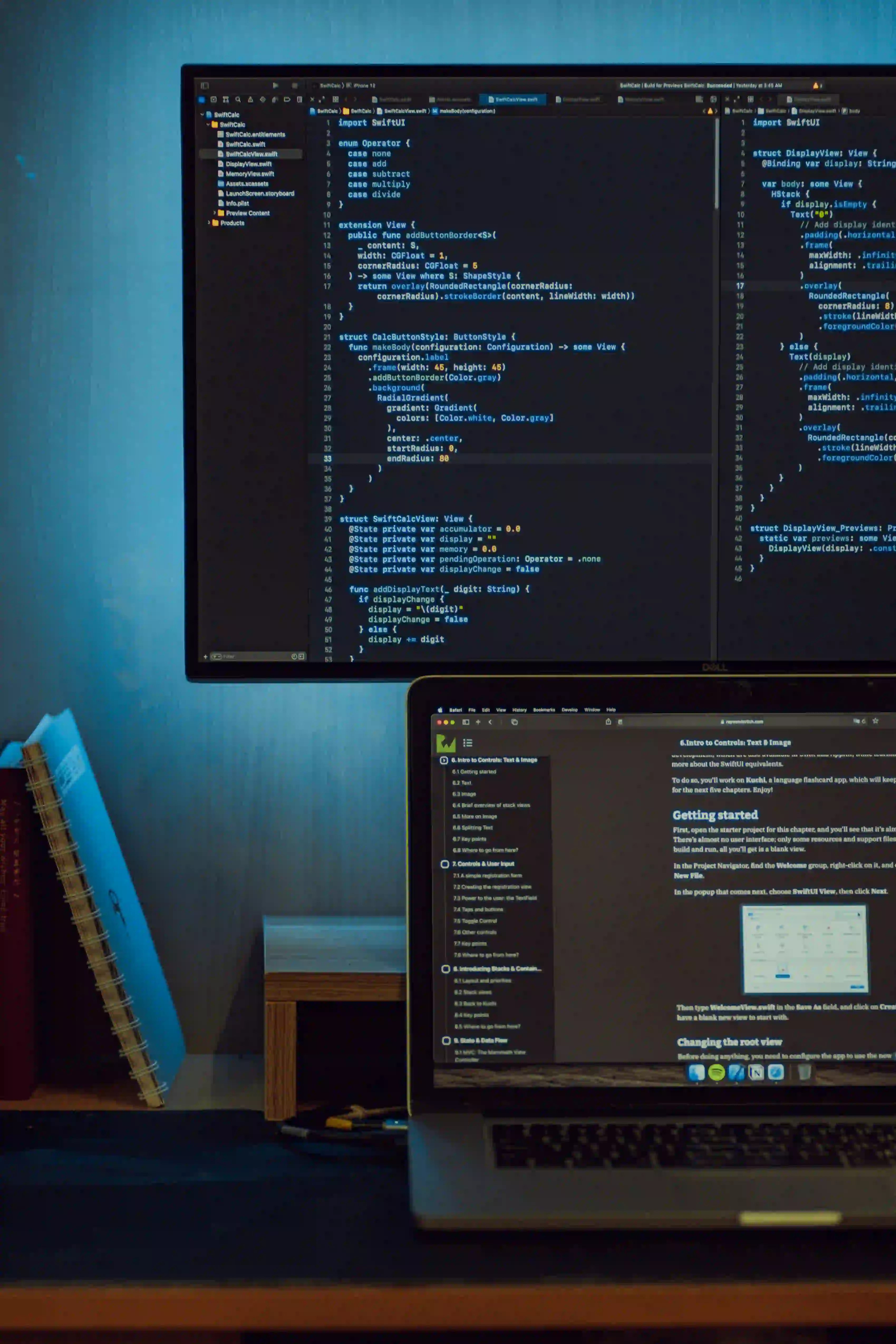
Make Your Code Testable: Simple Tips for Better Quality
Writing code is a fundamental skill for software developers. However, the quality of that code is what ultimately determines the success of any software project. One of the keys to ensuring high-quality code is making it testable. Testable code allows for easier debugging, maintenance, and overall better performance. In this post, we’ll explore some simple tips to enhance the testability of your Java code.
