Mastering Multi-Database Connections in Java Applications
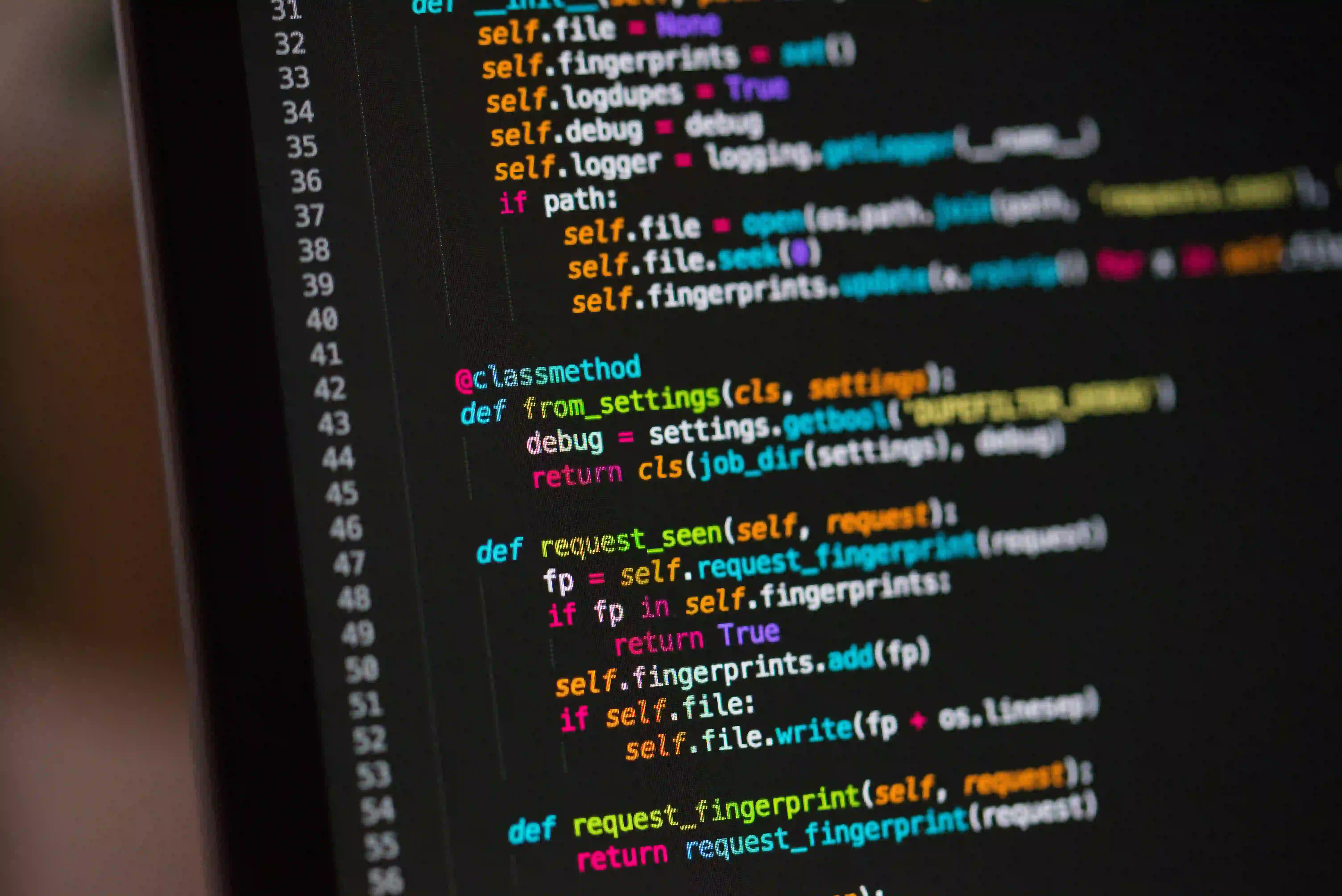
Mastering Multi-Database Connections in Java Applications
When developing Java applications, managing multiple database connections can often become a daunting task, especially as your application evolves. Understanding how to effectively connect and interact with multiple databases is essential for scalability and maintainability. In this blog post, we will dissect the intricacies of multi-database connections in Java, laying out frameworks, strategies, and best practices to enhance your application's performance.
Why Multiple Database Connections?
As applications grow, it is not uncommon for developers to leverage different databases for various components of an application. For example, a NoSQL database might be utilized for user-generated content while a relational database handles transactional data. Here are a few reasons why you might want to use multiple database connections:
- Technological Diversity: Different databases offer unique features. By leveraging multiple databases, you can take advantage of their respective strengths.
