Top 5 Common Docker Issues and How to Fix Them Fast
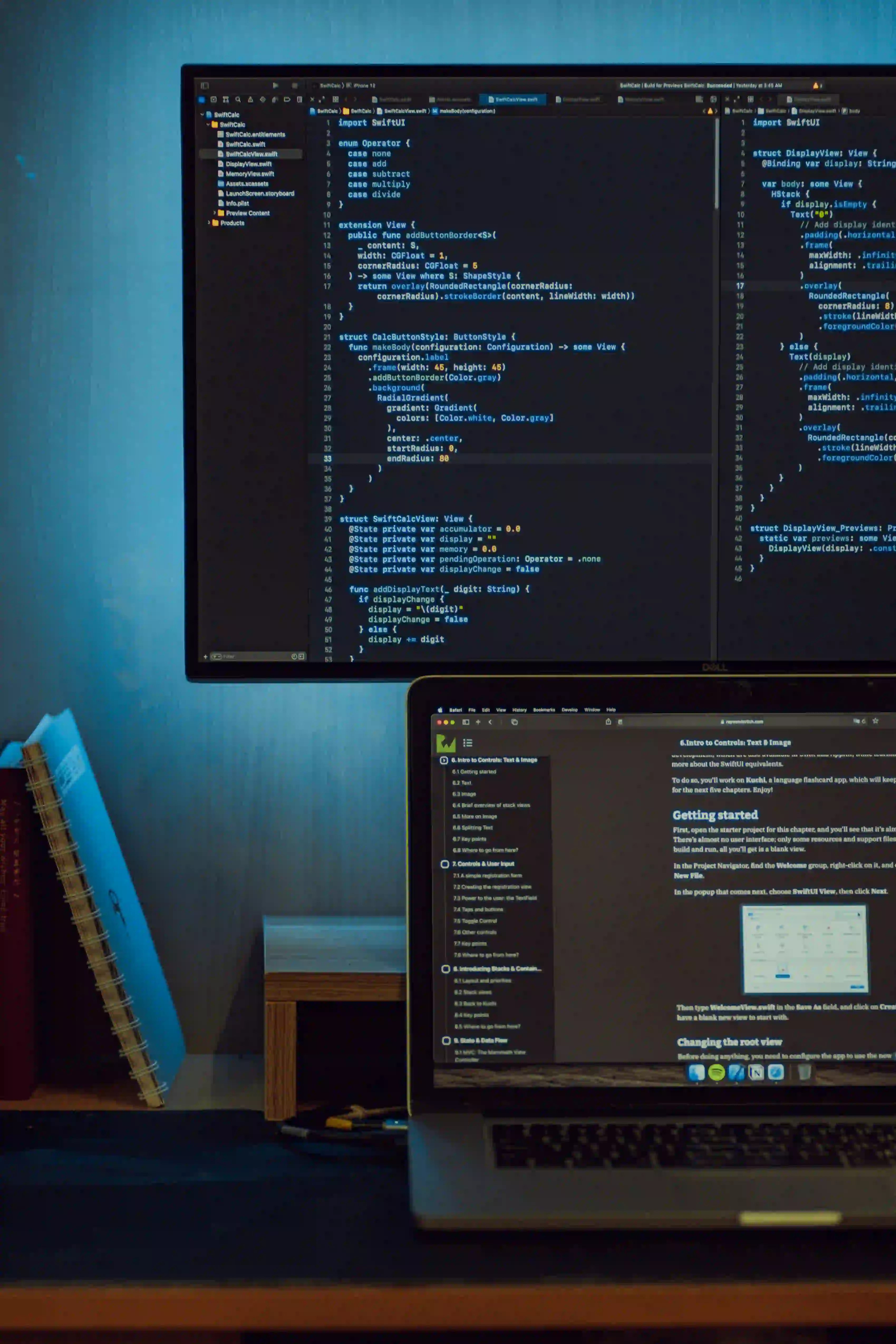
Top 5 Common Docker Issues and How to Fix Them Fast
Docker has revolutionized how we approach application deployment and management. Its containerization technology allows developers to package applications with their dependencies, ensuring that they work seamlessly across different environments. However, like any technology, Docker is not without its challenges. In this post, we’ll discuss the top five common Docker issues and how to resolve them effectively.
1. Image Pull or Build Failures
Problem:
One of the most frequent issues Docker users face is the failure to pull an image from a registry or build an image from a Dockerfile. This usually occurs due to network issues, incorrect image names, or problems with the Dockerfile syntax.