Table of Contents
- Understanding Testability
- Follow Single Responsibility Principle
- Use Dependency Injection
- Write Unit Tests Concurrently
- Embrace Mocking and Stubbing
- Conclusion
Understanding Testability
Testability refers to the ease with which a piece of software can be tested to ensure it behaves as expected. High testability often leads to fewer defects and higher quality code. But how do we achieve this testability in our code? Let’s delve into some practical tips that will improve your Java programming.
Follow Single Responsibility Principle
The Single Responsibility Principle (SRP) is one of the five SOLID principles of object-oriented design. According to SRP, a class should only have one reason to change, meaning it should have only one responsibility.
Code Example
public class UserService {
public void registerUser(User user) {
// registration logic
}
// Violates SRP
public void logRegistration(User user) {
// logging logic
}
}
In the above code, the UserService
class has two responsibilities: managing user registration and logging. This design complicates unit testing as we have intertwined logic.
Revised Code
public class UserService {
private final LoggerService loggerService;
public UserService(LoggerService loggerService) {
this.loggerService = loggerService;
}
public void registerUser(User user) {
// registration logic
loggerService.logRegistration(user);
}
}
public class LoggerService {
public void logRegistration(User user) {
// logging logic
}
}
By separating the logging functionality into its own LoggerService
class, we simplify testing. This class can now be easily mocked when testing UserService
.
Why This Matters
- Enhanced Readability: Each class has a well-defined purpose.
- Simpler Unit Tests: Less complexity means writing tests becomes more straightforward.
For more information on SOLID principles, check out this article on SOLID Principles.
Use Dependency Injection
Dependency Injection (DI) is a design pattern used to implement IoC (Inversion of Control). This practice allows for better management of dependencies and improves testability.
Code Example
Instead of creating instances of dependencies within your class, use DI to inject them.
public class NotificationService {
private final EmailService emailService;
public NotificationService(EmailService emailService) {
this.emailService = emailService;
}
public void notifyUser(User user) {
emailService.sendEmail(user);
}
}
Why This Matters
- Loose Coupling: Classes are not tightly linked, making swapping or mocking dependencies effortless.
- Easier Testing: You can inject mock services during testing, allowing for isolated tests.
Write Unit Tests Concurrently
Another way to improve your code's testability is to write your unit tests as you go. This encourages better design choices and helps in maintaining a test-first mindset.
Example of a Simple Unit Test
Using JUnit, we can quickly create a unit test for our NotificationService
.
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
public class NotificationServiceTest {
private NotificationService notificationService;
private EmailService emailService;
@BeforeEach
public void setUp() {
emailService = mock(EmailService.class);
notificationService = new NotificationService(emailService);
}
@Test
public void testNotifyUserSendsEmail() {
User user = new User("test@example.com");
notificationService.notifyUser(user);
verify(emailService).sendEmail(user);
}
}
Why This Matters
- Immediate Feedback: Writing tests concurrently allows for immediate validation of new features.
- Higher Code Quality: You are more conscious of writing test-friendly code.
Embrace Mocking and Stubbing
Mocking and stubbing are crucial when writing tests. They allow you to isolate the unit of work without pulling in external dependencies.
Using Mockito
Mockito is a popular mocking framework for Java. Here’s how you can use it effectively.
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testRegisterUserWithMock() {
LoggerService mockLogger = mock(LoggerService.class);
UserService userService = new UserService(mockLogger);
User user = new User("testUser");
userService.registerUser(user);
verify(mockLogger).logRegistration(user);
}
}
Why This Matters
- Isolation: Your tests can focus on the logic of the unit under test, rather than the complexity of its interactions.
- Performance: Executing tests that don’t rely on actual external services is faster.
To Wrap Things Up
Making your Java code testable is not just beneficial but essential for ensuring quality software. By following the Single Responsibility Principle, using Dependency Injection, writing tests alongside your code, and embracing mocking, you are setting a robust foundation for a maintainable and resilient codebase.
Testable code ensures that as your software evolves, the risk of introducing bugs is minimized. Hopefully, these tips provide you with a clearer roadmap to write better, more testable code. Remember, the goal isn’t just to write code that works, but code that can be efficiently validated and maintained over time.
Want to explore nuanced testing strategies further? Check out JUnit 5 User Guide for comprehensive details.
Happy coding!