- Data Segregation: Data isolation can improve performance, security, and manageability.
- Scalability: As your database size increases, scaling out with additional databases might be more efficient than scaling up a single database.
Setting Up Multi-Database Connections in Java
Basic Database Configuration
Before we dive into coding, let's start by defining how to establish connections to multiple databases using Java's JDBC (Java Database Connectivity) API. Below, we'll set up two example databases: MySQL and PostgreSQL.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnections {
private Connection mysqlConn;
private Connection postgresConn;
public void connectToMySQL(String url, String user, String password) {
try {
mysqlConn = DriverManager.getConnection(url, user, password);
System.out.println("Successfully connected to MySQL");
} catch (SQLException e) {
System.err.println("MySQL Connection Error: " + e.getMessage());
}
}
public void connectToPostgreSQL(String url, String user, String password) {
try {
postgresConn = DriverManager.getConnection(url, user, password);
System.out.println("Successfully connected to PostgreSQL");
} catch (SQLException e) {
System.err.println("PostgreSQL Connection Error: " + e.getMessage());
}
}
}
Commentary on the Code
- DriverManager: It's a fundamental part of JDBC that manages a list of database drivers. Utilizing DriverManager allows you to connect to any database, given the right driver is on your classpath.
- Connection Object: Each connection object represents a single connection to a database and allows you to execute SQL commands.
- Error Handling: Proper error handling is crucial. Here, we catch
SQLException
and display a relevant message notifying the user of connection issues.
Configuration via Properties
Rather than hard-coding your database URL and credentials, consider using a properties file. This approach allows for easier changes without altering the Java code. Below is an example of how this might look.
import java.io.FileInputStream;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Properties;
public class DatabaseConnections {
private Connection mysqlConn;
private Connection postgresConn;
public void loadPropertiesAndConnect() {
Properties properties = new Properties();
try (FileInputStream input = new FileInputStream("config.properties")) {
properties.load(input);
connectToMySQL(properties.getProperty("mysql.url"),
properties.getProperty("mysql.user"),
properties.getProperty("mysql.password"));
connectToPostgreSQL(properties.getProperty("postgres.url"),
properties.getProperty("postgres.user"),
properties.getProperty("postgres.password"));
} catch (IOException e) {
System.err.println("Error loading properties: " + e.getMessage());
}
}
private void connectToMySQL(String url, String user, String password) {
// Connection code as defined previously
}
private void connectToPostgreSQL(String url, String user, String password) {
// Connection code as defined previously
}
}
Commentary on the Code
- Properties File: By leveraging a properties file, you can separate configuration from your codebase. This aligns with the principle of separation of concerns, enhancing maintainability.
- FileInputStream: It allows Java to read properties directly from the file system.
- Error Handling: The try-with-resources statement ensures that your file input stream is closed properly.
Connection Pooling
For better performance, particularly when your application demands high throughput, consider implementing connection pooling. Libraries like HikariCP or Apache DBCP manage a pool of connections, reducing the time spent establishing new connections.
<!-- Example Maven dependency for HikariCP -->
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.1</version>
</dependency>
Here’s a simple implementation using HikariCP:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
import java.sql.Connection;
import java.sql.SQLException;
public class ConnectionPool {
private HikariDataSource dataSource;
public ConnectionPool() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/yourdb");
config.setUsername("user");
config.setPassword("password");
dataSource = new HikariDataSource(config);
}
public Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
}
Commentary on the Code
- HikariConfig: This class contains configuration settings for creating connections.
- HikariDataSource: This is the connection pool management class. By using data sources instead of creating connections directly, efficiency is greatly improved.
- Performance: Connection pooling alleviates connection overhead, proving advantageous for applications requiring rapid database operations.
Best Practices
- Separation of Concerns: Maintain a clean architecture by keeping database logic separate from your business logic.
- Use ORM Tools: Consider using Object-Relational Mapping (ORM) tools such as Hibernate or JPA (Java Persistence API) to ease the burden of managing multiple connections and queries.
- Connection Lifecycle: Always close connections after use to prevent memory leaks.
- Configuration Management: Use environment variables or configuration management tools to manage database credentials securely.
Conclusion
Mastering multiple database connections in Java is vital for developing scalable applications. Using JDBC, proper configuration management, connection pooling, and adhering to best practices can greatly enhance your application's performance and manageability. If you want to explore more about managing database connections effectively, take a look at the article "Simplifying Multi-Database Connections on One Host" on Tech Snags.
With the right approaches and tools in your toolkit, handling multi-database connections can become a streamlined process rather than a complex challenge. Happy coding!