Struggling with Connectivity in Android IoT App Development?
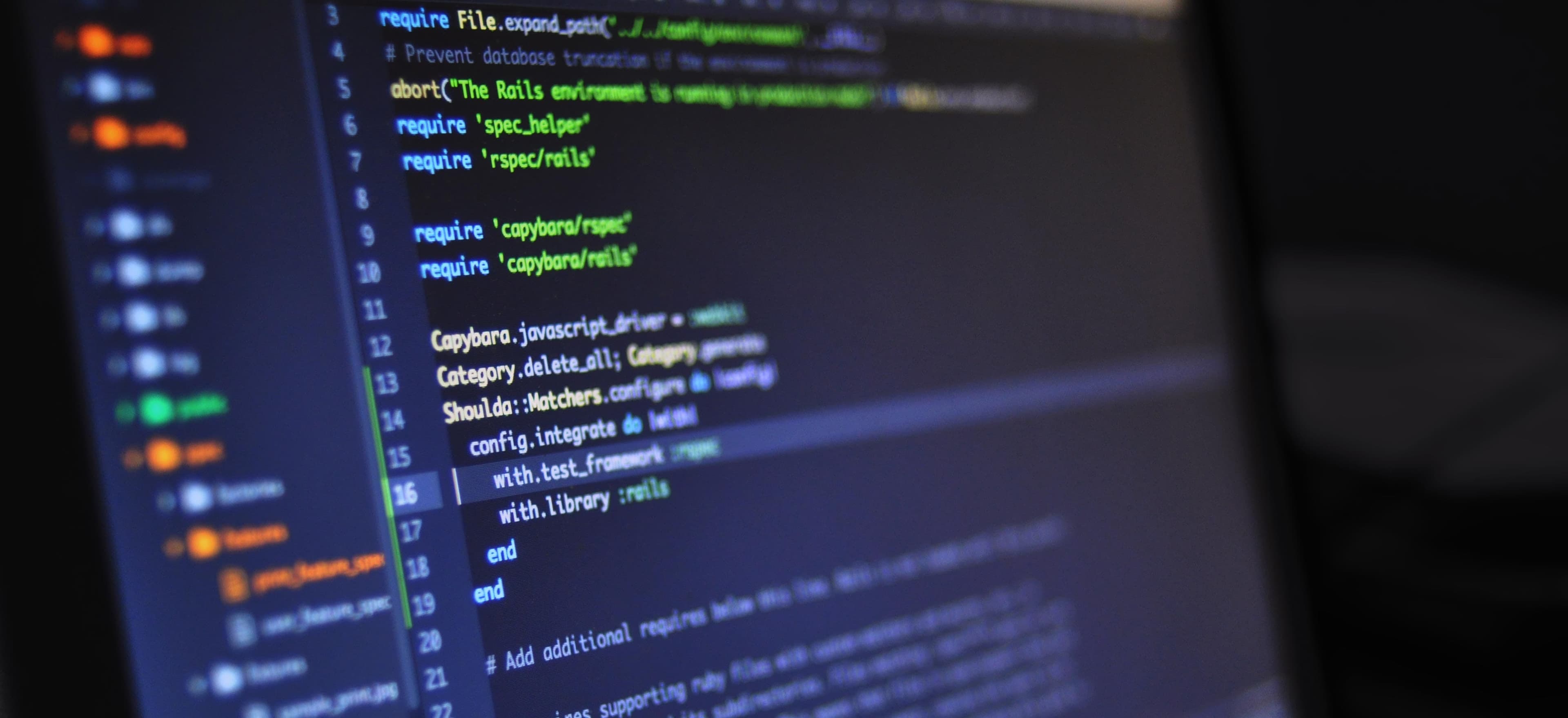
- Published on
Struggling with Connectivity in Android IoT App Development?
In the era of an interconnected world, the Internet of Things (IoT) has become instrumental in transforming our lives. Particularly for Android developers, creating robust IoT applications has emerged as a vital skill. However, one of the most common challenges developers face is managing connectivity. This blog aims to demystify connectivity issues in Android IoT app development and provide strategies to manage these challenges effectively.
Understanding Connectivity in IoT
Connectivity in IoT revolves around how devices communicate and share data. In the realm of Android, this entails creating applications that interact with various devices, whether they are sensors, smart appliances, or gateways. Various protocols and technologies, such as MQTT, HTTP, or WebSocket, serve different use cases. Therefore, understanding these is key to developing stable and efficient applications.
Why Connectivity Matters
Successful IoT applications depend on reliable communication between devices and the user interface. Without steady connectivity, users may experience delays, data loss, or even complete malfunctions. Effective connectivity ensures seamless data transmission, making your application responsive and user-friendly.
