Common RDBMS Calculation Errors and How to Fix Them
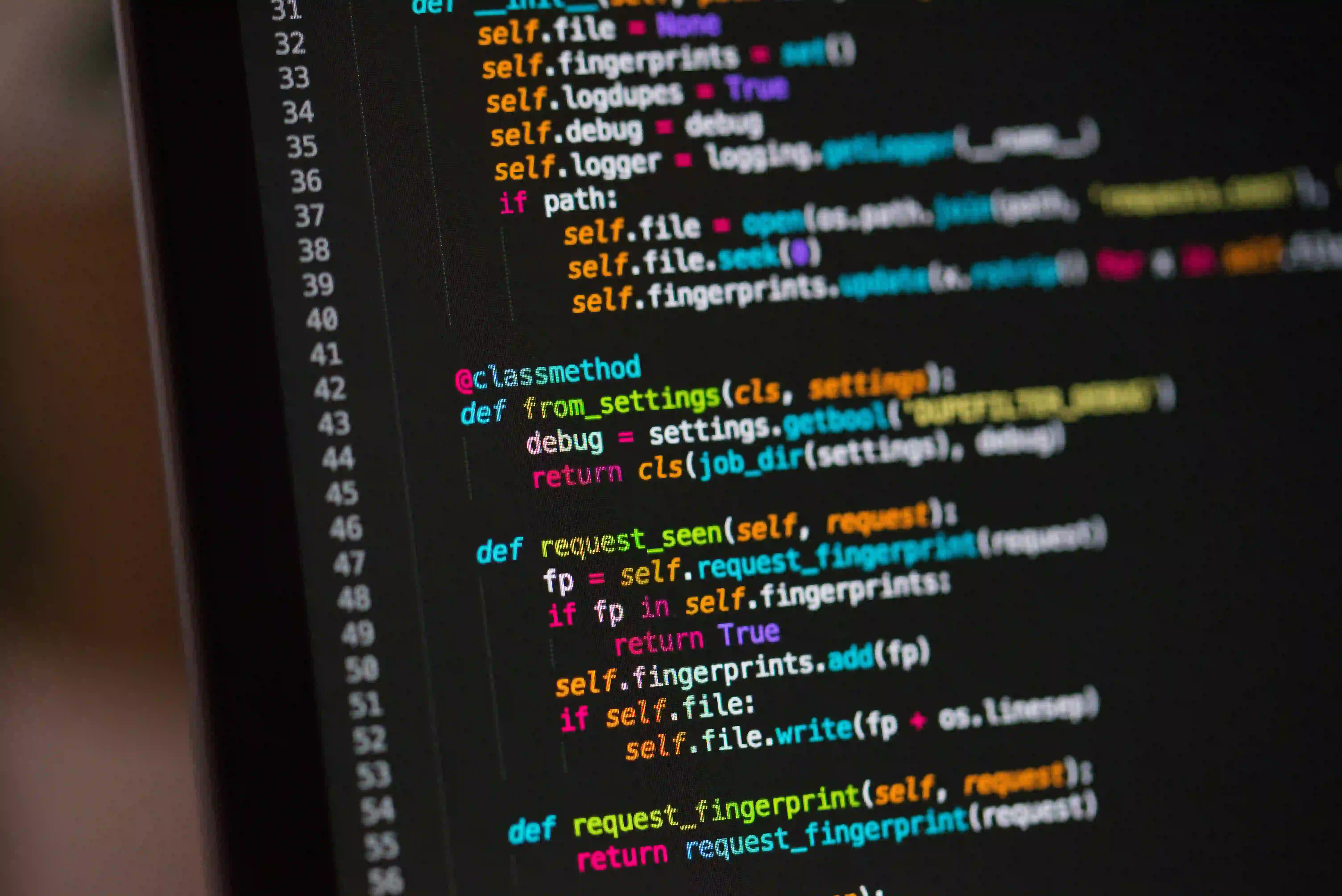
Common RDBMS Calculation Errors and How to Fix Them
Relational Database Management Systems (RDBMS) are pivotal in modern application development, providing a structured way to manage, store, and retrieve data. However, even seasoned developers can encounter calculation errors when working with SQL queries. This blog post will delve into common RDBMS calculation errors, provide insight into why these errors occur, and most importantly, offer solutions to fix them.
