Streamlining Java Applications with Multi-Database Connections
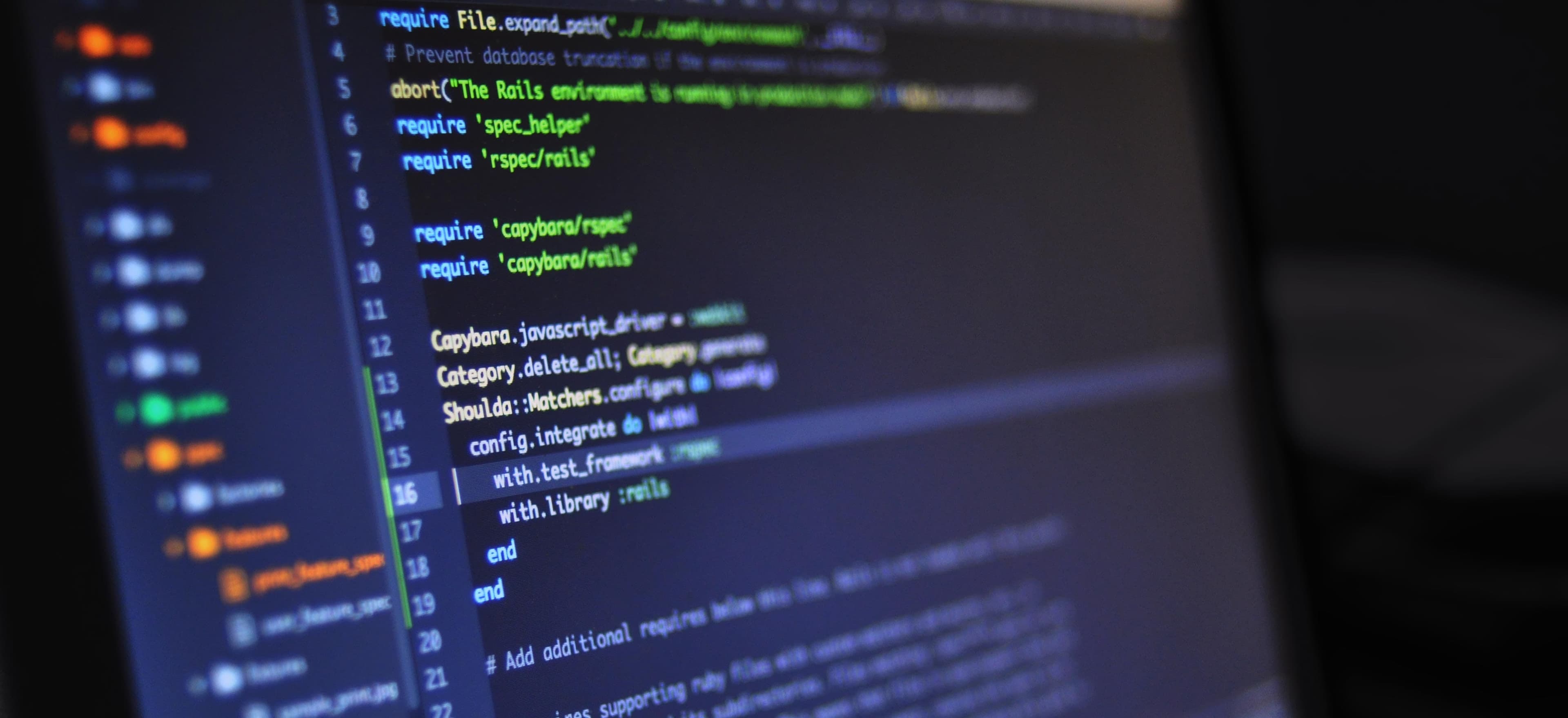
- Published on
Streamlining Java Applications with Multi-Database Connections
In today’s dynamic software landscape, applications often need to interact with multiple databases, whether due to architectural requirements or to optimize performance. Understanding how to effectively manage these multi-database connections in Java is essential for modern application development.
This blog post not only discusses ways to streamline Java applications using multi-database connections but also complements the insights from the article Simplifying Multi-Database Connections on One Host. We will explore best practices, useful libraries, and strategies to enhance scalability and maintainability.
Why Use Multiple Database Connections?
There are several reasons why one might choose to connect to multiple databases within a single application:
-
Data Segregation: Keeping different datasets in separate databases can lead to better organization and security.
-
Performance: Some databases may be optimized for specific operations. Your application might benefit from using a relational database for transactions while utilizing a NoSQL database for analytical processing.
-
Scalability: Horizontal scaling can be achieved effectively by distributing data across multiple databases.
