Why Strong Cryptography is Crucial for Data Security Today
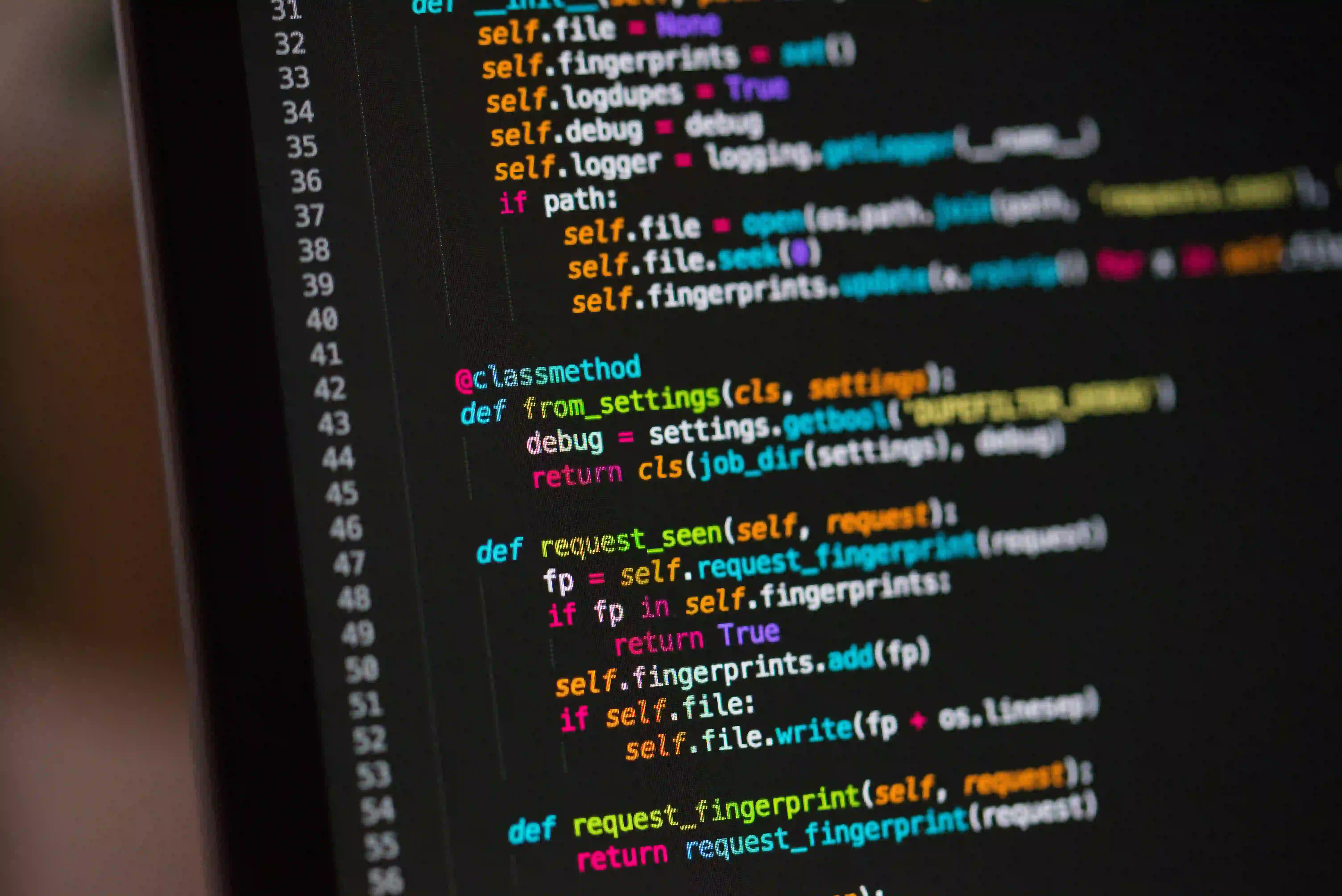
Why Strong Cryptography is Crucial for Data Security Today
In our increasingly digital world, data security has become a paramount concern for individuals and organizations alike. Every day, we rely on technology to manage our sensitive information, from personal identification numbers to corporate secrets. One of the cornerstones of data security is strong cryptography. Understanding its significance is not only important for tech enthusiasts but also for anyone comprehending the realities of modern data interactions.
What is Cryptography?
Cryptography is the practice and study of techniques for securing communication and information. It involves converting data into a format that is unreadable for unauthorized users while allowing authorized users to decipher it easily. This transformation is vital in cases where sensitive data could be intercepted.
Modern cryptography utilizes complex algorithms to enhance security. These algorithms can be classified into two main types:
- Symmetric Key Algorithms: These use a single key for both encryption and decryption. Examples include AES (Advanced Encryption Standard) and DES (Data Encryption Standard).