Table of Contents
The Approach
Working with RDBMS requires a careful approach, especially when dealing with calculations. Whether you are developing a financial application, managing inventory, or calculating user statistics, calculation errors can lead to inaccurate results, impairing decision-making processes. Let's explore some of the most frequent issues encountered during calculations in RDBMS and how to effectively resolve them.
Common Calculation Errors
1. Data Type Mismatch
Error Explanation
Data type mismatch occurs when an operation involves two different data types that cannot be implicitly converted or operated upon. For instance, trying to perform mathematical operations on a string and an integer can lead to unexpected results or errors.
Example
SELECT '10' + 5 AS Result; -- This will yield an error due to data type mismatch
Fix
Ensure that your data types align before performing calculations. You can cast or convert data types explicitly.
SELECT CAST('10' AS INT) + 5 AS Result; -- This will yield 15
2. Null Values
Error Explanation
Null values represent missing or unknown data. When performing calculations that involve null values, the result can often return null, which is misleading.
Example
SELECT salary + bonus AS TotalCompensation FROM Employees;
-- If bonus is null, TotalCompensation will be null for that record
Fix
Utilize the COALESCE
function or IFNULL
to provide default values when encountering nulls.
SELECT salary + COALESCE(bonus, 0) AS TotalCompensation FROM Employees;
-- This will treat null bonuses as zero, preventing null results
3. Integer Division
Error Explanation
Integer division is a common pitfall where dividing two integers yields an integer result, truncating any decimal places. This can lead to precision loss in financial calculations or other scenarios requiring decimal accuracy.
Example
SELECT 5 / 2 AS Result; -- This will return 2, rather than the expected 2.5
Fix
To ensure decimal precision, at least one operand in the division must be cast to a decimal type.
SELECT 5 / CAST(2 AS DECIMAL) AS Result; -- This will yield 2.5
4. Rounding Errors
Error Explanation
Rounding errors occur when performing calculations that require a fixed number of decimal places, but results are rounded incorrectly. This can lead to inaccuracies in reports and financial summaries.
Example
SELECT ROUND(123.4567, 2) AS Rounded; -- This results in 123.46
Fix
Double-check your rounding functions and set them appropriately to avoid misunderstandings. Use ROUND
, FLOOR
, or CEILING
functions as necessary.
SELECT ROUND(SUM(amount), 2) AS TotalRevenue
FROM Sales; -- Make sure to apply rounding after relevant calculations
5. Incorrect Aggregation
Error Explanation
Misunderstanding how aggregation works can result in returning non-representative values. For instance, applying an aggregate function without a proper GROUP BY
clause will lead to misleading data.
Example
SELECT product_id, AVG(price) FROM Products;
-- This will yield an error since product_id is not aggregated or grouped
Fix
Ensure you're using GROUP BY
appropriately.
SELECT product_id, AVG(price)
FROM Products
GROUP BY product_id; -- This will give the average price for each product
Bringing It All Together
Calculations in an RDBMS can be tricky, but understanding common pitfalls will equip developers and data analysts with the knowledge needed to navigate these issues effectively. Always validate data types, manage nulls gracefully, cast for precision, round results thoughtfully, and aggregate data correctly.
By following these best practices, the accuracy of calculations in your SQL queries can be significantly improved. With a deeper understanding of these common errors and their solutions, you can enhance the reliability of your RDBMS and make more informed business decisions.
For further reading on SQL data types and functions, consider checking out W3Schools SQL Data Types or the SQL Server documentation for more in-depth insights.
Happy querying!