-
Legacy Systems: In many enterprises, different departments may use distinct databases. Bridging these systems can be critical for coherent data flow.
Managing Multi-Database Connections in Java
Managing multiple database connections can be tricky, especially regarding the complexity of configuration and resource management. In Java, we can streamline these tasks through various methodologies which we will dive into below.
Using DataSource Interfaces
Java provides the DataSource
interface, which is a part of the Java Naming and Directory Interface (JNDI). This approach abstracts the database connections and allows us to manage them conveniently. Here’s how to set it up:
Configuring DataSources
First, configure your DataSources for each database in your application. Below is a simple example of creating DataSources for two different databases:
import javax.sql.DataSource;
import org.apache.commons.dbcp2.BasicDataSource;
public class DataSourceConfig {
public static DataSource getDataSource1() {
BasicDataSource ds = new BasicDataSource();
ds.setUrl("jdbc:mysql://localhost:3306/db1");
ds.setUsername("user1");
ds.setPassword("password1");
ds.setDriverClassName("com.mysql.cj.jdbc.Driver");
return ds;
}
public static DataSource getDataSource2() {
BasicDataSource ds = new BasicDataSource();
ds.setUrl("jdbc:postgresql://localhost:5432/db2");
ds.setUsername("user2");
ds.setPassword("password2");
ds.setDriverClassName("org.postgresql.Driver");
return ds;
}
}
Why Use DataSource?
Using a DataSource decouples your application from the specific database you are using. It also manages database connections efficiently with connection pooling, which optimizes resource usage.
Enhancing Connection Management
Connecting to multiple databases means that you need a robust management strategy. Connection pooling libraries can facilitate this very well. For instance, Apache Commons DBCP or HikariCP offer efficient connection pooling.
Here's how to implement HikariCP for optimal performance:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class HikariCPDataSource {
private static HikariDataSource dataSource;
static {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/db1");
config.setUsername("user1");
config.setPassword("password1");
config.setMaximumPoolSize(10);
dataSource = new HikariDataSource(config);
}
public static HikariDataSource getDataSource() {
return dataSource;
}
}
Why Use HikariCP?
HikariCP is known for its performance and robustness, making it an excellent choice for modern Java applications that require rapid and efficient database connections. The connection pooling mechanism enhances speed and decreases the overhead of establishing connections.
Simplifying Code with DAO Patterns
Incorporate the Data Access Object (DAO) pattern to manage database operations while keeping your code clean and modular. Here is an example of a DAO for user data:
public class UserDAO {
private DataSource dataSource;
public UserDAO(DataSource dataSource) {
this.dataSource = dataSource;
}
public User getUserById(int id) {
String query = "SELECT * FROM users WHERE id = ?";
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(query)) {
statement.setInt(1, id);
ResultSet resultSet = statement.executeQuery();
if(resultSet.next()) {
return new User(resultSet.getInt("id"), resultSet.getString("name"));
}
} catch (SQLException e) {
e.printStackTrace(); // Log the exception properly in production
}
return null;
}
}
Why Use DAO?
The DAO pattern abstracts the database interactions and encapsulates the data access logic, maintaining a clean separation of concerns in your application architecture. This is increasingly important in applications that rely on multiple data sources.
Best Practices for Multi-Database Connections
While managing multi-database connections in your Java applications, consider the following best practices:
-
Pooling Connections: Always use connection pooling to improve performance and reduce overhead. Avoid creating a new connection for each database operation.
-
Error Handling: Implement comprehensive error handling. If one database connection fails, it should not impact the entire application.
-
Manage Transactions Wisely: Be cautious when dealing with transactions across multiple databases. Use a distributed transaction manager like JTA if you require consistent state across databases.
-
Configuration Management: Centralize your configuration (URLs, credentials) rather than scattering them across your codebase. Use environment variables or configuration files for this purpose.
-
Testing: Test connections to each database independently. Ensure that your application can handle variations in response times and errors from different sources.
Final Considerations
Managing multi-database connections in Java applications doesn't have to be cumbersome. By leveraging DataSource interfaces, enhancing connection management with pooling libraries, and implementing the DAO pattern, developers can streamline database operations, improve performance, and maintain a clean codebase.
For a deeper insight into effectively managing these connections, refer to the article Simplifying Multi-Database Connections on One Host.
By following the discussed best practices and strategies, you can create robust Java applications capable of efficiently handling multi-database architectures, paving the way for a scalable and maintainable system architecture.
Keep experimenting, keep coding, and embrace the beauty of well-structured, efficient database management!
Checkout our other articles