- Asymmetric Key Algorithms: These use a pair of keys—one public and one private. RSA (Rivest–Shamir–Adleman) is the most notable example.
The Importance of Strong Cryptography
- Protection Against Data Breaches
Data breaches continue to plague organizations and individuals, with hackers becoming increasingly sophisticated. By employing strong cryptography, you add a robust layer of protection against unauthorized access.
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class SymmetricEncryptionExample {
public static void main(String[] args) throws Exception {
// Generate a strong key for AES encryption
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256); // Key size
SecretKey secretKey = keyGen.generateKey();
// Create a Cipher instance for AES
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
// Example data to encrypt
String originalData = "Sensitive Information";
byte[] encryptedData = cipher.doFinal(originalData.getBytes());
System.out.println("Encrypted Data: " + new String(encryptedData));
}
}
In the above example, we generate a 256-bit AES key that provides a high level of security. The data is transformed into an encrypted format that is nearly impossible to decipher without the key. It's a straightforward yet effective method for ensuring sensitive information remains private.
- Ensures Data Integrity
Data integrity assures that the information remains unchanged during transmission. With strong cryptography, you can use hashing algorithms, such as SHA-256, to create a unique digital fingerprint of the data.
import java.security.MessageDigest;
public class DataIntegrityExample {
public static void main(String[] args) throws Exception {
String dataToHash = "Data Integrity Check";
// Create a SHA-256 hash
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(dataToHash.getBytes());
System.out.println("Hash: " + bytesToHex(hash));
}
// Helper method to convert bytes to hex format
private static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
This code snippet generates a SHA-256 hash from the original data. Any change in the data will result in a different hash, allowing users to verify if the content has been tampered with. This feature is indispensable for file transfers, digital signatures, and secure communications.
- Facilitates Secure Communications
Consider email interactions, online banking, or even social media messages—encryption is essential to ensure that only intended parties can read the messages.
With asymmetric cryptography, you can securely exchange data, making it impossible for eavesdroppers to decipher.
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import javax.crypto.Cipher;
public class AsymmetricEncryptionExample {
public static void main(String[] args) throws Exception {
// Generate a key pair for RSA
KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("RSA");
keyPairGen.initialize(2048);
KeyPair keyPair = keyPairGen.generateKeyPair();
// Get public and private keys
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// Encrypt and decrypt a message
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
String message = "Hello, Secure World!";
byte[] encryptedMessage = cipher.doFinal(message.getBytes());
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedMessage = cipher.doFinal(encryptedMessage);
System.out.println("Decrypted Message: " + new String(decryptedMessage));
}
}
In this example, RSA is used to encrypt and decrypt a secure message. Only the holder of the private key can decrypt the message, ensuring secure communications even in hostile environments.
- Compliance with Regulations
Organizations must often comply with data protection regulations, such as GDPR in Europe or HIPAA in the United States. Strong cryptography can aid in meeting these legal obligations, protecting sensitive data, and avoiding hefty fines.
Implementing encryption is not just a technical decision but a strategic business choice. Read more about data privacy regulations on the European Commission website for a comprehensive understanding of compliance needs.
- Building Trust
When your customers know that their data is protected using robust cryptography, it helps build trust. Confidence in data security can differentiate your business from competitors, fostering long-term relationships.
Challenges in Implementing Strong Cryptography
Despite its advantages, there are challenges associated with implementing strong cryptography. Some of these include:
- Performance: Strong encryption can demand significant resources, especially if excessive data needs to be encrypted or decrypted frequently.
- Key Management: Securely storing and managing cryptographic keys is crucial. If keys are lost or exposed, the security of the data is compromised. Explore best practices for key management here.
- User Education: Users may not always understand the importance of encryption, leading to poor implementation practices.
The Future of Cryptography
As technology evolves, so do the threats. Post-quantum cryptography is an emerging field that aims to develop cryptographic systems resilient against quantum computer attacks. This is crucial as quantum technology grows, threatening existing encryption methods in the near future.
Additionally, blockchain technology introduces unique cryptographic challenges and opportunities, redefining how we think about authenticity and security in various applications.
Bringing It All Together
Strong cryptography is not merely an accessory but a necessity in today's data-driven world. From securing communications to ensuring data integrity and compliance with privacy regulations, cryptography is indispensable in protecting sensitive information.
Implementing strong cryptographic measures safeguards individuals and organizations, building trust and ensuring security. As threats evolve, staying abreast of developments in cryptography will be key to navigating the ever-changing data security landscape.
By valuing and investing in strong cryptography, you contribute to a safer digital ecosystem for everyone. Always remember, when it comes to data, prevention is better than cure.