Common Issues After Upgrading to JMetro 5.2
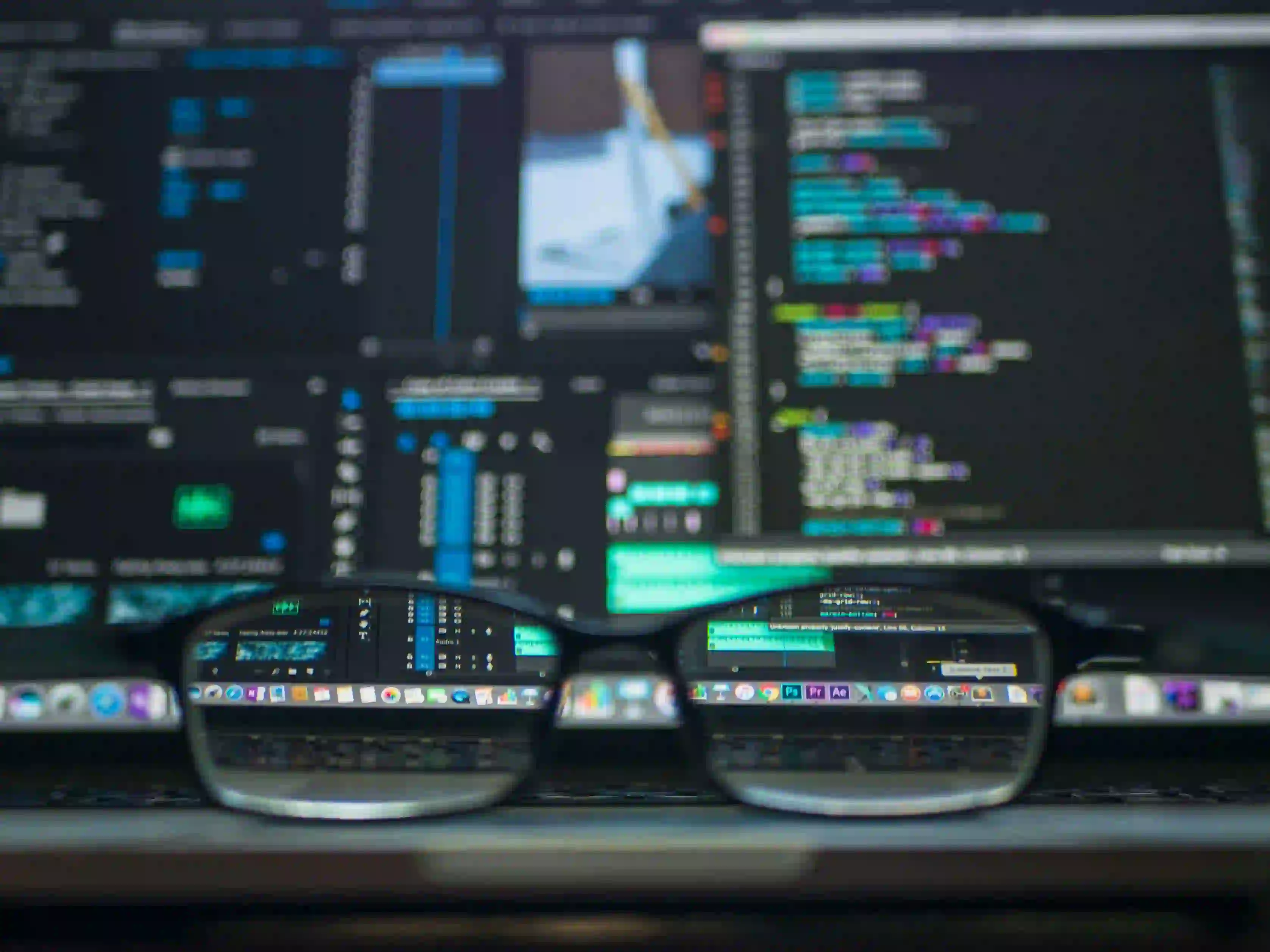
Common Issues After Upgrading to JMetro 5.2
Upgrading to a newer version of any software library can be an exciting yet daunting task. The recent upgrade to JMetro 5.2 from its previous version brings a plethora of enhancements tailored for improving user interface design with JavaFX applications. However, along with these upgrades come a set of common issues that developers may encounter. In this blog post, we'll explore these issues in detail while providing useful solutions and best practices for managing your JMetro integration.
