Overcoming Common JavaFX Game Development Pitfalls
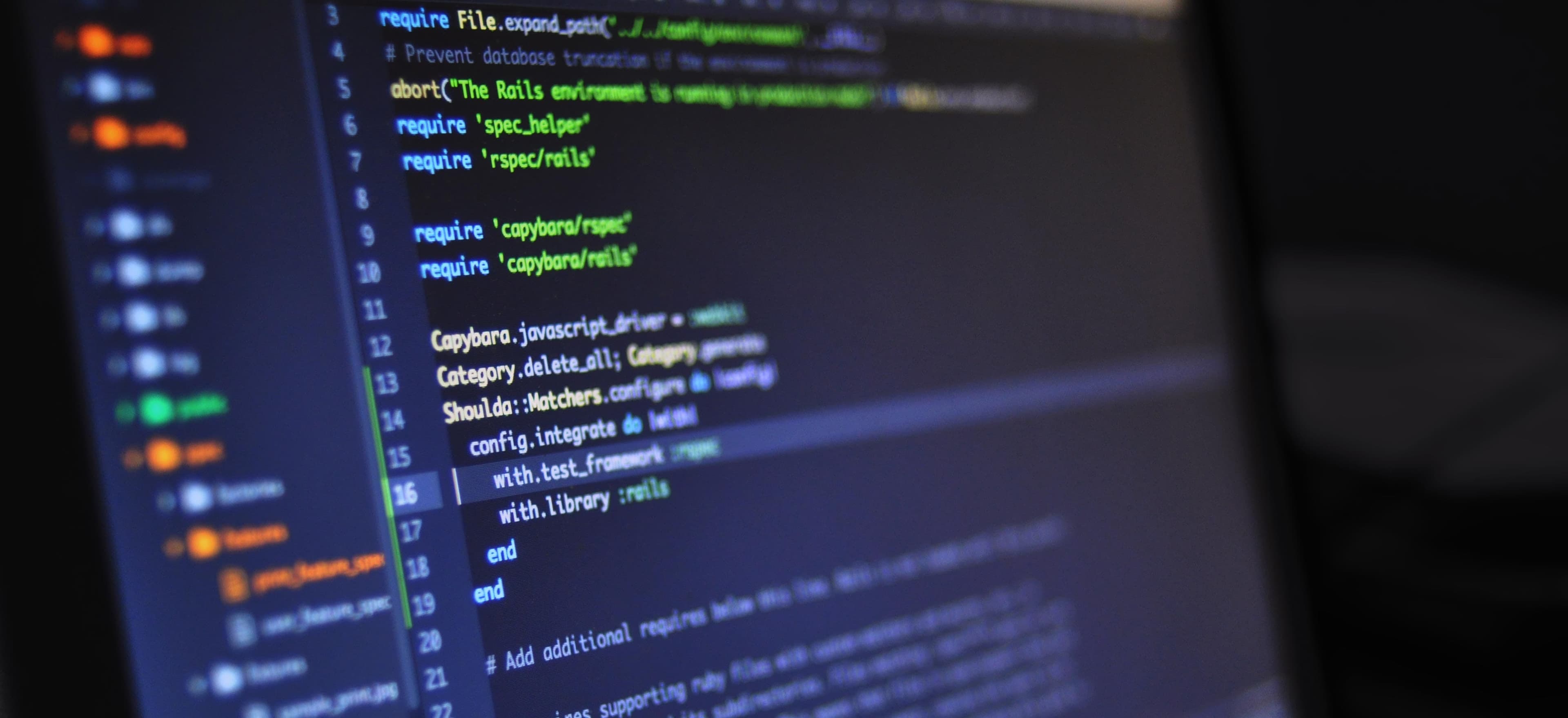
- Published on
Overcoming Common JavaFX Game Development Pitfalls
Game development is an exciting venture that requires creativity, logical thinking, and technical skills. For Java developers, JavaFX provides a powerful platform to design and build engaging games. However, it's not without its challenges. In this post, we will discuss some common pitfalls in JavaFX game development and how to overcome them.
Why JavaFX for Game Development?
JavaFX is a rich framework designed for building desktop applications and user interfaces. While its primary focus is not on game development, its features, such as 2D and 3D graphics support, animation capabilities, and a robust scene graph, make it suitable for creating visually appealing games. If you're interested in exploring JavaFX further, check out the official documentation for more insights.
Common Pitfalls and Solutions
1. Resource Management
Pitfall: New developers often struggle with resource management—loading and using assets such as images, sounds, and fonts. Improper management can lead to memory leaks and performance issues.