Solution:
To troubleshoot image pull or build failures, follow these steps:
-
Check Network Connectivity: Ensure that your machine has internet access and can reach Docker Hub or the specified image repository.
-
Verify Image Names: Double-check the image name and tag in your command. Use the following command to search for the correct image:
🔧snippet.shdocker search <image-name>
-
Review Dockerfile Syntax: Syntax errors in your Dockerfile can lead to build failures. Use
docker build
with the-t
option and review the output for error messages.
Example Dockerfile snippet:
FROM ubuntu:20.04
# Install dependencies
RUN apt-get update && apt-get install -y python3
# Set working directory
WORKDIR /app
# Copy application code
COPY . /app
# Run the application
CMD ["python3", "app.py"]
In this snippet, the syntax is straightforward. The RUN
command updates the package list and installs python3
, which is essential for running the app.
Resources:
For more information on Dockerfiles, visit Dockerfile Reference.
2. Container Exiting or Crashing
Problem:
Containers can exit unexpectedly for various reasons including application errors, insufficient memory, or misconfigurations of environment variables upon startup.
Solution:
When your container exits unexpectedly, take these steps:
-
View Container Logs: Use the following command to inspect logs:
🔧snippet.shdocker logs <container-id>
-
Allocate More Resources: Increase the memory or CPU allocated to your container. This can be done using the
docker run
command:🔧snippet.shdocker run -m 512m --cpus="1.0" <image-name>
-
Check Environment Variables: Misconfigured environment variables can lead to failures in your application. Verify that they are correctly set in your Dockerfile or during runtime.
Example Log Command:
When you want to see the last few lines of your logs, combine it with tail
:
docker logs --tail 100 -f <container-id>
Resources:
For further reading, you can explore the Docker logs documentation at Docker Logging Driver.
3. Volume Permission Issues
Problem:
Docker volumes are crucial for data persistence, but you may encounter permission issues when your application running inside a container cannot access the mounted volume.
Solution:
Addressing volume permission issues can be tackled through:
-
Setting Correct Owner: Ensure the user running the application inside the container has the appropriate permissions on the volume. You can set ownership during build using the
RUN
command:📄snippet.txtRUN chown -R user:user /path/to/volume
-
Use User Flags: You can specify the user while running the container to bypass permission issues:
🔧snippet.shdocker run -u user:user -v /host/path:/container/path <image-name>
Resources:
For an introduction to Docker volume management, you can review Docker Volumes.
4. Network Issues
Problem:
Networking can be a complex aspect of Docker, especially when containers cannot communicate with each other or external services. Issues can arise from network configurations or firewall settings.
Solution:
When faced with networking problems:
-
Inspect Network Settings: Use the following command to inspect the current network settings:
🔧snippet.shdocker network inspect <network-name>
-
Check Firewall Rules: Ensure that your firewall allows traffic on the ports used by your containers.
-
Use Docker’s Built-In DNS: Docker provides internal DNS resolution for containers. To access another container, you can directly use its name.
Example of a Docker Compose service using network:
version: '3'
services:
app:
image: my-app
networks:
- my-network
db:
image: postgres
networks:
- my-network
networks:
my-network:
In this snippet, both app
and db
containers can communicate over the my-network
. This eliminates the need for complex networking setups.
Resources:
To gain a deeper understanding of Docker networking, visit Docker Networking.
5. Docker Daemon Not Running
Problem:
Occasionally, the Docker daemon may not be running, resulting in an inability to run Docker commands.
Solution:
To resolve issues with the Docker daemon:
-
Start Docker Service: On most systems, you can start the Docker service with:
🔧snippet.shsudo systemctl start docker
-
Check Status: It’s good practice to check if Docker is running:
🔧snippet.shsudo systemctl status docker
-
Reboot Your System: If the service fails to start, it’s helpful to restart your machine.
Resources:
Learn more about managing the Docker service at Docker Engine Installation.
Bringing It All Together
Docker issues can be largely addressed by understanding how to troubleshoot effectively. By recognizing these common problems and applying the solutions detailed above, you will enhance your experience with Docker and containerization. Continuously keeping an eye on network settings, verifying logs, and managing volumes is crucial for maintaining a smooth workflow.
To stay updated on the latest Docker practices, consider subscribing to Docker’s official blog. Happy Dockering!