What is JMetro?
Before diving into the issues, let’s clarify what JMetro is. JMetro is a library that provides a sleek Metro style look and feel for JavaFX applications. With a modern aesthetic, JMetro enhances JavaFX's default styles, making it visually appealing for Windows and other platforms. To learn more about its features, visit the JMetro GitHub repository.
Why Upgrade to JMetro 5.2?
The upgrade to JMetro 5.2 introduces new features, improvements, and bug fixes that can significantly improve your JavaFX applications. Some notable enhancements include:
- Improved styling options for buttons and sliders
- Enhanced support for dark and light themes
- Bug fixes from previous versions
Despite these advancements, not all transitions are smooth; hence, recognizing common issues during and after the upgrade is crucial for developers.
Common Issues After Upgrading to JMetro 5.2
1. Compatibility Problems
Issue: Applications built with earlier versions of JMetro may not display correctly after the upgrade. This can manifest in various ways, such as elements being improperly styled or missing altogether.
Solution: Review your JavaFX components and how they interact with JMetro’s new style sheets. Look for deprecated methods or properties that may have changed in version 5.2.
Code Snippet: Updating Component Styles
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class JMetroUpgradeExample extends Application {
@Override
public void start(Stage primaryStage) {
// Create a button
Button button = new Button("Click Me");
// Set style using JMetro
button.getStyleClass().add("jmetro-button"); // Ensure this is updated in JMetro 5.2
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("JMetro 5.2 Upgrade Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the above example, ensure you are using the correct style class names for your UI components, as they may have been renamed or restructured in the 5.2 update.
2. Missing Icons and Resources
Issue: After upgrading, you might find that some icons are missing or are not displaying as intended. This usually happens when resource paths have changed or when resource files aren't included in your build configuration.
Solution: Check your project’s resource paths and ensure that all necessary resources are referenced correctly.
Code Snippet: Managing Resources
<resources>
<resource>path/to/your/icons/*.png</resource>
<resource>path/to/your/styles/*.css</resource>
</resources>
In the pom.xml
file (for Maven users), you can specify the resources to ensure icons and stylesheets are included in the build.
3. Issues with Animation and Effects
Issue: Some developers report that animations and effects don't work as they did with previous versions. This could be due to changes in the underlying libraries affecting how animations are applied.
Solution: Review the animation code and see if any method parameters have been altered. It may require refactoring portions of your animation setup.
Code Snippet: Updating Animation Effects
import javafx.animation.PauseTransition;
import javafx.scene.control.Button;
import javafx.util.Duration;
Button button = new Button("Hover Me");
button.setOnMouseEntered(event -> {
// Start animation on hover
PauseTransition pause = new PauseTransition(Duration.seconds(1));
pause.setOnFinished(e -> button.setStyle("-fx-background-color: blue;"));
pause.play();
});
In this example, ensure that any animation effects you apply are compatible with JMetro 5.2.
4. Theme Switching Issues
Issue: When attempting to switch between light and dark themes, some users notice that certain components don't update immediately, leading to inconsistent UI states.
Solution: Implement a method to force the application to refresh its stylesheet dynamically.
Code Snippet: Dynamic Theme Switching
import javafx.scene.Scene;
public void switchTheme(Scene scene, String theme) {
scene.getStylesheets().clear(); // Remove existing stylesheets
scene.getStylesheets().add(getClass().getResource(theme).toExternalForm());
}
This function clears the existing styles and adds the selected theme’s stylesheets, ensuring an up-to-date application UI.
Wrapping Up
Upgrading to JMetro 5.2 unlocks new possibilities for enhancing your JavaFX application's interface, but it is not without challenges. From compatibility problems to resource management and animation issues, being aware of common pitfalls will enable developers to navigate the upgrade smoothly.
By following the suggestions and code snippets provided in this post, you can mitigate the risks associated with upgrading. Always refer to the official documentation for the latest updates and community support.
A successful upgrade is not just about the new features; it is also about understanding and resolving the issues that come along. Happy coding!