Solution: Use a singleton pattern for your assets manager. Preload assets at the start and use them throughout the game. Below is an example of a simple asset manager that demonstrates how to load and retrieve images efficiently.
import javafx.scene.image.Image;
import java.util.HashMap;
public class AssetManager {
private static AssetManager instance;
private HashMap<String, Image> images;
private AssetManager() {
images = new HashMap<>();
}
public static AssetManager getInstance() {
if (instance == null) {
instance = new AssetManager();
}
return instance;
}
public Image getImage(String path) {
if (!images.containsKey(path)) {
images.put(path, new Image(path));
}
return images.get(path);
}
}
// Usage
Image playerImage = AssetManager.getInstance().getImage("path/to/player.png");
This approach minimizes the loading time and ensures that resources are managed properly throughout the game lifecycle.
2. Animation Performance
Pitfall: Another common mistake is not optimizing animations. JavaFX's animation framework is powerful, but if not properly handled, it can lead to laggy gameplay.
Solution: Use the Timeline
class for animations, which allows you to control the update rate. Below is an example illustrating how to create and manage an animation:
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.scene.shape.Rectangle;
import javafx.util.Duration;
public class GameAnimation {
private Rectangle player;
private Timeline timeline;
public GameAnimation(Rectangle player) {
this.player = player;
this.timeline = new Timeline(new KeyFrame(Duration.seconds(0.016), event -> update()));
timeline.setCycleCount(Timeline.INDEFINITE);
timeline.play();
}
private void update() {
// Add logic to update player position
player.setX(player.getX() + 1); // Simple movement logic
}
}
Using a consistent animation frame rate ensures smoother visuals and more responsive controls, which ultimately enhances the player's experience.
3. Managing Game State
Pitfall: Many developers overlook the importance of managing game states effectively. Without a proper state management system, transitioning between menus, gameplay, and game-over screens can become chaotic.
Solution: Implement a finite state machine (FSM) to clearly define and manage different game states. Here’s a basic structure you can use:
public enum GameState {
MENU,
PLAYING,
GAME_OVER
}
public class Game {
private GameState currentState;
public Game() {
currentState = GameState.MENU;
}
public void setState(GameState state) {
currentState = state;
// Adjust UI and game mechanics based on state
switch (currentState) {
case MENU:
showMenu();
break;
case PLAYING:
startGame();
break;
case GAME_OVER:
showGameOverScreen();
break;
}
}
private void showMenu() {
// Logic to display the menu
}
private void startGame() {
// Logic to start the game
}
private void showGameOverScreen() {
// Logic to display game-over screen
}
}
Using an FSM makes it easier to manage transitions and ensures that the game behaves predictably.
4. Handling Input
Pitfall: Failing to properly manage input can cause frustration for players. Improperly handled events may lead to unresponsive controls or unexpected behavior.
Solution: Utilize JavaFX event listeners effectively. Here’s a basic example demonstrating how to handle keyboard input:
import javafx.scene.Scene;
import javafx.scene.input.KeyCode;
public void setupInput(Scene scene) {
scene.setOnKeyPressed(event -> {
if (event.getCode() == KeyCode.LEFT) {
// Move player left
}
if (event.getCode() == KeyCode.RIGHT) {
// Move player right
}
});
}
Ensuring that input handling is responsive and intuitive keeps players engaged and satisfied.
5. Debugging and Performance Optimization
Pitfall: Not dedicating time to debugging and optimizing code can lead to frustrating gameplay experiences. Performance issues may go unnoticed until they impact user experience.
Solution: Use JavaFX's built-in profiling tools to identify bottlenecks. Additionally, make use of an efficient logging system to monitor gameplay behavior and issues. Here is a simple logging mechanism:
import java.util.logging.Logger;
public class GameLogger {
private static final Logger logger = Logger.getLogger(GameLogger.class.getName());
public static void logInfo(String message) {
logger.info(message);
}
public static void logError(String message) {
logger.severe(message);
}
}
By continually profiling and logging, you can catch issues early and optimize your game for better performance.
A Final Look
Game development with JavaFX presents unique challenges, but by being aware of common pitfalls and utilizing effective strategies, you can create engaging and smooth-running games. Remember to manage your assets properly, optimize animations, structure your game state efficiently, handle input gracefully, and prioritize debugging and optimization.
For additional resources, consider exploring Game Development with Java or the JavaFX Tutorials to deepen your understanding.
Happy coding, and may your game development journey in JavaFX be both successful and enjoyable!
Checkout our other articles