Common Connectivity Solutions in Android IoT
1. Wi-Fi Connectivity
Wi-Fi networks offer high-speed connections and easy integration with Android devices. Most smart devices utilize Wi-Fi for communication. Given its ubiquity, developers typically opt for Wi-Fi to facilitate broader device connectivity.
Example Code: Checking Wi-Fi Connectivity
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
public boolean isWiFiConnected(Context context) {
ConnectivityManager cm = (ConnectivityManager) context.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = cm.getActiveNetworkInfo();
return networkInfo != null && networkInfo.isConnected() && networkInfo.getType() == ConnectivityManager.TYPE_WIFI;
}
Why this works: This function checks the current network status. Using the ConnectivityManager
, it confirms whether the device is connected to Wi-Fi. The context
parameter allows the method to access system services. Understanding how to assess connectivity will help ensure that your app can provide value to users, even when they experience connectivity issues.
2. Bluetooth Connectivity
For short-range communication, Bluetooth is a preferred solution. It's suitable for applications requiring direct interaction with devices, such as wearables or home appliances.
Example Code: Discover Nearby Bluetooth Devices
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
public void discoverBluetoothDevices() {
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter != null && bluetoothAdapter.isEnabled()) {
bluetoothAdapter.startDiscovery();
registerReceiver(bReceiver, new IntentFilter(BluetoothDevice.ACTION_FOUND));
}
}
Why this works: This code snippet utilizes the BluetoothAdapter
to start discovering nearby devices. The broadcast receiver bReceiver
will help capture discovered devices. Bluetooth connectivity is critical for certain applications, highlighting the importance of being adept with various communication protocols.
3. Mobile Data Connectivity
In situations where Wi-Fi isn't available, mobile data can serve as an alternative. While consuming more battery, it provides a fallback solution, ensuring users can still interact with the IoT application.
Example Code: Checking Mobile Data Status
public boolean isMobileDataEnabled(Context context) {
ConnectivityManager cm = (ConnectivityManager) context.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = cm.getNetworkInfo(ConnectivityManager.TYPE_MOBILE);
return networkInfo != null && networkInfo.isConnected();
}
Why this works: Similar to the Wi-Fi check, this code allows you to ascertain whether mobile data is active. It's vital to provide users insight into their connectivity options, enabling better interaction with your IoT app.
Advanced Connectivity Challenges
While managing basic connectivity is essential, developers often face advanced challenges, such as scalability, latency, and data synchronization. Here are a few strategies to address these:
1. Employing MQTT Protocol
MQTT (Message Queuing Telemetry Transport) is a lightweight messaging protocol ideal for IoT applications, particularly where a large number of devices are involved. It offers minimal overhead, enabling quicker message delivery.
Example Code: Basic MQTT Setup
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttException;
public void connectToMqttBroker() {
try {
MqttClient client = new MqttClient("tcp://broker.hivemq.com:1883", MqttClient.generateClientId());
client.connect();
} catch (MqttException e) {
e.printStackTrace();
}
}
Why this works: This example demonstrates how to connect to an MQTT broker. MQTT’s publish/subscribe model allows numerous devices to communicate efficiently. By adopting MQTT, developers can ensure that their applications handle high volumes of data with minimal latency.
2. Implementing Offline Capability
A reliable IoT application should maintain functionality even when connectivity is lost. Implementing local caching or using SQLite can help store data temporarily until the connection is restored.
Example Code: Basic SQLite Setup
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put("key", "value");
db.insert("your_table_name", null, values);
Why this works: This snippet demonstrates how to insert data into an SQLite database. Offline capabilities create a user-friendly experience that mitigates frustrations during connectivity lapses.
Testing Connectivity
Testing connectivity is a crucial step that developers often overlook. Tools such as Postman for API testing and real devices for Bluetooth and Wi-Fi testing can help identify issues before launching your application.
Mocking Connectivity Scenarios
Using Android's tools to mock connectivity states is indispensable in testing. The Android Emulator allows you to simulate network conditions such as slow data connections or no connectivity, enabling comprehensive testing of your application's behavior.
Example Code: Mocking Network Condition
// This is done through Android Studio's emulator settings.
Why this matters: By testing under varied connectivity conditions, developers can ensure their applications behave predictably and remain user-friendly, even in challenging environments.
Final Considerations
Connectivity in Android IoT app development presents a unique range of challenges. However, armed with the right knowledge and tools, developers can create applications that stand the test of time and provide users with seamless experiences. The blend of Wi-Fi, Bluetooth, mobile data, and advanced protocols like MQTT forms a powerful toolkit for any Android IoT developer.
Next Steps
To deepen your understanding, consider exploring additional resources:
Embrace these techniques and keep refining your skills. The world of IoT is vast and filled with opportunities waiting to be unlocked!
Checkout our other articles